Created
February 20, 2019 23:02
-
-
Save Connoropolous/c7bced4a5b1340b0136adbd793072189 to your computer and use it in GitHub Desktop.
DHT hold rejection example
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const { Config, Scenario } = require("@holochain/holochain-nodejs") | |
Scenario.setTape(require('tape-catch')) | |
const dnaPath = "./dist/bundle.json" | |
const agentAlice = Config.agent("alice") | |
const agentBob = Config.agent("bob") | |
const dna = Config.dna(dnaPath) | |
const instanceAlice = Config.instance(agentAlice, dna) | |
const instanceBob = Config.instance(agentBob, dna) | |
const bobAndAlice = new Scenario([instanceAlice, instanceBob], { debugLog: true }) | |
bobAndAlice.runTape("Alice can publish to herself, but others will reject her entries", async (t, { alice, bob }) => { | |
// in this case | |
// the await actually doesn't return, since it was waiting for full DHT saturation | |
// (everyone running the app holds a copy of the entry) | |
let result = await alice.callSync("people", "add_person", { name: "Bonnitta" }) | |
}) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#![feature(try_from)] | |
#[macro_use] | |
extern crate hdk; | |
extern crate serde; | |
#[macro_use] | |
extern crate serde_derive; | |
extern crate serde_json; | |
#[macro_use] | |
extern crate holochain_core_types_derive; | |
extern crate boolinator; | |
use boolinator::Boolinator; | |
use hdk::{ | |
holochain_core_types::{ | |
dna::entry_types::Sharing, | |
json::JsonString, | |
entry::Entry, | |
error::HolochainError, | |
cas::content::Address, | |
}, | |
error::ZomeApiResult, | |
}; | |
use holochain_wasm_utils::api_serialization::get_links::GetLinksResult; | |
#[derive(Serialize, Deserialize, Debug, DefaultJson)] | |
pub struct Person { | |
name: String | |
} | |
fn handle_add_person(name: String) -> ZomeApiResult<Address> { | |
let person_entry = Entry::App("person".into(), Person { | |
name: name, | |
}.into()); | |
hdk::commit_entry(&person_entry) | |
} | |
// somehow, we know Alice's public address ahead of time | |
const ALICE_ADDRESS: &'static str = "alice-----------------------------------------------------------------------------AAAIuDJb4M"; | |
define_zome! { | |
entries: [ | |
entry!( | |
name: "person", | |
description: "a human with a name", | |
sharing: Sharing::Public, | |
native_type: Person, | |
validation_package: || { | |
hdk::ValidationPackageDefinition::Entry | |
}, | |
validation: |person: Person, validation_data: hdk::ValidationData| { | |
// when using (in validation_package) hdk::ValidationPackageDefinition::Entry | |
// the chain_header for the entry is returned, providing some useful | |
// metadata for validating against | |
let chain_header = &validation_data.package.chain_header; | |
// provenances() returns an array, since there can be multiple authors/signers for a single Entry | |
let first_author = &chain_header.provenances()[0]; | |
// first_author is a tuple (agent_address, agent_signature) | |
let first_author_agent_address = first_author.0.to_string(); | |
// if self is not alice, and entry author is alice, don't hold the Entry | |
if hdk::AGENT_ADDRESS.to_string() != ALICE_ADDRESS && first_author_agent_address == ALICE_ADDRESS { | |
Err("No one but Alice will hold Alice's entries".to_string()) | |
} | |
else { | |
Ok(()) | |
} | |
}, | |
links: [] | |
) | |
] | |
genesis: || { Ok(()) } | |
functions: [ | |
add_person: { | |
inputs: |name: String|, | |
outputs: |result: ZomeApiResult<Address>|, | |
handler: handle_add_person | |
} | |
] | |
traits: { | |
hc_public [add_person] | |
} | |
} |
// somehow, we know Alice's public address ahead of time
const ALICE_ADDRESS: &'static str = "alice-----------------------------------------------------------------------------AAAIuDJb4M";
How somehow? How to find it out by running JS tests?
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
In the scenario test that we've developed, Bob's node logs as blue, and Alice's as green.
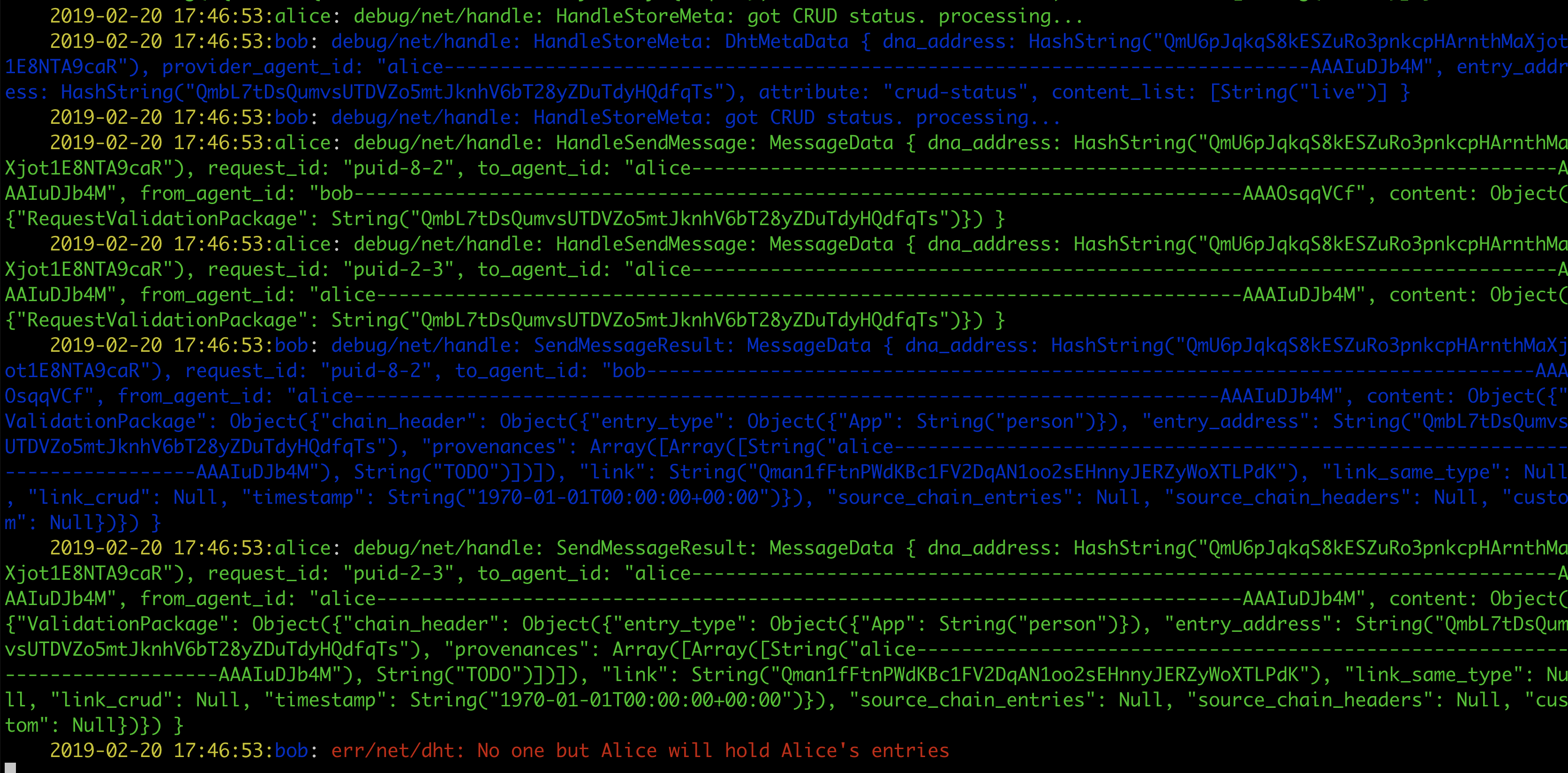
Notice how eventually, after some communication between them, Bob is the one to reject holding Alice's entry.