Last active
February 20, 2017 11:37
-
-
Save Cartman0/1ab25e1c8d5b782201847331983f9a23 to your computer and use it in GitHub Desktop.
基本情報技術者/technology/大分類1_基礎理論/中分類2:アルゴリズムとプログラミング/スタックとキュー
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"cells": [ | |
{ | |
"metadata": { | |
"toc": "true" | |
}, | |
"cell_type": "markdown", | |
"source": "# Table of Contents\n <p><div class=\"lev1 toc-item\"><a href=\"#大分類1-基礎理論-中分類2-アルゴリズムとプログラミング\" data-toc-modified-id=\"大分類1-基礎理論-中分類2-アルゴリズムとプログラミング-1\"><span class=\"toc-item-num\">1 </span>大分類1 基礎理論 中分類2 アルゴリズムとプログラミング</a></div><div class=\"lev2 toc-item\"><a href=\"#データ構造\" data-toc-modified-id=\"データ構造-11\"><span class=\"toc-item-num\">1.1 </span>データ構造</a></div><div class=\"lev3 toc-item\"><a href=\"#スタックとキュー\" data-toc-modified-id=\"スタックとキュー-111\"><span class=\"toc-item-num\">1.1.1 </span>スタックとキュー</a></div><div class=\"lev4 toc-item\"><a href=\"#スタック構造-stack-structure\" data-toc-modified-id=\"スタック構造-stack-structure-1111\"><span class=\"toc-item-num\">1.1.1.1 </span>スタック構造 stack structure</a></div><div class=\"lev5 toc-item\"><a href=\"#実装メモ(スタック)\" data-toc-modified-id=\"実装メモ(スタック)-11111\"><span class=\"toc-item-num\">1.1.1.1.1 </span>実装メモ(スタック)</a></div><div class=\"lev5 toc-item\"><a href=\"#code\" data-toc-modified-id=\"code-11112\"><span class=\"toc-item-num\">1.1.1.1.2 </span>code</a></div><div class=\"lev5 toc-item\"><a href=\"#参考(スタック)\" data-toc-modified-id=\"参考(スタック)-11113\"><span class=\"toc-item-num\">1.1.1.1.3 </span>参考(スタック)</a></div><div class=\"lev4 toc-item\"><a href=\"#キュー構造\" data-toc-modified-id=\"キュー構造-1112\"><span class=\"toc-item-num\">1.1.1.2 </span>キュー構造</a></div><div class=\"lev5 toc-item\"><a href=\"#実装メモ(キュー)\" data-toc-modified-id=\"実装メモ(キュー)-11121\"><span class=\"toc-item-num\">1.1.1.2.1 </span>実装メモ(キュー)</a></div><div class=\"lev5 toc-item\"><a href=\"#リングバッファでの実装\" data-toc-modified-id=\"リングバッファでの実装-11122\"><span class=\"toc-item-num\">1.1.1.2.2 </span>リングバッファでの実装</a></div><div class=\"lev5 toc-item\"><a href=\"#参考(キュー)\" data-toc-modified-id=\"参考(キュー)-11123\"><span class=\"toc-item-num\">1.1.1.2.3 </span>参考(キュー)</a></div>" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "# 大分類1 基礎理論 中分類2 アルゴリズムとプログラミング" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "## データ構造" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "### スタックとキュー" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "- [Gist](https://gist.github.com/Cartman0/1ab25e1c8d5b782201847331983f9a23)\n- [nbviewer](http://nbviewer.jupyter.org/gist/Cartman0/1ab25e1c8d5b782201847331983f9a23)" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "スタックとキュー\n\nスタックとキューの特徴,基本的な操作を理解する。\n\n用語例 \n- FIFO\n- LIFO\n- プッシュ\n- ポップ\n" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "#### スタック構造 stack structure" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "1次元配列に格納されたデータにおいて,\nあとから入れたものを先に出す構造.\n\n- スタックにデータを格納」pushプッシュ\n- 格納したデータを取り出す pop ポップ\n\nスタックの格納(push)と取り出し(pop)は,ともにリストの先頭に対して行われ,最も新しく格納されたデータが最初に取り出される.: **LIFO(Last-In First-Out)** 後入れ先出し\n\n```\n 3 データを積む push\n ↓\n|2|\n|1|\n---\n```\n\nFig.データをpush\n\n```\n3 データを取り出す pop\n↑\n|2|\n|1|\n---\n```\n\nFig.データをpop" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "##### 実装メモ(スタック)" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "- コンストラクタで `tail` を `0` とすることでスタックを空にする。\nこのとき配列(メモリ)の中に要素は残ったままですが、以降の push 操作によって上書きされる。\n\n```\n|Null| <- tail = 0\n```\n\nデータが1つ入ると,`tail`は1を指す.\n配列のindexと対応させると,\n`array[index] -> tail = index+1`\n```\n|data0| <- tail = 1\n```\n\n- `isEmpty` 関数は `tail` が `0` かどうかを調べスタックが空かどうかを判定します。\n\n```\n|Null| <- tail = 0\n```\n\n- `isFull` 関数はスタックが満杯かどうかを判定します。\n例えば、容量が `MAX` で定義されている場合は、`tail >= MAX` のときにスタックが満杯の状態になる。\n\n```\n|dataMax-1| <- tail=Max\n| | \n|data1 | \n|data0 | \n```\n\n- `push` 関数では tailの場所にデータを追加し,tail を1 つ増やす.\nただし、スタックが満杯の場合はなんらかのエラー処理を行う。\n\n- `pop` 関数では、`tail-1` が指す要素、つまりスタックの頂点の要素を返しつつ、`tail` の値を1つ減らすことで、頂点の要素を削除する。\nただし、スタックが空の場合はなんらかのエラー処理を行う。" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "##### code" | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:46.742713", | |
"end_time": "2017-02-20T19:40:46.779742" | |
}, | |
"trusted": true, | |
"collapsed": true | |
}, | |
"cell_type": "code", | |
"source": "class Stack:\n def __init__(self, max_size=100):\n self.tail = 0\n self.array = [None] * max_size\n self.bufferMax = max_size\n \n def isEmpty(self):\n return self.tail == 0\n \n def isFull(self):\n return self.tail >= self.bufferMax\n \n def push(self, data):\n if self.isFull():\n print(\"stack is full.\")\n return \n \n self.array[self.tail] = data\n self.tail += 1\n \n def pop(self):\n if self.isEmpty():\n print(\"stack is empty.\")\n return \n \n data = self.array[self.tail-1]\n self.tail -= 1\n return data\n \n def print(self):\n print(self.array[:self.tail])\n \n def get_array(self):\n return self.array[:self.tail]\n", | |
"execution_count": 1, | |
"outputs": [] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:46.783245", | |
"end_time": "2017-02-20T19:40:46.837545" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "s = Stack(max_size=3)\ns.pop()", | |
"execution_count": 2, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "stack is empty.\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:46.841547", | |
"end_time": "2017-02-20T19:40:46.877578" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "s.push(1)\ns.push(2)\ns.push(3)\ns.push(4)\ns.pop()", | |
"execution_count": 3, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "stack is full.\n", | |
"name": "stdout" | |
}, | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": "3" | |
}, | |
"metadata": {}, | |
"execution_count": 3 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:46.881583", | |
"end_time": "2017-02-20T19:40:46.887188" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "s.print()", | |
"execution_count": 4, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "[1, 2]\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "##### 参考(スタック)" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "参考:\n\n- http://judge.u-aizu.ac.jp/onlinejudge/commentary.jsp?id=ALDS1_3_A" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "#### キュー構造" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "平成22年 基本情報技術者 \n\n1次元配列で格納されたデータにおいて,\n一夫の端からデータを入れ他方の端からデータを利出す構造.\n**待ち行列**ともいう.\n\n- キューにデータを格納:**enqueue エンキュー**\n- キューからデータを取り出す:**dequeue デキュー**\n\nもっとも古いデータから取り出される.\n**FIFO: First-In First-Out 先入れ先出し**\n\n```\n -------------------\nデータ1 <- データ1 | データ2 | <- データ3\n --------------------\n取り出すdequeue 入れる enqueue\n```\nFig, キューのイメージ\n\n" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "##### 実装メモ(キュー)" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "- データを格納するための整数型 1 次元配列 : `Q`\n配列 `Q` の各要素に `enqueue` されたデータを格納する。\n問題に応じた十分な記憶領域を確保することが必要。\n上図では、既にいくつかの要素が格納されている。\n\n- 先頭ポインタである整数型変数:`head`\nキューの先頭の場所を指し示す変数。\n`dequeue` されると `head` で指されている要素が取り出される。キューの先頭の要素のインデックスが常に `0` とは限らないことに注意。\n\n- 末尾ポインタである整数型変数:`tail`\nキューの末尾`+1` の場所(最後の要素の隣)を指し示す変数。tail は新しい要素が追加される場所を示す。\n`head` と `tail` で挟まれた部分(tail が指す要素は含まない)が、キューの中身を表す。\n\n- キューに要素 x を追加する関数 `enqueue(data)`\n`Q[tail]` に `data` を格納し、`tail` を1つ増やす。\n\n- キューの先頭から要素を取り出す関数 `dequeue()`\n`Q[head]` の値を返し、`head` を1つ増やす。" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "流れ:\n\n(1). 初期\n\n```\n0 1 2 3 4 5\n\nh\nt\n```\n\n(2). enqueue(3)\n```\nq[tail=0] = 3\ntail + 1 = 1\n```\n```\n0 1 2 3 4 5\n3\nh\n t\n```\n\n(2). enqueue(7)\n```\nq[tail=1] = 7\ntail + 1 = 2\n```\n```\n0 1 2 3 4 5\n3 7\nh\n t\n```\n\n(3). enqueue(8)\n```\nq[tail=2] = 8\ntail + 1 = 3\n```\n```\n0 1 2 3 4 5\n3 7 8\nh\n t\n```\n\n(4). dequeue()\n```\nreturn q[head=0]:3\nhead + 1 = 1\n```\n```\n 0 1 2 3 4 5\n3<- 7 8\n h\n t\n```\n\n(5). enqueue(1)\n```\nq[tail=3] = 1\ntail + 1 = 4\n```\n```\n0 1 2 3 4 5\n 7 8 1\n h\n t\n```\n\n(6). dequeue()\n```\nreturn q[head=1] = 7\nhead + 1 = 2\n```\n```\n 0 1 2 3 4 5\n7<---- 8 1\n h\n t\n```\n\n(7). dequeue()\n```\nreturn q[head=2] = 8\nhead + 1 = 3\n```\n```\n 0 1 2 3 4 5\n8<------ 1\n h\n t\n```" | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:47.743398", | |
"end_time": "2017-02-20T19:40:47.785434" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "class Queue_simple:\n '''\n simple buffer\n '''\n def __init__(self, max_size=100):\n self.bufferMax = max_size\n self.head = 0\n self.tail = 0\n self.index = -1\n self.array = [None] * max_size\n \n def enqueue(self, data):\n if self.isFull():\n print(\"queue is full\")\n return\n \n self.array[self.tail] = data\n self.tail += 1\n \n def isEmpty(self):\n return self.head == self.tail\n \n def isFull(self):\n return (self.tail + 1) > self.bufferMax\n \n def dequeue(self):\n if self.isEmpty():\n print(\"queue is empty\")\n return\n \n data = self.array[self.head]\n self.array[self.head] = None\n self.head += 1\n return data\n \n def print(self):\n print(self.array[self.head:self.tail])\n \n def get_array(self):\n return self.array[self.head:self.tail]\n", | |
"execution_count": 5, | |
"outputs": [] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:47.789435", | |
"end_time": "2017-02-20T19:40:47.839469" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "q = Queue_simple(max_size=3)\nq.dequeue()", | |
"execution_count": 6, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "queue is empty\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:47.844472", | |
"end_time": "2017-02-20T19:40:47.855483" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "q.print()", | |
"execution_count": 7, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "[]\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:47.860483", | |
"end_time": "2017-02-20T19:40:47.870993" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "q.enqueue(1)\nq.enqueue(2)\nq.enqueue(3)\nq.enqueue(4)\nq.print()", | |
"execution_count": 8, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "queue is full\n[1, 2, 3]\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:47.875997", | |
"end_time": "2017-02-20T19:40:47.886153" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "q.dequeue()", | |
"execution_count": 9, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": "1" | |
}, | |
"metadata": {}, | |
"execution_count": 9 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:47.891156", | |
"end_time": "2017-02-20T19:40:47.898161" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "q.print()", | |
"execution_count": 10, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "[2, 3]\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "##### リングバッファでの実装" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "配列によってキューを実装すると、\n上図に示すように、データの追加と取り出し操作を繰り返すことによって、`head` と `tail` に挟まれたキューのデータ本体が配列の末尾に向かって(図の右側に)移動していく。\n\nこのままでは、`tail` と `head` が配列の容量をすぐに超えてしまう。`tail` が配列の領域を超えた時点でオーバーフローとして追加を諦めてしまっては、まだ使える配列の領域を無駄にしてしまう。\nしかし、それを防ぐために `dequeue()` が実行されたときに \n`head` を常に `0` に保つようにデータ全体を配列の先頭に(左に)向かって移動していては、その度に $O(n)$ の計算が必要になってしまう。\n\nこの問題を解決するために、配列によるキューの実装では次のように、配列を**リングバッファ**とみなしてデータを管理できる。\n\n>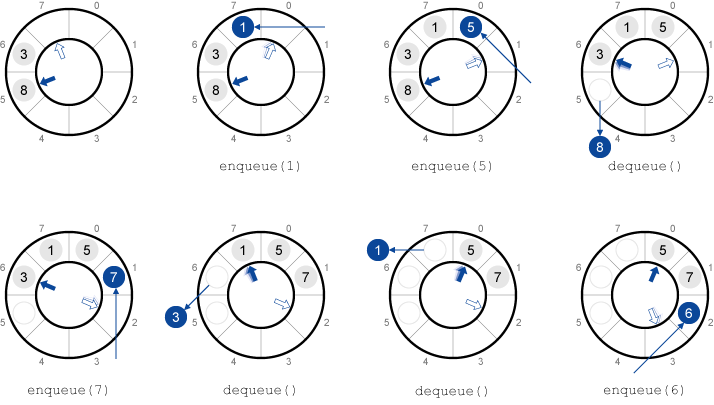\n> 引用:AOJ, キュー http://judge.u-aizu.ac.jp/onlinejudge/commentary.jsp?id=ALDS1_3_B\n\n上の図は既にいくつかのデータが格納されたキューにデータを出し入れしている様子を示している。\n最初に `enqueue(1)` によって 1 を追加したときに、tail の値が1 つ増えますが、既に `tail` が 7 であったため配列の領域を超えてしまうので `tail` を `0` に設定します。\nリングバッファを時計回りに見ると、キューにデータがある場合はポインタが `head` → `tail` の順番に並ぶ。\n\n- **キューが空のときと満杯のときを区別するために `tail` と `head` の間には常に1つ以上の空きを設ける**" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "- 格納されるデータ数が4の場合\n\nバッファサイズは `MAX`: $4+\\text{空き}1=5$ とする.\n\n(1):\n```\n初期状態\n0 1 2 3 4\nh\nt\n```\nif `head == tail` -> Empty\n\n\n(2):\n\n```\nenqueue(0)\nenqueue(1)\nenqueue(2)\nenqueue(3)\n```\n\nとすると,\n\n```\n0 1 2 3 4 \n0 1 2 3 N\nh\n t\n```\n\n`(tail + 1) % MAX = 5 % 5 = 0`\n\nif ((`head` = 0) == (`tail` + 1) % `MAX` = 0) -> Full\n\n(3):`dequeue()`すると,0番目\n\n```\ndequeue()\n```\n\n```\n 0 1 2 3 4 \n0 <- N 1 2 3 N\n h\n t\n```\n\nif ((`head` = 1) != (`tail` + 1) % `MAX` = 0) -> not Full\n\n\n(4):\n\n```\nenqueue(5)\n```\n\n```\n 0 1 2 3 4 \n0 <- N 1 2 3 5\n h\n t \n```\n\nheadとtailの間の0番が空く.\n" | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:48.440052", | |
"end_time": "2017-02-20T19:40:48.486086" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "class Queue(Queue_simple):\n '''\n using ling buffer\n '''\n def __init__(self, max_size=100):\n Queue_simple.__init__(self, max_size=max_size+1)\n \n def isFull(self):\n return (not self.isEmpty()) and (self.head == ((self.tail+1) % (self.bufferMax)))\n \n def dequeue(self):\n if self.isEmpty():\n print(\"queue is empty\")\n return\n \n data = self.array[self.head]\n self.array[self.head] = None\n \n # update head\n if self.head + 1 >= self.bufferMax:\n self.head = 0\n else:\n self.head += 1\n \n return data\n \n def enqueue(self, data):\n if self.isFull():\n print(\"queue is full\")\n return\n \n self.array[self.tail] = data\n \n # update tail\n if self.tail + 1 >= self.bufferMax:\n self.tail = 0\n else:\n self.tail += 1\n \n def print(self):\n print(self.get_array())\n print(\"head:\", self.head, \"tail:\", self.tail)\n \n def get_array(self):\n return self.array\n", | |
"execution_count": 11, | |
"outputs": [] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:48.491088", | |
"end_time": "2017-02-20T19:40:48.501095" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "q = Queue(max_size=4)\nq.dequeue()", | |
"execution_count": 12, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "queue is empty\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:48.506098", | |
"end_time": "2017-02-20T19:40:48.516106" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "q.enqueue(0)\nq.enqueue(1)\nq.enqueue(2)\nq.enqueue(3)\nq.print()", | |
"execution_count": 13, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "[0, 1, 2, 3, None]\nhead: 0 tail: 4\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:48.521109", | |
"end_time": "2017-02-20T19:40:48.532622" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "q.enqueue(5)", | |
"execution_count": 14, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "queue is full\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:48.537125", | |
"end_time": "2017-02-20T19:40:48.550136" | |
}, | |
"trusted": true, | |
"collapsed": false, | |
"scrolled": true | |
}, | |
"cell_type": "code", | |
"source": "q.dequeue()\nq.print()", | |
"execution_count": 15, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "[None, 1, 2, 3, None]\nhead: 1 tail: 4\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:48.555140", | |
"end_time": "2017-02-20T19:40:48.563146" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "q.enqueue(4)\nq.print()", | |
"execution_count": 16, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "[None, 1, 2, 3, 4]\nhead: 1 tail: 0\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:48.568148", | |
"end_time": "2017-02-20T19:40:48.582159" | |
}, | |
"trusted": true, | |
"collapsed": false | |
}, | |
"cell_type": "code", | |
"source": "q.enqueue(6)\nq.print()", | |
"execution_count": 17, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "queue is full\n[None, 1, 2, 3, 4]\nhead: 1 tail: 0\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"ExecuteTime": { | |
"start_time": "2017-02-20T19:40:48.587161", | |
"end_time": "2017-02-20T19:40:48.600170" | |
}, | |
"trusted": true, | |
"collapsed": false, | |
"scrolled": true | |
}, | |
"cell_type": "code", | |
"source": "q.dequeue()\nq.print()", | |
"execution_count": 18, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": "[None, None, 2, 3, 4]\nhead: 2 tail: 0\n", | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "##### 参考(キュー)" | |
}, | |
{ | |
"metadata": {}, | |
"cell_type": "markdown", | |
"source": "参考:\n\n- http://judge.u-aizu.ac.jp/onlinejudge/description.jsp?id=ALDS1_3_B&lang=jp" | |
} | |
], | |
"metadata": { | |
"kernelspec": { | |
"name": "python3", | |
"display_name": "Python 3", | |
"language": "python" | |
}, | |
"latex_envs": { | |
"eqNumInitial": 1, | |
"eqLabelWithNumbers": true, | |
"current_citInitial": 1, | |
"cite_by": "apalike", | |
"bibliofile": "biblio.bib", | |
"LaTeX_envs_menu_present": true, | |
"labels_anchors": false, | |
"latex_user_defs": false, | |
"user_envs_cfg": false, | |
"report_style_numbering": false | |
}, | |
"toc": { | |
"threshold": "6", | |
"number_sections": true, | |
"toc_cell": true, | |
"toc_window_display": true, | |
"toc_section_display": "block", | |
"sideBar": true, | |
"navigate_menu": false, | |
"moveMenuLeft": false, | |
"colors": { | |
"hover_highlight": "#DAA520", | |
"selected_highlight": "#FFD700", | |
"running_highlight": "#FF0000" | |
}, | |
"toc_position": { | |
"left": "0px", | |
"top": "160px", | |
"width": "221px", | |
"height": "475px", | |
"right": "569.531px" | |
} | |
}, | |
"language_info": { | |
"file_extension": ".py", | |
"mimetype": "text/x-python", | |
"name": "python", | |
"nbconvert_exporter": "python", | |
"version": "3.5.2", | |
"pygments_lexer": "ipython3", | |
"codemirror_mode": { | |
"name": "ipython", | |
"version": 3 | |
} | |
}, | |
"gist": { | |
"id": "1ab25e1c8d5b782201847331983f9a23", | |
"data": { | |
"description": "基本情報技術者/technology/大分類1_基礎理論/中分類2:アルゴリズムとプログラミング/スタックとキュー", | |
"public": true | |
} | |
}, | |
"_draft": { | |
"nbviewer_url": "https://gist.github.com/1ab25e1c8d5b782201847331983f9a23" | |
} | |
}, | |
"nbformat": 4, | |
"nbformat_minor": 0 | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment