Created
February 14, 2019 18:03
-
-
Save ctrueden/139978877ae1f8cde4c898a409dcadf2 to your computer and use it in GitHub Desktop.
Rendering Markdown dynamically from BeakerX/Groovy
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"cells": [ | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"This notebook demonstrates one way to dynamically render Markdown from Groovy using BeakerX.\n", | |
"\n", | |
"### 1. Use flexmark-java to define a Markdown-to-HTML function" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 1, | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"data": { | |
"application/vnd.jupyter.widget-view+json": { | |
"model_id": "", | |
"version_major": 2, | |
"version_minor": 0 | |
}, | |
"method": "display_data" | |
}, | |
"metadata": {}, | |
"output_type": "display_data" | |
}, | |
{ | |
"data": { | |
"application/vnd.jupyter.widget-view+json": { | |
"model_id": "b9dc0e70-0533-4589-92dc-d981dc11593d", | |
"version_major": 2, | |
"version_minor": 0 | |
}, | |
"method": "display_data" | |
}, | |
"metadata": {}, | |
"output_type": "display_data" | |
}, | |
{ | |
"data": { | |
"text/plain": [ | |
"script1550167287546$_run_closure1@2bbc389" | |
] | |
}, | |
"execution_count": 1, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"%classpath add mvn com.vladsch.flexmark flexmark 0.34.12\n", | |
"\n", | |
"import com.vladsch.flexmark.html.HtmlRenderer\n", | |
"import com.vladsch.flexmark.parser.Parser\n", | |
"\n", | |
"toHtml = { markdown ->\n", | |
" parser = Parser.builder().build()\n", | |
" doc = parser.parse(markdown)\n", | |
" renderer = HtmlRenderer.builder().build()\n", | |
" return renderer.render(doc)\n", | |
"}" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 2, | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/plain": [ | |
"<p>Hello <strong>world</strong>! <em>YEAH</em></p>\n" | |
] | |
}, | |
"execution_count": 2, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"toHtml(\"Hello **world**! _YEAH_\")" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"(Aside: given that BeakerX already offers built-in Markdown cell rendering, it is likely that there is a more elegant and succinct way to do this, without needing a third-party dependency.)" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"### 2. Define an interface for use as a cell output, and bind it to the right behavior, using the jvm-repr layer" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 3, | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/plain": [ | |
"null" | |
] | |
}, | |
"execution_count": 3, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"interface Markdown { String markdown() }\n", | |
"jupyter.Displayers.register(Markdown.class, { md -> [\"text/html\": toHtml(md.markdown()) ] })" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"### 3. Define a sample Markdown block in a Groovy cell" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 4, | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/plain": [ | |
"\n", | |
"# Overview\n", | |
"This is an _introduction_ to tautology.\n", | |
"\n", | |
"# Details\n", | |
"The study of tautology is the study of tautology.\n", | |
"\n", | |
"# Images\n", | |
"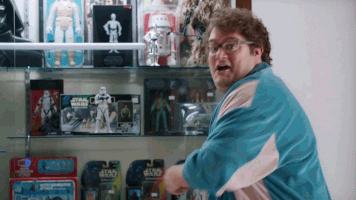\n" | |
] | |
}, | |
"execution_count": 4, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"md = \"\"\"\n", | |
"# Overview\n", | |
"This is an _introduction_ to tautology.\n", | |
"\n", | |
"# Details\n", | |
"The study of tautology is the study of tautology.\n", | |
"\n", | |
"# Images\n", | |
"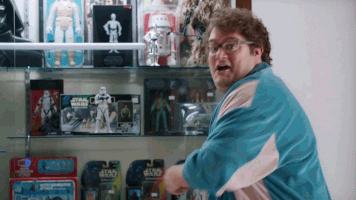\n", | |
"\"\"\"" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"### 4. Create an instance of our Markdown type, and enjoy the fruits of our labors" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 5, | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/html": [ | |
"<h1>Overview</h1>\n", | |
"<p>This is an <em>introduction</em> to tautology.</p>\n", | |
"<h1>Details</h1>\n", | |
"<p>The study of tautology is the study of tautology.</p>\n", | |
"<h1>Images</h1>\n", | |
"<p><img src=\"https://media.giphy.com/media/d2Z9QYzA2aidiWn6/giphy.gif\" alt=\"\" /></p>\n" | |
] | |
}, | |
"execution_count": 5, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"{ -> md } as Markdown" | |
] | |
} | |
], | |
"metadata": { | |
"kernelspec": { | |
"display_name": "Groovy", | |
"language": "groovy", | |
"name": "groovy" | |
}, | |
"language_info": { | |
"codemirror_mode": "groovy", | |
"file_extension": ".groovy", | |
"mimetype": "", | |
"name": "Groovy", | |
"nbconverter_exporter": "", | |
"version": "2.4.3" | |
}, | |
"toc": { | |
"base_numbering": 1, | |
"nav_menu": {}, | |
"number_sections": false, | |
"sideBar": false, | |
"skip_h1_title": false, | |
"title_cell": "Table of Contents", | |
"title_sidebar": "Contents", | |
"toc_cell": false, | |
"toc_position": {}, | |
"toc_section_display": false, | |
"toc_window_display": false | |
} | |
}, | |
"nbformat": 4, | |
"nbformat_minor": 2 | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment