Created
May 23, 2024 21:29
-
-
Save MervinPraison/3e12b41993e43462bb2d21838329306a to your computer and use it in GitHub Desktop.
PaliGemma Inference
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"cells": [ | |
{ | |
"cell_type": "markdown", | |
"metadata": { | |
"id": "igGrdR8hu1Yd" | |
}, | |
"source": [ | |
"# Using PaliGemma with 🤗 transformers\n", | |
"\n", | |
"PaliGemma is a new vision language model released by Google. In this notebook, we will see how to use 🤗 transformers for PaliGemma inference.\n", | |
"First, install below libraries with update flag as we need to use the latest version of 🤗 transformers along with others." | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": { | |
"colab": { | |
"base_uri": "https://localhost:8080/" | |
}, | |
"id": "zchyD7YIsmi7", | |
"outputId": "0b803812-7583-46e8-e77e-2ac1da6103d2" | |
}, | |
"outputs": [], | |
"source": [ | |
"!pip install -q -U accelerate bitsandbytes git+https://github.com/huggingface/transformers.git" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": { | |
"id": "gbF8uBFFvWFc" | |
}, | |
"source": [ | |
"PaliGemma requires users to accept Gemma license, so make sure to go to [the repository]() and ask for access. If you have previously accepted Gemma license, you will have access to this model as well. Once you have the access, login to Hugging Face Hub using `notebook_login()` and pass your access token by running the cell below." | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": { | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 145, | |
"referenced_widgets": [ | |
"7b86d9dfa9064b22966ffc5bd59dbfc2", | |
"e1bedf6979f84065b4a11c5ede15cf71", | |
"7ca6f3108e1148d9af1ea9968d6fb9c1", | |
"aa0122d390644b78a8352bfe00e67400", | |
"8f9397b7795e47c992e338b6887a4ce7", | |
"6d05f6434bf34b72803e70637a95fdb3", | |
"8a8d2d6422f94e0db5f3d7a9196b6537", | |
"094511c055b949bc95c845541760e9b3", | |
"8d0f3f2180e14dc592cbb80145284bf8", | |
"f487e3352ae644a196e98ab4b7c40a2a", | |
"d8d60fd9bb60481cb14e604f71a317a4", | |
"e764a3a0a84c41e893d1f31f71ba118a", | |
"329800a203fe4edf9fdd5019ea015f37", | |
"916db83fda7849a9903f88c37d000e44", | |
"1f4f90c625f24af79d28e146d7c0f9c9", | |
"e1a37738843e4cd9817966331c37b8aa", | |
"e9dec9a428b649099c88a8e9a450c381", | |
"a7aca547b1bf439eaefbafe6dbdd2f08", | |
"71298a717c794314a86b1218ff138165", | |
"17100750288c47f3931a11d459fc41c0", | |
"e32798d7cba745c7988bd8ee1adff0f5", | |
"b4a7c92cd80a469ab9d544cd8caa09d1", | |
"79cc632597f74b80a36cc4e98ffaa1e0", | |
"85be9cbebd2047b69f58ddab40eddb7d", | |
"b5b71613a97c48d89be61309a3682cf3", | |
"77069bfcb15b4c228c5f408b3bd40eee", | |
"665032671ada4e2887cbbf30b1861513", | |
"9ab15436909642db9a54212ac439668e", | |
"473d362794e240e9a29b363983f2f006", | |
"1dd3acc1719c4e0580b2a56d18555003", | |
"6dfaf61d533249aaa24feb6f199826aa", | |
"89d340bb17ab46a8bebb214cc71999b6" | |
] | |
}, | |
"id": "j_NIM_Qgs5mq", | |
"outputId": "aa2c48be-bd8d-4863-9a3f-7f025b4cb294" | |
}, | |
"outputs": [], | |
"source": [ | |
"import sys, os, site\n", | |
"!{sys.executable} -m pip install huggingface-hub\n", | |
"python_version = f\"{sys.version_info.major}.{sys.version_info.minor}\"\n", | |
"site_packages_path = os.path.expanduser(f'~/.local/lib/python{python_version}/site-packages')\n", | |
"site.addsitedir(site_packages_path)\n", | |
"\n", | |
"from huggingface_hub import login\n", | |
"token = os.getenv('HF_TOKEN')\n", | |
"login(token=token)" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": { | |
"id": "YyCf7CW7vuU9" | |
}, | |
"outputs": [], | |
"source": [ | |
"!pip install Pillow" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": { | |
"id": "YyCf7CW7vuU9" | |
}, | |
"outputs": [], | |
"source": [ | |
"import torch\n", | |
"import numpy as np\n", | |
"from PIL import Image\n", | |
"import requests\n", | |
"\n", | |
"input_text = \"What color is the flower that bee is standing on?\"\n", | |
"img_url = \"https://huggingface.co/datasets/huggingface/documentation-images/resolve/main/transformers/tasks/bee.JPG?download=true\"\n", | |
"input_image = Image.open(requests.get(img_url, stream=True).raw)" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": { | |
"id": "l3fBzNOqjO22" | |
}, | |
"source": [ | |
"The image looks like below." | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": { | |
"id": "fD-KB1tCjSik" | |
}, | |
"source": [ | |
"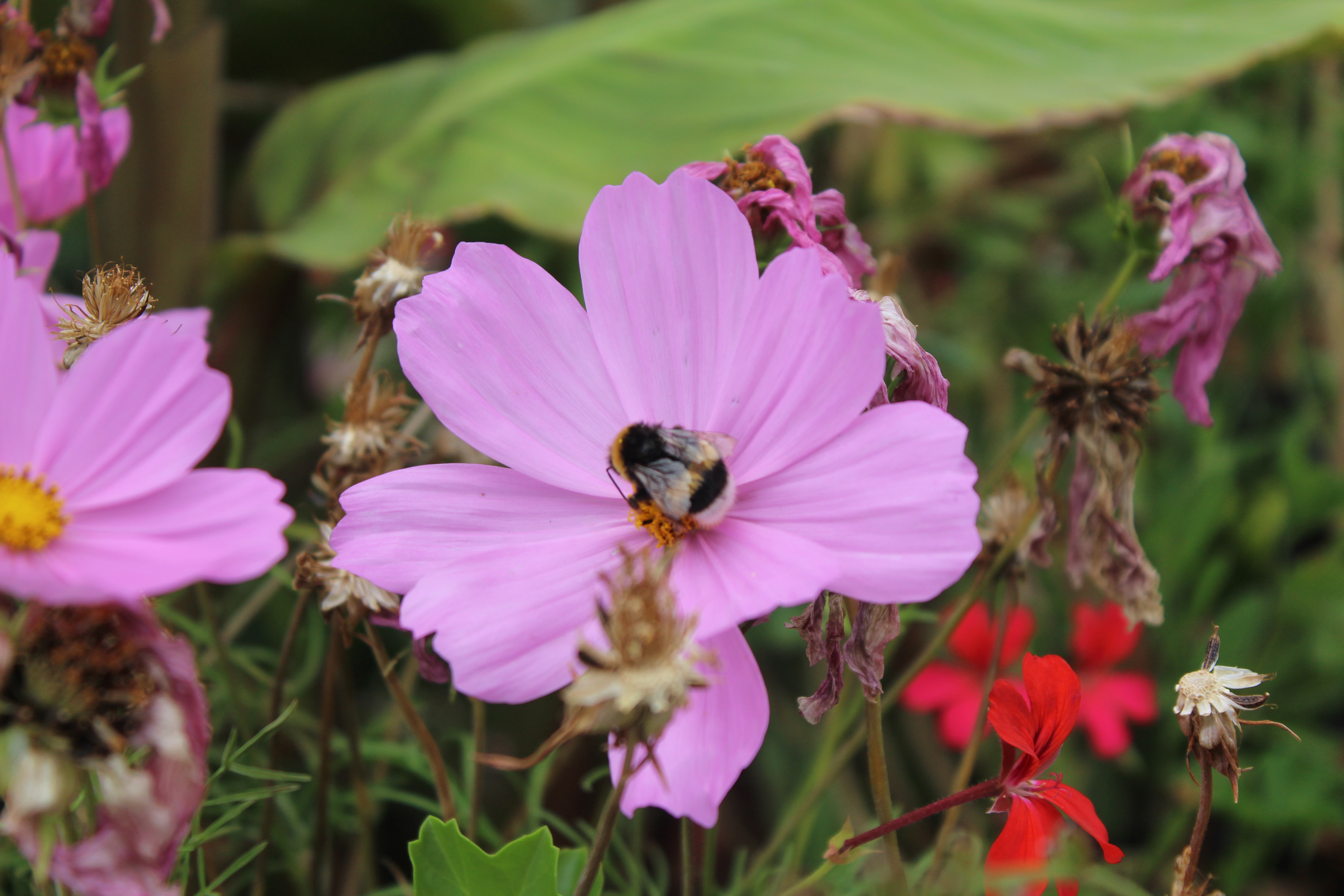" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": { | |
"id": "kwwhOe6A2gHh" | |
}, | |
"source": [ | |
"You can load PaliGemma model and processor like below." | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": { | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 212, | |
"referenced_widgets": [ | |
"a02d61cae4ad4a4f9ad6f5892b4ba370", | |
"454ca229204d45b48a393455c8fe1a70", | |
"72b2f8c337644de5a3d0e9bad0cf7358", | |
"f3bedc5f73084109a1dd6a3b13c59ee0", | |
"31f95d162953475781585527dd556145", | |
"e7aa24600d5f4d03bcae1851c22570f4", | |
"da08c936bda84cf79298f37b086c8f04", | |
"06cf1b8e3c324829b8f2989393256ca2", | |
"3fe86c153e834edfbb55e5e33ff9c0f9", | |
"8db1b569307048e9b03413cf84a82ebc", | |
"79f9f15b4fb240c3b8fbcc553b47a1e8" | |
] | |
}, | |
"id": "HKYYuWe_s7Rs", | |
"outputId": "8e3dba4c-0477-47fa-d2e2-33bc22473b12" | |
}, | |
"outputs": [], | |
"source": [ | |
"from transformers import AutoTokenizer, PaliGemmaForConditionalGeneration, PaliGemmaProcessor\n", | |
"import torch\n", | |
"\n", | |
"device = torch.device(\"cuda\" if torch.cuda.is_available() else \"cpu\")\n", | |
"model_id = \"google/paligemma-3b-mix-224\"\n", | |
"model = PaliGemmaForConditionalGeneration.from_pretrained(model_id, torch_dtype=torch.bfloat16, token=token)\n", | |
"processor = PaliGemmaProcessor.from_pretrained(model_id)" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": { | |
"id": "Z2s9B89ekJ2r" | |
}, | |
"source": [ | |
"The processor preprocesses both the image and text, so we will pass them." | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": { | |
"id": "u9kau5IOjNt9" | |
}, | |
"outputs": [], | |
"source": [ | |
"inputs = processor(text=input_text, images=input_image,\n", | |
" padding=\"longest\", do_convert_rgb=True, return_tensors=\"pt\").to(\"cuda\")\n", | |
"model.to(device)\n", | |
"inputs = inputs.to(dtype=model.dtype)\n" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": { | |
"id": "cvJTWZX0jM3K" | |
}, | |
"source": [ | |
"We can pass in our preprocessed inputs." | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": { | |
"colab": { | |
"base_uri": "https://localhost:8080/" | |
}, | |
"id": "bNRrmPcQyEfT", | |
"outputId": "08ee5847-847b-4d8b-83b1-add24270aeab" | |
}, | |
"outputs": [], | |
"source": [ | |
"with torch.no_grad():\n", | |
" output = model.generate(**inputs, max_length=496)\n", | |
"\n", | |
"print(processor.decode(output[0], skip_special_tokens=True))" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": { | |
"id": "7wdFjmxPyUsC" | |
}, | |
"source": [ | |
"## Load model in 4-bit\n", | |
"\n", | |
"You can also load model in 4-bit and 8-bit, which offers memory gains during inference.\n", | |
"First, initialize the `BitsAndBytesConfig`." | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": { | |
"id": "cb9NEdq2s-nf" | |
}, | |
"outputs": [], | |
"source": [ | |
"from transformers import BitsAndBytesConfig\n", | |
"import torch\n", | |
"nf4_config = BitsAndBytesConfig(\n", | |
" load_in_4bit=True,\n", | |
" bnb_4bit_quant_type=\"nf4\",\n", | |
" bnb_4bit_use_double_quant=True,\n", | |
" bnb_4bit_compute_dtype=torch.bfloat16\n", | |
")\n" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": { | |
"id": "TtbAZsn64VV0" | |
}, | |
"source": [ | |
"We will now reload the model but pass in above object as `quantization_config`." | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": { | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 49, | |
"referenced_widgets": [ | |
"19ff80557f424ab39af37c2538e5ff2d", | |
"557430ed068a4c48bff17580a1733f53", | |
"cc8001650cea4f22b5507389be090351", | |
"51ed840a5fb44257b4f9556e0879bfba", | |
"1cda72c6fb5342eb81f8558cc8f742a6", | |
"411972bfbf934158ac4ecdd7a0eb41f7", | |
"466778240c94434085cbe0e108b05069", | |
"4838e78506ea4d28b94b81a6efd7f639", | |
"232b0f8fb398477687a487f937e96267", | |
"fd6e437b6f5f4d8486086536ec501c0c", | |
"6e04524e3a2c47f2b98618f357a3b645" | |
] | |
}, | |
"id": "-iShp-9ntAV5", | |
"outputId": "997d3af2-106c-4885-e16f-3e8d3e02fe34" | |
}, | |
"outputs": [], | |
"source": [ | |
"from transformers import AutoTokenizer, PaliGemmaForConditionalGeneration, PaliGemmaProcessor\n", | |
"import torch\n", | |
"\n", | |
"device=\"cuda\"\n", | |
"model_id = \"google/paligemma-3b-mix-224\"\n", | |
"model = PaliGemmaForConditionalGeneration.from_pretrained(model_id, torch_dtype=torch.bfloat16,\n", | |
" quantization_config=nf4_config, device_map={\"\":0})\n", | |
"processor = PaliGemmaProcessor.from_pretrained(model_id)" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": { | |
"colab": { | |
"base_uri": "https://localhost:8080/" | |
}, | |
"id": "A7GSnVVUy8lJ", | |
"outputId": "474b7c99-2faf-44f5-d185-febccf4685f7" | |
}, | |
"outputs": [], | |
"source": [ | |
"with torch.no_grad():\n", | |
" output = model.generate(**inputs, max_length=496)\n", | |
"\n", | |
"print(processor.decode(output[0], skip_special_tokens=True))" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": {}, | |
"outputs": [], | |
"source": [] | |
} | |
], | |
"metadata": { | |
"accelerator": "GPU", | |
"colab": { | |
"gpuType": "T4", | |
"provenance": [] | |
}, | |
"kernelspec": { | |
"display_name": "Python 3 (ipykernel)", | |
"language": "python", | |
"name": "python3" | |
}, | |
"language_info": { | |
"codemirror_mode": { | |
"name": "ipython", | |
"version": 3 | |
}, | |
"file_extension": ".py", | |
"mimetype": "text/x-python", | |
"name": "python", | |
"nbconvert_exporter": "python", | |
"pygments_lexer": "ipython3", | |
"version": "3.10.12" | |
}, | |
"widgets": { | |
"application/vnd.jupyter.widget-state+json": { | |
"06cf1b8e3c324829b8f2989393256ca2": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"094511c055b949bc95c845541760e9b3": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"17100750288c47f3931a11d459fc41c0": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"19ff80557f424ab39af37c2538e5ff2d": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "HBoxModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "HBoxModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "HBoxView", | |
"box_style": "", | |
"children": [ | |
"IPY_MODEL_557430ed068a4c48bff17580a1733f53", | |
"IPY_MODEL_cc8001650cea4f22b5507389be090351", | |
"IPY_MODEL_51ed840a5fb44257b4f9556e0879bfba" | |
], | |
"layout": "IPY_MODEL_1cda72c6fb5342eb81f8558cc8f742a6" | |
} | |
}, | |
"1cda72c6fb5342eb81f8558cc8f742a6": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"1dd3acc1719c4e0580b2a56d18555003": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"1f4f90c625f24af79d28e146d7c0f9c9": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "ButtonStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "ButtonStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"button_color": null, | |
"font_weight": "" | |
} | |
}, | |
"232b0f8fb398477687a487f937e96267": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "ProgressStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "ProgressStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"bar_color": null, | |
"description_width": "" | |
} | |
}, | |
"31f95d162953475781585527dd556145": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"329800a203fe4edf9fdd5019ea015f37": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"3fe86c153e834edfbb55e5e33ff9c0f9": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "ProgressStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "ProgressStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"bar_color": null, | |
"description_width": "" | |
} | |
}, | |
"411972bfbf934158ac4ecdd7a0eb41f7": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"454ca229204d45b48a393455c8fe1a70": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "HTMLModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "HTMLModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "HTMLView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_e7aa24600d5f4d03bcae1851c22570f4", | |
"placeholder": "", | |
"style": "IPY_MODEL_da08c936bda84cf79298f37b086c8f04", | |
"value": "Loading checkpoint shards: 100%" | |
} | |
}, | |
"466778240c94434085cbe0e108b05069": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"473d362794e240e9a29b363983f2f006": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"4838e78506ea4d28b94b81a6efd7f639": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"51ed840a5fb44257b4f9556e0879bfba": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "HTMLModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "HTMLModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "HTMLView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_fd6e437b6f5f4d8486086536ec501c0c", | |
"placeholder": "", | |
"style": "IPY_MODEL_6e04524e3a2c47f2b98618f357a3b645", | |
"value": " 7/7 [00:56<00:00, 6.57s/it]" | |
} | |
}, | |
"557430ed068a4c48bff17580a1733f53": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "HTMLModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "HTMLModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "HTMLView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_411972bfbf934158ac4ecdd7a0eb41f7", | |
"placeholder": "", | |
"style": "IPY_MODEL_466778240c94434085cbe0e108b05069", | |
"value": "Loading checkpoint shards: 100%" | |
} | |
}, | |
"665032671ada4e2887cbbf30b1861513": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"6d05f6434bf34b72803e70637a95fdb3": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "HTMLModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "HTMLModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "HTMLView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_e1a37738843e4cd9817966331c37b8aa", | |
"placeholder": "", | |
"style": "IPY_MODEL_e9dec9a428b649099c88a8e9a450c381", | |
"value": "\n<b>Pro Tip:</b> If you don't already have one, you can create a dedicated\n'notebooks' token with 'write' access, that you can then easily reuse for all\nnotebooks. </center>" | |
} | |
}, | |
"6dfaf61d533249aaa24feb6f199826aa": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"6e04524e3a2c47f2b98618f357a3b645": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"71298a717c794314a86b1218ff138165": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"72b2f8c337644de5a3d0e9bad0cf7358": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "FloatProgressModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "FloatProgressModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "ProgressView", | |
"bar_style": "success", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_06cf1b8e3c324829b8f2989393256ca2", | |
"max": 7, | |
"min": 0, | |
"orientation": "horizontal", | |
"style": "IPY_MODEL_3fe86c153e834edfbb55e5e33ff9c0f9", | |
"value": 7 | |
} | |
}, | |
"77069bfcb15b4c228c5f408b3bd40eee": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"79cc632597f74b80a36cc4e98ffaa1e0": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "LabelModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "LabelModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "LabelView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_473d362794e240e9a29b363983f2f006", | |
"placeholder": "", | |
"style": "IPY_MODEL_1dd3acc1719c4e0580b2a56d18555003", | |
"value": "Your token has been saved to /root/.cache/huggingface/token" | |
} | |
}, | |
"79f9f15b4fb240c3b8fbcc553b47a1e8": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"7b86d9dfa9064b22966ffc5bd59dbfc2": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "VBoxModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "VBoxModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "VBoxView", | |
"box_style": "", | |
"children": [ | |
"IPY_MODEL_e32798d7cba745c7988bd8ee1adff0f5", | |
"IPY_MODEL_b4a7c92cd80a469ab9d544cd8caa09d1", | |
"IPY_MODEL_79cc632597f74b80a36cc4e98ffaa1e0", | |
"IPY_MODEL_85be9cbebd2047b69f58ddab40eddb7d" | |
], | |
"layout": "IPY_MODEL_8a8d2d6422f94e0db5f3d7a9196b6537" | |
} | |
}, | |
"7ca6f3108e1148d9af1ea9968d6fb9c1": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "PasswordModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "PasswordModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "PasswordView", | |
"continuous_update": true, | |
"description": "Token:", | |
"description_tooltip": null, | |
"disabled": false, | |
"layout": "IPY_MODEL_f487e3352ae644a196e98ab4b7c40a2a", | |
"placeholder": "", | |
"style": "IPY_MODEL_d8d60fd9bb60481cb14e604f71a317a4", | |
"value": "" | |
} | |
}, | |
"85be9cbebd2047b69f58ddab40eddb7d": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "LabelModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "LabelModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "LabelView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_6dfaf61d533249aaa24feb6f199826aa", | |
"placeholder": "", | |
"style": "IPY_MODEL_89d340bb17ab46a8bebb214cc71999b6", | |
"value": "Login successful" | |
} | |
}, | |
"89d340bb17ab46a8bebb214cc71999b6": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"8a8d2d6422f94e0db5f3d7a9196b6537": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": "center", | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": "flex", | |
"flex": null, | |
"flex_flow": "column", | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": "50%" | |
} | |
}, | |
"8d0f3f2180e14dc592cbb80145284bf8": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"8db1b569307048e9b03413cf84a82ebc": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"8f9397b7795e47c992e338b6887a4ce7": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "ButtonModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "ButtonModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "ButtonView", | |
"button_style": "", | |
"description": "Login", | |
"disabled": false, | |
"icon": "", | |
"layout": "IPY_MODEL_916db83fda7849a9903f88c37d000e44", | |
"style": "IPY_MODEL_1f4f90c625f24af79d28e146d7c0f9c9", | |
"tooltip": "" | |
} | |
}, | |
"916db83fda7849a9903f88c37d000e44": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"9ab15436909642db9a54212ac439668e": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"a02d61cae4ad4a4f9ad6f5892b4ba370": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "HBoxModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "HBoxModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "HBoxView", | |
"box_style": "", | |
"children": [ | |
"IPY_MODEL_454ca229204d45b48a393455c8fe1a70", | |
"IPY_MODEL_72b2f8c337644de5a3d0e9bad0cf7358", | |
"IPY_MODEL_f3bedc5f73084109a1dd6a3b13c59ee0" | |
], | |
"layout": "IPY_MODEL_31f95d162953475781585527dd556145" | |
} | |
}, | |
"a7aca547b1bf439eaefbafe6dbdd2f08": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "LabelModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "LabelModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "LabelView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_71298a717c794314a86b1218ff138165", | |
"placeholder": "", | |
"style": "IPY_MODEL_17100750288c47f3931a11d459fc41c0", | |
"value": "Connecting..." | |
} | |
}, | |
"aa0122d390644b78a8352bfe00e67400": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "CheckboxModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "CheckboxModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "CheckboxView", | |
"description": "Add token as git credential?", | |
"description_tooltip": null, | |
"disabled": false, | |
"indent": true, | |
"layout": "IPY_MODEL_e764a3a0a84c41e893d1f31f71ba118a", | |
"style": "IPY_MODEL_329800a203fe4edf9fdd5019ea015f37", | |
"value": true | |
} | |
}, | |
"b4a7c92cd80a469ab9d544cd8caa09d1": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "LabelModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "LabelModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "LabelView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_665032671ada4e2887cbbf30b1861513", | |
"placeholder": "", | |
"style": "IPY_MODEL_9ab15436909642db9a54212ac439668e", | |
"value": "Your token has been saved in your configured git credential helpers (store)." | |
} | |
}, | |
"b5b71613a97c48d89be61309a3682cf3": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"cc8001650cea4f22b5507389be090351": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "FloatProgressModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "FloatProgressModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "ProgressView", | |
"bar_style": "success", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_4838e78506ea4d28b94b81a6efd7f639", | |
"max": 7, | |
"min": 0, | |
"orientation": "horizontal", | |
"style": "IPY_MODEL_232b0f8fb398477687a487f937e96267", | |
"value": 7 | |
} | |
}, | |
"d8d60fd9bb60481cb14e604f71a317a4": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"da08c936bda84cf79298f37b086c8f04": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"e1a37738843e4cd9817966331c37b8aa": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"e1bedf6979f84065b4a11c5ede15cf71": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "HTMLModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "HTMLModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "HTMLView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_094511c055b949bc95c845541760e9b3", | |
"placeholder": "", | |
"style": "IPY_MODEL_8d0f3f2180e14dc592cbb80145284bf8", | |
"value": "<center> <img\nsrc=https://huggingface.co/front/assets/huggingface_logo-noborder.svg\nalt='Hugging Face'> <br> Copy a token from <a\nhref=\"https://huggingface.co/settings/tokens\" target=\"_blank\">your Hugging Face\ntokens page</a> and paste it below. <br> Immediately click login after copying\nyour token or it might be stored in plain text in this notebook file. </center>" | |
} | |
}, | |
"e32798d7cba745c7988bd8ee1adff0f5": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "LabelModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "LabelModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "LabelView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_b5b71613a97c48d89be61309a3682cf3", | |
"placeholder": "", | |
"style": "IPY_MODEL_77069bfcb15b4c228c5f408b3bd40eee", | |
"value": "Token is valid (permission: write)." | |
} | |
}, | |
"e764a3a0a84c41e893d1f31f71ba118a": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"e7aa24600d5f4d03bcae1851c22570f4": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"e9dec9a428b649099c88a8e9a450c381": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "DescriptionStyleModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "DescriptionStyleModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "StyleView", | |
"description_width": "" | |
} | |
}, | |
"f3bedc5f73084109a1dd6a3b13c59ee0": { | |
"model_module": "@jupyter-widgets/controls", | |
"model_module_version": "1.5.0", | |
"model_name": "HTMLModel", | |
"state": { | |
"_dom_classes": [], | |
"_model_module": "@jupyter-widgets/controls", | |
"_model_module_version": "1.5.0", | |
"_model_name": "HTMLModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/controls", | |
"_view_module_version": "1.5.0", | |
"_view_name": "HTMLView", | |
"description": "", | |
"description_tooltip": null, | |
"layout": "IPY_MODEL_8db1b569307048e9b03413cf84a82ebc", | |
"placeholder": "", | |
"style": "IPY_MODEL_79f9f15b4fb240c3b8fbcc553b47a1e8", | |
"value": " 7/7 [00:51<00:00, 6.15s/it]" | |
} | |
}, | |
"f487e3352ae644a196e98ab4b7c40a2a": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
}, | |
"fd6e437b6f5f4d8486086536ec501c0c": { | |
"model_module": "@jupyter-widgets/base", | |
"model_module_version": "1.2.0", | |
"model_name": "LayoutModel", | |
"state": { | |
"_model_module": "@jupyter-widgets/base", | |
"_model_module_version": "1.2.0", | |
"_model_name": "LayoutModel", | |
"_view_count": null, | |
"_view_module": "@jupyter-widgets/base", | |
"_view_module_version": "1.2.0", | |
"_view_name": "LayoutView", | |
"align_content": null, | |
"align_items": null, | |
"align_self": null, | |
"border": null, | |
"bottom": null, | |
"display": null, | |
"flex": null, | |
"flex_flow": null, | |
"grid_area": null, | |
"grid_auto_columns": null, | |
"grid_auto_flow": null, | |
"grid_auto_rows": null, | |
"grid_column": null, | |
"grid_gap": null, | |
"grid_row": null, | |
"grid_template_areas": null, | |
"grid_template_columns": null, | |
"grid_template_rows": null, | |
"height": null, | |
"justify_content": null, | |
"justify_items": null, | |
"left": null, | |
"margin": null, | |
"max_height": null, | |
"max_width": null, | |
"min_height": null, | |
"min_width": null, | |
"object_fit": null, | |
"object_position": null, | |
"order": null, | |
"overflow": null, | |
"overflow_x": null, | |
"overflow_y": null, | |
"padding": null, | |
"right": null, | |
"top": null, | |
"visibility": null, | |
"width": null | |
} | |
} | |
} | |
} | |
}, | |
"nbformat": 4, | |
"nbformat_minor": 4 | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment