-
-
Save jackcoldrick90/b38268080650f8531650c2b47f681d8d to your computer and use it in GitHub Desktop.
const hubspot = require('@hubspot/api-client'); | |
exports.main = (event, callback) => { | |
const hubspotClient = new hubspot.Client({ | |
apiKey: process.env.HAPIKEY | |
}); | |
//1) Get the enrolled deal - referrer_id property value | |
hubspotClient.crm.contacts.basicApi.getById(event.object.objectId, ["referrer"]) | |
.then(results => { | |
let referrer = results.body.properties.referrer; //contact Referrer ID | |
console.log(referrer); | |
//2) Search for the contact using the deals referrer_id and update | |
const filter = { propertyName: 'referrer_id', operator: 'EQ', value: referrer } | |
const filterGroup = { filters: [filter] } | |
const sort = JSON.stringify({ propertyName: 'referrer_id', direction: 'DESCENDING' }) | |
const properties = ['referrer_id', 'total_referrals', 'firstname', 'lastname', 'email'] | |
const limit = 100 | |
const after = 0 | |
const publicObjectSearchRequest = { | |
filterGroups: [filterGroup], | |
sorts: [sort], | |
properties, | |
limit, | |
after, | |
} | |
hubspotClient.crm.contacts.searchApi.doSearch(publicObjectSearchRequest).then(results =>{ | |
let contactId = results.body.results[0].id; | |
let referred_by = results.body.results[0].properties.email | |
let totalReferrals = 0; | |
if(results.body.results[0].properties.total_referrals === null || results.body.results[0].properties.total_referrals === undefined || results.body.results[0].properties.total_referrals === ""){ | |
totalReferrals = 0; | |
}else{ | |
totalReferrals = parseInt(results.body.results[0].properties.total_referrals) | |
} | |
let totalReferralsUpdated = totalReferrals + 1; | |
var d = new Date(); | |
d.setUTCHours(0,0,0,0); | |
hubspotClient.crm.contacts.basicApi.update(contactId, {"properties":{"total_referrals": totalReferralsUpdated, "recent_referral_date": d}}); | |
callback({ | |
outputFields: { | |
reffered_by: referred_by | |
} | |
}); | |
}); | |
}); | |
} |
const hubspot = require('@hubspot/api-client'); | |
exports.main = (event, callback) => { | |
const hubspotClient = new hubspot.Client({ | |
apiKey: process.env.HAPIKEY | |
}); | |
const referrer = event.inputFields['referrer']; | |
console.log("REFERRED ID: " + referrer); | |
//2) Search for the contact using the deals referrer_id and update | |
const filter = { | |
propertyName: 'referrer_id', | |
operator: 'EQ', | |
value: referrer | |
} | |
const filterGroup = { | |
filters: [filter] | |
} | |
const sort = JSON.stringify({ | |
propertyName: 'referrer_id', | |
direction: 'DESCENDING' | |
}) | |
const properties = ['referrer_id', 'total_referrals', 'firstname', 'lastname', 'email'] | |
const limit = 100 | |
const after = 0 | |
const publicObjectSearchRequest = { | |
filterGroups: [filterGroup], | |
sorts: [sort], | |
properties, | |
limit, | |
after, | |
} | |
hubspotClient.crm.contacts.searchApi.doSearch(publicObjectSearchRequest).then(results => { | |
let contactId = results.body.results[0].id; | |
let referrer_email = results.body.results[0].properties.email | |
let totalReferrals = 0; | |
if (results.body.results[0].properties.total_referrals === null || results.body.results[0].properties.total_referrals === undefined || results.body.results[0].properties.total_referrals === "") { | |
totalReferrals = 0; | |
} else { | |
totalReferrals = parseInt(results.body.results[0].properties.total_referrals) | |
} | |
let totalReferralsUpdated = totalReferrals + 1; | |
var d = new Date(); | |
d.setUTCHours(0, 0, 0, 0); | |
//Update Referred Contact | |
hubspotClient.crm.contacts.basicApi.update(event.object.objectId, { | |
"properties": { | |
"referred_by": referrer_email | |
} | |
}) | |
//Update Referring Contact | |
hubspotClient.crm.contacts.basicApi.update(contactId, { | |
"properties": { | |
"total_referrals": totalReferralsUpdated, | |
"recent_referral_date": d | |
} | |
}) | |
}); | |
} |
const hubspot = require('@hubspot/api-client'); | |
exports.main = (event, callback) => { | |
const hubspotClient = new hubspot.Client({ | |
apiKey: process.env.HAPIKEY | |
}); | |
//1) Get the enrolled deal - referrer_id property value | |
hubspotClient.crm.contacts.basicApi.getById(event.object.objectId, ["referrer"]) | |
.then(results => { | |
let referrer = results.body.properties.referrer; //contact Referrer ID | |
//2) Search for the contact using the deals referrer_id and update | |
const filter = { propertyName: 'referrer_id', operator: 'EQ', value: referrer } | |
const filterGroup = { filters: [filter] } | |
const sort = JSON.stringify({ propertyName: 'referrer_id', direction: 'DESCENDING' }) | |
const properties = ['referrer_id', 'total_referrals', 'firstname', 'lastname'] | |
const limit = 100 | |
const after = 0 | |
const publicObjectSearchRequest = { | |
filterGroups: [filterGroup], | |
sorts: [sort], | |
properties, | |
limit, | |
after, | |
} | |
hubspotClient.crm.contacts.searchApi.doSearch(publicObjectSearchRequest).then(results =>{ | |
let contactId = results.body.results[0].id; | |
let totalReferrals = 0; | |
if(results.body.results[0].properties.total_referrals === null || results.body.results[0].properties.total_referrals === undefined || results.body.results[0].properties.total_referrals === ""){ | |
totalReferrals = 0; | |
}else{ | |
totalReferrals = parseInt(results.body.results[0].properties.total_referrals) | |
} | |
let totalReferralsUpdated = totalReferrals + 1; | |
var d = new Date(); | |
d.setUTCHours(0,0,0,0); | |
hubspotClient.crm.contacts.basicApi.update(contactId, {"properties":{"total_referrals": totalReferralsUpdated, "recent_referral_date": d}}); | |
}); | |
}); | |
} |
Thanks for getting back! @jackcoldrick90
I made that change: Receiving error below.. I have attached images. Am I missing something?
2023-07-14T17:17:17.322Z INFO REFERRED ID: KXPPL2
2023-07-14T17:17:18.042Z ERROR Unhandled Promise Rejection {"errorType":"Runtime.UnhandledPromiseRejection","errorMessage":"HttpError: HTTP request failed","reason":{"errorType":"HttpError","errorMessage":"HTTP request failed","response":{"statusCode":401,"body":{"status":"error","message":"Authentication credentials not found. This API supports OAuth 2.0 authentication and you can find more details at https://developers.hubspot.com/docs/methods/auth/oauth-overview","correlationId":"fbf1dbde-63ca-4165-9f59-786a909642da","category":"INVALID_AUTHENTICATION"},"headers":{"date":"Fri, 14 Jul 2023 17:17:17 GMT","content-type":"application/json;charset=utf-8","content-length":"299","connection":"close","cf-ray":"7e6b6b1aede32d0a-IAD","cf-cache-status":"DYNAMIC","strict-transport-security":"max-age=31536000; includeSubDomains; preload","vary":"origin","access-control-allow-credentials":"false","x-envoy-upstream-service-time":"2","x-evy-trace-listener":"listener_https","x-evy-trace-route-configuration":"listener_https/all","x-evy-trace-route-service-name":"envoyset-translator","x-evy-trace-served-by-pod":"iad02/hubapi-td/envoy-proxy-598c95b5b7-xrsrb","x-evy-trace-virtual-host":"all","x-hubspot-auth-failure":"401 Unauthorized","x-hubspot-correlation-id":"fbf1dbde-63ca-4165-9f59-786a909642da","x-request-id":"fbf1dbde-63ca-4165-9f59-786a909642da","x-trace":"2B66AAB7DBF8AF31E1C80664D4651EA4B9CD2099EC000000000000000000","report-to":"{"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v3?s=eIdw3Wio0o8Mo8Ydgljz5Y1PWDqRrsXG%2FA128P7fzqAOCiqmT2zDBWTD3ve1QDqiQjJFv6KYxddRyNlf%2BefKXcMkta0xPrWWTfm8Z96IJQIMZOlZ5N3MzlwguzNX88Ep"}],"group":"cf-nel","max_age":604800}","nel":"{"success_fraction":0.01,"report_to":"cf-nel","max_age":604800}","server":"cloudflare","alt-svc":"h3=":443"; ma=86400"},"request":{"uri":{"protocol":"https:","slashes":true,"auth":null,"host":"api.hubapi.com","port":443,"hostname":"api.hubapi.com","hash":null,"search":null,"query":null,"pathname":"/crm/v3/objects/contacts/search","path":"/crm/v3/objects/contacts/search","href":"https://api.hubapi.com/crm/v3/objects/contacts/search"},"method":"POST","headers":{"User-Agent":"hubspot-api-client-nodejs; 3.4.1","Accept":"application/json","content-type":"application/json","content-length":272}}},"body":{},"statusCode":401,"name":"HttpError","stack":["HttpError: HTTP request failed"," at Request._callback (/opt/nodejs/node_modules/@hubspot/api-client/lib/codegen/crm/contacts/api/searchApi.js:151:40)"," at Request.self.callback (/opt/nodejs/node_modules/request/request.js:185:22)"," at Request.emit (node:events:513:28)"," at Request. (/opt/nodejs/node_modules/request/request.js:1154:10)"," at Request.emit (node:events:513:28)"," at IncomingMessage. (/opt/nodejs/node_modules/request/request.js:1076:12)"," at Object.onceWrapper (node:events:627:28)"," at IncomingMessage.emit (node:events:525:35)"," at endReadableNT (node:internal/streams/readable:1358:12)"," at processTicksAndRejections (node:internal/process/task_queues:83:21)"]},"promise":{},"stack":["Runtime.UnhandledPromiseRejection: HttpError: HTTP request failed"," at process. (file:///var/runtime/index.mjs:1189:17)"," at process.emit (node:events:513:28)"," at emit (node:internal/process/promises:140:20)"," at processPromiseRejections (node:internal/process/promises:274:27)"," at processTicksAndRejections (node:internal/process/task_queues:97:32)"]}
Unknown application error occurred
Runtime.Unknown
@jyejamesdisisto, your error stack reveals that you're getting an API authentication error. Please note that you need to name your secret HAPIKEY
and not PrivateApp_AccessToken
in this action (it differs from the first action in that way)
Hi @jackcoldrick90,
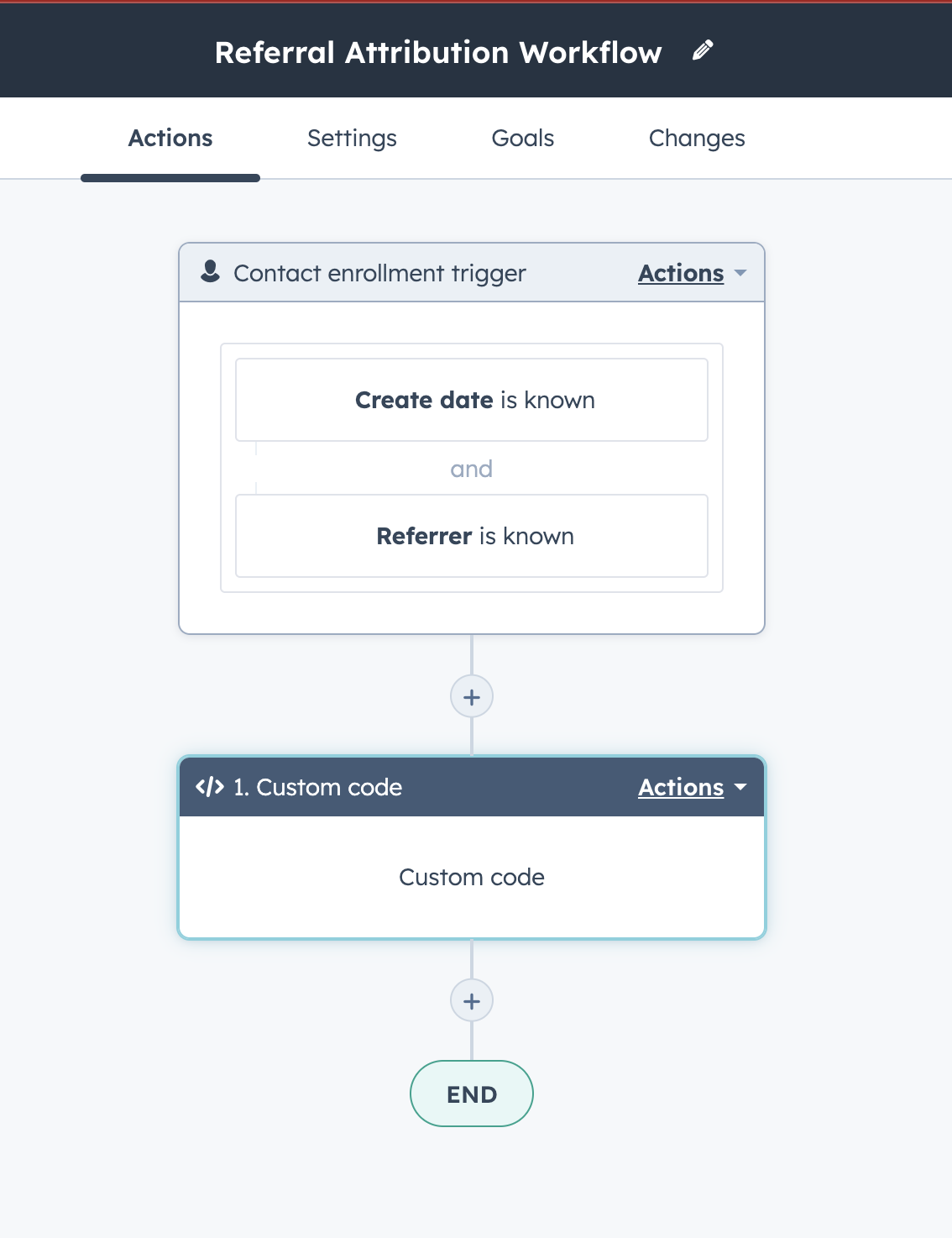
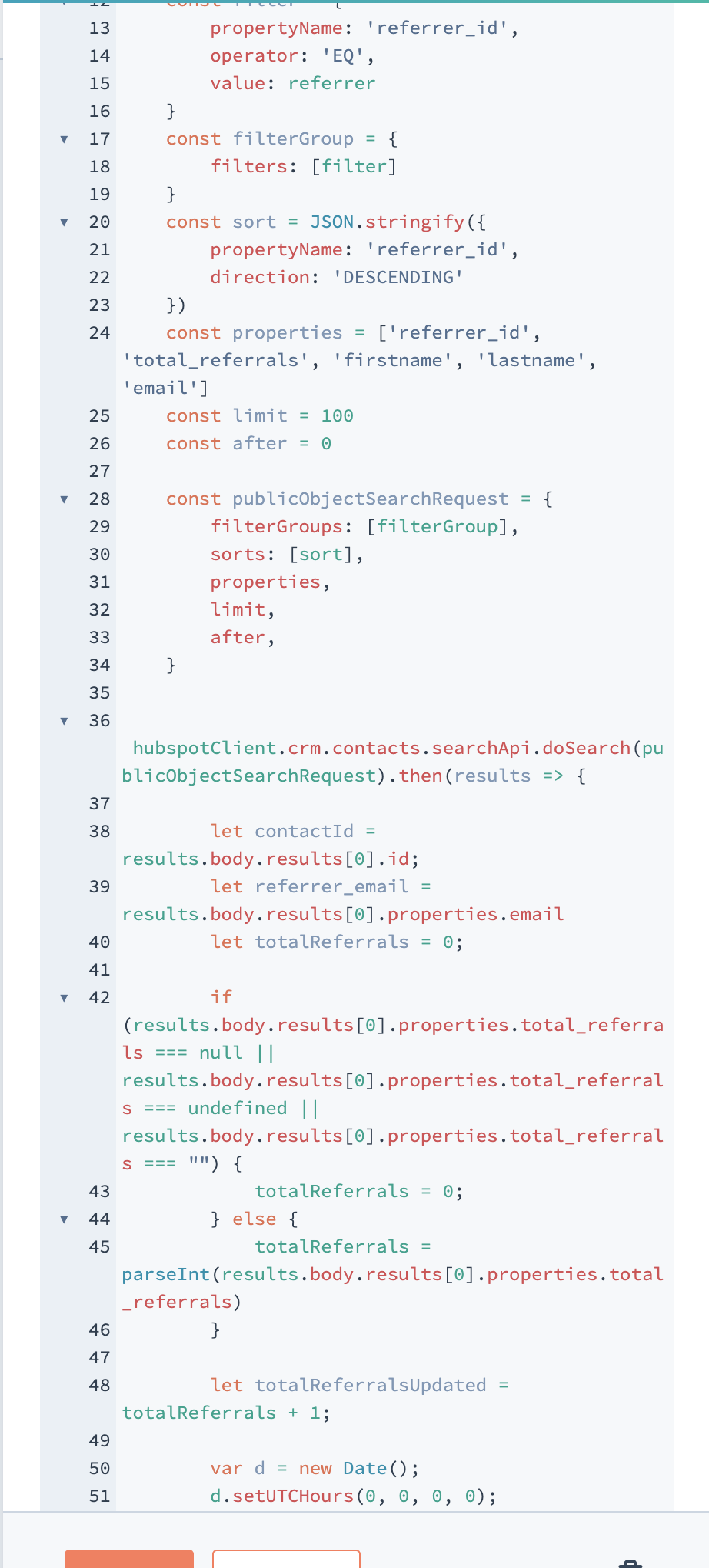
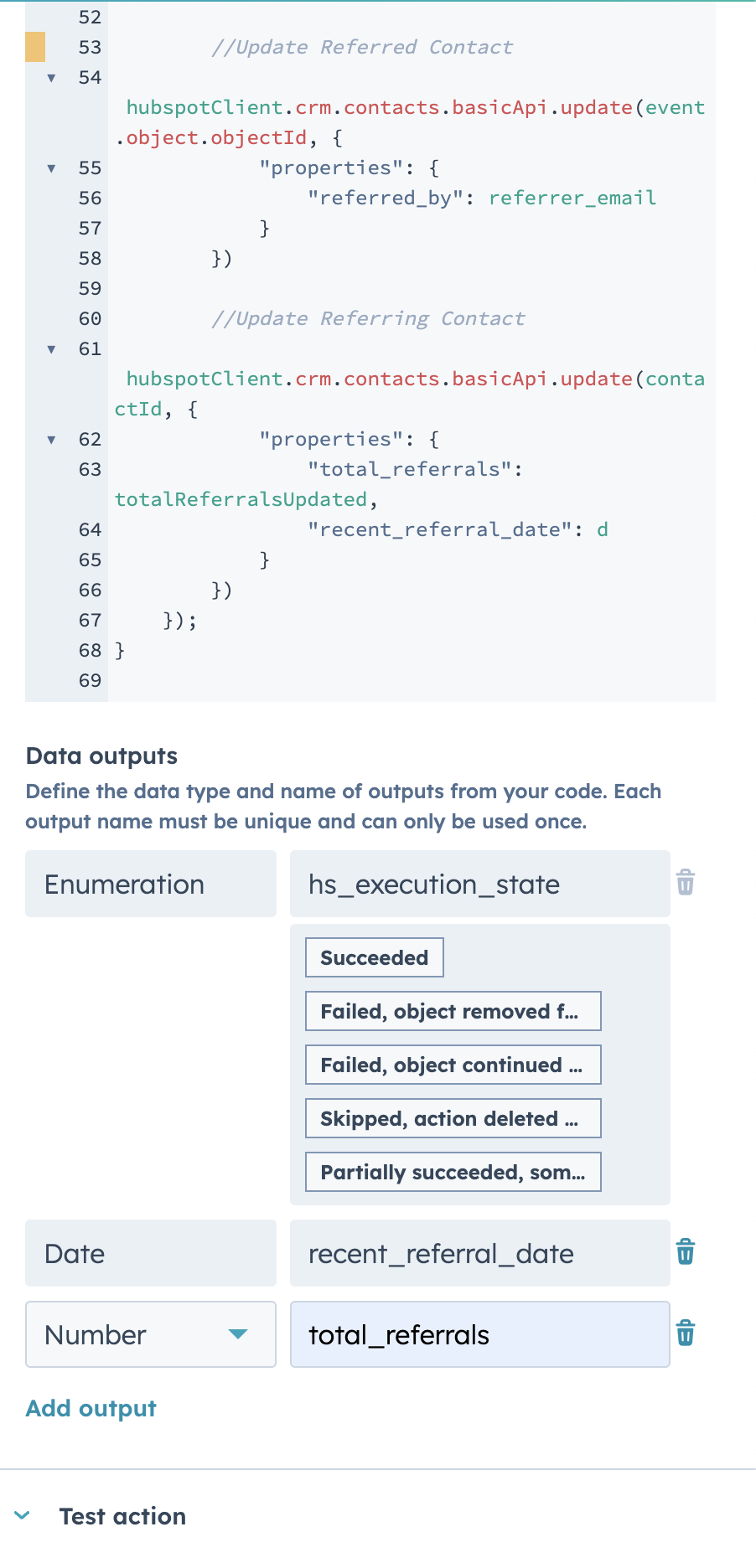
I have built your awesome HS referral program. I had no issues with the first workflow and custom code which is referring to the referrer ID. I am however having issues with the workflow attribution above - basically it is failing and the code is not updating total referrals or recent referral date. Any ideas as to what i am doing wrong?
<img width="469" alt="Screenshot 2023-07-13 a
t 11 11 14 AM" src="https://user-images.githubusercontent.co
m/139496878/253370064-5f723f9a-0a0b-4c6c-86db-7a2d6fad96b4.png">