-
-
Save PandaWhoCodes/a4b5b3bd4534989faf39a1d38c028d71 to your computer and use it in GitHub Desktop.
pandas.ipynb
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"nbformat": 4, | |
"nbformat_minor": 0, | |
"metadata": { | |
"colab": { | |
"name": "pandas.ipynb", | |
"version": "0.3.2", | |
"provenance": [], | |
"include_colab_link": true | |
}, | |
"kernelspec": { | |
"name": "python3", | |
"display_name": "Python 3" | |
} | |
}, | |
"cells": [ | |
{ | |
"cell_type": "markdown", | |
"metadata": { | |
"id": "view-in-github", | |
"colab_type": "text" | |
}, | |
"source": [ | |
"<a href=\"https://colab.research.google.com/gist/PandaWhoCodes/a4b5b3bd4534989faf39a1d38c028d71/pandas.ipynb\" target=\"_parent\"><img src=\"https://colab.research.google.com/assets/colab-badge.svg\" alt=\"Open In Colab\"/></a>" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "BJo7tF2DT6CU", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# **What is pandas ?**\n", | |
"\n", | |
"\n", | |
"Pandas is an open-source Python Library providing high-performance data manipulation and analysis tool using its powerful data structures. The name Pandas is derived from the word Panel Data – an Econometrics from Multidimensional data.\n" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "JMxTe9b9UvUV", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# imports\n", | |
"Let's start with the **imports** \n", | |
"Customarily, we import as follows:\n", | |
"\n", | |
"\n" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "xCE2R8ThU_9z", | |
"colab_type": "code", | |
"colab": {} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"import pandas as pd" | |
], | |
"execution_count": 0, | |
"outputs": [] | |
}, | |
{ | |
"metadata": { | |
"id": "uw7FDc6sVQOw", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Dataframe\n", | |
"A Data frame is a two-dimensional data structure, i.e., data is aligned in a tabular fashion in rows and columns.\n", | |
"\n", | |
"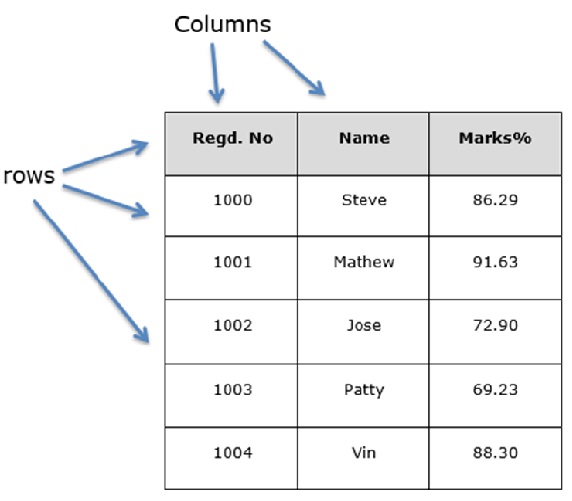\n", | |
"\n", | |
"# Dataset\n", | |
"For the purpose of this tutorial we will be using the pokemon dataset. You can download it for your own purposes [here](https://drive.google.com/open?id=1bse4wXOuXSCCXO_99DS0n7ol-1vXy6DF)\n", | |
"\n", | |
"\n", | |
"\n", | |
"# Google Drive\n", | |
"Since this notebook is hosted on google colab, I am using my google drive to load my dataset.\n", | |
"\n", | |
"# Loading the dataset to a dataframe\n", | |
"to read the csv instead of\n", | |
"\n", | |
"```\n", | |
"import csv\n", | |
"with open('employee_birthday.txt') as csv_file:\n", | |
" csv_reader = csv.reader(csv_file, delimiter=',')\n", | |
"```\n", | |
"We will just use the pandas **read_csv** function. This will directly load the csv into a dataframe.\n", | |
"Other such functions include **read_json**,**read_sql**,**_read_html**, [etc](https://pandas.pydata.org/pandas-docs/stable/search.html?q=read_&check_keywords=yes&area=default).\n" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "LGxKmB8ATb5Y", | |
"colab_type": "code", | |
"outputId": "6a21020e-d87c-4e29-a191-97af71f7ebad", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 34 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"from google.colab import drive\n", | |
"drive.mount('/content/drive')\n", | |
"# reading the dataset\n", | |
"df = pd.read_csv('/content/drive/My Drive/datasets/Pokemon.csv')\n" | |
], | |
"execution_count": 77, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": [ | |
"Drive already mounted at /content/drive; to attempt to forcibly remount, call drive.mount(\"/content/drive\", force_remount=True).\n" | |
], | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "VZEZYoS1co2j", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"## Learning about the dataset" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "w-_4KHjWTtqX", | |
"colab_type": "code", | |
"outputId": "7c3795a0-18d4-46bb-d992-c7b986cde894", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 284 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"# Generates descriptive statistics that summarize the central tendency, dispersion and shape of a dataset’s distribution, excluding NaN values.\n", | |
"df.describe()" | |
], | |
"execution_count": 78, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>#</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>count</th>\n", | |
" <td>800.000000</td>\n", | |
" <td>800.00000</td>\n", | |
" <td>800.000000</td>\n", | |
" <td>800.000000</td>\n", | |
" <td>800.000000</td>\n", | |
" <td>800.000000</td>\n", | |
" <td>800.000000</td>\n", | |
" <td>800.000000</td>\n", | |
" <td>800.00000</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>mean</th>\n", | |
" <td>362.813750</td>\n", | |
" <td>435.10250</td>\n", | |
" <td>69.258750</td>\n", | |
" <td>79.001250</td>\n", | |
" <td>73.842500</td>\n", | |
" <td>72.820000</td>\n", | |
" <td>71.902500</td>\n", | |
" <td>68.277500</td>\n", | |
" <td>3.32375</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>std</th>\n", | |
" <td>208.343798</td>\n", | |
" <td>119.96304</td>\n", | |
" <td>25.534669</td>\n", | |
" <td>32.457366</td>\n", | |
" <td>31.183501</td>\n", | |
" <td>32.722294</td>\n", | |
" <td>27.828916</td>\n", | |
" <td>29.060474</td>\n", | |
" <td>1.66129</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>min</th>\n", | |
" <td>1.000000</td>\n", | |
" <td>180.00000</td>\n", | |
" <td>1.000000</td>\n", | |
" <td>5.000000</td>\n", | |
" <td>5.000000</td>\n", | |
" <td>10.000000</td>\n", | |
" <td>20.000000</td>\n", | |
" <td>5.000000</td>\n", | |
" <td>1.00000</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>25%</th>\n", | |
" <td>184.750000</td>\n", | |
" <td>330.00000</td>\n", | |
" <td>50.000000</td>\n", | |
" <td>55.000000</td>\n", | |
" <td>50.000000</td>\n", | |
" <td>49.750000</td>\n", | |
" <td>50.000000</td>\n", | |
" <td>45.000000</td>\n", | |
" <td>2.00000</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>50%</th>\n", | |
" <td>364.500000</td>\n", | |
" <td>450.00000</td>\n", | |
" <td>65.000000</td>\n", | |
" <td>75.000000</td>\n", | |
" <td>70.000000</td>\n", | |
" <td>65.000000</td>\n", | |
" <td>70.000000</td>\n", | |
" <td>65.000000</td>\n", | |
" <td>3.00000</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>75%</th>\n", | |
" <td>539.250000</td>\n", | |
" <td>515.00000</td>\n", | |
" <td>80.000000</td>\n", | |
" <td>100.000000</td>\n", | |
" <td>90.000000</td>\n", | |
" <td>95.000000</td>\n", | |
" <td>90.000000</td>\n", | |
" <td>90.000000</td>\n", | |
" <td>5.00000</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>max</th>\n", | |
" <td>721.000000</td>\n", | |
" <td>780.00000</td>\n", | |
" <td>255.000000</td>\n", | |
" <td>190.000000</td>\n", | |
" <td>230.000000</td>\n", | |
" <td>194.000000</td>\n", | |
" <td>230.000000</td>\n", | |
" <td>180.000000</td>\n", | |
" <td>6.00000</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" # Total HP Attack Defense Sp. Atk \\\n", | |
"count 800.000000 800.00000 800.000000 800.000000 800.000000 800.000000 \n", | |
"mean 362.813750 435.10250 69.258750 79.001250 73.842500 72.820000 \n", | |
"std 208.343798 119.96304 25.534669 32.457366 31.183501 32.722294 \n", | |
"min 1.000000 180.00000 1.000000 5.000000 5.000000 10.000000 \n", | |
"25% 184.750000 330.00000 50.000000 55.000000 50.000000 49.750000 \n", | |
"50% 364.500000 450.00000 65.000000 75.000000 70.000000 65.000000 \n", | |
"75% 539.250000 515.00000 80.000000 100.000000 90.000000 95.000000 \n", | |
"max 721.000000 780.00000 255.000000 190.000000 230.000000 194.000000 \n", | |
"\n", | |
" Sp. Def Speed Generation \n", | |
"count 800.000000 800.000000 800.00000 \n", | |
"mean 71.902500 68.277500 3.32375 \n", | |
"std 27.828916 29.060474 1.66129 \n", | |
"min 20.000000 5.000000 1.00000 \n", | |
"25% 50.000000 45.000000 2.00000 \n", | |
"50% 70.000000 65.000000 3.00000 \n", | |
"75% 90.000000 90.000000 5.00000 \n", | |
"max 230.000000 180.000000 6.00000 " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 78 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "lue3u8cmT3ZP", | |
"colab_type": "code", | |
"outputId": "a2f9b897-b32a-4cc9-c613-fb1ad853c9e1", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 263 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"# Return the dtypes in the DataFrame.\n", | |
"df.dtypes" | |
], | |
"execution_count": 79, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": [ | |
"# int64\n", | |
"Name object\n", | |
"Type 1 object\n", | |
"Type 2 object\n", | |
"Total int64\n", | |
"HP int64\n", | |
"Attack int64\n", | |
"Defense int64\n", | |
"Sp. Atk int64\n", | |
"Sp. Def int64\n", | |
"Speed int64\n", | |
"Generation int64\n", | |
"Legendary bool\n", | |
"dtype: object" | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 79 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "5wbtfngWeoHE", | |
"colab_type": "code", | |
"outputId": "5ee1e7ec-ce40-4fd0-b9f7-02e686c876fd", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 195 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"# show the first 5 rows of the dataframe\n", | |
"df.head()" | |
], | |
"execution_count": 80, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>#</th>\n", | |
" <th>Name</th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>0</th>\n", | |
" <td>1</td>\n", | |
" <td>Bulbasaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>318</td>\n", | |
" <td>45</td>\n", | |
" <td>49</td>\n", | |
" <td>49</td>\n", | |
" <td>65</td>\n", | |
" <td>65</td>\n", | |
" <td>45</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>1</th>\n", | |
" <td>2</td>\n", | |
" <td>Ivysaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>405</td>\n", | |
" <td>60</td>\n", | |
" <td>62</td>\n", | |
" <td>63</td>\n", | |
" <td>80</td>\n", | |
" <td>80</td>\n", | |
" <td>60</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>2</th>\n", | |
" <td>3</td>\n", | |
" <td>Venusaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>525</td>\n", | |
" <td>80</td>\n", | |
" <td>82</td>\n", | |
" <td>83</td>\n", | |
" <td>100</td>\n", | |
" <td>100</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>3</th>\n", | |
" <td>3</td>\n", | |
" <td>VenusaurMega Venusaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>625</td>\n", | |
" <td>80</td>\n", | |
" <td>100</td>\n", | |
" <td>123</td>\n", | |
" <td>122</td>\n", | |
" <td>120</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>4</th>\n", | |
" <td>4</td>\n", | |
" <td>Charmander</td>\n", | |
" <td>Fire</td>\n", | |
" <td>NaN</td>\n", | |
" <td>309</td>\n", | |
" <td>39</td>\n", | |
" <td>52</td>\n", | |
" <td>43</td>\n", | |
" <td>60</td>\n", | |
" <td>50</td>\n", | |
" <td>65</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" # Name Type 1 Type 2 Total HP Attack Defense \\\n", | |
"0 1 Bulbasaur Grass Poison 318 45 49 49 \n", | |
"1 2 Ivysaur Grass Poison 405 60 62 63 \n", | |
"2 3 Venusaur Grass Poison 525 80 82 83 \n", | |
"3 3 VenusaurMega Venusaur Grass Poison 625 80 100 123 \n", | |
"4 4 Charmander Fire NaN 309 39 52 43 \n", | |
"\n", | |
" Sp. Atk Sp. Def Speed Generation Legendary \n", | |
"0 65 65 45 1 False \n", | |
"1 80 80 60 1 False \n", | |
"2 100 100 80 1 False \n", | |
"3 122 120 80 1 False \n", | |
"4 60 50 65 1 False " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 80 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "1jWzPlrQs1X4", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Pokemon Types " | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "MdnmPs9Os379", | |
"colab_type": "code", | |
"outputId": "3372704d-7fec-4b67-da07-6f226c0f3a75", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 70 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df['Type 1'].unique()" | |
], | |
"execution_count": 81, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": [ | |
"array(['Grass', 'Fire', 'Water', 'Bug', 'Normal', 'Poison', 'Electric',\n", | |
" 'Ground', 'Fairy', 'Fighting', 'Psychic', 'Rock', 'Ghost', 'Ice',\n", | |
" 'Dragon', 'Dark', 'Steel', 'Flying'], dtype=object)" | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 81 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "hGjz13CdfO_0", | |
"colab_type": "code", | |
"outputId": "81ff0ae9-c726-483f-dfa5-bbf6cd3e0edf", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 140 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"print(df[\"Speed\"].unique())\n", | |
"df[\"Speed\"].nunique()\n", | |
"# there are only 108 unique speeds in this dataset" | |
], | |
"execution_count": 82, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": [ | |
"[ 45 60 80 65 100 43 58 78 30 70 50 35 75 145 56 71 101 121\n", | |
" 72 97 55 90 110 40 41 76 85 20 25 95 120 115 105 150 15 130\n", | |
" 42 67 140 87 63 68 93 81 48 91 33 5 83 51 61 125 160 28\n", | |
" 135 10 23 32 52 180 31 36 108 66 34 39 112 74 84 82 102 92\n", | |
" 47 46 86 77 127 113 106 64 24 29 116 114 88 69 57 98 22 44\n", | |
" 59 79 103 109 38 111 128 99 73 104 122 62 126 89 49 118 54 123]\n" | |
], | |
"name": "stdout" | |
}, | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": [ | |
"108" | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 82 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "U2rL33BZf5Kc", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"If you want to get the value count for each speed use the value_counts() function" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "LCFZFoQKf93D", | |
"colab_type": "code", | |
"outputId": "e979b1f8-9ad3-4594-9e3e-3cf2ff18ffc1", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 1108 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df[\"Speed\"].value_counts()" | |
], | |
"execution_count": 83, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": [ | |
"50 46\n", | |
"60 44\n", | |
"70 37\n", | |
"65 36\n", | |
"30 35\n", | |
"80 33\n", | |
"40 32\n", | |
"90 31\n", | |
"100 31\n", | |
"55 30\n", | |
"45 29\n", | |
"85 27\n", | |
"95 27\n", | |
"35 22\n", | |
"75 16\n", | |
"110 15\n", | |
"20 15\n", | |
"105 12\n", | |
"115 11\n", | |
"25 10\n", | |
"15 9\n", | |
"58 8\n", | |
"108 7\n", | |
"101 7\n", | |
"68 6\n", | |
"130 6\n", | |
"48 6\n", | |
"71 6\n", | |
"42 6\n", | |
"91 5\n", | |
" ..\n", | |
"116 2\n", | |
"77 2\n", | |
"135 2\n", | |
"73 2\n", | |
"140 2\n", | |
"69 2\n", | |
"63 2\n", | |
"128 1\n", | |
"22 1\n", | |
"127 1\n", | |
"114 1\n", | |
"126 1\n", | |
"123 1\n", | |
"122 1\n", | |
"118 1\n", | |
"24 1\n", | |
"39 1\n", | |
"34 1\n", | |
"113 1\n", | |
"62 1\n", | |
"103 1\n", | |
"89 1\n", | |
"88 1\n", | |
"87 1\n", | |
"82 1\n", | |
"79 1\n", | |
"49 1\n", | |
"160 1\n", | |
"54 1\n", | |
"180 1\n", | |
"Name: Speed, Length: 108, dtype: int64" | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 83 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "8bfYyvc1gOEr", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"As you can see the speed value **50** is the most common among pokemons" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "LdF5fzcAfECF", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Fastest pokemon\n", | |
" to get the row number of the fastest pokemon we use\n", | |
" \n", | |
"\n", | |
"```\n", | |
"df['Speed'].idxmax()\n", | |
"```\n", | |
"This will return only the row index, to get the entire row we use - \n", | |
"\n", | |
"\n", | |
"```\n", | |
"df.iloc[df['Speed'].idxmax()]\n", | |
"```\n", | |
"Its basically getting the row using the row number like we get an array[0] element.\n", | |
"\n", | |
"\n", | |
"\n" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "9R4j-xABhop9", | |
"colab_type": "code", | |
"outputId": "cdb9f91c-40c1-4b1e-d642-dcff2f81884d", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 281 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"print(\"Pokemon with the fastest speed of:\",df['Speed'].idxmax())\n", | |
"df.iloc[df['Speed'].idxmax()]\n" | |
], | |
"execution_count": 84, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": [ | |
"Pokemon with the fastest speed of: 431\n" | |
], | |
"name": "stdout" | |
}, | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": [ | |
"# 386\n", | |
"Name DeoxysSpeed Forme\n", | |
"Type 1 Psychic\n", | |
"Type 2 NaN\n", | |
"Total 600\n", | |
"HP 50\n", | |
"Attack 95\n", | |
"Defense 90\n", | |
"Sp. Atk 95\n", | |
"Sp. Def 90\n", | |
"Speed 180\n", | |
"Generation 3\n", | |
"Legendary True\n", | |
"Name: 431, dtype: object" | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 84 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "ltIcjGc8ig20", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"\n", | |
"Fastest pokemon" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "wu5ubKS4iyq4", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Greatest attack\n", | |
"We can do the same to find the pokemon with the greatest attack power which is mewtwo X\n", | |
"\n", | |
"\n" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "Akea9oL8hri4", | |
"colab_type": "code", | |
"outputId": "3039aa13-483b-46b5-8e73-39fd387f7a71", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 281 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"print(\"Pokemon with the fastest speed of:\",df['Attack'].idxmax())\n", | |
"df.iloc[df['Attack'].idxmax()]\n" | |
], | |
"execution_count": 85, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": [ | |
"Pokemon with the fastest speed of: 163\n" | |
], | |
"name": "stdout" | |
}, | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": [ | |
"# 150\n", | |
"Name MewtwoMega Mewtwo X\n", | |
"Type 1 Psychic\n", | |
"Type 2 Fighting\n", | |
"Total 780\n", | |
"HP 106\n", | |
"Attack 190\n", | |
"Defense 100\n", | |
"Sp. Atk 154\n", | |
"Sp. Def 100\n", | |
"Speed 130\n", | |
"Generation 1\n", | |
"Legendary True\n", | |
"Name: 163, dtype: object" | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 85 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "OhnwUygoj6tk", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# legendary pokemon\n", | |
"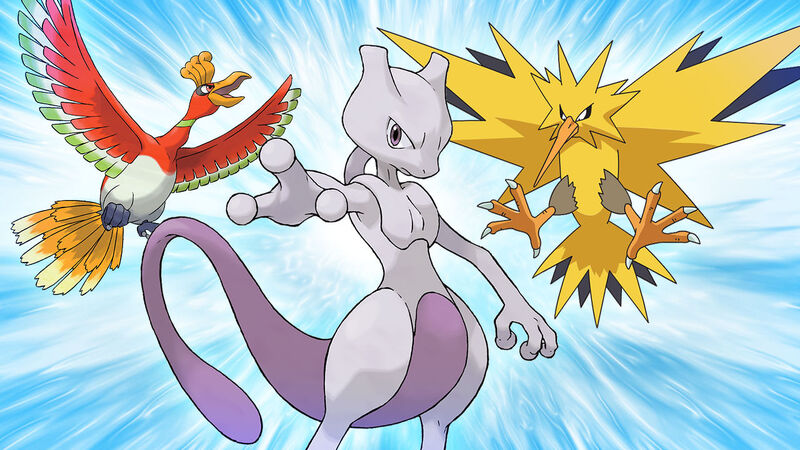\n", | |
"\n", | |
"* You might have noticed a column called legendary in the dataset. \n", | |
"* The value of that column is **True** if th particular pokemon is legendary.\n", | |
"* Let's select only legendary pokemon" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "3xxdTb4Vi-Qw", | |
"colab_type": "code", | |
"outputId": "1046c12c-94ee-4bd0-836f-270572f0b07e", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 122 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"true_false_list = df['Legendary']==True\n", | |
"print(true_false_list[:5])" | |
], | |
"execution_count": 86, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": [ | |
"0 False\n", | |
"1 False\n", | |
"2 False\n", | |
"3 False\n", | |
"4 False\n", | |
"Name: Legendary, dtype: bool\n" | |
], | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "hPkeg0zDmzIn", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"Now this is a list of True and False values telling us the rows which are legendary and which are not. To get the actual dataframe for jsut the legendary pokemon." | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "QL1pMGc8ntb5", | |
"colab_type": "code", | |
"outputId": "49c093af-8082-425e-96d2-3f5b3b2674f0", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 195 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"legendary = df[df['Legendary']==True]\n", | |
"# or\n", | |
"df[true_false_list].head()\n" | |
], | |
"execution_count": 87, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>#</th>\n", | |
" <th>Name</th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>156</th>\n", | |
" <td>144</td>\n", | |
" <td>Articuno</td>\n", | |
" <td>Ice</td>\n", | |
" <td>Flying</td>\n", | |
" <td>580</td>\n", | |
" <td>90</td>\n", | |
" <td>85</td>\n", | |
" <td>100</td>\n", | |
" <td>95</td>\n", | |
" <td>125</td>\n", | |
" <td>85</td>\n", | |
" <td>1</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>157</th>\n", | |
" <td>145</td>\n", | |
" <td>Zapdos</td>\n", | |
" <td>Electric</td>\n", | |
" <td>Flying</td>\n", | |
" <td>580</td>\n", | |
" <td>90</td>\n", | |
" <td>90</td>\n", | |
" <td>85</td>\n", | |
" <td>125</td>\n", | |
" <td>90</td>\n", | |
" <td>100</td>\n", | |
" <td>1</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>158</th>\n", | |
" <td>146</td>\n", | |
" <td>Moltres</td>\n", | |
" <td>Fire</td>\n", | |
" <td>Flying</td>\n", | |
" <td>580</td>\n", | |
" <td>90</td>\n", | |
" <td>100</td>\n", | |
" <td>90</td>\n", | |
" <td>125</td>\n", | |
" <td>85</td>\n", | |
" <td>90</td>\n", | |
" <td>1</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>162</th>\n", | |
" <td>150</td>\n", | |
" <td>Mewtwo</td>\n", | |
" <td>Psychic</td>\n", | |
" <td>NaN</td>\n", | |
" <td>680</td>\n", | |
" <td>106</td>\n", | |
" <td>110</td>\n", | |
" <td>90</td>\n", | |
" <td>154</td>\n", | |
" <td>90</td>\n", | |
" <td>130</td>\n", | |
" <td>1</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>163</th>\n", | |
" <td>150</td>\n", | |
" <td>MewtwoMega Mewtwo X</td>\n", | |
" <td>Psychic</td>\n", | |
" <td>Fighting</td>\n", | |
" <td>780</td>\n", | |
" <td>106</td>\n", | |
" <td>190</td>\n", | |
" <td>100</td>\n", | |
" <td>154</td>\n", | |
" <td>100</td>\n", | |
" <td>130</td>\n", | |
" <td>1</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" # Name Type 1 Type 2 Total HP Attack \\\n", | |
"156 144 Articuno Ice Flying 580 90 85 \n", | |
"157 145 Zapdos Electric Flying 580 90 90 \n", | |
"158 146 Moltres Fire Flying 580 90 100 \n", | |
"162 150 Mewtwo Psychic NaN 680 106 110 \n", | |
"163 150 MewtwoMega Mewtwo X Psychic Fighting 780 106 190 \n", | |
"\n", | |
" Defense Sp. Atk Sp. Def Speed Generation Legendary \n", | |
"156 100 95 125 85 1 True \n", | |
"157 85 125 90 100 1 True \n", | |
"158 90 125 85 90 1 True \n", | |
"162 90 154 90 130 1 True \n", | |
"163 100 154 100 130 1 True " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 87 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "6jv7ycddtQRL", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Deleting a column\n", | |
"* we can use **drop**" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "Dj7HvT6YmMdA", | |
"colab_type": "code", | |
"colab": {} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df=df.drop(['#'],axis=1)" | |
], | |
"execution_count": 0, | |
"outputs": [] | |
}, | |
{ | |
"metadata": { | |
"id": "NPsAS-k_truS", | |
"colab_type": "code", | |
"outputId": "d77c357f-4e87-43fd-ec5a-e6ecefe1da7e", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 195 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df.head()" | |
], | |
"execution_count": 89, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>Name</th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>0</th>\n", | |
" <td>Bulbasaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>318</td>\n", | |
" <td>45</td>\n", | |
" <td>49</td>\n", | |
" <td>49</td>\n", | |
" <td>65</td>\n", | |
" <td>65</td>\n", | |
" <td>45</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>1</th>\n", | |
" <td>Ivysaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>405</td>\n", | |
" <td>60</td>\n", | |
" <td>62</td>\n", | |
" <td>63</td>\n", | |
" <td>80</td>\n", | |
" <td>80</td>\n", | |
" <td>60</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>2</th>\n", | |
" <td>Venusaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>525</td>\n", | |
" <td>80</td>\n", | |
" <td>82</td>\n", | |
" <td>83</td>\n", | |
" <td>100</td>\n", | |
" <td>100</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>3</th>\n", | |
" <td>VenusaurMega Venusaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>625</td>\n", | |
" <td>80</td>\n", | |
" <td>100</td>\n", | |
" <td>123</td>\n", | |
" <td>122</td>\n", | |
" <td>120</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>4</th>\n", | |
" <td>Charmander</td>\n", | |
" <td>Fire</td>\n", | |
" <td>NaN</td>\n", | |
" <td>309</td>\n", | |
" <td>39</td>\n", | |
" <td>52</td>\n", | |
" <td>43</td>\n", | |
" <td>60</td>\n", | |
" <td>50</td>\n", | |
" <td>65</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" Name Type 1 Type 2 Total HP Attack Defense Sp. Atk \\\n", | |
"0 Bulbasaur Grass Poison 318 45 49 49 65 \n", | |
"1 Ivysaur Grass Poison 405 60 62 63 80 \n", | |
"2 Venusaur Grass Poison 525 80 82 83 100 \n", | |
"3 VenusaurMega Venusaur Grass Poison 625 80 100 123 122 \n", | |
"4 Charmander Fire NaN 309 39 52 43 60 \n", | |
"\n", | |
" Sp. Def Speed Generation Legendary \n", | |
"0 65 45 1 False \n", | |
"1 80 60 1 False \n", | |
"2 100 80 1 False \n", | |
"3 120 80 1 False \n", | |
"4 50 65 1 False " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 89 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "6qQkwJm9vjlV", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Deleting a row\n", | |
"use axis= 0 to delete rows\n" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "Eh9_4iOyvv0F", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Select" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "nu3VDNzyv1sM", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"## By column" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "a2nnYhhnvcRV", | |
"colab_type": "code", | |
"outputId": "eeb4e520-fc05-4ac7-8378-35c867048dab", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 122 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df[\"Name\"][:5]" | |
], | |
"execution_count": 90, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": [ | |
"0 Bulbasaur\n", | |
"1 Ivysaur\n", | |
"2 Venusaur\n", | |
"3 VenusaurMega Venusaur\n", | |
"4 Charmander\n", | |
"Name: Name, dtype: object" | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 90 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "oPY5fs4VwDRi", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"## By index" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "ZKyhyD9Vv_rP", | |
"colab_type": "code", | |
"outputId": "f5847ddd-a55f-489f-c31f-4480ecaeac38", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 246 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df.iloc[0]" | |
], | |
"execution_count": 91, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": [ | |
"Name Bulbasaur\n", | |
"Type 1 Grass\n", | |
"Type 2 Poison\n", | |
"Total 318\n", | |
"HP 45\n", | |
"Attack 49\n", | |
"Defense 49\n", | |
"Sp. Atk 65\n", | |
"Sp. Def 65\n", | |
"Speed 45\n", | |
"Generation 1\n", | |
"Legendary False\n", | |
"Name: 0, dtype: object" | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 91 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "0zbM_Vj-wWlN", | |
"colab_type": "code", | |
"outputId": "6d6527d8-84bd-43f0-88ea-39a0d74ad3d0", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 34 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"try:\n", | |
" df.loc[\"Pikachu\"]\n", | |
"except Exception as e:\n", | |
" print(e)" | |
], | |
"execution_count": 92, | |
"outputs": [ | |
{ | |
"output_type": "stream", | |
"text": [ | |
"'the label [Pikachu] is not in the [index]'\n" | |
], | |
"name": "stdout" | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "p0o_udCny9KL", | |
"colab_type": "code", | |
"colab": {} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"# Setting names as the index for the dataframe\n", | |
"df = df.set_index('Name')" | |
], | |
"execution_count": 0, | |
"outputs": [] | |
}, | |
{ | |
"metadata": { | |
"id": "SmhNJlhCzX0a", | |
"colab_type": "code", | |
"outputId": "39d01b1c-dd75-4576-8076-3301024f33e0", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 228 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"# Trying that again\n", | |
"df.loc[\"Pikachu\"]" | |
], | |
"execution_count": 94, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/plain": [ | |
"Type 1 Electric\n", | |
"Type 2 NaN\n", | |
"Total 320\n", | |
"HP 35\n", | |
"Attack 55\n", | |
"Defense 40\n", | |
"Sp. Atk 50\n", | |
"Sp. Def 50\n", | |
"Speed 90\n", | |
"Generation 1\n", | |
"Legendary False\n", | |
"Name: Pikachu, dtype: object" | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 94 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "96SFzdhQzZ3e", | |
"colab_type": "code", | |
"outputId": "7956887a-0573-464d-e0c7-9afaba4a930c", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 166 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df[4:7]" | |
], | |
"execution_count": 95, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Name</th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>Charmander</th>\n", | |
" <td>Fire</td>\n", | |
" <td>NaN</td>\n", | |
" <td>309</td>\n", | |
" <td>39</td>\n", | |
" <td>52</td>\n", | |
" <td>43</td>\n", | |
" <td>60</td>\n", | |
" <td>50</td>\n", | |
" <td>65</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Charmeleon</th>\n", | |
" <td>Fire</td>\n", | |
" <td>NaN</td>\n", | |
" <td>405</td>\n", | |
" <td>58</td>\n", | |
" <td>64</td>\n", | |
" <td>58</td>\n", | |
" <td>80</td>\n", | |
" <td>65</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Charizard</th>\n", | |
" <td>Fire</td>\n", | |
" <td>Flying</td>\n", | |
" <td>534</td>\n", | |
" <td>78</td>\n", | |
" <td>84</td>\n", | |
" <td>78</td>\n", | |
" <td>109</td>\n", | |
" <td>85</td>\n", | |
" <td>100</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" Type 1 Type 2 Total HP Attack Defense Sp. Atk Sp. Def \\\n", | |
"Name \n", | |
"Charmander Fire NaN 309 39 52 43 60 50 \n", | |
"Charmeleon Fire NaN 405 58 64 58 80 65 \n", | |
"Charizard Fire Flying 534 78 84 78 109 85 \n", | |
"\n", | |
" Speed Generation Legendary \n", | |
"Name \n", | |
"Charmander 65 1 False \n", | |
"Charmeleon 80 1 False \n", | |
"Charizard 100 1 False " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 95 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "j-jBV_Uj29ON", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Filtering\n", | |
"* To filter data in a dataframe you can use logical operators" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "aBE1n4B108ft", | |
"colab_type": "code", | |
"outputId": "ca320166-82a9-4420-8ab4-4cadc6106a94", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 225 | |
} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df[((df['Type 1']=='Ghost') | (df['Type 1']=='Dragon')) & ((df['Attack'] > 50))].head()\n" | |
], | |
"execution_count": 96, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Name</th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>Gengar</th>\n", | |
" <td>Ghost</td>\n", | |
" <td>Poison</td>\n", | |
" <td>500</td>\n", | |
" <td>60</td>\n", | |
" <td>65</td>\n", | |
" <td>60</td>\n", | |
" <td>130</td>\n", | |
" <td>75</td>\n", | |
" <td>110</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>GengarMega Gengar</th>\n", | |
" <td>Ghost</td>\n", | |
" <td>Poison</td>\n", | |
" <td>600</td>\n", | |
" <td>60</td>\n", | |
" <td>65</td>\n", | |
" <td>80</td>\n", | |
" <td>170</td>\n", | |
" <td>95</td>\n", | |
" <td>130</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Dratini</th>\n", | |
" <td>Dragon</td>\n", | |
" <td>NaN</td>\n", | |
" <td>300</td>\n", | |
" <td>41</td>\n", | |
" <td>64</td>\n", | |
" <td>45</td>\n", | |
" <td>50</td>\n", | |
" <td>50</td>\n", | |
" <td>50</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Dragonair</th>\n", | |
" <td>Dragon</td>\n", | |
" <td>NaN</td>\n", | |
" <td>420</td>\n", | |
" <td>61</td>\n", | |
" <td>84</td>\n", | |
" <td>65</td>\n", | |
" <td>70</td>\n", | |
" <td>70</td>\n", | |
" <td>70</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Dragonite</th>\n", | |
" <td>Dragon</td>\n", | |
" <td>Flying</td>\n", | |
" <td>600</td>\n", | |
" <td>91</td>\n", | |
" <td>134</td>\n", | |
" <td>95</td>\n", | |
" <td>100</td>\n", | |
" <td>100</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" Type 1 Type 2 Total HP Attack Defense Sp. Atk \\\n", | |
"Name \n", | |
"Gengar Ghost Poison 500 60 65 60 130 \n", | |
"GengarMega Gengar Ghost Poison 600 60 65 80 170 \n", | |
"Dratini Dragon NaN 300 41 64 45 50 \n", | |
"Dragonair Dragon NaN 420 61 84 65 70 \n", | |
"Dragonite Dragon Flying 600 91 134 95 100 \n", | |
"\n", | |
" Sp. Def Speed Generation Legendary \n", | |
"Name \n", | |
"Gengar 75 110 1 False \n", | |
"GengarMega Gengar 95 130 1 False \n", | |
"Dratini 50 50 1 False \n", | |
"Dragonair 70 70 1 False \n", | |
"Dragonite 100 80 1 False " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 96 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "gUBCDjoW4Hq7", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Replacing NaN values\n", | |
"* As you can see in the above filter, some of the values in **Type 2** are not available and are labelled as **NaN**\n", | |
"* Let's replace the NaN values in Type 2 with the values in Type 1\n", | |
"* pandas primarily uses the value np.nan to represent missing data.\n" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "_1ZVevf24OqE", | |
"colab_type": "code", | |
"colab": {} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df['Type 2'].fillna(df['Type 1'], inplace=True) #fill NaN values in Type2 with corresponding values of Type\n", | |
"# You can replace df[\"Type 1\"] with an list too, provided that the length is the same." | |
], | |
"execution_count": 0, | |
"outputs": [] | |
}, | |
{ | |
"metadata": { | |
"id": "dYZ5wtlT5MtA", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"Let's try the filter again after the replacement" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "z8_JWmEa5KeH", | |
"colab_type": "code", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 225 | |
}, | |
"outputId": "e80d892e-b941-4125-ea5c-208ca4b92bee" | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df[((df['Type 1']=='Ghost') | (df['Type 1']=='Dragon')) & ((df['Attack'] > 50))].head()\n", | |
"# No more NaN values in Type 2" | |
], | |
"execution_count": 98, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Name</th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>Gengar</th>\n", | |
" <td>Ghost</td>\n", | |
" <td>Poison</td>\n", | |
" <td>500</td>\n", | |
" <td>60</td>\n", | |
" <td>65</td>\n", | |
" <td>60</td>\n", | |
" <td>130</td>\n", | |
" <td>75</td>\n", | |
" <td>110</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>GengarMega Gengar</th>\n", | |
" <td>Ghost</td>\n", | |
" <td>Poison</td>\n", | |
" <td>600</td>\n", | |
" <td>60</td>\n", | |
" <td>65</td>\n", | |
" <td>80</td>\n", | |
" <td>170</td>\n", | |
" <td>95</td>\n", | |
" <td>130</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Dratini</th>\n", | |
" <td>Dragon</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>300</td>\n", | |
" <td>41</td>\n", | |
" <td>64</td>\n", | |
" <td>45</td>\n", | |
" <td>50</td>\n", | |
" <td>50</td>\n", | |
" <td>50</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Dragonair</th>\n", | |
" <td>Dragon</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>420</td>\n", | |
" <td>61</td>\n", | |
" <td>84</td>\n", | |
" <td>65</td>\n", | |
" <td>70</td>\n", | |
" <td>70</td>\n", | |
" <td>70</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Dragonite</th>\n", | |
" <td>Dragon</td>\n", | |
" <td>Flying</td>\n", | |
" <td>600</td>\n", | |
" <td>91</td>\n", | |
" <td>134</td>\n", | |
" <td>95</td>\n", | |
" <td>100</td>\n", | |
" <td>100</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" Type 1 Type 2 Total HP Attack Defense Sp. Atk \\\n", | |
"Name \n", | |
"Gengar Ghost Poison 500 60 65 60 130 \n", | |
"GengarMega Gengar Ghost Poison 600 60 65 80 170 \n", | |
"Dratini Dragon Dragon 300 41 64 45 50 \n", | |
"Dragonair Dragon Dragon 420 61 84 65 70 \n", | |
"Dragonite Dragon Flying 600 91 134 95 100 \n", | |
"\n", | |
" Sp. Def Speed Generation Legendary \n", | |
"Name \n", | |
"Gengar 75 110 1 False \n", | |
"GengarMega Gengar 95 130 1 False \n", | |
"Dratini 50 50 1 False \n", | |
"Dragonair 70 70 1 False \n", | |
"Dragonite 100 80 1 False " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 98 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "72lKP1d15iwf", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Groupby\n", | |
"* The **groupby** function - groups series using mapper (dict or key function, apply given function to group, return result as series) or by a series of columns.\n", | |
"* Let us group our pokemon by Generation and Type\n", | |
"\n" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "u5U-X7AG5Uvb", | |
"colab_type": "code", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 1911 | |
}, | |
"outputId": "9bcde6a2-fca0-42b8-f4f2-0bbd9acccba6" | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df2=df.groupby(['Generation','Type 1'])\n", | |
"df2.head()" | |
], | |
"execution_count": 99, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Name</th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>Bulbasaur</th>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>318</td>\n", | |
" <td>45</td>\n", | |
" <td>49</td>\n", | |
" <td>49</td>\n", | |
" <td>65</td>\n", | |
" <td>65</td>\n", | |
" <td>45</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Ivysaur</th>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>405</td>\n", | |
" <td>60</td>\n", | |
" <td>62</td>\n", | |
" <td>63</td>\n", | |
" <td>80</td>\n", | |
" <td>80</td>\n", | |
" <td>60</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Venusaur</th>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>525</td>\n", | |
" <td>80</td>\n", | |
" <td>82</td>\n", | |
" <td>83</td>\n", | |
" <td>100</td>\n", | |
" <td>100</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>VenusaurMega Venusaur</th>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>625</td>\n", | |
" <td>80</td>\n", | |
" <td>100</td>\n", | |
" <td>123</td>\n", | |
" <td>122</td>\n", | |
" <td>120</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Charmander</th>\n", | |
" <td>Fire</td>\n", | |
" <td>Fire</td>\n", | |
" <td>309</td>\n", | |
" <td>39</td>\n", | |
" <td>52</td>\n", | |
" <td>43</td>\n", | |
" <td>60</td>\n", | |
" <td>50</td>\n", | |
" <td>65</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Charmeleon</th>\n", | |
" <td>Fire</td>\n", | |
" <td>Fire</td>\n", | |
" <td>405</td>\n", | |
" <td>58</td>\n", | |
" <td>64</td>\n", | |
" <td>58</td>\n", | |
" <td>80</td>\n", | |
" <td>65</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Charizard</th>\n", | |
" <td>Fire</td>\n", | |
" <td>Flying</td>\n", | |
" <td>534</td>\n", | |
" <td>78</td>\n", | |
" <td>84</td>\n", | |
" <td>78</td>\n", | |
" <td>109</td>\n", | |
" <td>85</td>\n", | |
" <td>100</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>CharizardMega Charizard X</th>\n", | |
" <td>Fire</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>634</td>\n", | |
" <td>78</td>\n", | |
" <td>130</td>\n", | |
" <td>111</td>\n", | |
" <td>130</td>\n", | |
" <td>85</td>\n", | |
" <td>100</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>CharizardMega Charizard Y</th>\n", | |
" <td>Fire</td>\n", | |
" <td>Flying</td>\n", | |
" <td>634</td>\n", | |
" <td>78</td>\n", | |
" <td>104</td>\n", | |
" <td>78</td>\n", | |
" <td>159</td>\n", | |
" <td>115</td>\n", | |
" <td>100</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Squirtle</th>\n", | |
" <td>Water</td>\n", | |
" <td>Water</td>\n", | |
" <td>314</td>\n", | |
" <td>44</td>\n", | |
" <td>48</td>\n", | |
" <td>65</td>\n", | |
" <td>50</td>\n", | |
" <td>64</td>\n", | |
" <td>43</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Wartortle</th>\n", | |
" <td>Water</td>\n", | |
" <td>Water</td>\n", | |
" <td>405</td>\n", | |
" <td>59</td>\n", | |
" <td>63</td>\n", | |
" <td>80</td>\n", | |
" <td>65</td>\n", | |
" <td>80</td>\n", | |
" <td>58</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Blastoise</th>\n", | |
" <td>Water</td>\n", | |
" <td>Water</td>\n", | |
" <td>530</td>\n", | |
" <td>79</td>\n", | |
" <td>83</td>\n", | |
" <td>100</td>\n", | |
" <td>85</td>\n", | |
" <td>105</td>\n", | |
" <td>78</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>BlastoiseMega Blastoise</th>\n", | |
" <td>Water</td>\n", | |
" <td>Water</td>\n", | |
" <td>630</td>\n", | |
" <td>79</td>\n", | |
" <td>103</td>\n", | |
" <td>120</td>\n", | |
" <td>135</td>\n", | |
" <td>115</td>\n", | |
" <td>78</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Caterpie</th>\n", | |
" <td>Bug</td>\n", | |
" <td>Bug</td>\n", | |
" <td>195</td>\n", | |
" <td>45</td>\n", | |
" <td>30</td>\n", | |
" <td>35</td>\n", | |
" <td>20</td>\n", | |
" <td>20</td>\n", | |
" <td>45</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Metapod</th>\n", | |
" <td>Bug</td>\n", | |
" <td>Bug</td>\n", | |
" <td>205</td>\n", | |
" <td>50</td>\n", | |
" <td>20</td>\n", | |
" <td>55</td>\n", | |
" <td>25</td>\n", | |
" <td>25</td>\n", | |
" <td>30</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Butterfree</th>\n", | |
" <td>Bug</td>\n", | |
" <td>Flying</td>\n", | |
" <td>395</td>\n", | |
" <td>60</td>\n", | |
" <td>45</td>\n", | |
" <td>50</td>\n", | |
" <td>90</td>\n", | |
" <td>80</td>\n", | |
" <td>70</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Weedle</th>\n", | |
" <td>Bug</td>\n", | |
" <td>Poison</td>\n", | |
" <td>195</td>\n", | |
" <td>40</td>\n", | |
" <td>35</td>\n", | |
" <td>30</td>\n", | |
" <td>20</td>\n", | |
" <td>20</td>\n", | |
" <td>50</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Kakuna</th>\n", | |
" <td>Bug</td>\n", | |
" <td>Poison</td>\n", | |
" <td>205</td>\n", | |
" <td>45</td>\n", | |
" <td>25</td>\n", | |
" <td>50</td>\n", | |
" <td>25</td>\n", | |
" <td>25</td>\n", | |
" <td>35</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Pidgey</th>\n", | |
" <td>Normal</td>\n", | |
" <td>Flying</td>\n", | |
" <td>251</td>\n", | |
" <td>40</td>\n", | |
" <td>45</td>\n", | |
" <td>40</td>\n", | |
" <td>35</td>\n", | |
" <td>35</td>\n", | |
" <td>56</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Pidgeotto</th>\n", | |
" <td>Normal</td>\n", | |
" <td>Flying</td>\n", | |
" <td>349</td>\n", | |
" <td>63</td>\n", | |
" <td>60</td>\n", | |
" <td>55</td>\n", | |
" <td>50</td>\n", | |
" <td>50</td>\n", | |
" <td>71</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Pidgeot</th>\n", | |
" <td>Normal</td>\n", | |
" <td>Flying</td>\n", | |
" <td>479</td>\n", | |
" <td>83</td>\n", | |
" <td>80</td>\n", | |
" <td>75</td>\n", | |
" <td>70</td>\n", | |
" <td>70</td>\n", | |
" <td>101</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>PidgeotMega Pidgeot</th>\n", | |
" <td>Normal</td>\n", | |
" <td>Flying</td>\n", | |
" <td>579</td>\n", | |
" <td>83</td>\n", | |
" <td>80</td>\n", | |
" <td>80</td>\n", | |
" <td>135</td>\n", | |
" <td>80</td>\n", | |
" <td>121</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Rattata</th>\n", | |
" <td>Normal</td>\n", | |
" <td>Normal</td>\n", | |
" <td>253</td>\n", | |
" <td>30</td>\n", | |
" <td>56</td>\n", | |
" <td>35</td>\n", | |
" <td>25</td>\n", | |
" <td>35</td>\n", | |
" <td>72</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Ekans</th>\n", | |
" <td>Poison</td>\n", | |
" <td>Poison</td>\n", | |
" <td>288</td>\n", | |
" <td>35</td>\n", | |
" <td>60</td>\n", | |
" <td>44</td>\n", | |
" <td>40</td>\n", | |
" <td>54</td>\n", | |
" <td>55</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Arbok</th>\n", | |
" <td>Poison</td>\n", | |
" <td>Poison</td>\n", | |
" <td>438</td>\n", | |
" <td>60</td>\n", | |
" <td>85</td>\n", | |
" <td>69</td>\n", | |
" <td>65</td>\n", | |
" <td>79</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Pikachu</th>\n", | |
" <td>Electric</td>\n", | |
" <td>Electric</td>\n", | |
" <td>320</td>\n", | |
" <td>35</td>\n", | |
" <td>55</td>\n", | |
" <td>40</td>\n", | |
" <td>50</td>\n", | |
" <td>50</td>\n", | |
" <td>90</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Raichu</th>\n", | |
" <td>Electric</td>\n", | |
" <td>Electric</td>\n", | |
" <td>485</td>\n", | |
" <td>60</td>\n", | |
" <td>90</td>\n", | |
" <td>55</td>\n", | |
" <td>90</td>\n", | |
" <td>80</td>\n", | |
" <td>110</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Sandshrew</th>\n", | |
" <td>Ground</td>\n", | |
" <td>Ground</td>\n", | |
" <td>300</td>\n", | |
" <td>50</td>\n", | |
" <td>75</td>\n", | |
" <td>85</td>\n", | |
" <td>20</td>\n", | |
" <td>30</td>\n", | |
" <td>40</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Sandslash</th>\n", | |
" <td>Ground</td>\n", | |
" <td>Ground</td>\n", | |
" <td>450</td>\n", | |
" <td>75</td>\n", | |
" <td>100</td>\n", | |
" <td>110</td>\n", | |
" <td>45</td>\n", | |
" <td>55</td>\n", | |
" <td>65</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Nidoran♀</th>\n", | |
" <td>Poison</td>\n", | |
" <td>Poison</td>\n", | |
" <td>275</td>\n", | |
" <td>55</td>\n", | |
" <td>47</td>\n", | |
" <td>52</td>\n", | |
" <td>40</td>\n", | |
" <td>40</td>\n", | |
" <td>41</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>...</th>\n", | |
" <td>...</td>\n", | |
" <td>...</td>\n", | |
" <td>...</td>\n", | |
" <td>...</td>\n", | |
" <td>...</td>\n", | |
" <td>...</td>\n", | |
" <td>...</td>\n", | |
" <td>...</td>\n", | |
" <td>...</td>\n", | |
" <td>...</td>\n", | |
" <td>...</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Binacle</th>\n", | |
" <td>Rock</td>\n", | |
" <td>Water</td>\n", | |
" <td>306</td>\n", | |
" <td>42</td>\n", | |
" <td>52</td>\n", | |
" <td>67</td>\n", | |
" <td>39</td>\n", | |
" <td>56</td>\n", | |
" <td>50</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Barbaracle</th>\n", | |
" <td>Rock</td>\n", | |
" <td>Water</td>\n", | |
" <td>500</td>\n", | |
" <td>72</td>\n", | |
" <td>105</td>\n", | |
" <td>115</td>\n", | |
" <td>54</td>\n", | |
" <td>86</td>\n", | |
" <td>68</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Skrelp</th>\n", | |
" <td>Poison</td>\n", | |
" <td>Water</td>\n", | |
" <td>320</td>\n", | |
" <td>50</td>\n", | |
" <td>60</td>\n", | |
" <td>60</td>\n", | |
" <td>60</td>\n", | |
" <td>60</td>\n", | |
" <td>30</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Dragalge</th>\n", | |
" <td>Poison</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>494</td>\n", | |
" <td>65</td>\n", | |
" <td>75</td>\n", | |
" <td>90</td>\n", | |
" <td>97</td>\n", | |
" <td>123</td>\n", | |
" <td>44</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Clauncher</th>\n", | |
" <td>Water</td>\n", | |
" <td>Water</td>\n", | |
" <td>330</td>\n", | |
" <td>50</td>\n", | |
" <td>53</td>\n", | |
" <td>62</td>\n", | |
" <td>58</td>\n", | |
" <td>63</td>\n", | |
" <td>44</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Clawitzer</th>\n", | |
" <td>Water</td>\n", | |
" <td>Water</td>\n", | |
" <td>500</td>\n", | |
" <td>71</td>\n", | |
" <td>73</td>\n", | |
" <td>88</td>\n", | |
" <td>120</td>\n", | |
" <td>89</td>\n", | |
" <td>59</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Helioptile</th>\n", | |
" <td>Electric</td>\n", | |
" <td>Normal</td>\n", | |
" <td>289</td>\n", | |
" <td>44</td>\n", | |
" <td>38</td>\n", | |
" <td>33</td>\n", | |
" <td>61</td>\n", | |
" <td>43</td>\n", | |
" <td>70</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Heliolisk</th>\n", | |
" <td>Electric</td>\n", | |
" <td>Normal</td>\n", | |
" <td>481</td>\n", | |
" <td>62</td>\n", | |
" <td>55</td>\n", | |
" <td>52</td>\n", | |
" <td>109</td>\n", | |
" <td>94</td>\n", | |
" <td>109</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Tyrunt</th>\n", | |
" <td>Rock</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>362</td>\n", | |
" <td>58</td>\n", | |
" <td>89</td>\n", | |
" <td>77</td>\n", | |
" <td>45</td>\n", | |
" <td>45</td>\n", | |
" <td>48</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Tyrantrum</th>\n", | |
" <td>Rock</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>521</td>\n", | |
" <td>82</td>\n", | |
" <td>121</td>\n", | |
" <td>119</td>\n", | |
" <td>69</td>\n", | |
" <td>59</td>\n", | |
" <td>71</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Amaura</th>\n", | |
" <td>Rock</td>\n", | |
" <td>Ice</td>\n", | |
" <td>362</td>\n", | |
" <td>77</td>\n", | |
" <td>59</td>\n", | |
" <td>50</td>\n", | |
" <td>67</td>\n", | |
" <td>63</td>\n", | |
" <td>46</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Hawlucha</th>\n", | |
" <td>Fighting</td>\n", | |
" <td>Flying</td>\n", | |
" <td>500</td>\n", | |
" <td>78</td>\n", | |
" <td>92</td>\n", | |
" <td>75</td>\n", | |
" <td>74</td>\n", | |
" <td>63</td>\n", | |
" <td>118</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Dedenne</th>\n", | |
" <td>Electric</td>\n", | |
" <td>Fairy</td>\n", | |
" <td>431</td>\n", | |
" <td>67</td>\n", | |
" <td>58</td>\n", | |
" <td>57</td>\n", | |
" <td>81</td>\n", | |
" <td>67</td>\n", | |
" <td>101</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Goomy</th>\n", | |
" <td>Dragon</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>300</td>\n", | |
" <td>45</td>\n", | |
" <td>50</td>\n", | |
" <td>35</td>\n", | |
" <td>55</td>\n", | |
" <td>75</td>\n", | |
" <td>40</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Sliggoo</th>\n", | |
" <td>Dragon</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>452</td>\n", | |
" <td>68</td>\n", | |
" <td>75</td>\n", | |
" <td>53</td>\n", | |
" <td>83</td>\n", | |
" <td>113</td>\n", | |
" <td>60</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Goodra</th>\n", | |
" <td>Dragon</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>600</td>\n", | |
" <td>90</td>\n", | |
" <td>100</td>\n", | |
" <td>70</td>\n", | |
" <td>110</td>\n", | |
" <td>150</td>\n", | |
" <td>80</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Klefki</th>\n", | |
" <td>Steel</td>\n", | |
" <td>Fairy</td>\n", | |
" <td>470</td>\n", | |
" <td>57</td>\n", | |
" <td>80</td>\n", | |
" <td>91</td>\n", | |
" <td>80</td>\n", | |
" <td>87</td>\n", | |
" <td>75</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Phantump</th>\n", | |
" <td>Ghost</td>\n", | |
" <td>Grass</td>\n", | |
" <td>309</td>\n", | |
" <td>43</td>\n", | |
" <td>70</td>\n", | |
" <td>48</td>\n", | |
" <td>50</td>\n", | |
" <td>60</td>\n", | |
" <td>38</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Trevenant</th>\n", | |
" <td>Ghost</td>\n", | |
" <td>Grass</td>\n", | |
" <td>474</td>\n", | |
" <td>85</td>\n", | |
" <td>110</td>\n", | |
" <td>76</td>\n", | |
" <td>65</td>\n", | |
" <td>82</td>\n", | |
" <td>56</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>PumpkabooAverage Size</th>\n", | |
" <td>Ghost</td>\n", | |
" <td>Grass</td>\n", | |
" <td>335</td>\n", | |
" <td>49</td>\n", | |
" <td>66</td>\n", | |
" <td>70</td>\n", | |
" <td>44</td>\n", | |
" <td>55</td>\n", | |
" <td>51</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>PumpkabooSmall Size</th>\n", | |
" <td>Ghost</td>\n", | |
" <td>Grass</td>\n", | |
" <td>335</td>\n", | |
" <td>44</td>\n", | |
" <td>66</td>\n", | |
" <td>70</td>\n", | |
" <td>44</td>\n", | |
" <td>55</td>\n", | |
" <td>56</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>PumpkabooLarge Size</th>\n", | |
" <td>Ghost</td>\n", | |
" <td>Grass</td>\n", | |
" <td>335</td>\n", | |
" <td>54</td>\n", | |
" <td>66</td>\n", | |
" <td>70</td>\n", | |
" <td>44</td>\n", | |
" <td>55</td>\n", | |
" <td>46</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Bergmite</th>\n", | |
" <td>Ice</td>\n", | |
" <td>Ice</td>\n", | |
" <td>304</td>\n", | |
" <td>55</td>\n", | |
" <td>69</td>\n", | |
" <td>85</td>\n", | |
" <td>32</td>\n", | |
" <td>35</td>\n", | |
" <td>28</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Avalugg</th>\n", | |
" <td>Ice</td>\n", | |
" <td>Ice</td>\n", | |
" <td>514</td>\n", | |
" <td>95</td>\n", | |
" <td>117</td>\n", | |
" <td>184</td>\n", | |
" <td>44</td>\n", | |
" <td>46</td>\n", | |
" <td>28</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Noibat</th>\n", | |
" <td>Flying</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>245</td>\n", | |
" <td>40</td>\n", | |
" <td>30</td>\n", | |
" <td>35</td>\n", | |
" <td>45</td>\n", | |
" <td>40</td>\n", | |
" <td>55</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Noivern</th>\n", | |
" <td>Flying</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>535</td>\n", | |
" <td>85</td>\n", | |
" <td>70</td>\n", | |
" <td>80</td>\n", | |
" <td>97</td>\n", | |
" <td>80</td>\n", | |
" <td>123</td>\n", | |
" <td>6</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Yveltal</th>\n", | |
" <td>Dark</td>\n", | |
" <td>Flying</td>\n", | |
" <td>680</td>\n", | |
" <td>126</td>\n", | |
" <td>131</td>\n", | |
" <td>95</td>\n", | |
" <td>131</td>\n", | |
" <td>98</td>\n", | |
" <td>99</td>\n", | |
" <td>6</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Zygarde50% Forme</th>\n", | |
" <td>Dragon</td>\n", | |
" <td>Ground</td>\n", | |
" <td>600</td>\n", | |
" <td>108</td>\n", | |
" <td>100</td>\n", | |
" <td>121</td>\n", | |
" <td>81</td>\n", | |
" <td>95</td>\n", | |
" <td>95</td>\n", | |
" <td>6</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>HoopaHoopa Confined</th>\n", | |
" <td>Psychic</td>\n", | |
" <td>Ghost</td>\n", | |
" <td>600</td>\n", | |
" <td>80</td>\n", | |
" <td>110</td>\n", | |
" <td>60</td>\n", | |
" <td>150</td>\n", | |
" <td>130</td>\n", | |
" <td>70</td>\n", | |
" <td>6</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>HoopaHoopa Unbound</th>\n", | |
" <td>Psychic</td>\n", | |
" <td>Dark</td>\n", | |
" <td>680</td>\n", | |
" <td>80</td>\n", | |
" <td>160</td>\n", | |
" <td>60</td>\n", | |
" <td>170</td>\n", | |
" <td>130</td>\n", | |
" <td>80</td>\n", | |
" <td>6</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"<p>423 rows × 11 columns</p>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" Type 1 Type 2 Total HP Attack Defense \\\n", | |
"Name \n", | |
"Bulbasaur Grass Poison 318 45 49 49 \n", | |
"Ivysaur Grass Poison 405 60 62 63 \n", | |
"Venusaur Grass Poison 525 80 82 83 \n", | |
"VenusaurMega Venusaur Grass Poison 625 80 100 123 \n", | |
"Charmander Fire Fire 309 39 52 43 \n", | |
"Charmeleon Fire Fire 405 58 64 58 \n", | |
"Charizard Fire Flying 534 78 84 78 \n", | |
"CharizardMega Charizard X Fire Dragon 634 78 130 111 \n", | |
"CharizardMega Charizard Y Fire Flying 634 78 104 78 \n", | |
"Squirtle Water Water 314 44 48 65 \n", | |
"Wartortle Water Water 405 59 63 80 \n", | |
"Blastoise Water Water 530 79 83 100 \n", | |
"BlastoiseMega Blastoise Water Water 630 79 103 120 \n", | |
"Caterpie Bug Bug 195 45 30 35 \n", | |
"Metapod Bug Bug 205 50 20 55 \n", | |
"Butterfree Bug Flying 395 60 45 50 \n", | |
"Weedle Bug Poison 195 40 35 30 \n", | |
"Kakuna Bug Poison 205 45 25 50 \n", | |
"Pidgey Normal Flying 251 40 45 40 \n", | |
"Pidgeotto Normal Flying 349 63 60 55 \n", | |
"Pidgeot Normal Flying 479 83 80 75 \n", | |
"PidgeotMega Pidgeot Normal Flying 579 83 80 80 \n", | |
"Rattata Normal Normal 253 30 56 35 \n", | |
"Ekans Poison Poison 288 35 60 44 \n", | |
"Arbok Poison Poison 438 60 85 69 \n", | |
"Pikachu Electric Electric 320 35 55 40 \n", | |
"Raichu Electric Electric 485 60 90 55 \n", | |
"Sandshrew Ground Ground 300 50 75 85 \n", | |
"Sandslash Ground Ground 450 75 100 110 \n", | |
"Nidoran♀ Poison Poison 275 55 47 52 \n", | |
"... ... ... ... ... ... ... \n", | |
"Binacle Rock Water 306 42 52 67 \n", | |
"Barbaracle Rock Water 500 72 105 115 \n", | |
"Skrelp Poison Water 320 50 60 60 \n", | |
"Dragalge Poison Dragon 494 65 75 90 \n", | |
"Clauncher Water Water 330 50 53 62 \n", | |
"Clawitzer Water Water 500 71 73 88 \n", | |
"Helioptile Electric Normal 289 44 38 33 \n", | |
"Heliolisk Electric Normal 481 62 55 52 \n", | |
"Tyrunt Rock Dragon 362 58 89 77 \n", | |
"Tyrantrum Rock Dragon 521 82 121 119 \n", | |
"Amaura Rock Ice 362 77 59 50 \n", | |
"Hawlucha Fighting Flying 500 78 92 75 \n", | |
"Dedenne Electric Fairy 431 67 58 57 \n", | |
"Goomy Dragon Dragon 300 45 50 35 \n", | |
"Sliggoo Dragon Dragon 452 68 75 53 \n", | |
"Goodra Dragon Dragon 600 90 100 70 \n", | |
"Klefki Steel Fairy 470 57 80 91 \n", | |
"Phantump Ghost Grass 309 43 70 48 \n", | |
"Trevenant Ghost Grass 474 85 110 76 \n", | |
"PumpkabooAverage Size Ghost Grass 335 49 66 70 \n", | |
"PumpkabooSmall Size Ghost Grass 335 44 66 70 \n", | |
"PumpkabooLarge Size Ghost Grass 335 54 66 70 \n", | |
"Bergmite Ice Ice 304 55 69 85 \n", | |
"Avalugg Ice Ice 514 95 117 184 \n", | |
"Noibat Flying Dragon 245 40 30 35 \n", | |
"Noivern Flying Dragon 535 85 70 80 \n", | |
"Yveltal Dark Flying 680 126 131 95 \n", | |
"Zygarde50% Forme Dragon Ground 600 108 100 121 \n", | |
"HoopaHoopa Confined Psychic Ghost 600 80 110 60 \n", | |
"HoopaHoopa Unbound Psychic Dark 680 80 160 60 \n", | |
"\n", | |
" Sp. Atk Sp. Def Speed Generation Legendary \n", | |
"Name \n", | |
"Bulbasaur 65 65 45 1 False \n", | |
"Ivysaur 80 80 60 1 False \n", | |
"Venusaur 100 100 80 1 False \n", | |
"VenusaurMega Venusaur 122 120 80 1 False \n", | |
"Charmander 60 50 65 1 False \n", | |
"Charmeleon 80 65 80 1 False \n", | |
"Charizard 109 85 100 1 False \n", | |
"CharizardMega Charizard X 130 85 100 1 False \n", | |
"CharizardMega Charizard Y 159 115 100 1 False \n", | |
"Squirtle 50 64 43 1 False \n", | |
"Wartortle 65 80 58 1 False \n", | |
"Blastoise 85 105 78 1 False \n", | |
"BlastoiseMega Blastoise 135 115 78 1 False \n", | |
"Caterpie 20 20 45 1 False \n", | |
"Metapod 25 25 30 1 False \n", | |
"Butterfree 90 80 70 1 False \n", | |
"Weedle 20 20 50 1 False \n", | |
"Kakuna 25 25 35 1 False \n", | |
"Pidgey 35 35 56 1 False \n", | |
"Pidgeotto 50 50 71 1 False \n", | |
"Pidgeot 70 70 101 1 False \n", | |
"PidgeotMega Pidgeot 135 80 121 1 False \n", | |
"Rattata 25 35 72 1 False \n", | |
"Ekans 40 54 55 1 False \n", | |
"Arbok 65 79 80 1 False \n", | |
"Pikachu 50 50 90 1 False \n", | |
"Raichu 90 80 110 1 False \n", | |
"Sandshrew 20 30 40 1 False \n", | |
"Sandslash 45 55 65 1 False \n", | |
"Nidoran♀ 40 40 41 1 False \n", | |
"... ... ... ... ... ... \n", | |
"Binacle 39 56 50 6 False \n", | |
"Barbaracle 54 86 68 6 False \n", | |
"Skrelp 60 60 30 6 False \n", | |
"Dragalge 97 123 44 6 False \n", | |
"Clauncher 58 63 44 6 False \n", | |
"Clawitzer 120 89 59 6 False \n", | |
"Helioptile 61 43 70 6 False \n", | |
"Heliolisk 109 94 109 6 False \n", | |
"Tyrunt 45 45 48 6 False \n", | |
"Tyrantrum 69 59 71 6 False \n", | |
"Amaura 67 63 46 6 False \n", | |
"Hawlucha 74 63 118 6 False \n", | |
"Dedenne 81 67 101 6 False \n", | |
"Goomy 55 75 40 6 False \n", | |
"Sliggoo 83 113 60 6 False \n", | |
"Goodra 110 150 80 6 False \n", | |
"Klefki 80 87 75 6 False \n", | |
"Phantump 50 60 38 6 False \n", | |
"Trevenant 65 82 56 6 False \n", | |
"PumpkabooAverage Size 44 55 51 6 False \n", | |
"PumpkabooSmall Size 44 55 56 6 False \n", | |
"PumpkabooLarge Size 44 55 46 6 False \n", | |
"Bergmite 32 35 28 6 False \n", | |
"Avalugg 44 46 28 6 False \n", | |
"Noibat 45 40 55 6 False \n", | |
"Noivern 97 80 123 6 False \n", | |
"Yveltal 131 98 99 6 True \n", | |
"Zygarde50% Forme 81 95 95 6 True \n", | |
"HoopaHoopa Confined 150 130 70 6 True \n", | |
"HoopaHoopa Unbound 170 130 80 6 True \n", | |
"\n", | |
"[423 rows x 11 columns]" | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 99 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "dAdt6lVl8rBM", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Adding a column\n", | |
"* You might have noticed a slight issue with the Mega pokemon names\n", | |
"* Things like **PidgeotMega** or **BlastoiseMega Blastoise**\n", | |
"* If you didn't then look at the output above ^\n", | |
"* Let us create a new row called fixed names\n", | |
"* That converts names like - **BlastoiseMega Blastoise** to just **Mega Blastoise**\n", | |
"* For this we will be using [regex](https://medium.com/factory-mind/regex-tutorial-a-simple-cheatsheet-by-examples-649dc1c3f285) \n" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "gOWqPef86oXo", | |
"colab_type": "code", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 225 | |
}, | |
"outputId": "8a62e19c-7ca8-4cbc-96d8-90ddbaf2611c" | |
}, | |
"cell_type": "code", | |
"source": [ | |
"import re\n", | |
"new_names = []\n", | |
"for name in df.index: # we cannot use df[\"Name\"] cause we converted the Name column into index above\n", | |
" name = re.sub(r'(.*)Mega',\"Mega\", name)\n", | |
" new_names.append(name)\n", | |
"\n", | |
"# Adding the new column\n", | |
"df[\"Fixed Names\"] = new_names\n", | |
"df.head()" | |
], | |
"execution_count": 100, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" <th>Fixed Names</th>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Name</th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" <th></th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>Bulbasaur</th>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>318</td>\n", | |
" <td>45</td>\n", | |
" <td>49</td>\n", | |
" <td>49</td>\n", | |
" <td>65</td>\n", | |
" <td>65</td>\n", | |
" <td>45</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" <td>Bulbasaur</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Ivysaur</th>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>405</td>\n", | |
" <td>60</td>\n", | |
" <td>62</td>\n", | |
" <td>63</td>\n", | |
" <td>80</td>\n", | |
" <td>80</td>\n", | |
" <td>60</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" <td>Ivysaur</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Venusaur</th>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>525</td>\n", | |
" <td>80</td>\n", | |
" <td>82</td>\n", | |
" <td>83</td>\n", | |
" <td>100</td>\n", | |
" <td>100</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" <td>Venusaur</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>VenusaurMega Venusaur</th>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>625</td>\n", | |
" <td>80</td>\n", | |
" <td>100</td>\n", | |
" <td>123</td>\n", | |
" <td>122</td>\n", | |
" <td>120</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" <td>Mega Venusaur</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>Charmander</th>\n", | |
" <td>Fire</td>\n", | |
" <td>Fire</td>\n", | |
" <td>309</td>\n", | |
" <td>39</td>\n", | |
" <td>52</td>\n", | |
" <td>43</td>\n", | |
" <td>60</td>\n", | |
" <td>50</td>\n", | |
" <td>65</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" <td>Charmander</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" Type 1 Type 2 Total HP Attack Defense Sp. Atk \\\n", | |
"Name \n", | |
"Bulbasaur Grass Poison 318 45 49 49 65 \n", | |
"Ivysaur Grass Poison 405 60 62 63 80 \n", | |
"Venusaur Grass Poison 525 80 82 83 100 \n", | |
"VenusaurMega Venusaur Grass Poison 625 80 100 123 122 \n", | |
"Charmander Fire Fire 309 39 52 43 60 \n", | |
"\n", | |
" Sp. Def Speed Generation Legendary Fixed Names \n", | |
"Name \n", | |
"Bulbasaur 65 45 1 False Bulbasaur \n", | |
"Ivysaur 80 60 1 False Ivysaur \n", | |
"Venusaur 100 80 1 False Venusaur \n", | |
"VenusaurMega Venusaur 120 80 1 False Mega Venusaur \n", | |
"Charmander 50 65 1 False Charmander " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 100 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "-2pa6Ws5_xN1", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# [lambda function](https://www.w3schools.com/python/python_lambda.asp)\n", | |
"* Alernatively we can use a lambda function to do the above task\n", | |
"\n", | |
"\n" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "sdsofgxE94HT", | |
"colab_type": "code", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 195 | |
}, | |
"outputId": "41eab102-f217-447f-af19-4c434016791f" | |
}, | |
"cell_type": "code", | |
"source": [ | |
"df.reset_index(inplace=True)\n", | |
"def fix_name(name):\n", | |
" name = re.sub(r'(.*)Mega',\"Mega\", name)\n", | |
" return name\n", | |
" \n", | |
"df[\"Name\"]= df[\"Name\"].apply(fix_name)\n", | |
"df = df.drop([\"Fixed Names\"],axis=1)\n", | |
"df.head()\n" | |
], | |
"execution_count": 101, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>Name</th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>0</th>\n", | |
" <td>Bulbasaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>318</td>\n", | |
" <td>45</td>\n", | |
" <td>49</td>\n", | |
" <td>49</td>\n", | |
" <td>65</td>\n", | |
" <td>65</td>\n", | |
" <td>45</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>1</th>\n", | |
" <td>Ivysaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>405</td>\n", | |
" <td>60</td>\n", | |
" <td>62</td>\n", | |
" <td>63</td>\n", | |
" <td>80</td>\n", | |
" <td>80</td>\n", | |
" <td>60</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>2</th>\n", | |
" <td>Venusaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>525</td>\n", | |
" <td>80</td>\n", | |
" <td>82</td>\n", | |
" <td>83</td>\n", | |
" <td>100</td>\n", | |
" <td>100</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>3</th>\n", | |
" <td>Mega Venusaur</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Poison</td>\n", | |
" <td>625</td>\n", | |
" <td>80</td>\n", | |
" <td>100</td>\n", | |
" <td>123</td>\n", | |
" <td>122</td>\n", | |
" <td>120</td>\n", | |
" <td>80</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>4</th>\n", | |
" <td>Charmander</td>\n", | |
" <td>Fire</td>\n", | |
" <td>Fire</td>\n", | |
" <td>309</td>\n", | |
" <td>39</td>\n", | |
" <td>52</td>\n", | |
" <td>43</td>\n", | |
" <td>60</td>\n", | |
" <td>50</td>\n", | |
" <td>65</td>\n", | |
" <td>1</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" Name Type 1 Type 2 Total HP Attack Defense Sp. Atk Sp. Def \\\n", | |
"0 Bulbasaur Grass Poison 318 45 49 49 65 65 \n", | |
"1 Ivysaur Grass Poison 405 60 62 63 80 80 \n", | |
"2 Venusaur Grass Poison 525 80 82 83 100 100 \n", | |
"3 Mega Venusaur Grass Poison 625 80 100 123 122 120 \n", | |
"4 Charmander Fire Fire 309 39 52 43 60 50 \n", | |
"\n", | |
" Speed Generation Legendary \n", | |
"0 45 1 False \n", | |
"1 60 1 False \n", | |
"2 80 1 False \n", | |
"3 80 1 False \n", | |
"4 65 1 False " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 101 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "GP-f2WY2CxRJ", | |
"colab_type": "code", | |
"colab": {} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"# The above function can be done using a lambda function too\n", | |
"df[\"Name\"]= df[\"Name\"].apply(lambda name:re.sub(r'(.*)Mega',\"Mega\", name))" | |
], | |
"execution_count": 0, | |
"outputs": [] | |
}, | |
{ | |
"metadata": { | |
"id": "qeyIMB1KFrKn", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# sorting\n", | |
"* Let's list out the strongest pokemon" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "vHYF_d5dFcFt", | |
"colab_type": "code", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 195 | |
}, | |
"outputId": "9632fd62-1ea6-4e7f-dba5-63adcf767862" | |
}, | |
"cell_type": "code", | |
"source": [ | |
"pokemon = df.sort_values(by='Total', ascending=False) #sorting the rows in descending order\n", | |
"pokemon.head(5)" | |
], | |
"execution_count": 103, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>Name</th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>426</th>\n", | |
" <td>Mega Rayquaza</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>Flying</td>\n", | |
" <td>780</td>\n", | |
" <td>105</td>\n", | |
" <td>180</td>\n", | |
" <td>100</td>\n", | |
" <td>180</td>\n", | |
" <td>100</td>\n", | |
" <td>115</td>\n", | |
" <td>3</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>164</th>\n", | |
" <td>Mega Mewtwo Y</td>\n", | |
" <td>Psychic</td>\n", | |
" <td>Psychic</td>\n", | |
" <td>780</td>\n", | |
" <td>106</td>\n", | |
" <td>150</td>\n", | |
" <td>70</td>\n", | |
" <td>194</td>\n", | |
" <td>120</td>\n", | |
" <td>140</td>\n", | |
" <td>1</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>163</th>\n", | |
" <td>Mega Mewtwo X</td>\n", | |
" <td>Psychic</td>\n", | |
" <td>Fighting</td>\n", | |
" <td>780</td>\n", | |
" <td>106</td>\n", | |
" <td>190</td>\n", | |
" <td>100</td>\n", | |
" <td>154</td>\n", | |
" <td>100</td>\n", | |
" <td>130</td>\n", | |
" <td>1</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>422</th>\n", | |
" <td>KyogrePrimal Kyogre</td>\n", | |
" <td>Water</td>\n", | |
" <td>Water</td>\n", | |
" <td>770</td>\n", | |
" <td>100</td>\n", | |
" <td>150</td>\n", | |
" <td>90</td>\n", | |
" <td>180</td>\n", | |
" <td>160</td>\n", | |
" <td>90</td>\n", | |
" <td>3</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>424</th>\n", | |
" <td>GroudonPrimal Groudon</td>\n", | |
" <td>Ground</td>\n", | |
" <td>Fire</td>\n", | |
" <td>770</td>\n", | |
" <td>100</td>\n", | |
" <td>180</td>\n", | |
" <td>160</td>\n", | |
" <td>150</td>\n", | |
" <td>90</td>\n", | |
" <td>90</td>\n", | |
" <td>3</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" Name Type 1 Type 2 Total HP Attack Defense \\\n", | |
"426 Mega Rayquaza Dragon Flying 780 105 180 100 \n", | |
"164 Mega Mewtwo Y Psychic Psychic 780 106 150 70 \n", | |
"163 Mega Mewtwo X Psychic Fighting 780 106 190 100 \n", | |
"422 KyogrePrimal Kyogre Water Water 770 100 150 90 \n", | |
"424 GroudonPrimal Groudon Ground Fire 770 100 180 160 \n", | |
"\n", | |
