Last active
June 5, 2019 22:17
-
-
Save ryran/9c068ccb91614f5a033ef2d6ee74b443 to your computer and use it in GitHub Desktop.
uri-ify script: percent-encoding/decoding file content for OCPv4 MachineConfigs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/python3 | |
# Written May 2019 by Ryan Sawhill Aroha <rsaw@redhat.com> | |
# For dealing with OCPv4's MachineConfigs (which use Ignition) | |
# Ref: https://github.com/openshift/machine-config-operator | |
# Requires python v3.6+ | |
import urllib.parse | |
import os.path | |
from sys import argv, stdin, stderr, exit as sysexit | |
me = os.path.basename(argv[0]) | |
usage = f"Usage: {me} (-q/--quote | -u/--unquote) [FILE]" | |
content = None | |
def errquit(msg): | |
print(f"ERROR: {msg}\n{usage}", file=stderr) | |
sysexit(1) | |
# arg-validation | |
if not argv[1:] or argv[1] in ['-h', '--help']: | |
print(usage, file=stderr) | |
print("Use python3's urllib.parse to quote (percent-encode) or unquote content", file=stderr) | |
sysexit() | |
elif argv[1] not in '-q --quote -u --unquote'.split(): | |
errquit(f"Expecting either -q/--quote or -u/--unquote; not '{argv[1]}'") | |
elif not argv[2:]: | |
try: | |
content = stdin.read() | |
except KeyboardInterrupt: | |
print() | |
else: | |
print("-----") | |
if not content: | |
errquit("Either need a filename for input or input via stdin") | |
elif not os.path.isfile(argv[2]): | |
errquit(f"Expecting a filename for input; not '{argv[2]}' (alternatively: pass content via stdin)") | |
# read file | |
if not content: | |
with open(argv[2]) as fd: | |
content = fd.read() | |
# quote or unquote | |
if argv[1] in ['-q', '--quote']: | |
print(urllib.parse.quote(content)) | |
elif argv[1] in ['-u', '--unquote']: | |
print(urllib.parse.unquote(content)) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
In-action screenshot:
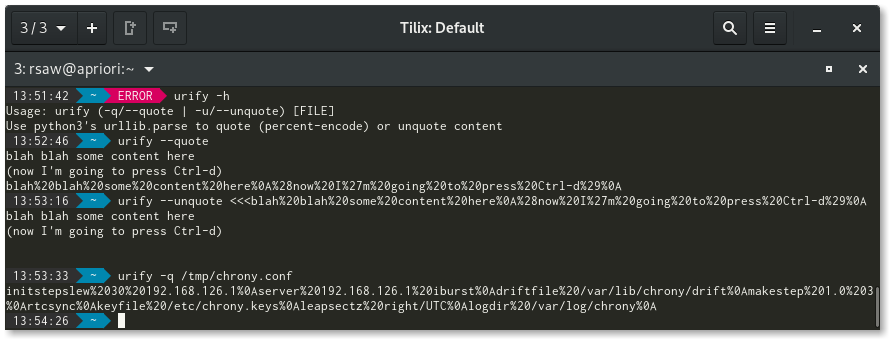