This gist is to create the smallest necessary contents to create a Python environment on macOS that can support running the Adafruit_Blinka library along with the blinka_displayio_pygamedisplay library. This primary purpose for this is to shorten the feedback look when developing and testing graphical elements for CircuitPython. This allows the developer to work locally on their computer, without the need to have a CircuitPython hardware environment on-hand to do tests.
While this was created specifically ON and FOR macOS, it should work on any other platform compatible with Python3. If it doesn't, I'm afraid I will be of no help to you. However, if you provide feedback what what doesn't work, or what change is needed, I'm happy to incorporate it.
The process to get this sample going is rather straightforward.
- Download the files from this gist
SimpleTest.py
CreateEnv.sh
- Put these files together in a directory where you'll be working from
- [Optional] Set the "execute" bit on
CreateEnv.sh
. - Execute
CreateEnv.sh
- If you did step 3, all you should need to do is
./CreateEnv.sh
- If you did not, you will need to call
CreateEnv.sh
with your prefered shell. Bash is assumed as a default.
- This will open the sample file in the system defined editor, from the
$EDITOR
environment variable, or its fallback.
- Take this opportunity to review the file, but don't make any changes, to ensure the first run is succesful with the test script as-is).
- When you exit the editor, the script will run. You should see a window that is 320x200 pixels, with a pleasing blue colour to it. To exit the application, quit as you would any other application.
The key element here, particularly for macOS, are on lines #10, #25 and #28
- Line #10: The value for the
auto_refresh
constructor parameter MUST beFalse
. - Line #25: The "forever" loop MUST be the runtime of the display window.
- Line #28: Calling
display.refresh()
is necessary to update the contents of the display window.
Since we've created a virtual environment (CreateEnv.sh
line #12) and then unloaded it (line #28), it will no longer be active once the script is done running. To do more work in this environment, make sure you load (activate) the virtual environment using the command source ./.venv/bin/activate
. When you're done, if you intend to keep your terminal open, use the command deactivate
, which will unload (deactivate) the virtual environment.
You can find more information about virtual Python environments using virtualenv on the project's documentation page.
A few notes of thanks and grartitude go to Adafruit for making such a wonderful ecosystem and community, to @foamyguy for the inspiration and "blinka_displayio_pygamedisplay" library, and the CircuitPython Community for help getting me going!
Just remember to take your time, have fun, and you've got this! I have faith you can do it!
👍
Hugo, huge thanks for writing up these instructions, I was able to get the blue screen on my Mac (running Big Sur). Yay!
Thanks for writing it up and making it easy to use!
Here's with a red box added to the blue box. Success!
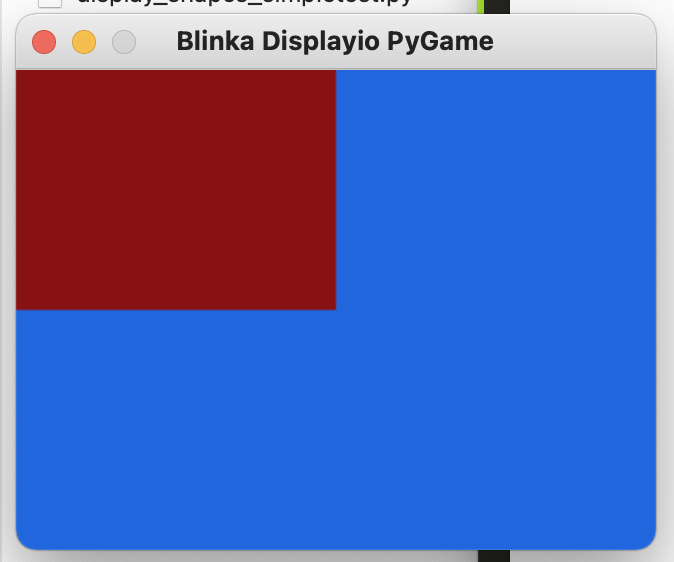
Two questions and one change I hade to make.
Question:
python3 CoolSnakes.py
by typing it onto the command line?Comment:
I had to add
source .venv/bin/activate
after creating the virtual environment. I found the instructions here, under the section called "Installing from PyPI".Here's my
CreateEnv.sh
file.