" Sp. Atk Sp. Def Speed Generation Legendary \n", | |
"426 180 100 115 3 True \n", | |
"164 194 120 140 1 True \n", | |
"163 154 100 130 1 True \n", | |
"422 180 160 90 3 True \n", | |
"424 150 90 90 3 True " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 103 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "_5v6Wi5YGdba", | |
"colab_type": "text" | |
}, | |
"cell_type": "markdown", | |
"source": [ | |
"# Strongest pokemon for each type\n", | |
"* Drop all duplicates for each type and keep the top most row" | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "Zlxj2EGeGC1p", | |
"colab_type": "code", | |
"colab": { | |
"base_uri": "https://localhost:8080/", | |
"height": 580 | |
}, | |
"outputId": "a0abb81e-6dd4-4089-dcd7-247923ef5823" | |
}, | |
"cell_type": "code", | |
"source": [ | |
"pokemon = pokemon.drop_duplicates(subset=['Type 1'],keep='first')\n", | |
"pokemon" | |
], | |
"execution_count": 104, | |
"outputs": [ | |
{ | |
"output_type": "execute_result", | |
"data": { | |
"text/html": [ | |
"<div>\n", | |
"<style scoped>\n", | |
" .dataframe tbody tr th:only-of-type {\n", | |
" vertical-align: middle;\n", | |
" }\n", | |
"\n", | |
" .dataframe tbody tr th {\n", | |
" vertical-align: top;\n", | |
" }\n", | |
"\n", | |
" .dataframe thead th {\n", | |
" text-align: right;\n", | |
" }\n", | |
"</style>\n", | |
"<table border=\"1\" class=\"dataframe\">\n", | |
" <thead>\n", | |
" <tr style=\"text-align: right;\">\n", | |
" <th></th>\n", | |
" <th>Name</th>\n", | |
" <th>Type 1</th>\n", | |
" <th>Type 2</th>\n", | |
" <th>Total</th>\n", | |
" <th>HP</th>\n", | |
" <th>Attack</th>\n", | |
" <th>Defense</th>\n", | |
" <th>Sp. Atk</th>\n", | |
" <th>Sp. Def</th>\n", | |
" <th>Speed</th>\n", | |
" <th>Generation</th>\n", | |
" <th>Legendary</th>\n", | |
" </tr>\n", | |
" </thead>\n", | |
" <tbody>\n", | |
" <tr>\n", | |
" <th>426</th>\n", | |
" <td>Mega Rayquaza</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>Flying</td>\n", | |
" <td>780</td>\n", | |
" <td>105</td>\n", | |
" <td>180</td>\n", | |
" <td>100</td>\n", | |
" <td>180</td>\n", | |
" <td>100</td>\n", | |
" <td>115</td>\n", | |
" <td>3</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>164</th>\n", | |
" <td>Mega Mewtwo Y</td>\n", | |
" <td>Psychic</td>\n", | |
" <td>Psychic</td>\n", | |
" <td>780</td>\n", | |
" <td>106</td>\n", | |
" <td>150</td>\n", | |
" <td>70</td>\n", | |
" <td>194</td>\n", | |
" <td>120</td>\n", | |
" <td>140</td>\n", | |
" <td>1</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>422</th>\n", | |
" <td>KyogrePrimal Kyogre</td>\n", | |
" <td>Water</td>\n", | |
" <td>Water</td>\n", | |
" <td>770</td>\n", | |
" <td>100</td>\n", | |
" <td>150</td>\n", | |
" <td>90</td>\n", | |
" <td>180</td>\n", | |
" <td>160</td>\n", | |
" <td>90</td>\n", | |
" <td>3</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>424</th>\n", | |
" <td>GroudonPrimal Groudon</td>\n", | |
" <td>Ground</td>\n", | |
" <td>Fire</td>\n", | |
" <td>770</td>\n", | |
" <td>100</td>\n", | |
" <td>180</td>\n", | |
" <td>160</td>\n", | |
" <td>150</td>\n", | |
" <td>90</td>\n", | |
" <td>90</td>\n", | |
" <td>3</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>552</th>\n", | |
" <td>Arceus</td>\n", | |
" <td>Normal</td>\n", | |
" <td>Normal</td>\n", | |
" <td>720</td>\n", | |
" <td>120</td>\n", | |
" <td>120</td>\n", | |
" <td>120</td>\n", | |
" <td>120</td>\n", | |
" <td>120</td>\n", | |
" <td>120</td>\n", | |
" <td>4</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>413</th>\n", | |
" <td>Mega Metagross</td>\n", | |
" <td>Steel</td>\n", | |
" <td>Psychic</td>\n", | |
" <td>700</td>\n", | |
" <td>80</td>\n", | |
" <td>145</td>\n", | |
" <td>150</td>\n", | |
" <td>105</td>\n", | |
" <td>110</td>\n", | |
" <td>110</td>\n", | |
" <td>3</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>268</th>\n", | |
" <td>Mega Tyranitar</td>\n", | |
" <td>Rock</td>\n", | |
" <td>Dark</td>\n", | |
" <td>700</td>\n", | |
" <td>100</td>\n", | |
" <td>164</td>\n", | |
" <td>150</td>\n", | |
" <td>95</td>\n", | |
" <td>120</td>\n", | |
" <td>71</td>\n", | |
" <td>2</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>545</th>\n", | |
" <td>GiratinaOrigin Forme</td>\n", | |
" <td>Ghost</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>680</td>\n", | |
" <td>150</td>\n", | |
" <td>120</td>\n", | |
" <td>100</td>\n", | |
" <td>120</td>\n", | |
" <td>100</td>\n", | |
" <td>90</td>\n", | |
" <td>4</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>270</th>\n", | |
" <td>Ho-oh</td>\n", | |
" <td>Fire</td>\n", | |
" <td>Flying</td>\n", | |
" <td>680</td>\n", | |
" <td>106</td>\n", | |
" <td>130</td>\n", | |
" <td>90</td>\n", | |
" <td>110</td>\n", | |
" <td>154</td>\n", | |
" <td>90</td>\n", | |
" <td>2</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>792</th>\n", | |
" <td>Xerneas</td>\n", | |
" <td>Fairy</td>\n", | |
" <td>Fairy</td>\n", | |
" <td>680</td>\n", | |
" <td>126</td>\n", | |
" <td>131</td>\n", | |
" <td>95</td>\n", | |
" <td>131</td>\n", | |
" <td>98</td>\n", | |
" <td>99</td>\n", | |
" <td>6</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>793</th>\n", | |
" <td>Yveltal</td>\n", | |
" <td>Dark</td>\n", | |
" <td>Flying</td>\n", | |
" <td>680</td>\n", | |
" <td>126</td>\n", | |
" <td>131</td>\n", | |
" <td>95</td>\n", | |
" <td>131</td>\n", | |
" <td>98</td>\n", | |
" <td>99</td>\n", | |
" <td>6</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>275</th>\n", | |
" <td>Mega Sceptile</td>\n", | |
" <td>Grass</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>630</td>\n", | |
" <td>70</td>\n", | |
" <td>110</td>\n", | |
" <td>75</td>\n", | |
" <td>145</td>\n", | |
" <td>85</td>\n", | |
" <td>145</td>\n", | |
" <td>3</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>498</th>\n", | |
" <td>Mega Lucario</td>\n", | |
" <td>Fighting</td>\n", | |
" <td>Steel</td>\n", | |
" <td>625</td>\n", | |
" <td>70</td>\n", | |
" <td>145</td>\n", | |
" <td>88</td>\n", | |
" <td>140</td>\n", | |
" <td>70</td>\n", | |
" <td>112</td>\n", | |
" <td>4</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>196</th>\n", | |
" <td>Mega Ampharos</td>\n", | |
" <td>Electric</td>\n", | |
" <td>Dragon</td>\n", | |
" <td>610</td>\n", | |
" <td>90</td>\n", | |
" <td>95</td>\n", | |
" <td>105</td>\n", | |
" <td>165</td>\n", | |
" <td>110</td>\n", | |
" <td>45</td>\n", | |
" <td>2</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>229</th>\n", | |
" <td>Mega Scizor</td>\n", | |
" <td>Bug</td>\n", | |
" <td>Steel</td>\n", | |
" <td>600</td>\n", | |
" <td>70</td>\n", | |
" <td>150</td>\n", | |
" <td>140</td>\n", | |
" <td>65</td>\n", | |
" <td>100</td>\n", | |
" <td>75</td>\n", | |
" <td>2</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>397</th>\n", | |
" <td>Mega Glalie</td>\n", | |
" <td>Ice</td>\n", | |
" <td>Ice</td>\n", | |
" <td>580</td>\n", | |
" <td>80</td>\n", | |
" <td>120</td>\n", | |
" <td>80</td>\n", | |
" <td>120</td>\n", | |
" <td>80</td>\n", | |
" <td>100</td>\n", | |
" <td>3</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>703</th>\n", | |
" <td>TornadusTherian Forme</td>\n", | |
" <td>Flying</td>\n", | |
" <td>Flying</td>\n", | |
" <td>580</td>\n", | |
" <td>79</td>\n", | |
" <td>100</td>\n", | |
" <td>80</td>\n", | |
" <td>110</td>\n", | |
" <td>90</td>\n", | |
" <td>121</td>\n", | |
" <td>5</td>\n", | |
" <td>True</td>\n", | |
" </tr>\n", | |
" <tr>\n", | |
" <th>183</th>\n", | |
" <td>Crobat</td>\n", | |
" <td>Poison</td>\n", | |
" <td>Flying</td>\n", | |
" <td>535</td>\n", | |
" <td>85</td>\n", | |
" <td>90</td>\n", | |
" <td>80</td>\n", | |
" <td>70</td>\n", | |
" <td>80</td>\n", | |
" <td>130</td>\n", | |
" <td>2</td>\n", | |
" <td>False</td>\n", | |
" </tr>\n", | |
" </tbody>\n", | |
"</table>\n", | |
"</div>" | |
], | |
"text/plain": [ | |
" Name Type 1 Type 2 Total HP Attack Defense \\\n", | |
"426 Mega Rayquaza Dragon Flying 780 105 180 100 \n", | |
"164 Mega Mewtwo Y Psychic Psychic 780 106 150 70 \n", | |
"422 KyogrePrimal Kyogre Water Water 770 100 150 90 \n", | |
"424 GroudonPrimal Groudon Ground Fire 770 100 180 160 \n", | |
"552 Arceus Normal Normal 720 120 120 120 \n", | |
"413 Mega Metagross Steel Psychic 700 80 145 150 \n", | |
"268 Mega Tyranitar Rock Dark 700 100 164 150 \n", | |
"545 GiratinaOrigin Forme Ghost Dragon 680 150 120 100 \n", | |
"270 Ho-oh Fire Flying 680 106 130 90 \n", | |
"792 Xerneas Fairy Fairy 680 126 131 95 \n", | |
"793 Yveltal Dark Flying 680 126 131 95 \n", | |
"275 Mega Sceptile Grass Dragon 630 70 110 75 \n", | |
"498 Mega Lucario Fighting Steel 625 70 145 88 \n", | |
"196 Mega Ampharos Electric Dragon 610 90 95 105 \n", | |
"229 Mega Scizor Bug Steel 600 70 150 140 \n", | |
"397 Mega Glalie Ice Ice 580 80 120 80 \n", | |
"703 TornadusTherian Forme Flying Flying 580 79 100 80 \n", | |
"183 Crobat Poison Flying 535 85 90 80 \n", | |
"\n", | |
" Sp. Atk Sp. Def Speed Generation Legendary \n", | |
"426 180 100 115 3 True \n", | |
"164 194 120 140 1 True \n", | |
"422 180 160 90 3 True \n", | |
"424 150 90 90 3 True \n", | |
"552 120 120 120 4 True \n", | |
"413 105 110 110 3 False \n", | |
"268 95 120 71 2 False \n", | |
"545 120 100 90 4 True \n", | |
"270 110 154 90 2 True \n", | |
"792 131 98 99 6 True \n", | |
"793 131 98 99 6 True \n", | |
"275 145 85 145 3 False \n", | |
"498 140 70 112 4 False \n", | |
"196 165 110 45 2 False \n", | |
"229 65 100 75 2 False \n", | |
"397 120 80 100 3 False \n", | |
"703 110 90 121 5 True \n", | |
"183 70 80 130 2 False " | |
] | |
}, | |
"metadata": { | |
"tags": [] | |
}, | |
"execution_count": 104 | |
} | |
] | |
}, | |
{ | |
"metadata": { | |
"id": "tuOft-hNGvYr", | |
"colab_type": "code", | |
"colab": {} | |
}, | |
"cell_type": "code", | |
"source": [ | |
"" | |
], | |
"execution_count": 0, | |
"outputs": [] | |
} | |
] | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment