Last active
September 15, 2022 06:52
-
-
Save anoop4real/deffa9bbed3741b6c3f19c07d8c87502 to your computer and use it in GitHub Desktop.
CapsuleSegmentedControl
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import UIKit | |
import PlaygroundSupport | |
// Ref: https://stackoverflow.com/questions/58315497/selectedtintcolor-of-segment-control-is-not-rounded-corner-on-ios-13 | |
class CapsuleSegmentedControl: UISegmentedControl { | |
override func layoutSubviews() { | |
super.layoutSubviews() | |
layer.cornerRadius = self.bounds.size.height / 2.0 | |
layer.borderColor = UIColor.gray.cgColor | |
layer.borderWidth = 1.0 | |
layer.masksToBounds = true | |
clipsToBounds = true | |
if #available(iOS 13.0, *) { | |
selectedSegmentTintColor = .clear | |
} else { | |
tintColor = .clear | |
} | |
for (index, subview) in subviews.enumerated() { | |
if ((subviews[index] as? UIImageView) != nil) && index == selectedSegmentIndex { | |
subview.backgroundColor = .white | |
subview.layer.cornerRadius = subview.bounds.size.height / 2.0 | |
} else { | |
subview.backgroundColor = .clear | |
} | |
} | |
} | |
} | |
class MyViewController : UIViewController { | |
override func loadView() { | |
let view = UIView() | |
view.backgroundColor = .white | |
self.view = view | |
setUpView() | |
} | |
private func setUpView() { | |
let segmentedControl = CapsuleSegmentedControl(items: ["One", "Two", "Three"]) | |
segmentedControl.translatesAutoresizingMaskIntoConstraints = false | |
segmentedControl.backgroundColor = UIColor.gray | |
view.addSubview(segmentedControl) | |
segmentedControl.selectedSegmentIndex = 0 | |
NSLayoutConstraint.activate([ | |
segmentedControl.leftAnchor.constraint(equalTo: view.leftAnchor, constant: 15), | |
segmentedControl.topAnchor.constraint(equalTo: view.topAnchor, constant: 44), | |
segmentedControl.heightAnchor.constraint(equalToConstant: 31) | |
]) | |
} | |
} | |
// Present the view controller in the Live View window | |
PlaygroundPage.current.liveView = MyViewController() | |
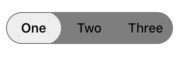 |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment