Last active
October 18, 2021 13:02
-
-
Save AakashKhatu/09eae5b54191f2e77138bc911b51b536 to your computer and use it in GitHub Desktop.
Edit deleted messages while quoting them on Whatsapp Web
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
window.f = {}; | |
// exposes the whatsapp API | |
function Ua() { | |
if (!window.f.Chat) { | |
var a = function (a) { | |
var d = 0, | |
b = [{ | |
id: "Store", | |
conditions: function (a) { | |
return a.Chat && a.Msg ? a : null | |
} | |
}, { | |
id: "MediaCollection", | |
conditions: function (a) { | |
return a["default"] && | |
a["default"].prototype && void 0 !== a["default"].prototype.processFiles ? a["default"] : null | |
} | |
}, { | |
id: "MediaProcess", | |
conditions: function (a) { | |
return a.BLOB ? a : null | |
} | |
}, { | |
id: "Wap", | |
conditions: function (a) { | |
return a.createGroup ? a : null | |
} | |
}, { | |
id: "ServiceWorker", | |
conditions: function (a) { | |
return a["default"] && a["default"].killServiceWorker ? a : null | |
} | |
}, { | |
id: "State", | |
conditions: function (a) { | |
return a.STATE && a.STREAM ? a : null | |
} | |
}, { | |
id: "WapDelete", | |
conditions: function (a) { | |
return a.sendConversationDelete && 2 == a.sendConversationDelete.length ? a : null | |
} | |
}, | |
{ | |
id: "Conn", | |
conditions: function (a) { | |
return a["default"] && a["default"].ref && a["default"].refTTL ? a["default"] : null | |
} | |
}, { | |
id: "WapQuery", | |
conditions: function (a) { | |
return a.queryExist ? a : a["default"] && a["default"].queryExist ? a["default"] : null | |
} | |
}, { | |
id: "CryptoLib", | |
conditions: function (a) { | |
return a.decryptE2EMedia ? a : null | |
} | |
}, { | |
id: "OpenChat", | |
conditions: function (a) { | |
return a["default"] && a["default"].prototype && a["default"].prototype.openChat ? a["default"] : null | |
} | |
}, { | |
id: "UserConstructor", | |
conditions: function (a) { | |
return a["default"] && | |
a["default"].prototype && a["default"].prototype.isServer && a["default"].prototype.isUser ? a["default"] : null | |
} | |
}, { | |
id: "SendTextMsgToChat", | |
conditions: function (a) { | |
return a.sendTextMsgToChat ? a.sendTextMsgToChat : null | |
} | |
}, { | |
id: "SendSeen", | |
conditions: function (a) { | |
return a.sendSeen ? a.sendSeen : null | |
} | |
}, { | |
id: "sendDelete", | |
conditions: function (a) { | |
return a.sendDelete ? a.sendDelete : null | |
} | |
} | |
], | |
c; | |
for (c in a) | |
if ("object" === typeof a[c] && null !== a[c]) { | |
var t = Object.values(a[c])[0]; | |
if ("object" === typeof t && t.exports) { | |
t = {}; | |
for (var Va in a[c]) { | |
t.$jscomp$loop$prop$module$13 = | |
a(Va); | |
if (t.$jscomp$loop$prop$module$13 && (b.forEach(function (a) { | |
return function (b) { | |
if (b.conditions && !b.foundedModule) { | |
var h = b.conditions(a.$jscomp$loop$prop$module$13); | |
null !== h && (d++ , b.foundedModule = h) | |
} | |
} | |
}(t)), d == b.length)) break; | |
t = { | |
$jscomp$loop$prop$module$13: t.$jscomp$loop$prop$module$13 | |
} | |
} | |
a = b.find(function (a) { | |
return "Store" === a.id | |
}); | |
window.f = a.foundedModule ? a.foundedModule : {}; | |
b.splice(b.indexOf(a), 1); | |
b.forEach(function (a) { | |
a.foundedModule && (window.f[a.id] = a.foundedModule) | |
}); | |
window.f.sendMessage = function (a) { | |
return window.f.SendTextMsgToChat.apply(window.f, | |
[this].concat($jscomp.arrayFromIterable(arguments))) | |
}; | |
return window.f | |
} | |
} | |
}; | |
webpackJsonp([], { | |
f: function (d, b, c) { | |
return a(c) | |
} | |
}, ["f"]) | |
} | |
} | |
Ua(); | |
// You can see the available functions for whatsapp web now using window.f | |
// helper functions for using the window.f methods | |
var $jscomp = $jscomp || {}; | |
$jscomp.scope = {}; | |
$jscomp.arrayIteratorImpl = function (b) { | |
var c = 0; | |
return function () { | |
return c < b.length ? { | |
done: !1, | |
value: b[c++] | |
} : { | |
done: !0 | |
} | |
} | |
}; | |
$jscomp.arrayIterator = function (b) { | |
return { | |
next: $jscomp.arrayIteratorImpl(b) | |
} | |
}; | |
$jscomp.makeIterator = function (b) { | |
var c = "undefined" != typeof Symbol && Symbol.iterator && b[Symbol.iterator]; | |
return c ? c.call(b) : $jscomp.arrayIterator(b) | |
}; | |
$jscomp.arrayFromIterator = function (b) { | |
for (var c, e = []; !(c = b.next()).done;) e.push(c.value); | |
return e | |
}; | |
$jscomp.arrayFromIterable = function (b) { | |
return b instanceof Array ? b : $jscomp.arrayFromIterator($jscomp.makeIterator(b)) | |
}; | |
$jscomp.getGlobal = function (b) { | |
return "undefined" != typeof window && window === b ? b : "undefined" != typeof global && null != global ? global : b | |
}; | |
$jscomp.global = $jscomp.getGlobal(this); | |
$jscomp.ASSUME_ES5 = !1; | |
$jscomp.ASSUME_NO_NATIVE_MAP = !1; | |
$jscomp.ASSUME_NO_NATIVE_SET = !1; | |
$jscomp.SIMPLE_FROUND_POLYFILL = !1; | |
$jscomp.defineProperty = $jscomp.ASSUME_ES5 || "function" == typeof Object.defineProperties ? Object.defineProperty : function (b, c, e) { | |
b != Array.prototype && b != Object.prototype && (b[c] = e.value) | |
}; | |
$jscomp.polyfill = function (b, c, e, r) { | |
if (c) { | |
e = $jscomp.global; | |
b = b.split("."); | |
for (r = 0; r < b.length - 1; r++) { | |
var q = b[r]; | |
q in e || (e[q] = {}); | |
e = e[q] | |
} | |
b = b[b.length - 1]; | |
r = e[b]; | |
c = c(r); | |
c != r && null != c && $jscomp.defineProperty(e, b, { | |
configurable: !0, | |
writable: !0, | |
value: c | |
}) | |
} | |
}; | |
$jscomp.FORCE_POLYFILL_PROMISE = !1; | |
$jscomp.polyfill("Promise", function (b) { | |
function c() { | |
this.batch_ = null | |
} | |
function e(b) { | |
return b instanceof q ? b : new q(function (c, y) { | |
c(b) | |
}) | |
} | |
if (b && !$jscomp.FORCE_POLYFILL_PROMISE) return b; | |
c.prototype.asyncExecute = function (b) { | |
if (null == this.batch_) { | |
this.batch_ = []; | |
var c = this; | |
this.asyncExecuteFunction(function () { | |
c.executeBatch_() | |
}) | |
} | |
this.batch_.push(b) | |
}; | |
var r = $jscomp.global.setTimeout; | |
c.prototype.asyncExecuteFunction = function (b) { | |
r(b, 0) | |
}; | |
c.prototype.executeBatch_ = function () { | |
for (; this.batch_ && this.batch_.length;) { | |
var b = | |
this.batch_; | |
this.batch_ = []; | |
for (var c = 0; c < b.length; ++c) { | |
var e = b[c]; | |
b[c] = null; | |
try { | |
e() | |
} catch (N) { | |
this.asyncThrow_(N) | |
} | |
} | |
} | |
this.batch_ = null | |
}; | |
c.prototype.asyncThrow_ = function (b) { | |
this.asyncExecuteFunction(function () { | |
throw b; | |
}) | |
}; | |
var q = function (b) { | |
this.state_ = 0; | |
this.result_ = void 0; | |
this.onSettledCallbacks_ = []; | |
var c = this.createResolveAndReject_(); | |
try { | |
b(c.resolve, c.reject) | |
} catch (D) { | |
c.reject(D) | |
} | |
}; | |
q.prototype.createResolveAndReject_ = function () { | |
function b(b) { | |
return function (y) { | |
e || (e = !0, b.call(c, y)) | |
} | |
} | |
var c = this, | |
e = !1; | |
return { | |
resolve: b(this.resolveTo_), | |
reject: b(this.reject_) | |
} | |
}; | |
q.prototype.resolveTo_ = function (b) { | |
if (b === this) this.reject_(new TypeError("A Promise cannot resolve to itself")); | |
else if (b instanceof q) this.settleSameAsPromise_(b); | |
else { | |
a: switch (typeof b) { | |
case "object": | |
var c = null != b; | |
break a; | |
case "function": | |
c = !0; | |
break a; | |
default: | |
c = !1 | |
} | |
c ? this.resolveToNonPromiseObj_(b) : this.fulfill_(b) | |
} | |
}; | |
q.prototype.resolveToNonPromiseObj_ = function (b) { | |
var c = void 0; | |
try { | |
c = b.then | |
} catch (D) { | |
this.reject_(D); | |
return | |
} | |
"function" == typeof c ? | |
this.settleSameAsThenable_(c, b) : this.fulfill_(b) | |
}; | |
q.prototype.reject_ = function (b) { | |
this.settle_(2, b) | |
}; | |
q.prototype.fulfill_ = function (b) { | |
this.settle_(1, b) | |
}; | |
q.prototype.settle_ = function (b, c) { | |
if (0 != this.state_) throw Error("Cannot settle(" + b + ", " + c + "): Promise already settled in state" + this.state_); | |
this.state_ = b; | |
this.result_ = c; | |
this.executeOnSettledCallbacks_() | |
}; | |
q.prototype.executeOnSettledCallbacks_ = function () { | |
if (null != this.onSettledCallbacks_) { | |
for (var b = 0; b < this.onSettledCallbacks_.length; ++b) v.asyncExecute(this.onSettledCallbacks_[b]); | |
this.onSettledCallbacks_ = null | |
} | |
}; | |
var v = new c; | |
q.prototype.settleSameAsPromise_ = function (b) { | |
var c = this.createResolveAndReject_(); | |
b.callWhenSettled_(c.resolve, c.reject) | |
}; | |
q.prototype.settleSameAsThenable_ = function (b, c) { | |
var e = this.createResolveAndReject_(); | |
try { | |
b.call(c, e.resolve, e.reject) | |
} catch (N) { | |
e.reject(N) | |
} | |
}; | |
q.prototype.then = function (b, c) { | |
function e(b, c) { | |
return "function" == typeof b ? function (c) { | |
try { | |
r(b(c)) | |
} catch (ma) { | |
y(ma) | |
} | |
} : c | |
} | |
var r, y, v = new q(function (b, c) { | |
r = b; | |
y = c | |
}); | |
this.callWhenSettled_(e(b, r), e(c, y)); | |
return v | |
}; | |
q.prototype["catch"] = function (b) { | |
return this.then(void 0, b) | |
}; | |
q.prototype.callWhenSettled_ = function (b, c) { | |
function e() { | |
switch (q.state_) { | |
case 1: | |
b(q.result_); | |
break; | |
case 2: | |
c(q.result_); | |
break; | |
default: | |
throw Error("Unexpected state: " + q.state_); | |
} | |
} | |
var q = this; | |
null == this.onSettledCallbacks_ ? v.asyncExecute(e) : this.onSettledCallbacks_.push(e) | |
}; | |
q.resolve = e; | |
q.reject = function (b) { | |
return new q(function (c, e) { | |
e(b) | |
}) | |
}; | |
q.race = function (b) { | |
return new q(function (c, q) { | |
for (var r = $jscomp.makeIterator(b), y = r.next(); !y.done; y = | |
r.next()) e(y.value).callWhenSettled_(c, q) | |
}) | |
}; | |
q.all = function (b) { | |
var c = $jscomp.makeIterator(b), | |
r = c.next(); | |
return r.done ? e([]) : new q(function (b, q) { | |
function y(c) { | |
return function (e) { | |
v[c] = e; | |
D--; | |
0 == D && b(v) | |
} | |
} | |
var v = [], | |
D = 0; | |
do v.push(void 0), D++ , e(r.value).callWhenSettled_(y(v.length - 1), q), r = c.next(); while (!r.done) | |
}) | |
}; | |
return q | |
}, "es6", "es3"); | |
$jscomp.SYMBOL_PREFIX = "jscomp_symbol_"; | |
$jscomp.initSymbol = function () { | |
$jscomp.initSymbol = function () { }; | |
$jscomp.global.Symbol || ($jscomp.global.Symbol = $jscomp.Symbol) | |
}; | |
$jscomp.SymbolClass = function (b, c) { | |
this.$jscomp$symbol$id_ = b; | |
$jscomp.defineProperty(this, "description", { | |
configurable: !0, | |
writable: !0, | |
value: c | |
}) | |
}; | |
$jscomp.SymbolClass.prototype.toString = function () { | |
return this.$jscomp$symbol$id_ | |
}; | |
$jscomp.Symbol = function () { | |
function b(e) { | |
if (this instanceof b) throw new TypeError("Symbol is not a constructor"); | |
return new $jscomp.SymbolClass($jscomp.SYMBOL_PREFIX + (e || "") + "_" + c++, e) | |
} | |
var c = 0; | |
return b | |
}(); | |
$jscomp.initSymbolIterator = function () { | |
$jscomp.initSymbol(); | |
var b = $jscomp.global.Symbol.iterator; | |
b || (b = $jscomp.global.Symbol.iterator = $jscomp.global.Symbol("Symbol.iterator")); | |
"function" != typeof Array.prototype[b] && $jscomp.defineProperty(Array.prototype, b, { | |
configurable: !0, | |
writable: !0, | |
value: function () { | |
return $jscomp.iteratorPrototype($jscomp.arrayIteratorImpl(this)) | |
} | |
}); | |
$jscomp.initSymbolIterator = function () { } | |
}; | |
$jscomp.initSymbolAsyncIterator = function () { | |
$jscomp.initSymbol(); | |
var b = $jscomp.global.Symbol.asyncIterator; | |
b || (b = $jscomp.global.Symbol.asyncIterator = $jscomp.global.Symbol("Symbol.asyncIterator")); | |
$jscomp.initSymbolAsyncIterator = function () { } | |
}; | |
$jscomp.iteratorPrototype = function (b) { | |
$jscomp.initSymbolIterator(); | |
b = { | |
next: b | |
}; | |
b[$jscomp.global.Symbol.iterator] = function () { | |
return this | |
}; | |
return b | |
}; | |
$jscomp.underscoreProtoCanBeSet = function () { | |
var b = { | |
a: !0 | |
}, | |
c = {}; | |
try { | |
return c.__proto__ = b, c.a | |
} catch (e) { } | |
return !1 | |
}; | |
$jscomp.setPrototypeOf = "function" == typeof Object.setPrototypeOf ? Object.setPrototypeOf : $jscomp.underscoreProtoCanBeSet() ? function (b, c) { | |
b.__proto__ = c; | |
if (b.__proto__ !== c) throw new TypeError(b + " is not extensible"); | |
return b | |
} : null; | |
$jscomp.generator = {}; | |
$jscomp.generator.ensureIteratorResultIsObject_ = function (b) { | |
if (!(b instanceof Object)) throw new TypeError("Iterator result " + b + " is not an object"); | |
}; | |
$jscomp.generator.Context = function () { | |
this.isRunning_ = !1; | |
this.yieldAllIterator_ = null; | |
this.yieldResult = void 0; | |
this.nextAddress = 1; | |
this.finallyAddress_ = this.catchAddress_ = 0; | |
this.finallyContexts_ = this.abruptCompletion_ = null | |
}; | |
$jscomp.generator.Context.prototype.start_ = function () { | |
if (this.isRunning_) throw new TypeError("Generator is already running"); | |
this.isRunning_ = !0 | |
}; | |
$jscomp.generator.Context.prototype.stop_ = function () { | |
this.isRunning_ = !1 | |
}; | |
$jscomp.generator.Context.prototype.jumpToErrorHandler_ = function () { | |
this.nextAddress = this.catchAddress_ || this.finallyAddress_ | |
}; | |
$jscomp.generator.Context.prototype.next_ = function (b) { | |
this.yieldResult = b | |
}; | |
$jscomp.generator.Context.prototype.throw_ = function (b) { | |
this.abruptCompletion_ = { | |
exception: b, | |
isException: !0 | |
}; | |
this.jumpToErrorHandler_() | |
}; | |
$jscomp.generator.Context.prototype["return"] = function (b) { | |
this.abruptCompletion_ = { | |
"return": b | |
}; | |
this.nextAddress = this.finallyAddress_ | |
}; | |
$jscomp.generator.Context.prototype.jumpThroughFinallyBlocks = function (b) { | |
this.abruptCompletion_ = { | |
jumpTo: b | |
}; | |
this.nextAddress = this.finallyAddress_ | |
}; | |
$jscomp.generator.Context.prototype.yield = function (b, c) { | |
this.nextAddress = c; | |
return { | |
value: b | |
} | |
}; | |
$jscomp.generator.Context.prototype.yieldAll = function (b, c) { | |
var e = $jscomp.makeIterator(b), | |
r = e.next(); | |
$jscomp.generator.ensureIteratorResultIsObject_(r); | |
if (r.done) this.yieldResult = r.value, this.nextAddress = c; | |
else return this.yieldAllIterator_ = e, this.yield(r.value, c) | |
}; | |
$jscomp.generator.Context.prototype.jumpTo = function (b) { | |
this.nextAddress = b | |
}; | |
$jscomp.generator.Context.prototype.jumpToEnd = function () { | |
this.nextAddress = 0 | |
}; | |
$jscomp.generator.Context.prototype.setCatchFinallyBlocks = function (b, c) { | |
this.catchAddress_ = b; | |
void 0 != c && (this.finallyAddress_ = c) | |
}; | |
$jscomp.generator.Context.prototype.setFinallyBlock = function (b) { | |
this.catchAddress_ = 0; | |
this.finallyAddress_ = b || 0 | |
}; | |
$jscomp.generator.Context.prototype.leaveTryBlock = function (b, c) { | |
this.nextAddress = b; | |
this.catchAddress_ = c || 0 | |
}; | |
$jscomp.generator.Context.prototype.enterCatchBlock = function (b) { | |
this.catchAddress_ = b || 0; | |
b = this.abruptCompletion_.exception; | |
this.abruptCompletion_ = null; | |
return b | |
}; | |
$jscomp.generator.Context.prototype.enterFinallyBlock = function (b, c, e) { | |
e ? this.finallyContexts_[e] = this.abruptCompletion_ : this.finallyContexts_ = [this.abruptCompletion_]; | |
this.catchAddress_ = b || 0; | |
this.finallyAddress_ = c || 0 | |
}; | |
$jscomp.generator.Context.prototype.leaveFinallyBlock = function (b, c) { | |
var e = this.finallyContexts_.splice(c || 0)[0]; | |
if (e = this.abruptCompletion_ = this.abruptCompletion_ || e) { | |
if (e.isException) return this.jumpToErrorHandler_(); | |
void 0 != e.jumpTo && this.finallyAddress_ < e.jumpTo ? (this.nextAddress = e.jumpTo, this.abruptCompletion_ = null) : this.nextAddress = this.finallyAddress_ | |
} else this.nextAddress = b | |
}; | |
$jscomp.generator.Context.prototype.forIn = function (b) { | |
return new $jscomp.generator.Context.PropertyIterator(b) | |
}; | |
$jscomp.generator.Context.PropertyIterator = function (b) { | |
this.object_ = b; | |
this.properties_ = []; | |
for (var c in b) this.properties_.push(c); | |
this.properties_.reverse() | |
}; | |
$jscomp.generator.Context.PropertyIterator.prototype.getNext = function () { | |
for (; 0 < this.properties_.length;) { | |
var b = this.properties_.pop(); | |
if (b in this.object_) return b | |
} | |
return null | |
}; | |
$jscomp.generator.Engine_ = function (b) { | |
this.context_ = new $jscomp.generator.Context; | |
this.program_ = b | |
}; | |
$jscomp.generator.Engine_.prototype.next_ = function (b) { | |
this.context_.start_(); | |
if (this.context_.yieldAllIterator_) return this.yieldAllStep_(this.context_.yieldAllIterator_.next, b, this.context_.next_); | |
this.context_.next_(b); | |
return this.nextStep_() | |
}; | |
$jscomp.generator.Engine_.prototype.return_ = function (b) { | |
this.context_.start_(); | |
var c = this.context_.yieldAllIterator_; | |
if (c) return this.yieldAllStep_("return" in c ? c["return"] : function (b) { | |
return { | |
value: b, | |
done: !0 | |
} | |
}, b, this.context_["return"]); | |
this.context_["return"](b); | |
return this.nextStep_() | |
}; | |
$jscomp.generator.Engine_.prototype.throw_ = function (b) { | |
this.context_.start_(); | |
if (this.context_.yieldAllIterator_) return this.yieldAllStep_(this.context_.yieldAllIterator_["throw"], b, this.context_.next_); | |
this.context_.throw_(b); | |
return this.nextStep_() | |
}; | |
$jscomp.generator.Engine_.prototype.yieldAllStep_ = function (b, c, e) { | |
try { | |
var r = b.call(this.context_.yieldAllIterator_, c); | |
$jscomp.generator.ensureIteratorResultIsObject_(r); | |
if (!r.done) return this.context_.stop_(), r; | |
var q = r.value | |
} catch (v) { | |
return this.context_.yieldAllIterator_ = null, this.context_.throw_(v), this.nextStep_() | |
} | |
this.context_.yieldAllIterator_ = null; | |
e.call(this.context_, q); | |
return this.nextStep_() | |
}; | |
$jscomp.generator.Engine_.prototype.nextStep_ = function () { | |
for (; this.context_.nextAddress;) try { | |
var b = this.program_(this.context_); | |
if (b) return this.context_.stop_(), { | |
value: b.value, | |
done: !1 | |
} | |
} catch (c) { | |
this.context_.yieldResult = void 0, this.context_.throw_(c) | |
} | |
this.context_.stop_(); | |
if (this.context_.abruptCompletion_) { | |
b = this.context_.abruptCompletion_; | |
this.context_.abruptCompletion_ = null; | |
if (b.isException) throw b.exception; | |
return { | |
value: b["return"], | |
done: !0 | |
} | |
} | |
return { | |
value: void 0, | |
done: !0 | |
} | |
}; | |
$jscomp.generator.Generator_ = function (b) { | |
this.next = function (c) { | |
return b.next_(c) | |
}; | |
this["throw"] = function (c) { | |
return b.throw_(c) | |
}; | |
this["return"] = function (c) { | |
return b.return_(c) | |
}; | |
$jscomp.initSymbolIterator(); | |
this[Symbol.iterator] = function () { | |
return this | |
} | |
}; | |
$jscomp.generator.createGenerator = function (b, c) { | |
var e = new $jscomp.generator.Generator_(new $jscomp.generator.Engine_(c)); | |
$jscomp.setPrototypeOf && $jscomp.setPrototypeOf(e, b.prototype); | |
return e | |
}; | |
$jscomp.asyncExecutePromiseGenerator = function (b) { | |
function c(c) { | |
return b.next(c) | |
} | |
function e(c) { | |
return b["throw"](c) | |
} | |
return new Promise(function (r, q) { | |
function v(b) { | |
b.done ? r(b.value) : Promise.resolve(b.value).then(c, e).then(v, q) | |
} | |
v(b.next()) | |
}) | |
}; | |
$jscomp.asyncExecutePromiseGeneratorFunction = function (b) { | |
return $jscomp.asyncExecutePromiseGenerator(b()) | |
}; | |
$jscomp.asyncExecutePromiseGeneratorProgram = function (b) { | |
return $jscomp.asyncExecutePromiseGenerator(new $jscomp.generator.Generator_(new $jscomp.generator.Engine_(b))) | |
}; | |
function ayo(x) { | |
if (x.target === x.currentTarget) { | |
d = this; | |
// gets the id of the selected message and stores it in variable c | |
for (i in d) i.startsWith("__reactInternalInstance$") && (c = d[i]["return"]["return"].key); | |
var b = $(this); | |
if (x.ctrlKey) { | |
a = prompt("Enter Your Reply", "Reply text"); | |
var text = prompt("Enter the Quoted Text", "deleted message text"); | |
c = void 0 !== c ? c.replace("msg-", "") : ""; | |
b && (b = window.f.Chat.where({ | |
__x_active: !0 | |
}), c = window.f.Msg.get(c), b[0].sendMessage = b[0].sendMessage ? b[0].sendMessage : function () { | |
return window.f.sendMessage.apply(this, | |
arguments) | |
}, void 0 !== c && ((c.__x_type = "chat", c.__x_body = text, c.__x_text = text), c.__x_isRevoked = !1), b[0].sendMessage(a, { | |
quotedMsg: c | |
})); | |
return !1 | |
} | |
} | |
} | |
$(document).on("click", ".message-in", ayo) |
Hi there,
I also have the same problem. Was anybody able to solve it?
I'm not very familiar with javascript and the DOM objects.
It would be great if a software developer with experience could take a look at this code an give hints how the problem can be solved.
Thank you very much!
same
Hi guys.
Any update on this issue? I've tried and had the same result.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hey there , since Whatsapp has updated this code doesn't fully work anymore. Maybe you can provide a modified/new code, it would help a lot . If you are short on time and cant, which is fully understandable , just mention on you post on hackernoon that this code doesn't work for the new updates. Thanks. Here is a screesnshot of the error.
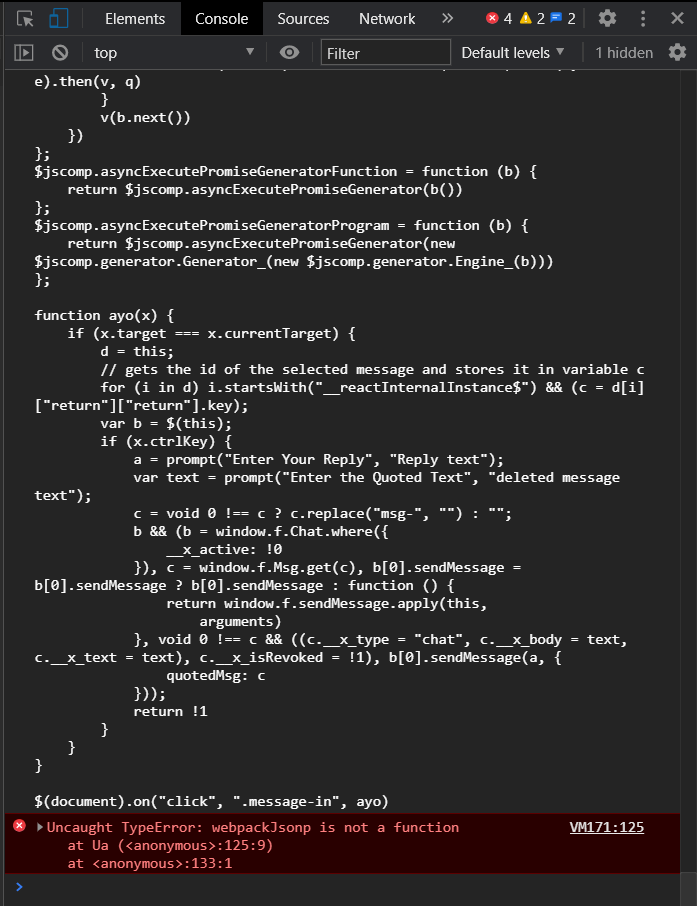