Last active
August 9, 2022 18:23
-
-
Save hugohadfield/9f014e2402122ca928fb1ade3083f04a to your computer and use it in GitHub Desktop.
2D PGA project reject in clifford + pyganja https://enkimute.github.io/ganja.js/examples/coffeeshop.html#pga2d_project_and_reject
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{"cells":[{"metadata":{"trusted":true},"cell_type":"code","source":"from clifford import Cl\nfrom pyganja import *\nimport numpy as np\n\nlayout, blades = Cl(2, 0, 1, firstIdx=0)\nlocals().update(blades)\n\n# for shorter reprs\nlayout.__name__ = 'layout'\nlayout.__module__ = __name__\n\ndef point(x, y):\n return (e0 + x*e1 + y*e2).dual()\n\ndef line(a, b, c):\n return a*e1 + b*e2 + c*e0\n\ndef dist(x, y):\n return abs(x & y)\n\ndef angle(x, y):\n return np.arccos((x|y).value[0])\n\ndef project(a, b):\n return (a|b)/b\n\ndef reject(a, b):\n return (a|b)\n\n\n# Define some points and lines.\nA = point(1, 1)\nB = point(-1, 1)\nC = point(-1, -1)\nAC = A & C","execution_count":1,"outputs":[{"output_type":"stream","text":"/srv/conda/envs/notebook/lib/python3.7/site-packages/pyganja/__init__.py:2: UserWarning: Failed to import cef_gui, cef functions will be unavailable\n from .script_api import *\n","name":"stderr"}]},{"metadata":{"trusted":true},"cell_type":"code","source":"from pyganja.script_api import _to_scene_string\n\ndef generate_notebook_js(scene, sig=None, *,\n default_color=Color.DEFAULT, default_static=False, **kwargs):\n script_json = _to_scene_string(scene, default_color=default_color, default_static=default_static)\n if sig is not None:\n p = (sig > 0).sum().item() # convert to json-compatible scalar\n q = (sig < 0).sum().item() # convert to json-compatible scalar\n r = len(sig) - p - q\n else:\n p = 4\n q = 1\n r = 0\n mv_length = str(2 ** (p + q + r))\n\n # not the best way to test conformal, as it prevents non-euclidean geometry\n if q != 0:\n conformal = True\n else:\n conformal = False\n\n opts = dict(\n p=p, q=q, r=r, mv_length=mv_length,\n graph=dict(\n conformal=conformal,\n gl=True,\n useUnnaturalLineDisplayForPointPairs=True,\n )\n )\n if p - q == 2:\n kwargs['gl'] = False # 2d\n opts[\"graph\"].update(kwargs)\n js = \"\"\"\n // We load ganja.js with requireJS, since `module.exports` / require\n // only work if things are in separate files. In most situations\n // `module` is already undefined, but in VSCode is is not (gh-46).\n // Explicitly clearing it makes ganja.js fall back to RequireJS.\n let module = undefined;\n \"\"\"\n js += read_ganja()\n js += \"\"\"\n // take a closure on element before the next cell replaces it\n (function(element) {\n (requirejs||require)(['Algebra'], function(Algebra) {\n var opts = \"\"\" + json.dumps(opts) + \"\"\"; // injected from python\n var output = Algebra({p: opts.p, q: opts.q, r: opts.r, baseType: Float64Array}).inline((opts)=>{\n var data = \"\"\" + script_json + \"\"\"; // injected from python\n // When we get a file, we load and display.\n var canvas;\n var h=0, p=0;\n // convert arrays of floats back to CGA elements.\n data = data.map(x=>x.length==opts.mv_length?new Element(x):x);\n // add the graph to the page.\n canvas = this.graph(data, opts.graph);\n canvas.options.h = h;\n canvas.options.p = p;\n // make it big.\n canvas.style.width = '100%';\n canvas.style.height = '50vh';\n return canvas;\n })(opts);\n element.append(output);\n var a = document.createElement(\"button\");\n var t = document.createTextNode(\"\\N{FLOPPY DISK} Save\");\n a.appendChild(t);\n function screenshot(){\n //output.width = 1920; output.height = 1080;\n output.update(output.value);\n output.toBlob(function(blob) {\n var url = URL.createObjectURL(blob);\n window.open(url, '_blank');\n });\n }\n window.addEventListener('resize', function() {\n output.update(output.value);\n });\n a.onclick = screenshot\n var butnelem = element.append(a);\n });\n })(element);\n \"\"\"\n return Javascript(js)","execution_count":2,"outputs":[]},{"metadata":{"trusted":true},"cell_type":"code","source":"gs = GanjaScene()\ngs.add_facet([A, B, C], color=0xD0FFE1)\ngs.add_object(project(B, AC), label=\"project B onto AC\", color=0x882288)\ngs.add_object(project(AC, B), label=\"project AC onto B\", color=0x882288)\ngs.add_object(reject(AC, B), label=\"reject AC from B\", color=0x008844)\ngs.add_object(AC, label=\"AC\", color=0x00AA88)\ngs.add_object(A, label=\"A\", color=0x224488)\ngs.add_object(B, label=\"B\", color=0x224488)\ngs.add_object(C, label=\"C\", color=0x224488)\n\ngenerate_notebook_js(gs, sig=layout.sig, gl=False, grid=True, labels=True)","execution_count":3,"outputs":[{"output_type":"execute_result","execution_count":3,"data":{"text/plain":"<IPython.core.display.Javascript object>","application/javascript":"\n // We load ganja.js with requireJS, since `module.exports` / require\n // only work if things are in separate files. In most situations\n // `module` is already undefined, but in VSCode is is not (gh-46).\n // Explicitly clearing it makes ganja.js fall back to RequireJS.\n let module = undefined;\n /** Ganja.js - Geometric Algebra - Not Just Algebra.\n * @author Enki\n * @link https://github.com/enkimute/ganja.js\n */\n\n/*********************************************************************************************************************/\n//\n// Ganja.js is an Algebra generator for javascript. It generates a wide variety of Algebra's and supports operator\n// overloading, algebraic literals and a variety of graphing options.\n//\n// Ganja.js is designed with prototyping and educational purposes in mind. Clean mathematical syntax is the primary\n// target.\n//\n// Ganja.js exports only one function called *Algebra*. This function is used to generate Algebra classes. (say complex\n// numbers, minkowski or 3D CGA). The returned class can be used to create, add, multiply etc, but also to upgrade\n// javascript functions with algebraic literals, operator overloading, vectors, matrices and much more.\n//\n// As a simple example, multiplying two complex numbers 3+2i and 1+4i could be done like this :\n//\n// var complex = Algebra(0,1);\n// var a = new complex([3,2]);\n// var b = new complex([1,3]);\n// var result = a.Mul(b);\n//\n// But the same can be written using operator overloading and algebraic literals. (where scientific notation with\n// lowercase e is overloaded to directly specify generators (e1, e2, e12, ...))\n//\n// var result = Algebra(0,1,()=>(3+2e1)*(1+4e1));\n//\n// Please see github for user documentation and examples.\n//\n/*********************************************************************************************************************/\n\n// Documentation below is for implementors. I'll assume you know about Clifford Algebra's, grades, its products, etc ..\n// I'll also assume you are familiar with ES6. My style may feel a bith mathematical, advise is to read slow.\n\n(function (name, context, definition) {\n if (typeof module != 'undefined' && module.exports) module.exports = definition();\n else if (typeof define == 'function' && define.amd) define(name, definition);\n else context[name] = definition();\n}('Algebra', this, function () {\n\n/** The Algebra class generator. Possible calling signatures :\n * Algebra([func]) => algebra with no dimensions, i.e. R. Optional function for the translator.\n * Algebra(p,[func]) => 'p' positive dimensions and an optional function to pass to the translator.\n * Algebra(p,q,[func]) => 'p' positive and 'q' negative dimensions and optional function.\n * Algebra(p,q,r,[func]) => 'p' positive, 'q' negative and 'r' zero dimensions and optional function.\n * Algebra({ => for custom basis, cayley, mixing, etc pass in an object as first parameter.\n * [p:p], => optional 'p' for # of positive dimensions\n * [q:q], => optional 'q' for # of negative dimensions\n * [r:r], => optional 'r' for # of zero dimensions\n * [metric:array], => alternative for p,q,r. e.g. ([1,1,1,-1] for spacetime)\n * [basis:array], => array of strings with basis names. (e.g. ['1','e1','e2','e12'])\n * [Cayley:Cayley], => optional custom Cayley table (strings). (e.g. [['1','e1'],['e1','-1']])\n * [mix:boolean], => Allows mixing of various algebras. (for space efficiency).\n * [graded:boolean], => Use a graded algebra implementation. (automatic for +6D)\n * [baseType:Float32Array] => optional basetype to use. (only for flat generator)\n * },[func]) => optional function for the translator.\n **/\n return function Algebra(p,q,r) {\n // Resolve possible calling signatures so we know the numbers for p,q,r. Last argument can always be a function.\n var fu=arguments[arguments.length-1],options=p; if (options instanceof Object) {\n q = (p.q || (p.metric && p.metric.filter(x=>x==-1).length))| 0;\n r = (p.r || (p.metric && p.metric.filter(x=>x==0).length)) | 0;\n p = p.p === undefined ? (p.metric && p.metric.filter(x=>x==1).length) || 0 : p.p || 0;\n } else { options={}; p=p|0; r=r|0; q=q|0; };\n\n // Support for multi-dual-algebras\n if (options.dual || (p==0 && q==0 && r<0)) { r=options.dual=options.dual||-r; // Create a dual number algebra if r<0 (old) or options.dual set(new)\n options.basis = [...Array(r+1)].map((a,i)=>i?'e0'+i:'1'); options.metric = [1,...Array(r)]; options.tot=r+1;\n options.Cayley = [...Array(r+1)].map((a,i)=>[...Array(r+1)].map((y,j)=>i*j==0?((i+j)?'e0'+(i+j):'1'):'0'));\n }\n if (options.over) options.baseType = Array;\n\n // Calculate the total number of dimensions.\n var tot = options.tot = (options.tot||(p||0)+(q||0)+(r||0)||(options.basis&&options.basis.length))|0;\n\n // Unless specified, generate a full set of Clifford basis names. We generate them as an array of strings by starting\n // from numbers in binary representation and changing the set bits into their relative position.\n // Basis names are ordered first per grade, then lexically (not cyclic!).\n // For 10 or more dimensions all names will be double digits ! 1e01 instead of 1e1 ..\n var basis=(options.basis&&(options.basis.length==2**tot||r<0||options.Cayley)&&options.basis)||[...Array(2**tot)] // => [undefined, undefined, undefined, undefined, undefined, undefined, undefined, undefined]\n .map((x,xi)=>(((1<<30)+xi).toString(2)).slice(-tot||-1) // => [\"000\", \"001\", \"010\", \"011\", \"100\", \"101\", \"110\", \"111\"] (index of array in base 2)\n .replace(/./g,(a,ai)=>a=='0'?'':String.fromCharCode(66+ai-(r!=0)))) // => [\"\", \"3\", \"2\", \"23\", \"1\", \"13\", \"12\", \"123\"] (1 bits replaced with their positions, 0's removed)\n .sort((a,b)=>(a.toString().length==b.toString().length)?(a>b?1:b>a?-1:0):a.toString().length-b.toString().length) // => [\"\", \"1\", \"2\", \"3\", \"12\", \"13\", \"23\", \"123\"] (sorted numerically)\n .map(x=>x&&'e'+(x.replace(/./g,x=>('0'+(x.charCodeAt(0)-65)).slice(tot>9?-2:-1) ))||'1') // => [\"1\", \"e1\", \"e2\", \"e3\", \"e12\", \"e13\", \"e23\", \"e123\"] (converted to commonly used basis names)\n\n // See if the basis names start from 0 or 1, store grade per component and lowest component per grade.\n var low=basis.length==1?1:basis[1].match(/\\d+/g)[0]*1,\n grades=options.grades||(options.dual&&basis.map((x,i)=>i?1:0))||basis.map(x=>tot>9?(x.length-1)/2:x.length-1),\n grade_start=grades.map((a,b,c)=>c[b-1]!=a?b:-1).filter(x=>x+1).concat([basis.length]);\n\n // String-simplify a concatenation of two basis blades. (and supports custom basis names e.g. e21 instead of e12)\n // This is the function that implements e1e1 = +1/-1/0 and e1e2=-e2e1. The brm function creates the remap dictionary.\n var simplify = (s,p,q,r)=>{\n var sign=1,c,l,t=[],f=true,ss=s.match(tot>9?/(\\d\\d)/g:/(\\d)/g);if (!ss) return s; s=ss; l=s.length;\n while (f) { f=false;\n // implement Ex*Ex = metric.\n for (var i=0; i<l;) if (s[i]===s[i+1]) { if (options.metric) sign*=options.metric[s[i]]; else if ((s[i]-low)>=(p+r)) sign*=-1; else if ((s[i]-low)<r) sign=0;i+=2; f=true; } else t.push(s[i++]);\n // implement Ex*Ey = -Ey*Ex while sorting basis vectors.\n for (var i=0; i<t.length-1; i++) if (t[i]>t[i+1]) { c=t[i];t[i]=t[i+1];t[i+1]=c;sign*=-1;f=true; break;} if (f) { s=t;t=[];l=s.length; }\n }\n var ret=(sign==0)?'0':((sign==1)?'':'-')+(t.length?'e'+t.join(''):'1'); return (brm&&brm[ret])||(brm&&brm['-'+ret]&&'-'+brm['-'+ret])||ret;\n },\n brm=(x=>{ var ret={}; for (var i in basis) ret[basis[i]=='1'?'1':simplify(basis[i],p,q,r)] = basis[i]; return ret; })(basis);\n\n // As an alternative to the string fiddling, one can also bit-fiddle. In this case the basisvectors are represented by integers with 1 bit per generator set.\n var simplify_bits = (A,B,p2)=>{ var n=p2||(p+q+r),t=0,ab=A&B,res=A^B; if (ab&((1<<r)-1)) return [0,0]; while (n--) t^=(A=A>>1); t&=B; t^=ab>>(p+r); t^=t>>16; t^=t>>8; t^=t>>4; return [1-2*(27030>>(t&15)&1),res]; },\n bc = (v)=>{ v=v-((v>>1)& 0x55555555); v=(v&0x33333333)+((v>>2)&0x33333333); c=((v+(v>>4)&0xF0F0F0F)*0x1010101)>>24; return c };\n\n if (!options.graded && tot <= 6 || options.graded===false || options.Cayley) {\n // Faster and degenerate-metric-resistant dualization. (a remapping table that maps items into their duals).\n var drm=basis.map((a,i)=>{ return {a:a,i:i} })\n .sort((a,b)=>a.a.length>b.a.length?1:a.a.length<b.a.length?-1:(+a.a.slice(1).split('').sort().join(''))-(+b.a.slice(1).split('').sort().join('')) )\n .map(x=>x.i).reverse(),\n drms=drm.map((x,i)=>(x==0||i==0)?1:simplify(basis[x]+basis[i])[0]=='-'?-1:1);\n\n /// Store the full metric (also for bivectors etc ..)\n var metric = options.Cayley&&options.Cayley.map((x,i)=>x[i]) || basis.map((x,xi)=>simplify(x+x,p,q,r)|0);\n\n /// Generate multiplication tables for the outer and geometric products.\n var mulTable = options.Cayley||basis.map(x=>basis.map(y=>(x==1)?y:(y==1)?x:simplify(x+y,p,q,r)));\n\n // subalgebra support. (must be bit-order basis blades, does no error checking.)\n if (options.even) options.basis = basis.filter(x=>x.length%2==1);\n if (options.basis && !options.Cayley && r>=0 && options.basis.length != 2**tot) {\n metric = metric.filter((x,i)=>options.basis.indexOf(basis[i])!=-1);\n mulTable = mulTable.filter((x,i)=>options.basis.indexOf(basis[i])!=-1).map(x=>x.filter((x,i)=>options.basis.indexOf(basis[i])!=-1));\n basis = options.basis;\n }\n\n /// Convert Cayley table to product matrices. The outer product selects the strict sum of the GP (but without metric), the inner product\n /// is the left contraction.\n var gp=basis.map(x=>basis.map(x=>'0')), cp=gp.map(x=>gp.map(x=>'0')), cps=gp.map(x=>gp.map(x=>'0')), op=gp.map(x=>gp.map(x=>'0')), gpo={}; // Storage for our product tables.\n basis.forEach((x,xi)=>basis.forEach((y,yi)=>{ var n = mulTable[xi][yi].replace(/^-/,''); if (!gpo[n]) gpo[n]=[]; gpo[n].push([xi,yi]); }));\n basis.forEach((o,oi)=>{\n gpo[o].forEach(([xi,yi])=>op[oi][xi]=(grades[oi]==grades[xi]+grades[yi])?((mulTable[xi][yi]=='0')?'0':((mulTable[xi][yi][0]!='-')?'':'-')+'b['+yi+']*this['+xi+']'):'0');\n gpo[o].forEach(([xi,yi])=>{\n gp[oi][xi] =((gp[oi][xi]=='0')?'':gp[oi][xi]+'+') + ((mulTable[xi][yi]=='0')?'0':((mulTable[xi][yi][0]!='-')?'':'-')+'b['+yi+']*this['+xi+']');\n cp[oi][xi] =((cp[oi][xi]=='0')?'':cp[oi][xi]+'+') + ((grades[oi]==grades[yi]-grades[xi])?gp[oi][xi]:'0');\n cps[oi][xi]=((cps[oi][xi]=='0')?'':cps[oi][xi]+'+') + ((grades[oi]==Math.abs(grades[yi]-grades[xi]))?gp[oi][xi]:'0');\n });\n });\n\n /// Flat Algebra Multivector Base Class.\n var generator = class MultiVector extends (options.baseType||Float32Array) {\n /// constructor - create a floating point array with the correct number of coefficients.\n constructor(a) { super(a||basis.length); return this; }\n\n /// grade selection - return a only the part of the input with the specified grade.\n Grade(grade,res) { res=res||new this.constructor(); for (var i=0,l=res.length; i<l; i++) if (grades[i]==grade) res[i]=this[i]; else res[i]=0; return res; }\n Even(res) { res=res||new this.constructor(); for (var i=0,l=res.length; i<l; i++) if (grades[i]%2==0) res[i]=this[i]; else res[i]=0; return res; }\n\n /// grade creation - convert array with just one grade to full multivector.\n nVector(grade,...args) { this.set(args,grade_start[grade]); return this; }\n\n /// Fill in coordinates (accepts sequence of index,value as arguments)\n Coeff() { for (var i=0,l=arguments.length; i<l; i+=2) this[arguments[i]]=arguments[i+1]; return this; }\n\n /// Negates specific grades (passed in as args)\n Map(res, ...a) { for (var i=0, l=res.length; i<l; i++) res[i] = (~a.indexOf(grades[i]))?-this[i]:this[i]; return res; }\n\n /// Returns the vector grade only.\n get Vector () { return this.slice(grade_start[1],grade_start[2]); };\n\n toString() { var res=[]; for (var i=0; i<basis.length; i++) if (Math.abs(this[i])>1e-10) res.push(((this[i]==1)&&i?'':((this[i]==-1)&&i)?'-':(this[i].toFixed(10)*1))+(i==0?'':tot==1&&q==1?'i':basis[i].replace('e','e_'))); return res.join('+').replace(/\\+-/g,'-')||'0'; }\n\n /// Reversion, Involutions, Conjugation for any number of grades, component acces shortcuts.\n get Negative (){ var res = new this.constructor(); for (var i=0; i<this.length; i++) res[i]= -this[i]; return res; };\n get Reverse (){ var res = new this.constructor(); for (var i=0; i<this.length; i++) res[i]= this[i]*[1,1,-1,-1][grades[i]%4]; return res; };\n get Involute (){ var res = new this.constructor(); for (var i=0; i<this.length; i++) res[i]= this[i]*[1,-1,1,-1][grades[i]%4]; return res; };\n get Conjugate (){ var res = new this.constructor(); for (var i=0; i<this.length; i++) res[i]= this[i]*[1,-1,-1,1][grades[i]%4]; return res; };\n\n /// The Dual, Length, non-metric length and normalized getters.\n get Dual (){ if (r) return this.map((x,i,a)=>a[drm[i]]*drms[i]); var res = new this.constructor(); res[res.length-1]=1; return res.Mul(this); };\n get Length (){ return options.over?Math.sqrt(Math.abs(this.Mul(this.Conjugate).s.s)):Math.sqrt(Math.abs(this.Mul(this.Conjugate).s)); };\n get VLength (){ var res = 0; for (var i=0; i<this.length; i++) res += this[i]*this[i]; return Math.sqrt(res); };\n get Normalized (){ var res = new this.constructor(),l=this.Length; if (!l) return this; l=1/l; for (var i=0; i<this.length; i++) if (options.over) {res[i]=this[i].Scale(l);} else {res[i]=this[i]*l}; return res; };\n }\n\n /// Convert symbolic matrices to code. (skipping zero's on dot and wedge matrices).\n /// These all do straightforward string fiddling. If the 'mix' option is set they reference basis components using e.g. '.e1' instead of eg '[3]' .. so that\n /// it will work for elements of subalgebras etc.\n generator.prototype.Add = new Function('b,res','res=res||new this.constructor();\\n'+basis.map((x,xi)=>'res['+xi+']=b['+xi+']+this['+xi+']').join(';\\n').replace(/(b|this)\\[(.*?)\\]/g,(a,b,c)=>options.mix?'('+b+'.'+(c|0?basis[c]:'s')+'||0)':a)+';\\nreturn res')\n generator.prototype.Scale = new Function('b,res','res=res||new this.constructor();\\n'+basis.map((x,xi)=>'res['+xi+']=b*this['+xi+']').join(';\\n').replace(/(b|this)\\[(.*?)\\]/g,(a,b,c)=>options.mix?'('+b+'.'+(c|0?basis[c]:'s')+'||0)':a)+';\\nreturn res')\n generator.prototype.Sub = new Function('b,res','res=res||new this.constructor();\\n'+basis.map((x,xi)=>'res['+xi+']=this['+xi+']-b['+xi+']').join(';\\n').replace(/(b|this)\\[(.*?)\\]/g,(a,b,c)=>options.mix?'('+b+'.'+(c|0?basis[c]:'s')+'||0)':a)+';\\nreturn res')\n generator.prototype.Mul = new Function('b,res','res=res||new this.constructor();\\n'+gp.map((r,ri)=>'res['+ri+']='+r.join('+').replace(/\\+\\-/g,'-').replace(/(\\w*?)\\[(.*?)\\]/g,(a,b,c)=>options.mix?'('+b+'.'+(c|0?basis[c]:'s')+'||0)':a).replace(/\\+0/g,'')+';').join('\\n')+'\\nreturn res;');\n generator.prototype.LDot = new Function('b,res','res=res||new this.constructor();\\n'+cp.map((r,ri)=>'res['+ri+']='+r.join('+').replace(/\\+\\-/g,'-').replace(/\\+0/g,'').replace(/(\\w*?)\\[(.*?)\\]/g,(a,b,c)=>options.mix?'('+b+'.'+(c|0?basis[c]:'s')+'||0)':a)+';').join('\\n')+'\\nreturn res;');\n generator.prototype.Dot = new Function('b,res','res=res||new this.constructor();\\n'+cps.map((r,ri)=>'res['+ri+']='+r.join('+').replace(/\\+\\-/g,'-').replace(/\\+0/g,'').replace(/(\\w*?)\\[(.*?)\\]/g,(a,b,c)=>options.mix?'('+b+'.'+(c|0?basis[c]:'s')+'||0)':a)+';').join('\\n')+'\\nreturn res;');\n generator.prototype.Wedge = new Function('b,res','res=res||new this.constructor();\\n'+op.map((r,ri)=>'res['+ri+']='+r.join('+').replace(/\\+\\-/g,'-').replace(/\\+0/g,'').replace(/(\\w*?)\\[(.*?)\\]/g,(a,b,c)=>options.mix?'('+b+'.'+(c|0?basis[c]:'s')+'||0)':a)+';').join('\\n')+'\\nreturn res;');\n generator.prototype.Vee = new Function('b,res','res=res||new this.constructor();\\n'+op.map((r,ri)=>'res['+drm[ri]+']='+r.map(x=>x.replace(/\\[(.*?)\\]/g,function(a,b){return '['+(drm[b|0])+']'})).join('+').replace(/\\+\\-/g,'-').replace(/\\+0/g,'').replace(/(\\w*?)\\[(.*?)\\]/g,(a,b,c)=>options.mix?'('+b+'.'+(c|0?basis[c]:'s')+'||0)':a)+';').join('\\n')+'\\nreturn res;');\n\n /// Add getter and setters for the basis vectors/bivectors etc ..\n basis.forEach((b,i)=>Object.defineProperty(generator.prototype, i?b:'s', {\n configurable: true, get(){ return this[i] }, set(x){ this[i]=x; }\n }));\n\n /// Graded generator for high-dimensional algebras.\n } else {\n\n /// extra graded lookups.\n var basisg = grade_start.slice(0,grade_start.length-1).map((x,i)=>basis.slice(x,grade_start[i+1]));\n var counts = grade_start.map((x,i,a)=>i==a.length-1?0:a[i+1]-x).slice(0,tot+1);\n var basis_bits = basis.map(x=>x=='1'?0:x.slice(1).match(tot>9?/\\d\\d/g:/\\d/g).reduce((a,b)=>a+(1<<(b-low)),0)),\n bits_basis = []; basis_bits.forEach((b,i)=>bits_basis[b]=i);\n var metric = basisg.map((x,xi)=>x.map((y,yi)=>simplify_bits(basis_bits[grade_start[xi]+yi],basis_bits[grade_start[xi]+yi])[0]));\n var drms = basisg.map((x,xi)=>x.map((y,yi)=>simplify_bits(basis_bits[grade_start[xi]+yi],(~basis_bits[grade_start[xi]+yi])&((1<<tot)-1))[0]));\n\n /// Flat Algebra Multivector Base Class.\n var generator = class MultiVector extends Array {\n /// constructor - create a floating point array with the correct number of coefficients.\n constructor(a) { super(a||tot); return this; }\n\n /// grade selection - return a only the part of the input with the specified grade.\n Grade(grade,res) { res=new this.constructor(); res[grade] = this[grade]; return res; }\n\n /// grade creation - convert array with just one grade to full multivector.\n nVector(grade,...args) { this[grade]=args; return this; }\n\n /// Fill in coordinates (accepts sequence of index,value as arguments)\n Coeff() {\n for (var i=0,l=arguments.length; i<l; i+=2) if (arguments[i+1]) {\n var gi = grades[arguments[i]];\n if (this[gi]==undefined) this[gi]=[];\n this[gi][arguments[i]-grade_start[gi]]=arguments[i+1];\n }\n return this;\n }\n\n /// Negates specific grades (passed in as args)\n Map(res, ...a) { /* tbc */ }\n\n /// Returns the vector grade only.\n get Vector () { return this[1] };\n\n /// multivector addition, subtraction and scalar multiplication.\n Add(b,r) {\n r=r||new this.constructor();\n for (var i=0,l=Math.max(this.length,b.length);i<l;i++)\n if (!this[i] ^ !b[i]) r[i] = (!this[i]) ? b[i].slice():this[i].slice();\n else if (!(this[i]||b[i])) {}\n else { if (r[i]==undefined) r[i]=[]; for(var j=0,m=Math.max(this[i].length,b[i].length);j<m;j++)\n {\n if (typeof this[i][j]==\"string\" || typeof r[i][j]==\"string\" || typeof b[i][j]==\"string\") {\n if (!this[i][j]) r[i][j] = \"\"+b[i][j];\n else if (!b[i][j]) r[i][j] = \"\"+this[i][j];\n else r[i][j]=\"(\"+(this[i][j]||\"0\")+(b[i][j][0]==\"-\"?\"\":\"+\")+(b[i][j]||\"0\")+\")\";\n } else r[i][j]=(this[i][j]||0)+(b[i][j]||0);\n }}\n return r;\n }\n Sub(b,r) {\n r=r||new this.constructor();\n for (var i=0,l=Math.max(this.length,b.length);i<l;i++)\n if (!this[i] || !b[i]) r[i] = (!this[i]) ? (b[i]?b[i].map(x=>(typeof x==\"string\")?\"-\"+x:-x):undefined):this[i];\n else { if (r[i]==undefined) r[i]=[]; for(var j=0,m=Math.max(this[i].length,b[i].length);j<m;j++)\n if (typeof this[i][j]==\"string\" || typeof r[i][j]==\"string\" || typeof b[i][j]==\"string\") r[i][j]=\"(\"+(this[i][j]||\"0\")+\"-\"+(b[i][j]||\"0\")+\")\";\n else r[i][j]=(this[i][j]||0)-(b[i][j]||0);\n }\n return r;\n }\n Scale(s) { return this.map(x=>x&&x.map(y=>typeof y==\"string\"?y+\"*\"+s:y*s)); }\n\n // geometric product.\n Mul(b,r) {\n r=r||new this.constructor(); var gotstring=false;\n for (var i=0,x,gsx; gsx=grade_start[i],x=this[i],i<this.length; i++) if (x) for (var j=0,y,gsy;gsy=grade_start[j],y=b[j],j<b.length; j++) if (y) for (var a=0; a<x.length; a++) if (x[a]) for (var bb=0; bb<y.length; bb++) if (y[bb]) {\n if (i==j && a==bb) { r[0] = r[0]||(typeof x[0]==\"string\" || typeof y[bb]==\"string\"?[\"\"]:[0]);\n if (typeof x[a]==\"string\" || typeof r[0][0]==\"string\" || typeof y[bb]==\"string\") {\n r[0][0] = (r[0][0]?(r[0][0]+(x[a][0]==\"-\"?\"\":\"+\")):\"\")+ x[a]+\"*\"+y[bb]+(metric[i][a]!=1?\"*\"+metric[i][a]:\"\"); gotstring=true;\n } else r[0][0] += x[a]*y[bb]*metric[i][a];\n } else {\n var rn=simplify_bits(basis_bits[gsx+a],basis_bits[gsy+bb]), g=bc(rn[1]), e=bits_basis[rn[1]]-grade_start[g];\n if (!r[g])r[g]=[];\n if (typeof r[g][e]==\"string\"||typeof x[a]==\"string\"||typeof y[bb]==\"string\") {\n r[g][e] = (r[g][e]?r[g][e]+\"+\":\"\") + (rn[0]!=1?rn[0]+\"*\":\"\")+ x[a]+(y[bb]!=1?\"*\"+y[bb]:\"\"); gotstring=true;\n } else r[g][e] = (r[g][e]||0) + rn[0]*x[a]*y[bb];\n }\n }\n if (gotstring) return r.map(g=>g.map(e=>e&&'('+e+')'))\n return r;\n }\n // outer product.\n Wedge(b,r) {\n r=r||new this.constructor();\n for (var i=0,x,gsx; gsx=grade_start[i],x=this[i],i<this.length; i++) if (x) for (var j=0,y,gsy;gsy=grade_start[j],y=b[j],j<b.length; j++) if (y) for (var a=0; a<x.length; a++) if (x[a]) for (var bb=0; bb<y.length; bb++) if (y[bb]) {\n if (i!=j || a!=bb) {\n var n1=basis_bits[gsx+a], n2=basis_bits[gsy+bb], rn=simplify_bits(n1,n2,tot), g=bc(rn[1]), e=bits_basis[rn[1]]-grade_start[g];\n if (g == i+j) { if (!r[g]) r[g]=[]; r[g][e] = (r[g][e]||0) + rn[0]*x[a]*y[bb]; }\n }\n }\n return r;\n }\n // outer product glsl output.\n OPNS_GLSL(b,point_source) {\n var r='',count=0,curg;\n for (var i=0,x,gsx; gsx=grade_start[i],x=this[i],i<this.length; i++) if (x) for (var j=0,y,gsy;gsy=grade_start[j],y=b[j],j<b.length; j++) if (y) for (var a=0; a<counts[i]; a++) for (var bb=0; bb<counts[j]; bb++) {\n if (i!=j || a!=bb) {\n var n1=basis_bits[gsx+a], n2=basis_bits[gsy+bb], rn=simplify_bits(n1,n2,tot), g=bc(rn[1]), e=bits_basis[rn[1]]-grade_start[g];\n if (g == i+j) { curg=g; r += `res[${e}]${rn[0]=='1'?\"+=\":\"-=\"}(${point_source[a]})*b[${bb}]; //${count++}\\n`; }\n }\n }\n r=r.split('\\n').filter(x=>x).sort((a,b)=>((a.match(/\\d+/)[0]|0)-(b.match(/\\d+/)[0]|0))||((a.match(/\\d+$/)[0]|0)-(b.match(/\\d+$/)[0]|0))).map(x=>x.replace(/\\/\\/\\d+$/,''));\n var r2 = 'float sum=0.0; float res=0.0;\\n', g=0;\n r.forEach(x=>{\n var cg = x.match(/\\d+/)[0]|0;\n if (cg != g) r2 += \"sum \"+(((metric[curg][g]==-1))?\"-=\":\"+=\")+\" res*res;\\nres = 0.0;\\n\";\n r2 += x.replace(/\\[\\d+\\]/,'') + '\\n';\n g=cg;\n });\n r2+= \"sum \"+((metric[curg][g]==-1)?\"-=\":\"+=\")+\" res*res;\\n\";\n return r2;\n }\n // Left contraction.\n LDot(b,r) {\n r=r||new this.constructor();\n for (var i=0,x,gsx; gsx=grade_start[i],x=this[i],i<this.length; i++) if (x) for (var j=0,y,gsy;gsy=grade_start[j],y=b[j],j<b.length; j++) if (y) for (var a=0; a<x.length; a++) if (x[a]) for (var bb=0; bb<y.length; bb++) if (y[bb]) {\n if (i==j && a==bb) { r[0] = r[0]||[0]; r[0][0] += x[a]*y[bb]*metric[i][a]; }\n else {\n var rn=simplify_bits(basis_bits[gsx+a],basis_bits[gsy+bb]), g=bc(rn[1]), e=bits_basis[rn[1]]-grade_start[g];\n if (g == j-i) { if (!r[g])r[g]=[]; r[g][e] = (r[g][e]||0) + rn[0]*x[a]*y[bb]; }\n }\n }\n return r;\n }\n // Symmetric contraction.\n Dot(b,r) {\n r=r||new this.constructor();\n for (var i=0,x,gsx; gsx=grade_start[i],x=this[i],i<this.length; i++) if (x) for (var j=0,y,gsy;gsy=grade_start[j],y=b[j],j<b.length; j++) if (y) for (var a=0; a<x.length; a++) if (x[a]) for (var bb=0; bb<y.length; bb++) if (y[bb]) {\n if (i==j && a==bb) { r[0] = r[0]||[0]; r[0][0] += x[a]*y[bb]*metric[i][a]; }\n else {\n var rn=simplify_bits(basis_bits[gsx+a],basis_bits[gsy+bb]), g=bc(rn[1]), e=bits_basis[rn[1]]-grade_start[g];\n if (g == Math.abs(j-i)) { if (!r[g])r[g]=[]; r[g][e] = (r[g][e]||0) + rn[0]*x[a]*y[bb]; }\n }\n }\n return r;\n }\n // Should be optimized..\n Vee(b,r) { return (this.Dual.Wedge(b.Dual)).Dual; }\n // Output, lengths, involutions, normalized, dual.\n toString() { return [...this].map((g,gi)=>g&&g.map((c,ci)=>!c?undefined:c+basisg[gi][ci]).filter(x=>x).join('+')).filter(x=>x).join('+').replace(/\\+\\-/g,'-'); }\n get s () { if (this[0]) return this[0][0]||0; return 0; }\n get Length () { var res=0; this.forEach((g,gi)=>g&&g.forEach((e,ei)=>res+=(e||0)**2*metric[gi][ei])); return Math.abs(res)**.5; }\n get VLength () { var res=0; this.forEach((g,gi)=>g&&g.forEach((e,ei)=>res+=(e||0)**2)); return Math.abs(res)**.5; }\n get Reverse () { var r=new this.constructor(); this.forEach((x,gi)=>x&&x.forEach((e,ei)=>{if(!r[gi])r[gi]=[]; r[gi][ei] = this[gi][ei]*[1,1,-1,-1][gi%4]; })); return r; }\n get Involute () { var r=new this.constructor(); this.forEach((x,gi)=>x&&x.forEach((e,ei)=>{if(!r[gi])r[gi]=[]; r[gi][ei] = this[gi][ei]*[1,-1,1,-1][gi%4]; })); return r; }\n get Conjugate () { var r=new this.constructor(); this.forEach((x,gi)=>x&&x.forEach((e,ei)=>{if(!r[gi])r[gi]=[]; r[gi][ei] = this[gi][ei]*[1,-1,-1,1][gi%4]; })); return r; }\n get Dual() { var r=new this.constructor(); this.forEach((g,gi)=>{ if (!g) return; r[tot-gi]=[]; g.forEach((e,ei)=>r[tot-gi][counts[gi]-1-ei]=drms[gi][ei]*e); }); return r; }\n get Normalized () { return this.Scale(1/this.Length); }\n }\n\n\n // This generator is UNDER DEVELOPMENT - I'm publishing it so I can test on observable.\n }\n\n // Generate a new class for our algebra. It extends the javascript typed arrays (default float32 but can be specified in options).\n var res = class Element extends generator {\n\n // constructor - create a floating point array with the correct number of coefficients.\n constructor(a) { super(a); if (this.upgrade) this.upgrade(); return this; }\n\n // Grade selection. (implemented by parent class).\n Grade(grade,res) { res=res||new Element(); return super.Grade(grade,res); }\n\n // Right and Left divide - Defined on the elements, shortcuts to multiplying with the inverse.\n Div (b,res) { return this.Mul(b.Inverse,res); }\n LDiv (b,res) { return b.Inverse.Mul(this,res); }\n\n // Taylor exp - for PGA bivectors in 2D and 3D closed form solution is used.\n Exp () {\n if (options.dual) { var f=Math.exp(this.s); return this.map((x,i)=>i?x*f:f); }\n if (r==1 && tot<=4 && Math.abs(this[0])<1E-9 && !options.over) {\n var u = Math.sqrt(Math.abs(this.Dot(this).s)); if (Math.abs(u)<1E-5) return this.Add(Element.Scalar(1));\n var v = this.Wedge(this).Scale(-1/(2*u)); \n var res2 = Element.Add(Element.Sub(Math.cos(u),v.Scale(Math.sin(u))),Element.Div(Element.Mul((Element.Add(Math.sin(u),v.Scale(Math.cos(u)))),this),(Element.Add(u,v))));\n return res2; \n }\n var res = Element.Scalar(1), y=1, M= this.Scale(1), N=this.Scale(1); for (var x=1; x<15; x++) { res=res.Add(M.Scale(1/y)); M=M.Mul(N); y=y*(x+1); }; return res;\n }\n \n // Log - only for up to 3D PGA for now\n Log () {\n if (r!=1 || tot>4 || options.over) return;\n var b = this.Grade(2), bdb = Element.Dot(b,b);\n if (Math.abs(bdb.s)<=1E-5) return this.s<0?b.Scale(-1):b;\n var s = Math.sqrt(-bdb), bwb = Element.Wedge(b,b); \n if (Math.abs(bwb.e0123)<=1E-5) return b.Scale(Math.atan2(s,this.s)/s);\n var p = bwb.Scale(-1/(2*s));\n return Element.Div(Element.Mul(Element.Mul((Element.Add(Math.atan2(s,this.s),Element.Div(p,this.s))),b),(Element.Sub(s,p))),(Element.Mul(s,s))); \n } \n\n // Helper for efficient inverses. (custom involutions - negates grades in arguments).\n Map () { var res=new Element(); return super.Map(res,...arguments); }\n\n // Factories - Make it easy to generate vectors, bivectors, etc when using the functional API. None of the examples use this but\n // users that have used other GA libraries will expect these calls. The Coeff() is used internally when translating algebraic literals.\n static Element() { return new Element([...arguments]); };\n static Coeff() { return (new Element()).Coeff(...arguments); }\n static Scalar(x) { return (new Element()).Coeff(0,x); }\n static Vector() { return (new Element()).nVector(1,...arguments); }\n static Bivector() { return (new Element()).nVector(2,...arguments); }\n static Trivector() { return (new Element()).nVector(3,...arguments); }\n static nVector(n) { return (new Element()).nVector(...arguments); }\n\n // Static operators. The parser will always translate operators to these static calls so that scalars, vectors, matrices and other non-multivectors can also be handled.\n // The static operators typically handle functions and matrices, calling through to element methods for multivectors. They are intended to be flexible and allow as many\n // types of arguments as possible. If performance is a consideration, one should use the generated element methods instead. (which only accept multivector arguments)\n static toEl(x) { if (x instanceof Function) x=x(); if (!(x instanceof Element)) x=Element.Scalar(x); return x; }\n\n // Addition and subtraction. Subtraction with only one parameter is negation.\n static Add(a,b,res) {\n // Resolve expressions passed in.\n while(a.call)a=a(); while(b.call)b=b(); if (a.Add && b.Add) return a.Add(b,res);\n // If either is a string, the result is a string.\n if ((typeof a=='string')||(typeof b=='string')) return a.toString()+b.toString();\n // If only one is an array, add the other element to each of the elements.\n if ((a instanceof Array && !a.Add)^(b instanceof Array && !b.Add)) return (a instanceof Array)?a.map(x=>Element.Add(x,b)):b.map(x=>Element.Add(a,x));\n // If both are equal length arrays, add elements one-by-one\n if ((a instanceof Array)&&(b instanceof Array)&&a.length==b.length) return a.map((x,xi)=>Element.Add(x,b[xi]));\n // If they're both not elements let javascript resolve it.\n if (!(a instanceof Element || b instanceof Element)) return a+b;\n // Here we're left with scalars and multivectors, call through to generated code.\n a=Element.toEl(a); b=Element.toEl(b); return a.Add(b,res);\n }\n\n static Sub(a,b,res) {\n // Resolve expressions passed in.\n while(a.call)a=a(); while(b&&b.call) b=b(); if (a.Sub && b && b.Sub) return a.Sub(b,res);\n // If only one is an array, add the other element to each of the elements.\n if (b&&((a instanceof Array)^(b instanceof Array))) return (a instanceof Array)?a.map(x=>Element.Sub(x,b)):b.map(x=>Element.Sub(a,x));\n // If both are equal length arrays, add elements one-by-one\n if (b&&(a instanceof Array)&&(b instanceof Array)&&a.length==b.length) return a.map((x,xi)=>Element.Sub(x,b[xi]));\n // Negation\n if (arguments.length==1) return Element.Mul(a,-1);\n // If none are elements here, let js do it.\n if (!(a instanceof Element || b instanceof Element)) return a-b;\n // Here we're left with scalars and multivectors, call through to generated code.\n a=Element.toEl(a); b=Element.toEl(b); return a.Sub(b,res);\n }\n\n // The geometric product. (or matrix*matrix, matrix*vector, vector*vector product if called with 1D and 2D arrays)\n static Mul(a,b,res) {\n // Resolve expressions\n while(a.call&&!a.length)a=a(); while(b.call&&!b.length)b=b(); if (a.Mul && b.Mul) return a.Mul(b,res);\n // still functions -> experimental curry style (dont use this.)\n if (a.call && b.call) return (ai,bi)=>Element.Mul(a(ai),b(bi));\n // scalar mul.\n if (Number.isFinite(a) && b.Scale) return b.Scale(a); else if (Number.isFinite(b) && a.Scale) return a.Scale(b);\n // Handle matrices and vectors.\n if ((a instanceof Array)&&(b instanceof Array)) {\n // vector times vector performs a dot product. (which internally uses the GP on each component)\n if((!(a[0] instanceof Array) || (a[0] instanceof Element)) &&(!(b[0] instanceof Array) || (b[0] instanceof Element))) { var r=tot?Element.Scalar(0):0; a.forEach((x,i)=>r=Element.Add(r,Element.Mul(x,b[i]),r)); return r; }\n // Array times vector\n if(!(b[0] instanceof Array)) return a.map((x,i)=>Element.Mul(a[i],b));\n // Array times Array\n var r=a.map((x,i)=>b[0].map((y,j)=>{ var r=tot?Element.Scalar(0):0; x.forEach((xa,k)=>r=Element.Add(r,Element.Mul(xa,b[k][j]))); return r; }));\n // Return resulting array or scalar if 1 by 1.\n if (r.length==1 && r[0].length==1) return r[0][0]; else return r;\n }\n // Only one is an array multiply each of its elements with the other.\n if ((a instanceof Array)^(b instanceof Array)) return (a instanceof Array)?a.map(x=>Element.Mul(x,b)):b.map(x=>Element.Mul(a,x));\n // Try js multiplication, else call through to geometric product.\n var r=a*b; if (!isNaN(r)) return r;\n a=Element.toEl(a); b=Element.toEl(b); return a.Mul(b,res);\n }\n\n // The inner product. (default is left contraction).\n static LDot(a,b,res) {\n // Expressions\n while(a.call)a=a(); while(b.call)b=b(); //if (a.LDot) return a.LDot(b,res);\n // Map elements in array\n if (b instanceof Array && !(a instanceof Array)) return b.map(x=>Element.LDot(a,x));\n if (a instanceof Array && !(b instanceof Array)) return a.map(x=>Element.LDot(x,b));\n // js if numbers, else contraction product.\n if (!(a instanceof Element || b instanceof Element)) return a*b;\n a=Element.toEl(a);b=Element.toEl(b); return a.LDot(b,res);\n }\n\n // The symmetric inner product. (default is left contraction).\n static Dot(a,b,res) {\n // Expressions\n while(a.call)a=a(); while(b.call)b=b(); //if (a.LDot) return a.LDot(b,res);\n // js if numbers, else contraction product.\n if (!(a instanceof Element || b instanceof Element)) return a|b;\n a=Element.toEl(a);b=Element.toEl(b); return a.Dot(b,res);\n }\n\n // The outer product. (Grassman product - no use of metric)\n static Wedge(a,b,res) {\n // Expressions\n while(a.call)a=a(); while(b.call)b=b(); if (a.Wedge) return a.Wedge(Element.toEl(b),res);\n // The outer product of two vectors is a matrix .. internally Mul not Wedge !\n if (a instanceof Array && b instanceof Array) return a.map(xa=>b.map(xb=>Element.Mul(xa,xb)));\n // js, else generated wedge product.\n if (!(a instanceof Element || b instanceof Element)) return a*b;\n a=Element.toEl(a);b=Element.toEl(b); return a.Wedge(b,res);\n }\n\n // The regressive product. (Dual of the outer product of the duals).\n static Vee(a,b,res) {\n // Expressions\n while(a.call)a=a(); while(b.call)b=b(); if (a.Vee) return a.Vee(Element.toEl(b),res);\n // js, else generated vee product. (shortcut for dual of wedge of duals)\n if (!(a instanceof Element || b instanceof Element)) return 0;\n a=Element.toEl(a);b=Element.toEl(b); return a.Vee(b,res);\n }\n\n // The sandwich product. Provided for convenience (>>> operator)\n static sw(a,b) {\n // Expressions\n while(a.call)a=a(); while(b.call)b=b(); if (a.sw) return a.sw(b);\n // Map elements in array\n if (b instanceof Array && !b.Add) return b.map(x=>Element.sw(a,x));\n // Call through. no specific generated code for it so just perform the muls.\n a=Element.toEl(a); b=Element.toEl(b); return a.Mul(b).Mul(a.Reverse);\n }\n\n // Division - scalars or cal through to element method.\n static Div(a,b,res) {\n // Expressions\n while(a.call)a=a(); while(b.call)b=b();\n // js or call through to element divide.\n if (!(a instanceof Element || b instanceof Element)) return a/b;\n a=Element.toEl(a);\n if (Number.isFinite(b)) { return a.Scale(1/b,res); }\n b=Element.toEl(b); return a.Div(b,res);\n }\n\n // Pow - needs obvious extensions for natural powers. (exponentiation by squaring)\n static Pow(a,b,res) {\n // Expressions\n while(a.call)a=a(); while(b.call)b=b(); if (a.Pow) return a.Pow(b,res);\n // Exponentiation.\n if (a===Math.E && b.Exp) return b.Exp();\n // Squaring\n if (b===2) return this.Mul(a,a,res);\n // No elements, call through to js\n if (!(a instanceof Element || b instanceof Element)) return a**b;\n // Inverse\n if (b===-1) return a.Inverse;\n // Call through to element pow.\n a=Element.toEl(a); return a.Pow(b);\n }\n\n // Handles scalars and calls through to element method.\n static exp(a) {\n // Expressions.\n while(a.call)a=a();\n // If it has an exp callthrough, use it, else call through to math.\n if (a.Exp) return a.Exp();\n return Math.exp(a);\n }\n\n // Dual, Involute, Reverse, Conjugate, Normalize and length, all direct call through. Conjugate handles matrices.\n static Dual(a) { return Element.toEl(a).Dual; };\n static Involute(a) { return Element.toEl(a).Involute; };\n static Reverse(a) { return Element.toEl(a).Reverse; };\n static Conjugate(a) { if (a.Conjugate) return a.Conjugate; if (a instanceof Array) return a[0].map((c,ci)=>a.map((r,ri)=>Element.Conjugate(a[ri][ci]))); return Element.toEl(a).Conjugate; }\n static Normalize(a) { return Element.toEl(a).Normalized; };\n static Length(a) { return Element.toEl(a).Length };\n\n // Comparison operators always use length. Handle expressions, then js or length comparison\n static eq(a,b) { if (!(a instanceof Element)||!(b instanceof Element)) return a==b; while(a.call)a=a(); while(b.call)b=b(); for (var i=0; i<a.length; i++) if (a[i]!=b[i]) return false; return true; }\n static neq(a,b) { if (!(a instanceof Element)||!(b instanceof Element)) return a!=b; while(a.call)a=a(); while(b.call)b=b(); for (var i=0; i<a.length; i++) if (a[i]!=b[i]) return true; return false; }\n static lt(a,b) { while(a.call)a=a(); while(b.call)b=b(); return (a instanceof Element?a.Length:a)<(b instanceof Element?b.Length:b); }\n static gt(a,b) { while(a.call)a=a(); while(b.call)b=b(); return (a instanceof Element?a.Length:a)>(b instanceof Element?b.Length:b); }\n static lte(a,b) { while(a.call)a=a(); while(b.call)b=b(); return (a instanceof Element?a.Length:a)<=(b instanceof Element?b.Length:b); }\n static gte(a,b) { while(a.call)a=a(); while(b.call)b=b(); return (a instanceof Element?a.Length:a)>=(b instanceof Element?b.Length:b); }\n\n // Debug output and printing multivectors.\n static describe(x) { if (x===true) console.log(`Basis\\n${basis}\\nMetric\\n${metric.slice(1,1+tot)}\\nCayley\\n${mulTable.map(x=>(x.map(x=>(' '+x).slice(-2-tot)))).join('\\n')}\\nMatrix Form:\\n`+gp.map(x=>x.map(x=>x.match(/(-*b\\[\\d+\\])/)).map(x=>x&&((x[1].match(/-/)||' ')+String.fromCharCode(65+1*x[1].match(/\\d+/)))||' 0')).join('\\n')); return {basis:basisg||basis,metric,mulTable} }\n\n // Direct sum of algebras - experimental\n static sum(B){\n var A = Element;\n // Get the multiplication tabe and basis.\n var T1 = A.describe().mulTable, T2 = B.describe().mulTable;\n var B1 = A.describe().basis, B2 = B.describe().basis;\n // Get the maximum index of T1, minimum of T2 and rename T2 if needed.\n var max_T1 = B1.filter(x=>x.match(/e/)).map(x=>x.match(/\\d/g)).flat().map(x=>x|0).sort((a,b)=>b-a)[0];\n var max_T2 = B2.filter(x=>x.match(/e/)).map(x=>x.match(/\\d/g)).flat().map(x=>x|0).sort((a,b)=>b-a)[0];\n var min_T2 = B2.filter(x=>x.match(/e/)).map(x=>x.match(/\\d/g)).flat().map(x=>x|0).sort((a,b)=>a-b)[0];\n // remapping ..\n T2 = T2.map(x=>x.map(y=>y.match(/e/)?y.replace(/(\\d)/g,(x)=>(x|0)+max_T1):y.replace(\"1\",\"e\"+(1+max_T2+max_T1))));\n B2 = B2.map((y,i)=>i==0?y.replace(\"1\",\"e\"+(1+max_T2+max_T1)):y.replace(/(\\d)/g,(x)=>(x|0)+max_T1));\n // Build the new basis and multable..\n var basis = [...B1,...B2];\n var Cayley = T1.map((x,i)=>[...x,...T2[0].map(x=>\"0\")]).concat(T2.map((x,i)=>[...T1[0].map(x=>\"0\"),...x]))\n // Build the new algebra.\n var grades = [...B1.map(x=>x==\"1\"?0:x.length-1),...B2.map((x,i)=>i?x.length-1:0)];\n var a = Algebra({basis,Cayley,grades,tot:Math.log2(B1.length)+Math.log2(B2.length)})\n // And patch up ..\n a.Scalar = function(x) {\n var res = new a();\n for (var i=0; i<res.length; i++) res[i] = basis[i] == Cayley[i][i] ? x:0;\n return res;\n }\n return a;\n }\n\n // The graphing function supports several modes. It can render 1D functions and 2D functions on canvas, and PGA2D, PGA3D and CGA2D functions using SVG.\n // It handles animation and interactivity.\n // graph(function(x)) => function of 1 parameter will be called with that parameter from -1 to 1 and graphed on a canvas. Returned values should also be in the [-1 1] range\n // graph(function(x,y)) => functions of 2 parameters will be called from -1 to 1 on both arguments. Returned values can be 0-1 for greyscale or an array of three RGB values.\n // graph(array) => array of algebraic elements (points, lines, circles, segments, texts, colors, ..) is graphed.\n // graph(function=>array) => same as above, for animation scenario's this function is called each frame.\n // An optional second parameter is an options object { width, height, animate, camera, scale, grid, canvas }\n static graph(f,options) {\n // Store the original input\n if (!f) return; var origf=f;\n // generate default options.\n options=options||{}; options.scale=options.scale||1; options.camera=options.camera||(tot<4?Element.Scalar(1):new Element([0.7071067690849304, 0, 0, 0, 0, 0, 0, 0, 0, 0.7071067690849304, 0, 0, 0, 0, 0, 0]));\n if (options.conformal && tot==4) var ni = options.ni||this.Coeff(4,1,3,1), no = options.no||this.Coeff(4,0.5,3,-0.5), minus_no = no.Scale(-1);\n var ww=options.width, hh=options.height, cvs=options.canvas, tpcam=new Element([0,0,0,0,0,0,0,0,0,0,0,-5,0,0,1,0]),tpy=this.Coeff(4,1),tp=new Element(),\n // project 3D to 2D. This allows to render 3D and 2D PGA with the same code.\n project=(o)=>{ if (!o) return o; while (o.call) o=o(); return (tot==4 && (o.length==16))?(tpcam).Vee(options.camera.Mul(o).Mul(options.camera.Conjugate)).Wedge(tpy):(o.length==2**tot)?Element.sw(options.camera,o):o;};\n // gl escape.\n if (options.gl && !(tot==4 && options.conformal)) return Element.graphGL(f,options); if (options.up) return Element.graphGL2(f,options);\n // if we get an array or function without parameters, we render c2d or p2d SVG points/lines/circles/etc\n if (!(f instanceof Function) || f.length===0) {\n // Our current cursor, color, animation state and 2D mapping.\n var lx,ly,lr,color,res,anim=false,to2d=(tot==3)?[0,1,2,3,4,5,6,7]:[0,7,9,10,13,12,14,15];\n // Make sure we have an array of elements. (if its an object, convert to array with elements and names.)\n if (f instanceof Function) f=f(); if (!(f instanceof Array)) f=[].concat.apply([],Object.keys(f).map((k)=>typeof f[k]=='number'?[f[k]]:[f[k],k]));\n // The build function generates the actual SVG. It will be called everytime the user interacts or the anim flag is set.\n function build(f,or) {\n // Make sure we have an aray.\n if (or && f && f instanceof Function) f=f();\n // Reset position and color for cursor.\n lx=-2;ly=-1.85;lr=0;color='#444';\n // Create the svg element. (master template string till end of function)\n var svg=new DOMParser().parseFromString(`<SVG onmousedown=\"if(evt.target==this)this.sel=undefined\" viewBox=\"-2 -${2*(hh/ww||1)} 4 ${4*(hh/ww||1)}\" style=\"width:${ww||512}px; height:${hh||512}px; background-color:#eee; -webkit-user-select:none; -moz-user-select:none; -ms-user-select:none; user-select:none\">\n // Add a grid (option)\n ${options.grid?(()=>{\n var n = Math.floor(10 / options.scale);\n return n>50?'':[...Array(2*n + 1)].map((x,xi)=>`<line x1=\"-10\" y1=\"${((xi-n)/2-(tot<4?2*options.camera.e02:0))*options.scale}\" x2=\"10\" y2=\"${((xi-n)/2-(tot<4?2*options.camera.e02:0))*options.scale}\" stroke-width=\"0.005\" stroke=\"#CCC\"/><line y1=\"-10\" x1=\"${((xi-n)/2-(tot<4?2*options.camera.e01:0))*options.scale}\" y2=\"10\" x2=\"${((xi-n)/2-(tot<4?2*options.camera.e01:0))*options.scale}\" stroke-width=\"0.005\" stroke=\"#CCC\"/>`);\n })():''}\n // Handle conformal 2D elements.\n ${options.conformal?f.map&&f.map((o,oidx)=>{\n // Optional animation handling.\n if((o==Element.graph && or!==false)||(oidx==0&&options.animate&&or!==false)) { anim=true; requestAnimationFrame(()=>{var r=build(origf,(!res)||(document.body.contains(res))).innerHTML; if (res) res.innerHTML=r; }); if (!options.animate) return; }\n // Resolve expressions passed in.\n while (o.call) o=o();\n if (options.ipns && o instanceof Element) o = o.Dual;\n var sc = options.scale;\n var lineWidth = options.lineWidth || 1;\n var pointRadius = options.pointRadius || 1;\n var dash_for_r2 = (r2, render_r, target_width) => {\n // imaginary circles are dotted\n if (r2 >= 0) return 'none';\n var half_circum = render_r*Math.PI;\n var width = half_circum / Math.max(Math.round(half_circum / target_width), 1);\n return `${width} ${width}`;\n };\n // Arrays are rendered as segments or polygons. (2 or more elements)\n if (o instanceof Array) { lx=ly=lr=0; o=o.map(o=>{ while(o.call)o=o(); return o.Scale(-1/o.Dot(ni).s); }); o.forEach((o)=>{lx+=sc*(o.e1);ly+=sc*(-o.e2)});lx/=o.length;ly/=o.length; return o.length>2?`<POLYGON STYLE=\"pointer-events:none; fill:${color};opacity:0.7\" points=\"${o.map(o=>(sc*o.e1+','+(-o.e2*sc)+' '))}\"/>`:`<LINE style=\"pointer-events:none\" x1=${o[0].e1*sc} y1=${-o[0].e2*sc} x2=${o[1].e1*sc} y2=${-o[1].e2*sc} stroke-width=\"${lineWidth*0.005}\" stroke=\"${color||'#888'}\"/>`; }\n // Strings are rendered at the current cursor position.\n if (typeof o =='string') { var res2=(o[0]=='_')?'':`<text x=\"${lx}\" y=\"${ly}\" font-family=\"Verdana\" font-size=\"${options.fontSize*0.1||0.1}\" style=\"pointer-events:none\" fill=\"${color||'#333'}\" transform=\"rotate(${lr},${lx},${ly})\"> ${o} </text>`; ly+=0.14; return res2; }\n // Numbers change the current color.\n if (typeof o =='number') { color='#'+(o+(1<<25)).toString(16).slice(-6); return ''; };\n // All other elements are rendered ..\n var ni_part = o.Dot(no.Scale(-1)); // O_i + n_o O_oi\n var no_part = ni.Scale(-1).Dot(o); // O_o + O_oi n_i\n if (ni_part.VLength * 1e-6 > no_part.VLength) {\n // direction or dual - nothing to render\n return \"\";\n }\n var no_ni_part = no_part.Dot(no.Scale(-1)); // O_oi\n var no_only_part = ni.Wedge(no_part).Dot(no.Scale(-1)); // O_o\n\n /* Note: making 1e-6 smaller increases the maximum circle radius before they are drawn as lines */\n if (no_ni_part.VLength * 1e-6 > no_only_part.VLength) {\n var is_flat = true;\n var direction = no_ni_part;\n }\n else {\n var is_flat = false;\n var direction = no_only_part;\n }\n // normalize to make the direction unitary\n var dl = direction.Length;\n o = o.Scale(1/dl);\n direction = direction.Scale(1/dl)\n\n var b0=direction.Grade(0).VLength>0.001,b1=direction.Grade(1).VLength>0.001,b2=direction.Grade(2).VLength>0.001;\n if (!is_flat && b0 && !b1 && !b2) {\n // Points\n if (direction.s < 0) { o = Element.Sub(o); }\n lx=sc*(o.e1); ly=sc*(-o.e2); lr=0; return res2=`<CIRCLE onmousedown=\"this.parentElement.sel=${oidx}\" cx=\"${lx}\" cy=\"${ly}\" r=\"${pointRadius*0.03}\" fill=\"${color||'green'}\"/>`;\n } else if (is_flat && !b0 && b1 && !b2) {\n // Lines.\n var loc=minus_no.LDot(o).Div(o), att=ni.Dot(o);\n lx=sc*(-loc.e1); ly=sc*(loc.e2); lr=Math.atan2(-o[14],o[13])/Math.PI*180; return `<LINE style=\"pointer-events:none\" x1=${lx-10} y1=${ly} x2=${lx+10} y2=${ly} stroke-width=\"${lineWidth*0.005}\" stroke=\"${color||'#888'}\" transform=\"rotate(${lr},${lx},${ly})\"/>`;\n } else if (!is_flat && !b0 && !b1 && b2) {\n // Circles\n var loc=o.Div(ni.LDot(o)); lx=sc*(-loc.e1); ly=sc*(loc.e2);\n var r2=o.Mul(o.Conjugate).s;\n var r = Math.sqrt(Math.abs(r2))*sc;\n return `<CIRCLE onmousedown=\"this.parentElement.sel=${oidx}\" cx=\"${lx}\" cy=\"${ly}\" r=\"${r}\" stroke-width=\"${lineWidth*0.005}\" fill=\"none\" stroke=\"${color||'green'}\" stroke-dasharray=\"${dash_for_r2(r2, r, lineWidth*0.020)}\"/>`;\n } else if (!is_flat && !b0 && b1 && !b2) {\n // Point Pairs.\n lr=0; var ei=ni,eo=no, nix=o.Wedge(ei), sqr=o.LDot(o).s/nix.LDot(nix).s, r=Math.sqrt(Math.abs(sqr)), attitude=((ei.Wedge(eo)).LDot(nix)).Normalized.Mul(Element.Scalar(r)), pos=o.Div(nix); pos=pos.Div( pos.LDot(Element.Sub(ei)));\n if (nix==0) { pos = o.Dot(Element.Coeff(4,-1)); sqr=-1; }\n lx=sc*(pos.e1); ly=sc*(-pos.e2);\n if (sqr==0) return `<CIRCLE onmousedown=\"this.parentElement.sel=${oidx}\" cx=\"${lx}\" cy=\"${ly}\" r=\"${pointRadius*0.03}\" stroke-width=\"${lineWidth*0.01}\" fill=\"none\" stroke=\"${color||'green'}\"/>`;\n // Draw imaginary pairs hollow\n if (sqr > 0) var fill = color||'green', stroke = 'none', dash_array = 'none';\n else var fill = 'none', stroke = color||'green';\n lx=sc*(pos.e1+attitude.e1); ly=sc*(-pos.e2-attitude.e2);\n var res2=`<CIRCLE onmousedown=\"this.parentElement.sel=${oidx}\" cx=\"${lx}\" cy=\"${ly}\" r=\"${pointRadius*0.03}\" fill=\"${fill}\" stroke-width=\"${lineWidth*0.01}\" stroke=\"${stroke}\" stroke-dasharray=\"${dash_for_r2(sqr, pointRadius*0.03, lineWidth*0.020)}\" />`;\n lx=sc*(pos.e1-attitude.e1); ly=sc*(-pos.e2+attitude.e2);\n return res2+`<CIRCLE onmousedown=\"this.parentElement.sel=${oidx}\" cx=\"${lx}\" cy=\"${ly}\" r=\"${pointRadius*0.03}\" fill=\"${fill}\" stroke-width=\"${lineWidth*0.01}\" stroke=\"${stroke}\" stroke-dasharray=\"${dash_for_r2(sqr, pointRadius*0.03, lineWidth*0.020)}\" />`;\n } else {\n /* Unrecognized */\n return \"\";\n }\n // Handle projective 2D and 3D elements.\n }):f.map&&f.map((o,oidx)=>{ if((o==Element.graph && or!==false)||(oidx==0&&options.animate&&or!==false)) { anim=true; requestAnimationFrame(()=>{var r=build(origf,(!res)||(document.body.contains(res))).innerHTML; if (res) res.innerHTML=r; }); if (!options.animate) return; } while (o instanceof Function) o=o(); o=(o instanceof Array)?o.map(project):project(o); if (o===undefined) return;\n // dual option dualizes before render\n if (options.dual && o instanceof Element) o = o.Dual;\n // line segments and polygons\n if (o instanceof Array && o.length) { lx=ly=lr=0; o.forEach((o)=>{while (o.call) o=o(); lx+=options.scale*((drm[1]==6||drm[1]==14)?-1:1)*o[drm[2]]/o[drm[1]];ly+=options.scale*o[drm[3]]/o[drm[1]]});lx/=o.length;ly/=o.length; return o.length>2?`<POLYGON STYLE=\"pointer-events:none; fill:${color};opacity:0.7\" points=\"${o.map(o=>((drm[1]==6||drm[1]==14)?-1:1)*options.scale*o[drm[2]]/o[drm[1]]+','+options.scale*o[drm[3]]/o[drm[1]]+' ')}\"/>`:`<LINE style=\"pointer-events:none\" x1=${options.scale*((drm[1]==6||drm[1]==14)?-1:1)*o[0][drm[2]]/o[0][drm[1]]} y1=${options.scale*o[0][drm[3]]/o[0][drm[1]]} x2=${options.scale*((drm[1]==6||drm[1]==14)?-1:1)*o[1][drm[2]]/o[1][drm[1]]} y2=${options.scale*o[1][drm[3]]/o[1][drm[1]]} stroke-width=\"${options.lineWidth*0.005||0.005}\" stroke=\"${color||'#888'}\"/>`; }\n // svg\n if (typeof o =='string' && o[0]=='<') { return o; }\n // Labels\n if (typeof o =='string') { var res2=(o[0]=='_')?'':`<text x=\"${lx}\" y=\"${ly}\" font-family=\"Verdana\" font-size=\"${options.fontSize*0.1||0.1}\" style=\"pointer-events:none\" fill=\"${color||'#333'}\" transform=\"rotate(${lr},0,0)\"> ${o} </text>`; ly+=0.14; return res2; }\n // Colors\n if (typeof o =='number') { color='#'+(o+(1<<25)).toString(16).slice(-6); return ''; };\n // Points\n if (o[to2d[6]]**2 >0.0001) { lx=options.scale*o[drm[2]]/o[drm[1]]; if (drm[1]==6||drm[1]==14) lx*=-1; ly=options.scale*o[drm[3]]/o[drm[1]]; lr=0; var res2=`<CIRCLE onmousedown=\"this.parentElement.sel=${oidx}\" cx=\"${lx}\" cy=\"${ly}\" r=\"${options.pointRadius*0.03||0.03}\" fill=\"${color||'green'}\"/>`; ly-=0.05; lx-=0.1; return res2; }\n // Lines\n if (o[to2d[2]]**2+o[to2d[3]]**2>0.0001) { var l=Math.sqrt(o[to2d[2]]**2+o[to2d[3]]**2); o[to2d[2]]/=l; o[to2d[3]]/=l; o[to2d[1]]/=l; lx=0.5; ly=options.scale*((drm[1]==6)?-1:-1)*o[to2d[1]]; lr=-Math.atan2(o[to2d[2]],o[to2d[3]])/Math.PI*180; var res2=`<LINE style=\"pointer-events:none\" x1=-10 y1=${ly} x2=10 y2=${ly} stroke-width=\"${options.lineWidth*0.005||0.005}\" stroke=\"${color||'#888'}\" transform=\"rotate(${lr},0,0)\"/>`; ly-=0.05; return res2; }\n // Vectors\n if (o[to2d[4]]**2+o[to2d[5]]**2>0.0001) { lr=0; ly+=0.05; lx+=0.1; var res2=`<LINE style=\"pointer-events:none\" x1=${lx} y1=${ly} x2=${lx-o.e02} y2=${ly+o.e01} stroke-width=\"0.005\" stroke=\"${color||'#888'}\"/>`; ly=ly+o.e01/4*3-0.05; lx=lx-o.e02/4*3; return res2; }\n }).join()}`,'text/html').body;\n // return the inside of the created svg element.\n return svg.removeChild(svg.firstChild);\n };\n // Create the initial svg and install the mousehandlers.\n res=build(f); res.value=f; res.options=options;\n res.onmousemove=(e)=>{ if (res.sel===undefined || !e.buttons) return;var resx=res.getBoundingClientRect().width,resy=res.getBoundingClientRect().height,x=((e.clientX-res.getBoundingClientRect().left)/(resx/4||128)-2)*(resx>resy?resx/resy:1),y=((e.clientY-res.getBoundingClientRect().top)/(resy/4||128)-2)*(resy>resx?resy/resx:1);x/=options.scale;y/=options.scale; if (options.conformal) { f[res.sel].set(this.Coeff(1,x,2,-y).Add(no).Add(ni.Scale(0.5*(x*x+y*y))) ) } else {f[res.sel][drm[2]]=((drm[1]==6)?-x:x)-((tot<4)?2*options.camera.e01:0); f[res.sel][drm[3]]=y+((tot<4)?2*options.camera.e02:0); f[res.sel][drm[1]]=1; f[res.sel].set(f[res.sel].Normalized)} if (!anim) {var r=build(origf,(!res)||(document.body.contains(res))).innerHTML; if (res) res.innerHTML=r; } res.dispatchEvent(new CustomEvent('input')) };\n return res;\n }\n // 1d and 2d functions are rendered on a canvas.\n cvs=cvs||document.createElement('canvas'); if(ww)cvs.width=ww; if(hh)cvs.height=hh; var w=cvs.width,h=cvs.height,context=cvs.getContext('2d'), data=context.getImageData(0,0,w,h);\n // two parameter functions .. evaluate for both and set resulting color.\n if (f.length==2) for (var px=0; px<w; px++) for (var py=0; py<h; py++) { var res=f(px/w*2-1, py/h*2-1); res=res.buffer?[].slice.call(res):res.slice?res:[res,res,res]; data.data.set(res.map(x=>x*255).concat([255]),py*w*4+px*4); }\n // one parameter function.. go over x range, use result as y.\n else if (f.length==1) for (var px=0; px<w; px++) { var res=f(px/w*2-1); res=Math.round((res/2+0.5)*h); if (res > 0 && res < h-1) data.data.set([0,0,0,255],res*w*4+px*4); }\n return context.putImageData(data,0,0),cvs;\n }\n\n // webGL2 Graphing function. (for OPNS/IPNS implicit 2D and 1D surfaces in 3D space).\n static graphGL2(f,options) {\n // Create canvas, get webGL2 context.\n var canvas=document.createElement('canvas'); canvas.style.width=options.width||''; canvas.style.height=options.height||''; canvas.style.backgroundColor='#EEE';\n if (options.width && options.width.match && options.width.match(/px/i)) canvas.width = parseFloat(options.width)*(options.devicePixelRatio||1); if (options.height && options.height.match && options.height.match(/px/i)) canvas.height = parseFloat(options.height)*(options.devicePixelRatio||1);\n var gl=canvas.getContext('webgl2',{alpha:options.alpha||false,preserveDrawingBuffer:true,antialias:true,powerPreference:'high-performance'});\n var gl2=!!gl; if (!gl) gl=canvas.getContext('webgl',{alpha:options.alpha||false,preserveDrawingBuffer:true,antialias:true,powerPreference:'high-performance'});\n gl.clearColor(240/255,240/255,240/255,1.0); gl.enable(gl.DEPTH_TEST); if (!gl2) { gl.getExtension(\"EXT_frag_depth\"); gl.va = gl.getExtension('OES_vertex_array_object'); }\n else gl.va = { createVertexArrayOES : gl.createVertexArray.bind(gl), bindVertexArrayOES : gl.bindVertexArray.bind(gl), deleteVertexArrayOES : gl.deleteVertexArray.bind(gl) }\n // Compile vertex and fragment shader, return program.\n var compile=(vs,fs)=>{\n var s=[gl.VERTEX_SHADER,gl.FRAGMENT_SHADER].map((t,i)=>{\n var r=gl.createShader(t); gl.shaderSource(r,[vs,fs][i]); gl.compileShader(r);\n return gl.getShaderParameter(r, gl.COMPILE_STATUS)&&r||console.error(gl.getShaderInfoLog(r));\n });\n var p = gl.createProgram(); gl.attachShader(p, s[0]); gl.attachShader(p, s[1]); gl.linkProgram(p);\n gl.getProgramParameter(p, gl.LINK_STATUS)||console.error(gl.getProgramInfoLog(p));\n return p;\n };\n // Create vertex array and buffers, upload vertices and optionally texture coordinates.\n var createVA=function(vtx) {\n var r = gl.va.createVertexArrayOES(); gl.va.bindVertexArrayOES(r);\n var b = gl.createBuffer(); gl.bindBuffer(gl.ARRAY_BUFFER, b);\n gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(vtx), gl.STATIC_DRAW);\n gl.vertexAttribPointer(0, 3, gl.FLOAT, false, 0, 0); gl.enableVertexAttribArray(0);\n return {r,b}\n },\n // Destroy Vertex array and delete buffers.\n destroyVA=function(va) {\n if (va.b) gl.deleteBuffer(va.b); if (va.r) gl.va.deleteVertexArrayOES(va.r);\n }\n // Drawing function\n var M=[1,0,0,0,0,1,0,0,0,0,1,0,0,0,5,1];\n var draw=function(p, tp, vtx, color, color2, ratio, texc, va, b,color3,r,g){\n gl.useProgram(p); gl.uniformMatrix4fv(gl.getUniformLocation(p, \"mv\"),false,M);\n gl.uniformMatrix4fv(gl.getUniformLocation(p, \"p\"),false, [5,0,0,0,0,5*(ratio||1),0,0,0,0,1,2,0,0,-1,0])\n gl.uniform3fv(gl.getUniformLocation(p, \"color\"),new Float32Array(color));\n gl.uniform3fv(gl.getUniformLocation(p, \"color2\"),new Float32Array(color2));\n if (color3) gl.uniform3fv(gl.getUniformLocation(p, \"color3\"),new Float32Array(color3));\n if (b) gl.uniform1fv(gl.getUniformLocation(p, \"b\"),(new Float32Array(counts[g])).map((x,i)=>b[g][i]||0));\n if (texc) gl.uniform1i(gl.getUniformLocation(p, \"texc\"),0);\n if (r) gl.uniform1f(gl.getUniformLocation(p,\"ratio\"),r);\n var v; if (!va) v = createVA(vtx); else gl.va.bindVertexArrayOESOES(va.r);\n gl.drawArrays(tp, 0, (va&&va.tcount)||vtx.length/3);\n if (v) destroyVA(v);\n }\n // Compile the OPNS renderer. (sphere tracing)\n var programs = [], genprog = grade=>compile(`${gl2?\"#version 300 es\":\"\"}\n ${gl2?\"in\":\"attribute\"} vec4 position; ${gl2?\"out\":\"varying\"} vec4 Pos; uniform mat4 mv; uniform mat4 p;\n void main() { Pos=mv*position; gl_Position = p*Pos; }`,\n `${!gl2?\"#extension GL_EXT_frag_depth : enable\":\"#version 300 es\"}\n precision highp float;\n uniform vec3 color; uniform vec3 color2;\n uniform vec3 color3; uniform float b[${counts[grade]}];\n uniform float ratio; ${gl2?\"out vec4 col;\":\"\"}\n ${gl2?\"in\":\"varying\"} vec4 Pos;\n float dist (in float z, in float y, in float x, in float[${counts[grade]}] b) {\n ${this.nVector(1,[]).OPNS_GLSL(this.nVector(grade,[]), options.up)}\n return ${grade!=tot-1?\"sign(sum)*sqrt(abs(sum))\":\"res\"};\n }\n vec3 trace_depth (in vec3 start, vec3 dir, in float thresh) {\n vec3 orig=start; float lastd = 1000.0; const int count=${(options.maxSteps||64)};\n float s = sign(dist(start[0],start[1],start[2],b));\n for (int i=0; i<count; i++) {\n float d = s*dist(start[0],start[1],start[2],b);\n if (d < thresh) return start - lastd*${(options.stepSize||0.25)}*dir*(thresh-d)/(lastd-d);\n lastd = d; start += dir*${(options.stepSize||0.25)}*d;\n }\n return orig;\n }\n void main() {\n vec3 p = -5.0*normalize(color2);\n vec3 dir = normalize((-Pos[0]/5.0)*color + color2 + vec3(0.0,Pos[1]/5.0*ratio,0.0)); p += 1.0*dir;\n vec3 L = 5.0*normalize( -0.5*color + 0.85*color2 + vec3(0.0,-0.5,0.0) );\n vec3 d2 = trace_depth( p , dir, ${grade!=tot-1?(options.thresh||0.2):\"0.0075\"} );\n float dl2 = dot(d2-p,d2-p); const float h=0.1;\n if (dl2>0.0) {\n vec3 n = normalize(vec3(\n dist(d2[0]+h,d2[1],d2[2],b)-dist(d2[0]-h,d2[1],d2[2],b),\n dist(d2[0],d2[1]+h,d2[2],b)-dist(d2[0],d2[1]-h,d2[2],b),\n dist(d2[0],d2[1],d2[2]+h,b)-dist(d2[0],d2[1],d2[2]-h,b)\n ));\n ${gl2?\"gl_FragDepth\":\"gl_FragDepthEXT\"} = dl2/50.0;\n ${gl2?\"col\":\"gl_FragColor\"} = vec4(max(0.2,abs(dot(n,normalize(L-d2))))*color3 + pow(abs(dot(n,normalize(normalize(L-d2)+dir))),100.0),1.0);\n } else discard;\n }`),genprog2D = grade=>compile(`${gl2?\"#version 300 es\":\"\"}\n ${gl2?\"in\":\"attribute\"} vec4 position; ${gl2?\"out\":\"varying\"} vec4 Pos; uniform mat4 mv; uniform mat4 p;\n void main() { Pos=mv*position; gl_Position = p*Pos; }`,\n `${!gl2?\"#extension GL_EXT_frag_depth : enable\":\"#version 300 es\"}\n precision highp float;\n uniform vec3 color; uniform vec3 color2;\n uniform vec3 color3; uniform float b[${counts[grade]}];\n uniform float ratio; ${gl2?\"out vec4 col;\":\"\"}\n ${gl2?\"in\":\"varying\"} vec4 Pos;\n float dist (in float z, in float y, in float x, in float[${counts[grade]}] b) {\n ${this.nVector(1,[]).OPNS_GLSL(this.nVector(grade,[]), options.up)}\n return ${grade!=tot-1?\"sqrt(abs(sum))\":\"res\"};\n }\n float trace_depth (in vec3 start, vec3 dir, in float thresh) {\n vec3 orig=start; float lastd = 1000.0; const int count=${(options.maxSteps||64)};\n float s = dist(start[0]*5.0,start[1]*5.0,start[2]*5.0,b);\n s=s*s;\n return 1.0-s*150.0;\n }\n void main() {\n vec3 p = -5.0*normalize(color2);\n vec3 dir = normalize((-Pos[0]/5.0)*color + color2 + vec3(0.0,Pos[1]/5.0*ratio,0.0)); p += 1.0*dir;\n vec3 L = 5.0*normalize( -0.5*color + 0.85*color2 + vec3(0.0,-0.5,0.0) );\n float d2 = trace_depth( p , dir, ${grade!=tot-1?(options.thresh||0.2):\"0.0075\"} );\n if (d2>0.0) {\n ${gl2?\"gl_FragDepth\":\"gl_FragDepthEXT\"} = d2/50.0;\n ${gl2?\"col\":\"gl_FragColor\"} = vec4(d2*color3,d2);\n } else discard;\n }`)\n // canvas update will (re)render the content.\n var armed=0;\n canvas.update = (x)=>{\n // Start by updating canvas size if needed and viewport.\n var s = getComputedStyle(canvas); if (s.width) { canvas.width = parseFloat(s.width)*(options.devicePixelRatio||1); canvas.height = parseFloat(s.height)*(options.devicePixelRatio||1); }\n gl.viewport(0,0, canvas.width|0,canvas.height|0); var r=canvas.width/canvas.height;\n // Defaults, resolve function input\n var a,p=[],l=[],t=[],c=[.5,.5,.5],alpha=0,lastpos=[-2,2,0.2]; gl.clear(gl.COLOR_BUFFER_BIT+gl.DEPTH_BUFFER_BIT); while (x.call) x=x();\n // Loop over all items to render.\n for (var i=0,ll=x.length;i<ll;i++) {\n var e=x[i]; while (e&&e.call) e=e(); if (e==undefined) continue;\n if (typeof e == \"number\") { alpha=((e>>>24)&0xff)/255; c[0]=((e>>>16)&0xff)/255; c[1]=((e>>>8)&0xff)/255; c[2]=(e&0xff)/255; }\n if (e instanceof Element){\n var tt = options.spin?-performance.now()*options.spin/1000:-options.h||0; tt+=Math.PI/2; var r = canvas.height/canvas.width;\n var g=tot-1; while(!e[g]&&g>1) g--;\n if (!programs[tot-1-g]) programs[tot-1-g] = (options.up.find(x=>x.match&&x.match(\"z\")))?genprog(g):genprog2D(g);\n gl.enable(gl.BLEND); gl.blendFunc(gl.ONE, gl.ONE_MINUS_SRC_ALPHA);\n draw(programs[tot-1-g],gl.TRIANGLES,[-2,-2,0,-2,2,0,2,-2,0,-2,2,0,2,-2,0,2,2,0],[Math.cos(tt),0,-Math.sin(tt)],[Math.sin(tt),0,Math.cos(tt)],undefined,undefined,undefined,e,c,r,g);\n gl.disable(gl.BLEND);\n }\n }\n // if we're no longer in the page .. stop doing the work.\n armed++; if (document.body.contains(canvas)) armed=0; if (armed==2) return;\n canvas.value=x; if (options&&!options.animate) canvas.dispatchEvent(new CustomEvent('input'));\n if (options&&options.animate) { requestAnimationFrame(canvas.update.bind(canvas,f,options)); }\n if (options&&options.still) { canvas.value=x; canvas.dispatchEvent(new CustomEvent('input')); canvas.im.width=canvas.width; canvas.im.height=canvas.height; canvas.im.src = canvas.toDataURL(); }\n }\n // Basic mouse interactivity. needs more love.\n var sel=-1; canvas.oncontextmenu = canvas.onmousedown = (e)=>{ e.preventDefault(); e.stopPropagation(); sel=-2;\n var rc = canvas.getBoundingClientRect(), mx=(e.x-rc.left)/(rc.right-rc.left)*2-1, my=((e.y-rc.top)/(rc.bottom-rc.top)*-4+2)*canvas.height/canvas.width;\n canvas.onwheel=e=>{e.preventDefault(); e.stopPropagation(); options.z = (options.z||5)+e.deltaY/100; if (!options.animate) requestAnimationFrame(canvas.update.bind(canvas,f,options));}\n canvas.onmouseup=e=>sel=-1; canvas.onmouseleave=e=>sel=-1;\n canvas.onmousemove=(e)=>{\n var rc = canvas.getBoundingClientRect();\n var mx =(e.movementX)/(rc.right-rc.left)*2, my=((e.movementY)/(rc.bottom-rc.top)*-2)*canvas.height/canvas.width;\n if (sel==-2) { options.h = (options.h||0)+mx; if (!options.animate) requestAnimationFrame(canvas.update.bind(canvas,f,options)); return; }; if (sel < 0) return;\n }\n }\n canvas.value = f.call?f():f; canvas.options = options;\n if (options&&options.still) {\n var i=new Image(); canvas.im = i; return requestAnimationFrame(canvas.update.bind(canvas,f,options)),i;\n } else return requestAnimationFrame(canvas.update.bind(canvas,f,options)),canvas;\n\n }\n\n\n // webGL Graphing function. (for parametric defined objects)\n static graphGL(f,options) {\n // Create a canvas, webgl2 context and set some default GL options.\n var canvas=document.createElement('canvas'); canvas.style.width=options.width||''; canvas.style.height=options.height||''; canvas.style.backgroundColor='#EEE';\n if (options.width && options.width.match && options.width.match(/px/i)) canvas.width = parseFloat(options.width); if (options.height && options.height.match && options.height.match(/px/i)) canvas.height = parseFloat(options.height);\n var gl=canvas.getContext('webgl',{alpha:options.alpha||false,antialias:true,preserveDrawingBuffer:options.still||true,powerPreference:'high-performance'});\n gl.enable(gl.DEPTH_TEST); gl.depthFunc(gl.LEQUAL); if (!options.alpha) gl.clearColor(240/255,240/255,240/255,1.0); gl.getExtension(\"OES_standard_derivatives\"); gl.va=gl.getExtension(\"OES_vertex_array_object\");\n // Compile vertex and fragment shader, return program.\n var compile=(vs,fs)=>{\n var s=[gl.VERTEX_SHADER,gl.FRAGMENT_SHADER].map((t,i)=>{\n var r=gl.createShader(t); gl.shaderSource(r,[vs,fs][i]); gl.compileShader(r);\n return gl.getShaderParameter(r, gl.COMPILE_STATUS)&&r||console.error(gl.getShaderInfoLog(r));\n });\n var p = gl.createProgram(); gl.attachShader(p, s[0]); gl.attachShader(p, s[1]); gl.linkProgram(p);\n gl.getProgramParameter(p, gl.LINK_STATUS)||console.error(gl.getProgramInfoLog(p));\n return p;\n };\n // Create vertex array and buffers, upload vertices and optionally texture coordinates.\n var createVA=function(vtx, texc, idx, clr) {\n var r = gl.va.createVertexArrayOES(); gl.va.bindVertexArrayOES(r);\n var b = gl.createBuffer(); gl.bindBuffer(gl.ARRAY_BUFFER, b);\n gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(vtx), gl.STATIC_DRAW);\n gl.vertexAttribPointer(0, 3, gl.FLOAT, false, 0, 0); gl.enableVertexAttribArray(0);\n if (texc){\n var b2=gl.createBuffer(); gl.bindBuffer(gl.ARRAY_BUFFER, b2);\n gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(texc), gl.STATIC_DRAW);\n gl.vertexAttribPointer(1, 2, gl.FLOAT, false, 0, 0); gl.enableVertexAttribArray(1);\n }\n if (clr){\n var b3=gl.createBuffer(); gl.bindBuffer(gl.ARRAY_BUFFER, b3);\n gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(clr), gl.STATIC_DRAW);\n gl.vertexAttribPointer(texc?2:1, 3, gl.FLOAT, false, 0, 0); gl.enableVertexAttribArray(texc?2:1);\n }\n if (idx) {\n var b4=gl.createBuffer(); gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, b4);\n gl.bufferData(gl.ELEMENT_ARRAY_BUFFER, new Uint16Array(idx), gl.STATIC_DRAW);\n }\n return {r,b,b2,b4,b3}\n },\n // Destroy Vertex array and delete buffers.\n destroyVA=function(va) {\n [va.b,va.b2,va.b4,va.b3].forEach(x=>{if(x) gl.deleteBuffer(x)}); if (va.r) gl.va.deleteVertexArrayOES(va.r);\n }\n // Default modelview matrix, convert camera to matrix (biquaternion->matrix)\n var M=[1,0,0,0,0,1,0,0,0,0,1,0,0,0,5,1], mtx = x=>{ var t=options.spin?performance.now()*options.spin/1000:options.h||0, t2=options.p||0;\n var ct = Math.cos(t), st= Math.sin(t), ct2 = Math.cos(t2), st2 = Math.sin(t2), xx=options.posx||0, y=options.posy||0, z=options.posz||0, zoom=options.z||5;\n if (tot==5) return [ct,st*-st2,st*ct2,0,0,ct2,st2,0,-st,ct*-st2,ct*ct2,0,xx*ct+z*-st,y*ct2+(xx*st+z*ct)*-st2,y*st2+xx*st+z*ct*ct2+zoom,1];\n x=x.Normalized; var y=x.Mul(x.Dual),X=-x.e23,Y=-x.e13,Z=x.e12,W=x.s,m=Array(16);\n var xx = X*X, xy = X*Y, xz = X*Z, xw = X*W, yy = Y*Y, yz = Y*Z, yw = Y*W, zz = Z*Z, zw = Z*W;\n var mtx = [ 1-2*(yy+zz), 2*(xy+zw), 2*(xz-yw), 0, 2*(xy-zw), 1-2*(xx+zz), 2*(yz+xw), 0, 2*(xz+yw), 2*(yz-xw), 1-2*(xx+yy), 0, -2*y.e23, -2*y.e13, 2*y.e12+5, 1];\n var mtx2 = [ct,st*-st2,st*ct2,0,0,ct2,st2,0,-st,ct*-st2,ct*ct2,0,0,0,0,1], mtx3=[0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0];\n for (var i=0; i<4; ++i) for (var j=0; j<4; ++j) for (var k=0; k<4; ++k) mtx3[i+k*4] += mtx[i+j*4]*mtx2[j+k*4]; return mtx3;\n return mtx;\n }\n // Render the given vertices. (autocreates/destroys vertex array if not supplied).\n var draw=function(p, tp, vtx, color, color2, ratio, texc, va){\n gl.useProgram(p); gl.uniformMatrix4fv(gl.getUniformLocation(p, \"mv\"),false,M);\n gl.uniformMatrix4fv(gl.getUniformLocation(p, \"p\"),false, [5,0,0,0,0,5*(ratio||2),0,0,0,0,1,2,0,0,-1,0])\n gl.uniform3fv(gl.getUniformLocation(p, \"color\"),new Float32Array(color));\n gl.uniform3fv(gl.getUniformLocation(p, \"color2\"),new Float32Array(color2));\n if (texc) gl.uniform1i(gl.getUniformLocation(p, \"texc\"),0);\n var v; if (!va) v = createVA(vtx, texc); else gl.va.bindVertexArrayOES(va.r);\n if (va && va.b4) {\n gl.drawElements(tp, va.tcount, gl.UNSIGNED_SHORT, 0);\n } else {\n gl.drawArrays(tp, 0, (va&&va.tcount)||vtx.length/3);\n }\n if (v) destroyVA(v);\n }\n // Program for the geometry. Derivative based normals. Basic lambert shading.\n var program = compile(`attribute vec4 position; varying vec4 Pos; uniform mat4 mv; uniform mat4 p;\n void main() { gl_PointSize=12.0; Pos=mv*position; gl_Position = p*Pos; }`,\n `#extension GL_OES_standard_derivatives : enable\n precision highp float; uniform vec3 color; uniform vec3 color2; varying vec4 Pos;\n void main() { vec3 ldir = normalize(Pos.xyz - vec3(2.0,2.0,-4.0));\n vec3 normal = normalize(cross(dFdx(Pos.xyz), dFdy(Pos.xyz))); float l=dot(normal,ldir);\n vec3 E = normalize(-Pos.xyz); vec3 R = normalize(reflect(ldir,normal));\n gl_FragColor = vec4(max(0.0,l)*color+vec3(0.5*pow(max(dot(R,E),0.0),20.0))+color2, 1.0); }`);\n var programPoint = compile(`attribute vec4 position; varying vec4 Pos; uniform mat4 mv; uniform mat4 p;\n void main() { gl_PointSize=${((options.pointRadius||1)*8.0).toFixed(2)}; Pos=mv*position; gl_Position = p*Pos; }`,\n `precision highp float; uniform vec3 color; uniform vec3 color2; varying vec4 Pos;\n void main() { float distanceToCenter = length(gl_PointCoord - vec2(0.5)); if (distanceToCenter>0.5) discard; \n gl_FragColor = vec4(color+color2, (distanceToCenter<0.5?1.0:0.0)); }`);\n var programcol = compile(`attribute vec4 position; attribute vec3 col; varying vec3 Col; varying vec4 Pos; uniform mat4 mv; uniform mat4 p;\n void main() { gl_PointSize=6.0; Pos=mv*position; gl_Position = p*Pos; Col=col; }`,\n `#extension GL_OES_standard_derivatives : enable\n precision highp float; uniform vec3 color; uniform vec3 color2; varying vec4 Pos; varying vec3 Col;\n void main() { vec3 ldir = normalize(Pos.xyz - vec3(1.0,1.0,2.0));\n vec3 normal = normalize(cross(dFdx(Pos.xyz), dFdy(Pos.xyz))); float l=dot(normal,ldir);\n vec3 E = normalize(-Pos.xyz); vec3 R = normalize(reflect(ldir,normal));\n gl_FragColor = vec4(max(0.3,l)*Col+vec3(pow(max(dot(R,E),0.0),20.0))+color2, 1.0); }`);\n var programmot = compile(`attribute vec4 position; attribute vec2 texc; attribute vec3 col; varying vec3 Col; varying vec4 Pos; uniform mat4 mv; uniform mat4 p; uniform vec3 color2;\n void main() { gl_PointSize=2.0; float blend=fract(color2.x+texc.r)*0.5; Pos=mv*(position*(1.0-blend) + (blend)*vec4(col,1.0)); gl_Position = p*Pos; Col=vec3(length(col-position.xyz)*1.); gl_PointSize = 8.0 - Col.x; Col.y=sin(blend*2.*3.1415); }`,\n `precision highp float; uniform vec3 color; uniform vec3 color2; varying vec4 Pos; varying vec3 Col; \n void main() { float distanceToCenter = length(gl_PointCoord - vec2(0.5));gl_FragColor = vec4(1.0-pow(Col.x,2.0),0.0,0.0,(.6-Col.x*0.05)*(distanceToCenter<0.5?1.0:0.0)*Col.y); }`);\n gl.lineWidth(options.lineWidth||1); // doesn't work yet (nobody supports it)\n // Create a font texture, lucida console or otherwise monospaced.\n var fw=33, font = Object.assign(document.createElement('canvas'),{width:(19+94)*fw,height:48}),\n ctx = Object.assign(font.getContext('2d'),{font:'bold 48px lucida console, monospace'}),\n ftx = gl.createTexture(); gl.activeTexture(gl.TEXTURE0); gl.bindTexture(gl.TEXTURE_2D, ftx);\n for (var i=33; i<127; i++) ctx.fillText(String.fromCharCode(i),(i-33)*fw,40);\n var specialChars = \"∞≅¹²³₀₁₂₃₄₅₆₇₈₉⋀⋁∆⋅\"; specialChars.split('').forEach((x,i)=>ctx.fillText(x,(i-33+127)*fw,40));\n // 2.0 gl.texImage2D(gl.TEXTURE_2D,0,gl.RGBA,94*fw,32,0,gl.RGBA,gl.UNSIGNED_BYTE,font);\n gl.texImage2D(gl.TEXTURE_2D, 0, gl.RGBA, gl.RGBA, gl.UNSIGNED_BYTE, font);\n gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_MAG_FILTER, gl.LINEAR); gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_MIN_FILTER, gl.LINEAR);\n gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_WRAP_S, gl.CLAMP_TO_EDGE); gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_WRAP_T, gl.CLAMP_TO_EDGE);\n // Font rendering program. Renders billboarded fonts, transforms offset passed as color2.\n var program2 = compile(`attribute vec4 position; attribute vec2 texc; varying vec2 tex; varying vec4 Pos; uniform mat4 mv; uniform mat4 p; uniform vec3 color2;\n void main() { tex=texc; gl_PointSize=6.0; vec4 o=mv*vec4(color2,0.0); Pos=(-1.0/(o.z-mv[3][2]))*position+vec4(mv[3][0],mv[3][1],mv[3][2],0.0)+o; gl_Position = p*Pos; }`,\n `precision highp float; uniform vec3 color; varying vec4 Pos; varying vec2 tex;\n uniform sampler2D texm; void main() { vec4 c = texture2D(texm,tex); if (c.a<0.01) discard; gl_FragColor = vec4(color,c.a);}`);\n // Conformal space needs a bit extra magic to extract euclidean parametric representations.\n if (tot==5 && options.conformal) var ni = Element.Coeff(4,1).Add(Element.Coeff(5,1)), no = Element.Coeff(4,0.5).Sub(Element.Coeff(5,0.5));\n var interprete = (x)=>{\n if (!(x instanceof Element)) return { tp:0 };\n if (options.ipns) x=x.Dual;\n // tp = { 0:unknown 1:point 2:line, 3:plane, 4:circle, 5:sphere\n var X2 = (x.Mul(x)).s, tp=0, weight2, opnix = ni.Wedge(x), ipnix = ni.LDot(x),\n attitude, pos, normal, tg,btg,epsilon = 0.000001/(options.scale||1), I3=Element.Coeff(16,-1);\n var x2zero = Math.abs(X2) < epsilon, ipnixzero = ipnix.VLength < epsilon, opnixzero = opnix.VLength < epsilon;\n if (opnixzero && ipnixzero) { // free flat\n } else if (opnixzero && !ipnixzero) { // bound flat (lines)\n attitude = no.Wedge(ni).LDot(x);\n weight2 = Math.abs(attitude.LDot(attitude).s)**.5;\n pos = attitude.LDot(x.Reverse); //Inverse);\n pos = [-pos.e15/pos.e45,-pos.e25/pos.e45,-pos.e34/pos.e45];\n if (x.Grade(3).VLength) {\n normal = [attitude.e1/weight2,attitude.e2/weight2,attitude.e3/weight2]; tp=2;\n } else if (x.Grade(2).VLength) { // point pair with ni\n tp = 1;\n } else {\n normal = Element.LDot(Element.Mul(attitude,1/weight2),I3).Normalized;\n var r=normal.Mul(Element.Coeff(3,1)); if (r[0]==-1) r[0]=1; else {r[0]+=1; r=r.Normalized;}\n tg = [...r.Mul(Element.Coeff(1,1)).Mul(r.Conjugate)].slice(1,4);\n btg = [...r.Mul(Element.Coeff(2,1)).Mul(r.Conjugate)].slice(1,4);\n normal = [...normal.slice(1,4)]; tp=3;\n }\n } else if (!opnixzero && ipnixzero) { // dual bound flat\n } else if (x2zero) { // bound vec,biv,tri (points)\n if (options.ipns) x=x.Dual;\n attitude = ni.Wedge(no).LDot(ni.Wedge(x));\n pos = [...(Element.LDot(1/(ni.LDot(x)).s,x)).slice(1,4)].map(x=>-x);\n tp=1;\n } else if (!x2zero) { // round (point pair,circle,sphere)\n tp = x.Grade(3).VLength?4:x.Grade(2).VLength?6:5;\n var nix = ni.Wedge(x), nix2 = (nix.Mul(nix)).s;\n attitude = ni.Wedge(no).LDot(nix);\n pos = [...(x.Mul(ni).Mul(x)).slice(1,4)].map(x=>-x/(2.0*nix2));\n weight2 = Math.abs((x.LDot(x)).s / nix2)**.5;\n if (tp==4) {\n if (x.LDot(x).s < 0) { weight2 = -weight2; }\n normal = Element.LDot(Element.Mul(attitude,1/weight2),I3).Normalized;\n var r=normal.Mul(Element.Coeff(3,1)); if (r[0]==-1) r[0]=1; else {r[0]+=1; r=r.Normalized;}\n tg = [...r.Mul(Element.Coeff(1,1)).Mul(r.Conjugate)].slice(1,4);\n btg = [...r.Mul(Element.Coeff(2,1)).Mul(r.Conjugate)].slice(1,4);\n normal = [...normal.slice(1,4)];\n } else if (tp==6) {\n weight2 = (x.LDot(x).s < 0)?-(weight2):weight2;\n normal = Element.Mul(attitude.Normalized,weight2).slice(1,4);\n } else {\n normal = [...((Element.LDot(Element.Mul(attitude,1/weight2),I3)).Normalized).slice(1,4)];\n }\n }\n return {tp,pos:pos?pos.map(x=>x*(options.scale||1)):[0,0,0],normal,tg,btg,weight2:weight2*(options.scale||1)}\n };\n // canvas update will (re)render the content.\n var armed=0,sphere,e14 = Element.Coeff(14,1);\n canvas.update = (x)=>{\n // restore from still..\n if (options && !options.still && canvas.im && canvas.im.parentElement) { canvas.im.parentElement.insertBefore(canvas,canvas.im); canvas.im.parentElement.removeChild(canvas.im); }\n // Start by updating canvas size if needed and viewport.\n var s = getComputedStyle(canvas); if (s.width) { canvas.width = parseFloat(s.width)*(options.devicePixelRatio||1); canvas.height = parseFloat(s.height)*(options.devicePixelRatio||1); }\n gl.viewport(0,0, canvas.width|0,canvas.height|0); var r=canvas.width/canvas.height;\n // Defaults, resolve function input\n var a,p=[],l=[],t=[],c=[.5,.5,.5],alpha=0,lastpos=[-2,2,0.2]; gl.clear(gl.COLOR_BUFFER_BIT+gl.DEPTH_BUFFER_BIT); while (x.call) x=x();\n // Create default camera matrix and initial lastposition (contra-compensated for camera)\n M = mtx(options.camera); lastpos = options.camera.Normalized.Conjugate.Mul(((a=new this()).set(lastpos,11),a)).Mul(options.camera.Normalized).slice(11,14);\n // Grid.\n if (options.grid) {\n if (!options.gridLines) { options.gridLines=[[],[],[]]; for (var i=-5; i<=5; i++) {\n options.gridLines[0].push(i,0,5, i,0,-5, 5,0,i, -5,0,i); options.gridLines[1].push(i,5,0, i,-5,0, 5,i,0, -5,i,0); options.gridLines[2].push(0,i,5, 0,i,-5, 0,5,i, 0,-5,i);\n }}\n gl.depthMask(false);\n draw(program,gl.LINES,options.gridLines[0],[0,0,0],[.6,1,.6],r); draw(program,gl.LINES,options.gridLines[1],[0,0,0],[1,.8,.8],r); draw(program,gl.LINES,options.gridLines[2],[0,0,0],[.8,.8,1],r);\n gl.depthMask(true);\n }\n // Z-buffer override.\n if (options.noZ) gl.depthMask(false);\n // Loop over all items to render.\n for (var i=0,ll=x.length;i<ll;i++) {\n var e=x[i]; while (e&&e.call&&e.length==0) e=e(); if (e==undefined) continue;\n // CGA\n if (tot==5 && options.conformal) {\n if (e instanceof Array && e.length==2) { e.forEach(x=>{ while (x.call) x=x.call(); x=interprete(x);l.push.apply(l,x.pos); }); var d = {tp:-1}; }\n else if (e instanceof Array && e.length==3) { e.forEach(x=>{ while (x.call) x=x.call(); x=interprete(x);t.push.apply(t,x.pos); }); var d = {tp:-1}; }\n else var d = interprete(e);\n if (d.tp) lastpos=d.pos;\n if (d.tp==1) p.push.apply(p,d.pos);\n if (d.tp==2) { l.push.apply(l,d.pos.map((x,i)=>x-d.normal[i]*10)); l.push.apply(l,d.pos.map((x,i)=>x+d.normal[i]*10)); }\n if (d.tp==3) { t.push.apply(t,d.pos.map((x,i)=>x+d.tg[i]+d.btg[i])); t.push.apply(t,d.pos.map((x,i)=>x-d.tg[i]+d.btg[i])); t.push.apply(t,d.pos.map((x,i)=>x+d.tg[i]-d.btg[i]));\n t.push.apply(t,d.pos.map((x,i)=>x-d.tg[i]+d.btg[i])); t.push.apply(t,d.pos.map((x,i)=>x+d.tg[i]-d.btg[i])); t.push.apply(t,d.pos.map((x,i)=>x-d.tg[i]-d.btg[i])); }\n if (d.tp==4) {\n var ne=0,la=0;\n if (d.weight2<0) { c[0]=1;c[1]=0;c[2]=0; }\n for (var j=0; j<65; j++) {\n ne = d.pos.map((x,i)=>x+Math.cos(j/32*Math.PI)*d.weight2*d.tg[i]+Math.sin(j/32*Math.PI)*d.weight2*d.btg[i]); if (ne&&la&&(d.weight2>0||j%2==0)) { l.push.apply(l,la); l.push.apply(l,ne); }; la=ne;\n }\n }\n if (d.tp==6) {\n if (d.weight2<0) { c[0]=1;c[1]=0;c[2]=0; }\n if (options.useUnnaturalLineDisplayForPointPairs) {\n l.push.apply(l,d.pos.map((x,i)=>x-d.normal[i]*(options.scale||1)));\n l.push.apply(l,d.pos.map((x,i)=>x+d.normal[i]*(options.scale||1)));\n }\n p.push.apply(p,d.pos.map((x,i)=>x-d.normal[i]*(options.scale||1)));\n p.push.apply(p,d.pos.map((x,i)=>x+d.normal[i]*(options.scale||1)));\n }\n if (d.tp==5) {\n if (!sphere) {\n var pnts = [], tris=[], S=Math.sin, C=Math.cos, pi=Math.PI, W=96, H=48;\n for (var j=0; j<W+1; j++) for (var k=0; k<H; k++) {\n pnts.push( [S(2*pi*j/W)*S(pi*k/(H-1)), C(2*pi*j/W)*S(pi*k/(H-1)), C(pi*k/(H-1))]);\n if (j && k) {\n tris.push.apply(tris, pnts[(j-1)*H+k-1]);tris.push.apply(tris, pnts[(j-1)*H+k]);tris.push.apply(tris, pnts[j*H+k-1]);\n tris.push.apply(tris, pnts[j*H+k-1]); tris.push.apply(tris, pnts[(j-1)*H+k]); tris.push.apply(tris, pnts[j*H+k]);\n }}\n sphere = { va : createVA(tris,undefined) }; sphere.va.tcount = tris.length/3;\n }\n var oldM = M;\n M=[].concat.apply([],Element.Mul([[d.weight2,0,0,0],[0,d.weight2,0,0],[0,0,d.weight2,0],[d.pos[0],d.pos[1],d.pos[2],1]],[[M[0],M[1],M[2],M[3]],[M[4],M[5],M[6],M[7]],[M[8],M[9],M[10],M[11]],[M[12],M[13],M[14],M[15]]])).map(x=>x.s);\n gl.enable(gl.BLEND); gl.blendFunc(gl.CONSTANT_ALPHA, gl.ONE_MINUS_CONSTANT_ALPHA); gl.blendColor(1,1,1,0.5); gl.enable(gl.CULL_FACE)\n draw(program,gl.TRIANGLES,undefined,c,[0,0,0],r,undefined,sphere.va);\n gl.disable(gl.BLEND); gl.disable(gl.CULL_FACE);\n M = oldM;\n }\n if (i==ll-1 || d.tp==0) {\n // render triangles, lines, points.\n if (alpha) { gl.enable(gl.BLEND); gl.blendFunc(gl.CONSTANT_ALPHA, gl.ONE_MINUS_CONSTANT_ALPHA); gl.blendColor(1,1,1,1-alpha); }\n if (t.length) { draw(program,gl.TRIANGLES,t,c,[0,0,0],r); t.forEach((x,i)=>{ if (i%9==0) lastpos=[0,0,0]; lastpos[i%3]+=x/3; }); t=[]; }\n if (l.length) { draw(program,gl.LINES,l,[0,0,0],c,r); var l2=l.length-1; lastpos=[(l[l2-2]+l[l2-5])/2,(l[l2-1]+l[l2-4])/2+0.1,(l[l2]+l[l2-3])/2]; l=[]; }\n if (p.length) { gl.enable(gl.BLEND); gl.blendFunc(gl.SRC_ALPHA, gl.ONE_MINUS_SRC_ALPHA); draw(programPoint,gl.POINTS,p,[0,0,0],c,r); lastpos = p.slice(-3); lastpos[0]-=0.075; lastpos[1]+=0.075; p=[]; gl.disable(gl.BLEND); }\n // Motor orbits\n if ( e.call && e.length==2 && !e.va3) { var countx=e.dx||32,county=e.dy||32;\n var temp=new Float32Array(3*countx*county),o=new Float32Array(3),et=[];\n for (var pp=0,ii=0; ii<countx; ii++) for (var jj=0; jj<county; jj++,pp+=3)\n temp.set(Element.sw(e(ii/(countx-1),jj/(county-1)),no).slice(1,4),pp);\n for (ii=0; ii<countx-1; ii++) for (var jj=0; jj<county; jj++)\n et.push((ii+0)*county+(jj+0),(ii+0)*county+(jj+1),(ii+1)*county+(jj+1),(ii+0)*county+(jj+0),(ii+1)*county+(jj+1),(ii+1)*county+(jj+0));\n e.va3 = createVA(temp,undefined,et.map(x=>x%(countx*county))); e.va3.tcount = (countx-1)*county*2*3;\n }\n if ( e.call && e.length==1 && !e.va2) { var countx=e.dx||256;\n var temp=new Float32Array(3*countx),o=new Float32Array(3),et=[];\n for (var ii=0; ii<countx; ii++) { temp.set(Element.sw(e(ii/(countx-1)),no).slice(1,4),ii*3); if (ii) et.push(ii-1,ii); }\n e.va2 = createVA(temp,undefined,et); e.va2.tcount = et.length;\n }\n // Experimental display of motors using particle systems.\n if (e instanceof Object && e.motor) {\n if (!e.va || e.recalc) {\n var seed = 1; function random() { var x = Math.sin(seed++) * 10000; return x - Math.floor(x); }\n e.xRange = e.xRange === undefined ? 1:e.xRange; e.yRange = e.yRange === undefined ? 1:e.yRange; e.zRange = e.zRange === undefined ? 1:e.zRange;\n var vtx=[], tx=[], vtx2=[];\n for (var i=0; i<(e.zRange*e.xRange*e.yRange===0?2500:Math.pow(e.zRange*e.xRange*e.yRange,1/3)*6000); i++) {\n var [x,y,z] = [random()*(2*e.xRange)-e.xRange,random()*2*e.yRange-e.yRange,random()*2*e.zRange-e.zRange];\n var xyz = (x*x+y*y+z*z)*0.5;\n var p = Element.Vector(x,y,z,xyz-0.5,xyz+0.5);\n var p2 = Element.sw(e.motor,p);\n var d = p2[5]-p2[4]; p2[1]/=d; p2[2]/=d; p2[3]/=d;\n tx.push(random(), random());\n vtx.push(...p.slice(1,4)); vtx2.push(...p2.slice(1,4));\n } \n e.va = createVA(vtx,tx,undefined,vtx2); e.va.tcount = vtx.length/3;\n e.recalc = false;\n } \n var time = performance.now()/1000;\n gl.enable(gl.BLEND); gl.blendFunc(gl.SRC_ALPHA, gl.ONE_MINUS_SRC_ALPHA); gl.disable(gl.DEPTH_TEST);\n draw(programmot, gl.POINTS,t,c,[time%1,0,0],r,undefined,e.va);\n gl.disable(gl.BLEND); gl.enable(gl.DEPTH_TEST);\n }\n // we could also be an object with cached vertex array of triangles ..\n else if (e.va || e.va2 || e.va3 || (e instanceof Object && e.data)) {\n // Create the vertex array and store it for re-use.\n if (!e.va3 && !e.va2) {\n var et=[],et2=[],et3=[],lc=0,pc=0,tc=0; e.data.forEach(e=>{\n if (e instanceof Array && e.length==3) { tc++; e.forEach(x=>{ while (x.call) x=x.call(); x=interprete(x);et3.push.apply(et3,x.pos); }); var d = {tp:-1}; }\n else {\n var d = interprete(e);\n if (d.tp==1) { pc++; et.push(...d.pos); }\n if (d.tp==2) { lc++; et2.push(...d.pos.map((x,i)=>x-d.normal[i]*10),...d.pos.map((x,i)=>x+d.normal[i]*10)); }\n }\n });\n e.va = createVA(et,undefined); e.va.tcount = pc;\n e.va2 = createVA(et2,undefined); e.va2.tcount = lc*2;\n e.va3 = createVA(et3,undefined); e.va3.tcount = tc*3;\n }\n // render the vertex array.\n if (e.va && e.va.tcount) { gl.enable(gl.BLEND); gl.blendFunc(gl.SRC_ALPHA, gl.ONE_MINUS_SRC_ALPHA); draw(programPoint,gl.POINTS,undefined,[0,0,0],c,r,undefined,e.va); gl.disable(gl.BLEND); };\n if (e.va2 && e.va2.tcount) draw(program,gl.LINES,undefined,[0,0,0],c,r,undefined,e.va2);\n if (e.va3 && e.va3.tcount) draw(program,gl.TRIANGLES,undefined,c,[0,0,0],r,undefined,e.va3);\n }\n if (alpha) gl.disable(gl.BLEND); // no alpha for text printing.\n // setup a new color\n if (typeof e == \"number\") { alpha=((e>>>24)&0xff)/255; c[0]=((e>>>16)&0xff)/255; c[1]=((e>>>8)&0xff)/255; c[2]=(e&0xff)/255; }\n if (typeof(e)=='string') {\n if (options.htmlText) {\n if (!x['_'+i]) { console.log('creating div'); Object.defineProperty(x,'_'+i, {value: document.body.appendChild(document.createElement('div')), enumerable:false }) };\n var rc = canvas.getBoundingClientRect(), div = x['_'+i];\n var pos2 = Element.Mul( [[M[0],M[4],M[8],M[12]],[M[1],M[5],M[9],M[13]],[M[2],M[6],M[10],M[14]],[M[3],M[7],M[11],M[15]]], [...lastpos,1]).map(x=>x.s);\n pos2 = Element.Mul( [[5,0,0,0],[0,5*(r||2),0,0],[0,0,1,-1],[0,0,2,0]], pos2).map(x=>x.s).map((x,i,a)=>x/a[3]);\n Object.assign(div.style,{position:'fixed',pointerEvents:'none',left:rc.left + (rc.right-rc.left)*(pos2[0]/2+0.5),top: rc.top + (rc.bottom-rc.top)*(-pos2[1]/2+0.5) - 20});\n if (div.last != e) { div.innerHTML = e; div.last = e; if (self.renderMathInElement) self.renderMathInElement(div); }\n } else { \n gl.enable(gl.BLEND); gl.blendFunc(gl.SRC_ALPHA,gl.ONE_MINUS_SRC_ALPHA);\n var fw = 113, mapChar = (x)=>{ var c = x.charCodeAt(0)-33; if (c>=94) c = 94+specialChars.indexOf(x); return c/fw; }\n draw(program2,gl.TRIANGLES,\n [...Array(e.length*6*3)].map((x,i)=>{ var x=0,z=-0.2, o=x+(i/18|0)*1.1; return (0.05*(options.z||5))*[o,-1,z,o+1.2,-1,z,o,1,z,o+1.2,-1,z,o+1.2,1,z,o,1,z][i%18]}),c,lastpos,r,\n [...Array(e.length*6*2)].map((x,i)=>{ var o=mapChar(e[i/12|0]); return [o,1,o+1/fw,1,o,0,o+1/fw,1,o+1/fw,0,o,0][i%12]})); gl.disable(gl.BLEND); lastpos[1]-=0.18;\n } \n }\n }\n continue;\n }\n // PGA\n if (options.dual && e instanceof Element) e = e.Dual;\n // Convert planes to polygons.\n if (e instanceof Element && e.Grade(1).Length) {\n var m = Element.Add(1, Element.Mul(e.Normalized, Element.Coeff(3,1))).Normalized, e0 = 0;\n e=Element.sw(m,[[-1,-1],[-1,1],[1,1],[-1,-1],[1,1],[1,-1]].map(([x,z])=>Element.Trivector(x,e0,z,1)));\n }\n // Convert lines to line segments.\n if (e instanceof Element && e.Grade(2).Length)\n e=[e.LDot(e14).Wedge(e).Add(e.Wedge(Element.Coeff(1,1)).Mul(Element.Coeff(0,-500))),e.LDot(e14).Wedge(e).Add(e.Wedge(Element.Coeff(1,1)).Mul(Element.Coeff(0,500)))];\n // If euclidean point, store as point, store line segments and triangles.\n if (e.e123) p.push.apply(p,e.slice(11,14).map((y,i)=>(i==0?1:-1)*y/e[14]).reverse());\n if (e instanceof Array && e.length==2) l=l.concat.apply(l,e.map(x=>[...x.slice(11,14).map((y,i)=>(i==0?1:-1)*y/x[14]).reverse()]));\n if (e instanceof Array && e.length%3==0) t=t.concat.apply(t,e.map(x=>[...x.slice(11,14).map((y,i)=>(i==0?1:-1)*y/x[14]).reverse()]));\n // Render orbits of parametrised motors, as well as lists of points.. \n function sw_mot_orig(A,R){\n var a0=A[0],a1=A[5],a2=A[6],a3=A[7],a4=A[8],a5=A[9],a6=A[10],a7=A[15];\n R[2] = -2*(a0*a3+a4*a7-a6*a2-a5*a1);\n R[1] = -2*(a4*a1-a0*a2-a6*a3+a5*a7);\n R[0] = 2*(a0*a1+a4*a2+a5*a3+a6*a7);\n return R\n }\n if ( e.call && e.length==1) { var count=e.dx||64;\n for (var ismot,xx,o=new Float32Array(3),ii=0; ii<count; ii++) {\n if (ii>1) l.push(xx[0],xx[1],xx[2]);\n var m = e(ii/(count-1));\n if (ii==0) ismot = m[0]||m[5]||m[6]||m[7]||m[8]||m[9]||m[10];\n xx = ismot?sw_mot_orig(m,o):m.slice(11,14).map((y,i)=>(i==0?1:-1)*y).reverse(); //Element.sw(e(ii/(count-1)),o);\n l.push(xx[0],xx[1],xx[2]);\n }\n }\n if ( e.call && e.length==2 && !e.va) { var countx=e.dx||64,county=e.dy||32;\n var temp=new Float32Array(3*countx*county),o=new Float32Array(3),et=[];\n for (var pp=0,ii=0; ii<countx; ii++) for (var jj=0; jj<county; jj++,pp+=3) temp.set(sw_mot_orig(e(ii/(countx-1),jj/(county-1)),o),pp);\n for (ii=0; ii<countx-1; ii++) for (var jj=0; jj<county; jj++) et.push((ii+0)*county+(jj+0),(ii+0)*county+(jj+1),(ii+1)*county+(jj+1),(ii+0)*county+(jj+0),(ii+1)*county+(jj+1),(ii+1)*county+(jj+0));\n e.va = createVA(temp,undefined,et.map(x=>x%(countx*county))); e.va.tcount = (countx-1)*county*2*3;\n }\n // Experimental display of motors using particle systems.\n if (e instanceof Object && e.motor) {\n if (!e.va || e.recalc) {\n var seed = 1; function random() { var x = Math.sin(seed++) * 10000; return x - Math.floor(x); }\n e.xRange = e.xRange === undefined ? 1:e.xRange; e.yRange = e.yRange === undefined ? 1:e.yRange; e.zRange = e.zRange === undefined ? 1:e.zRange;\n var vtx=[], tx=[], vtx2=[];\n for (var i=0; i<(e.zRange===0?5000:60000); i++) {\n var p = Element.Trivector(random()*(2*e.xRange)-e.xRange,random()*2*e.yRange-e.yRange,random()*2*e.zRange-e.zRange,1);\n// var p2 = Element.sw(e.motor,p);\n var p2 = e.motor.Mul(p).Mul(e.motor.Inverse);\n tx.push(random(), random());\n vtx.push(...p.slice(11,14).reverse()); vtx2.push(...p2.slice(11,14).reverse());\n } \n e.va = createVA(vtx,tx,undefined,vtx2); e.va.tcount = vtx.length/3;\n e.recalc = false;\n } \n var time = performance.now()/1000;\n gl.enable(gl.BLEND); gl.blendFunc(gl.SRC_ALPHA, gl.ONE_MINUS_SRC_ALPHA); gl.disable(gl.DEPTH_TEST);\n draw(programmot, gl.POINTS,t,c,[time%1,0,0],r,undefined,e.va);\n gl.disable(gl.BLEND); gl.enable(gl.DEPTH_TEST);\n }\n // we could also be an object with cached vertex array of triangles ..\n else if (e.va || (e instanceof Object && e.data)) {\n // Create the vertex array and store it for re-use.\n if (!e.va) {\n if (e.idx) {\n var et = e.data.map(x=>[...x.slice(11,14).map((y,i)=>(i==0?1:-1)*y/x[14]).reverse()]).flat();\n } else {\n var et=[]; e.data.forEach(e=>{if (e instanceof Array && e.length==3) et=et.concat.apply(et,e.map(x=>[...x.slice(11,14).map((y,i)=>(i==0?1:-1)*y/x[14]).reverse()]));});\n }\n e.va = createVA(et,undefined,e.idx,e.color?new Float32Array(e.color):undefined); e.va.tcount = (e.idx && e.idx.length)?e.idx.length:e.data.length*3;\n }\n // render the vertex array.\n if (e.transform) { M=mtx(options.camera.Mul(e.transform)); }\n if (alpha) { gl.enable(gl.BLEND); gl.blendFunc(gl.CONSTANT_ALPHA, gl.ONE_MINUS_CONSTANT_ALPHA); gl.blendColor(1,1,1,1-alpha); }\n draw(e.color?programcol:program,gl.TRIANGLES,t,c,[0,0,0],r,undefined,e.va);\n if (alpha) gl.disable(gl.BLEND);\n if (e.transform) { M=mtx(options.camera); }\n }\n // if we're a number (color), label or the last item, we output the collected items.\n else if (typeof e=='number' || i==ll-1 || typeof e == 'string') {\n // render triangles, lines, points.\n if (alpha) { gl.enable(gl.BLEND); gl.blendFunc(gl.CONSTANT_ALPHA, gl.ONE_MINUS_CONSTANT_ALPHA); gl.blendColor(1,1,1,1-alpha); }\n if (t.length) { draw(program,gl.TRIANGLES,t,c,[0,0,0],r); t.forEach((x,i)=>{ if (i%9==0) lastpos=[0,0,0]; lastpos[i%3]+=x/3; }); t=[]; }\n if (l.length) { draw(program,gl.LINES,l,[0,0,0],c,r); var l2=l.length-1; lastpos=[(l[l2-2]+l[l2-5])/2,(l[l2-1]+l[l2-4])/2+0.1,(l[l2]+l[l2-3])/2]; l=[]; }\n if (p.length) { gl.enable(gl.BLEND); gl.blendFunc(gl.SRC_ALPHA, gl.ONE_MINUS_SRC_ALPHA); draw(programPoint,gl.POINTS,p,[0,0,0],c,r); lastpos = p.slice(-3); lastpos[0]-=0.075; lastpos[1]+=0.075; p=[];gl.disable(gl.BLEND); }\n if (alpha) gl.disable(gl.BLEND);\n // setup a new color\n if (typeof e == \"number\") { alpha=((e>>>24)&0xff)/255; c[0]=((e>>>16)&0xff)/255; c[1]=((e>>>8)&0xff)/255; c[2]=(e&0xff)/255; }\n // render a label\n if (typeof(e)=='string') {\n if (options.htmlText) { \n if (!canvas['_'+i]) { console.log('creating div'); Object.defineProperty(canvas,'_'+i, {value: document.body.appendChild(document.createElement('div')), enumerable:false }) };\n var rc = canvas.getBoundingClientRect(), div = canvas['_'+i];\n var pos2 = Element.Mul( [[M[0],M[4],M[8],M[12]],[M[1],M[5],M[9],M[13]],[M[2],M[6],M[10],M[14]],[M[3],M[7],M[11],M[15]]], [...lastpos,1]).map(x=>x.s);\n pos2 = Element.Mul( [[5,0,0,0],[0,5*(r||2),0,0],[0,0,1,-1],[0,0,2,0]], pos2).map(x=>x.s).map((x,i,a)=>x/a[3]);\n Object.assign(div.style,{position:'fixed',pointerEvents:'none',left:rc.left + (rc.right-rc.left)*(pos2[0]/2+0.5),top: rc.top + (rc.bottom-rc.top)*(-pos2[1]/2+0.5) - 20});\n if (div.last != e) { div.innerHTML = e; div.last = e; if (self.renderMathInElement) self.renderMathInElement(div,{output:'html'}); }\n } else { \n gl.enable(gl.BLEND); gl.blendFunc(gl.SRC_ALPHA,gl.ONE_MINUS_SRC_ALPHA);\n var fw = 113, mapChar = (x)=>{ var c = x.charCodeAt(0)-33; if (c>=94) c = 94+specialChars.indexOf(x); return c/fw; }\n draw(program2,gl.TRIANGLES,\n [...Array(e.length*6*3)].map((x,i)=>{ var x=0,z=-0.2, o=x+(i/18|0)*1.1; return 0.25*[o,-1,z,o+1.2,-1,z,o,1,z,o+1.2,-1,z,o+1.2,1,z,o,1,z][i%18]}),c,lastpos,r,\n [...Array(e.length*6*2)].map((x,i)=>{ var o=mapChar(e[i/12|0]); return [o,1,o+1/fw,1,o,0,o+1/fw,1,o+1/fw,0,o,0][i%12]})); gl.disable(gl.BLEND); lastpos[1]-=0.18;\n }\n }\n }\n };\n // if we're no longer in the page .. stop doing the work.\n armed++; if (document.body.contains(canvas)) armed=0; if (armed==2) return;\n canvas.value=x; if (options&&!options.animate) canvas.dispatchEvent(new CustomEvent('input')); canvas.options=options;\n if (options&&options.animate) { requestAnimationFrame(canvas.update.bind(canvas,f,options)); }\n if (options&&options.still) { canvas.value=x; canvas.dispatchEvent(new CustomEvent('input')); canvas.im.style.width=canvas.style.width; canvas.im.style.height=canvas.style.height; canvas.im.src = canvas.toDataURL();\n var p=canvas.parentElement; if (p) { p.insertBefore(canvas.im,canvas); p.removeChild(canvas); }\n }\n }\n // Basic mouse interactivity. needs more love.\n var sel=-1; canvas.oncontextmenu = canvas.onmousedown = (e)=>{e.preventDefault(); e.stopPropagation(); if (e.detail===0) return;\n var rc = canvas.getBoundingClientRect(), mx=(e.x-rc.left)/(rc.right-rc.left)*2-1, my=((e.y-rc.top)/(rc.bottom-rc.top)*-4+2)*canvas.height/canvas.width;\n sel = (e.button==2)?-3:-2; canvas.value.forEach((x,i)=>{\n if (tot != 5) { if (x[14]) {\n var pos2 = Element.Mul( [[M[0],M[4],M[8],M[12]],[M[1],M[5],M[9],M[13]],[M[2],M[6],M[10],M[14]],[M[3],M[7],M[11],M[15]]], [-x[13]/x[14],-x[12]/x[14],x[11]/x[14],1]).map(x=>x.s);\n pos2 = Element.Mul( [[5,0,0,0],[0,5*(2),0,0],[0,0,1,-1],[0,0,2,0]], pos2).map(x=>x.s).map((x,i,a)=>x/a[3]);\n if ((mx-pos2[0])**2 + ((my)-pos2[1])**2 < 0.01) sel=i;\n }} else {\n x = interprete(x); if (x.tp==1) {\n var pos2 = Element.Mul( [[M[0],M[4],M[8],M[12]],[M[1],M[5],M[9],M[13]],[M[2],M[6],M[10],M[14]],[M[3],M[7],M[11],M[15]]], [...x.pos,1]).map(x=>x.s);\n pos2 = Element.Mul( [[5,0,0,0],[0,5*(r||2),0,0],[0,0,1,-1],[0,0,2,0]], pos2).map(x=>x.s).map((x,i,a)=>x/a[3]);\n if ((mx-pos2[0])**2 + (my-pos2[1])**2 < 0.01) sel=i;\n }\n }\n });\n canvas.onwheel=e=>{e.preventDefault(); e.stopPropagation(); options.z = (options.z||5)+e.deltaY/100; if (!options.animate) requestAnimationFrame(canvas.update.bind(canvas,f,options));}\n canvas.onmouseup=e=>sel=-1; canvas.onmouseleave=e=>sel=-1;\n var tx,ty; canvas.ontouchstart = (e)=>{e.preventDefault(); canvas.focus(); var x = e.changedTouches[0].pageX, y = e.changedTouches[0].pageY; tx=x; ty=y; }\n canvas.ontouchmove = function (e) { e.preventDefault();\n var x = e.changedTouches[0].pageX, y = e.changedTouches[0].pageY, mx = (x-(tx||x))/1000, my = -(y-(ty||y))/1000; tx=x; ty=y;\n options.h = (options.h||0)+mx; options.p = Math.max(-Math.PI/2,Math.min(Math.PI/2, (options.p||0)+my)); if (!options.animate) requestAnimationFrame(canvas.update.bind(canvas,f,options)); return;\n };\n canvas.onmousemove=(e)=>{\n var rc = canvas.getBoundingClientRect(),x; if (sel>=0) { if (tot==5) x=interprete(canvas.value[sel]); else { x=canvas.value[sel]; x={pos:[-x[13]/x[14],-x[12]/x[14],x[11]/x[14]]}; }}\n var mx =(e.movementX)/(rc.right-rc.left)*2, my=((e.movementY)/(rc.bottom-rc.top)*-2)*canvas.height/canvas.width;\n if (sel==-2) { options.h = (options.h||0)+mx; options.p = Math.max(-Math.PI/2,Math.min(Math.PI/2, (options.p||0)+my)); if (!options.animate) requestAnimationFrame(canvas.update.bind(canvas,f,options)); return; };\n if (sel==-3) { var ct = Math.cos(options.h||0), st= Math.sin(options.h||0), ct2 = Math.cos(options.p||0), st2 = Math.sin(options.p||0);\n if (e.shiftKey) { options.posy = (options.posy||0)+my; } else { options.posx = (options.posx||0)+mx*ct+my*st; options.posz = (options.posz||0)+mx*-st+my*ct*ct2; } if (!options.animate) requestAnimationFrame(canvas.update.bind(canvas,f,options));return; }; if (sel < 0) return;\n if (tot==5) { \n x.pos[0] += (e.buttons!=2)?Math.cos(-(options.h||0))*mx:Math.sin((options.h||0))*my; x.pos[1]+=(e.buttons!=2)?my:0; x.pos[2]+=(e.buttons!=2)?Math.sin(-(options.h||0))*mx:Math.cos((options.h||0))*my;\n canvas.value[sel].set(Element.Mul(ni,(x.pos[0]**2+x.pos[1]**2+x.pos[2]**2)*0.5).Sub(no)); canvas.value[sel].set(x.pos,1); }\n else if (x) { \n x.pos[2] += (e.buttons!=2)?Math.sin(-(options.h||0))*mx:Math.cos((options.h||0))*my; x.pos[1]+=(e.buttons!=2)?my:0; x.pos[0]+=(e.buttons!=2)?Math.cos(-(options.h||0))*mx:Math.sin((options.h||0))*my;\n canvas.value[sel].set([x.pos[2],-x.pos[1],-x.pos[0],1],11);\n }\n if (!options.animate) requestAnimationFrame(canvas.update.bind(canvas,f,options));\n }\n }\n canvas.value = f.call?f():f; canvas.options=options;\n if (options&&options.still) {\n var i=new Image(); canvas.im = i; return requestAnimationFrame(canvas.update.bind(canvas,f,options)),canvas;\n } else return requestAnimationFrame(canvas.update.bind(canvas,f,options)),canvas;\n }\n\n // The inline function is a js to js translator that adds operator overloading and algebraic literals.\n // It can be called with a function, a string, or used as a template function.\n static inline(intxt) {\n // If we are called as a template function.\n if (arguments.length>1 || intxt instanceof Array) {\n var args=[].slice.call(arguments,1);\n return res.inline(new Function(args.map((x,i)=>'_template_'+i).join(),'return ('+intxt.map((x,i)=>(x||'')+(args[i]&&('_template_'+i)||'')).join('')+')')).apply(res,args);\n }\n // Get the source input text.\n var txt = (intxt instanceof Function)?intxt.toString():`function(){return (${intxt})}`;\n // Our tokenizer reads the text token by token and stores it in the tok array (as type/token tuples).\n var tok = [], resi=[], t, tokens = [/^[\\s\\uFFFF]|^[\\u000A\\u000D\\u2028\\u2029]|^\\/\\/[^\\n]*\\n|^\\/\\*[\\s\\S]*?\\*\\//g, // 0: whitespace/comments\n /^\\\"\\\"|^\\'\\'|^\\\".*?[^\\\\]\\\"|^\\'.*?[^\\\\]\\'|^\\`[\\s\\S]*?[^\\\\]\\`/g, // 1: literal strings\n /^\\d+[.]{0,1}\\d*[ei][\\+\\-_]{0,1}\\d*|^\\.\\d+[ei][\\+\\-_]{0,1}\\d*|^e_\\d*/g, // 2: literal numbers in scientific notation (with small hack for i and e_ asciimath)\n /^\\d+[.]{0,1}\\d*[E][+-]{0,1}\\d*|^\\.\\d+[E][+-]{0,1}\\d*|^0x\\d+|^\\d+[.]{0,1}\\d*|^\\.\\d+|^\\(\\/.*[^\\\\]\\/\\)/g, // 3: literal hex, nonsci numbers and regex (surround regex with extra brackets!)\n /^(\\.Normalized|\\.Length|\\.\\.\\.|>>>=|===|!==|>>>|<<=|>>=|=>|\\|\\||[<>\\+\\-\\*%&|^\\/!\\=]=|\\*\\*|\\+\\+|\\-\\-|<<|>>|\\&\\&|\\^\\^|^[{}()\\[\\];.,<>\\+\\-\\*%|&^!~?:=\\/]{1})/g, // 4: punctuator\n /^[A-Za-z0-9_]*/g] // 5: identifier\n while (txt.length) for(t in tokens) if(resi=txt.match(tokens[t])){ tok.push([t|0,resi[0]]); txt=txt.slice(resi[0].length); break;} // tokenise\n // Translate algebraic literals. (scientific e-notation to \"this.Coeff\"\n tok=tok.map(t=>(t[0]==2)?[2,'Element.Coeff('+basis.indexOf((!options.Cayley?simplify:(x)=>x)('e'+t[1].split(/e_|e|i/)[1]||1).replace('-',''))+','+(simplify(t[1].split(/e_|e|i/)[1]||1).match('-')?\"-1*\":\"\")+parseFloat(t[1][0]=='e'?1:t[1].split(/e_|e|i/)[0])+')']:t);\n // String templates (limited support - needs fundamental changes.).\n tok=tok.map(t=>(t[0]==1 && t[1][0]=='`')?[1,t[1].replace(/\\$\\{(.*?)\\}/g,a=>\"${\"+Element.inline(a.slice(2,-1)).toString().match(/return \\((.*)\\)/)[1]+\"}\")]:t); \n // We support two syntaxes, standard js or if you pass in a text, asciimath.\n var syntax = (intxt instanceof Function)?[[['.Normalized','Normalize',2],['.Length','Length',2]],[['~','Conjugate',1],['!','Dual',1]],[['**','Pow',0,1]],[['^','Wedge'],['&','Vee'],['<<','LDot']],[['*','Mul'],['/','Div']],[['|','Dot']],[['>>>','sw',0,1]],[['-','Sub'],['+','Add']],[['%','%']],[['==','eq'],['!=','neq'],['<','lt'],['>','gt'],['<=','lte'],['>=','gte']]]\n :[[['pi','Math.PI'],['sin','Math.sin']],[['ddot','this.Reverse'],['tilde','this.Involute'],['hat','this.Conjugate'],['bar','this.Dual']],[['^','Pow',0,1]],[['^^','Wedge'],['*','LDot']],[['**','Mul'],['/','Div']],[['-','Sub'],['+','Add']],[['<','lt'],['>','gt'],['<=','lte'],['>=','gte']]];\n // For asciimath, some fixed translations apply (like pi->Math.PI) etc ..\n tok=tok.map(t=>(t[0]!=5)?t:[].concat.apply([],syntax).filter(x=>x[0]==t[1]).length?[5,[].concat.apply([],syntax).filter(x=>x[0]==t[1])[0][1]]:t);\n // Now the token-stream is translated recursively.\n function translate(tokens) {\n // helpers : first token to the left of x that is not of a type in the skip list.\n var left = (x=ti-1,skip=[0])=>{ while(x>=0&&~skip.indexOf(tokens[x][0])) x--; return x; },\n // first token to the right of x that is not of a type in the skip list.\n right= (x=ti+1,skip=[0])=>{ while(x<tokens.length&&~skip.indexOf(tokens[x][0])) x++; return x; },\n // glue from x to y as new type, optionally replace the substring with sub.\n glue = (x,y,tp=5,sub)=>{tokens.splice(x,y-x+1,[tp,...(sub||tokens.slice(x,y+1))])},\n // match O-C pairs. returns the 'matching bracket' position\n match = (O=\"(\",C=\")\")=>{var o=1,x=ti+1; while(o){if(tokens[x][1]==O)o++;if(tokens[x][1]==C)o--; x++;}; return x-1;};\n // grouping (resolving brackets).\n for (var ti=0,t,si;t=tokens[ti];ti++) if (t[1]==\"(\") glue(ti,si=match(),6,[[4,\"(\"],...translate(tokens.slice(ti+1,si)),[4,\")\"]]);\n // [] dot call and new\n for (var ti=0,t,si; t=tokens[ti];ti++) {\n if (t[1]==\"[\") { glue(ti,si=match(\"[\",\"]\"),6,[[4,\"[\"],...translate(tokens.slice(ti+1,si)),[4,\"]\"]]); if (ti)ti--;} // matching []\n else if (t[1]==\".\") { glue(left(),right()); ti--; } // dot operator\n else if (t[0]==6 && ti && left()>=0 && tokens[left()][0]>=5 && tokens[left()][1]!=\"return\") { glue(left(),ti--) } // collate ( and [\n else if (t[1]=='new') { glue(ti,right()) }; // collate new keyword\n }\n // ++ and --\n for (var ti=0,t; t=tokens[ti];ti++) if (t[1]==\"++\" || t[1]==\"--\") glue(left(),ti);\n // unary - and + are handled seperately from syntax ..\n for (var ti=0,t,si; t=tokens[ti];ti++)\n if (t[1]==\"-\" && (left()<0 || (tokens[left()]||[4])[0]==4)) glue(ti,right(),5,[\"Element.Sub(\",tokens[right()],\")\"]); // unary minus works on all types.\n else if (t[1]==\"+\" && (tokens[left()]||[0])[0]==4 && (tokens[left()]||[0])[1][0]!=\".\") glue(ti,ti+1); // unary plus is glued, only on scalars.\n // now process all operators in the syntax list ..\n for (var si=0,s; s=syntax[si]; si++) for (var ti=s[0][3]?tokens.length-1:0,t; t=tokens[ti];s[0][3]?ti--:ti++) for (var opi=0,op; op=s[opi]; opi++) if (t[1]==op[0]) {\n // exception case .. \".Normalized\" and \".Length\" properties are re-routed (so they work on scalars etc ..)\n if (op[2]==2) { var arg=tokens[left()]; glue(ti-1,ti,5,[\"Element.\"+op[1],\"(\",arg,\")\"]); }\n // unary operators (all are to the left)\n else if (op[2]) { var arg=tokens[right()]; glue(ti, right(), 5, [\"Element.\"+op[1],\"(\",arg,\")\"]); }\n // binary operators\n else { var l=left(),r=right(),a1=tokens[l],a2=tokens[r]; if (op[0]==op[1]) glue(l,r,5,[a1,op[1],a2]); else glue(l,r,5,[\"Element.\"+op[1],\"(\",a1,\",\",a2,\")\"]); ti--; }\n }\n return tokens;\n }\n // Glue all back together and return as bound function.\n return eval( ('('+(function f(t){return t.map(t=>t instanceof Array?f(t):typeof t == \"string\"?t:\"\").join('');})(translate(tok))+')') );\n }\n }\n\n if (options.dual) {\n Object.defineProperty(res.prototype, 'Inverse', {configurable:true, get(){ var s = 1/this.s**2; return this.map((x,i)=>i?-x*s:1/x ); }});\n } else {\n // Matrix-free inverses up to 5D. Should translate this to an inline call for readability.\n // http://repository.essex.ac.uk/17282/1/TechReport_CES-534.pdf\n Object.defineProperty(res.prototype, 'Inverse', {configurable: true, get(){\n return (tot==0)?new this.constructor.Scalar([1/this[0]]):\n (tot==1)?this.Involute.Mul(this.constructor.Scalar(1/this.Mul(this.Involute)[0])):\n (tot==2)?this.Conjugate.Mul(this.constructor.Scalar(1/this.Mul(this.Conjugate)[0])):\n (tot==3)?this.Reverse.Mul(this.Involute).Mul(this.Conjugate).Mul( this.constructor.Scalar(1/this.Mul(this.Conjugate).Mul(this.Involute).Mul(this.Reverse)[0])):\n (tot==4)?this.Conjugate.Mul(this.Mul(this.Conjugate).Map(3,4)).Mul( this.constructor.Scalar(1/this.Mul(this.Conjugate).Mul(this.Mul(this.Conjugate).Map(3,4))[0])):\n this.Conjugate.Mul(this.Involute).Mul(this.Reverse).Mul(this.Mul(this.Conjugate).Mul(this.Involute).Mul(this.Reverse).Map(1,4)).Mul(this.constructor.Scalar(1/this.Mul(this.Conjugate).Mul(this.Involute).Mul(this.Reverse).Mul(this.Mul(this.Conjugate).Mul(this.Involute).Mul(this.Reverse).Map(1,4))[0]));\n }});\n }\n\n if (options.over) {\n // experimental. do not use.\n res.over = options.over;\n [\"Mul\",\"Add\",\"Sub\",\"Scale\",\"Dot\",\"Wedge\",\"LDot\",\"Vee\"].forEach(x=>res.prototype[x] = options.over.inline(res.prototype[x]));\n res.prototype.Coeff = function() { for (var i=0,l=arguments.length; i<l; i+=2) this[arguments[i]]=(arguments[i+1] instanceof options.over)?arguments[i+1]:options.over.Scalar(arguments[i+1]); return this; }\n res.prototype.upgrade = function () { for (var i=0; i<this.length; i++) this[i] = options.over.Scalar(0); }\n Object.defineProperty(res.prototype, 'Conjugate', {configurable:true,get(){var res = new this.constructor(); for (var i=0; i<this.length; i++) res[i]= this[i].slice().Scale([1,-1,-1,1][grades[i]%4]); return res; }});\n Object.defineProperty(res.prototype, 'Reverse', {configurable:true,get(){var res = new this.constructor(); for (var i=0; i<this.length; i++) res[i]= this[i].slice().Scale([1,1,-1,-1][grades[i]%4]); return res; }});\n Object.defineProperty(res.prototype, 'Involute', {configurable:true,get(){var res = new this.constructor(); for (var i=0; i<this.length; i++) res[i]= this[i].slice().Scale([1,-1,1,-1][grades[i]%4]); return res; }});\n Object.defineProperty(res.prototype, 'Inverse', {configurable: true, get(){\n return (tot==0)?new this.constructor.Scalar([this[0].Inverse]):\n (tot==1)?this.Involute.Mul(this.constructor.Scalar(this.Mul(this.Involute)[0].Inverse)):\n (tot==2)?this.Conjugate.Mul(this.constructor.Scalar(this.Mul(this.Conjugate)[0].Inverse)):\n (tot==3)?this.Reverse.Mul(this.Involute).Mul(this.Conjugate).Mul( this.constructor.Scalar(this.Mul(this.Conjugate).Mul(this.Involute).Mul(this.Reverse)[0].Inverse)):\n (tot==4)?this.Conjugate.Mul(this.Mul(this.Conjugate).Map(3,4)).Mul( this.constructor.Scalar(this.Mul(this.Conjugate).Mul(this.Mul(this.Conjugate).Map(3,4))[0].Inverse)):\n this.Conjugate.Mul(this.Involute).Mul(this.Reverse).Mul(this.Mul(this.Conjugate).Mul(this.Involute).Mul(this.Reverse).Map(1,4)).Mul(this.constructor.Scalar(this.Mul(this.Conjugate).Mul(this.Involute).Mul(this.Reverse).Mul(this.Mul(this.Conjugate).Mul(this.Involute).Mul(this.Reverse).Map(1,4))[0].Inverse));\n }});\n res.prototype.toString = function() { return [...this].map((x,i)=>x==0?undefined:(i?'('+x+')'+basis[i]:x.toString())).filter(x=>x).join(' + '); }\n }\n\n // Experimental differential operator.\n var _D, _DT, _DA, totd = basis.length;\n function makeD(transpose=false){\n _DA = _DA || Algebra({ p:p,q:q,r:r,basis:options.basis,even:options.even,over:Algebra({dual:totd})}); // same algebra, but over dual numbers.\n return (func)=>{\n var dfunc = _DA.inline(func); // convert input function to dual algebra\n return (val,...args)=>{ // return a new function (the derivative w.r.t 1st param)\n if (!(val instanceof res)) val = res.Scalar(val); // allow to be called with scalars.\n args = args.map(x=>{ var r = _DA.Scalar(0); for (var i=0; i<totd; i++) r[i][0]=x[i]; return r;}); // upcast args.\n for (var dval=_DA.Scalar(0),i=0; i<totd; i++) { dval[i][0] = val[i]; dval[i][1+i] = 1; }; // fill in coefficients and dual components\n var rval = dfunc(dval,...args); var r = [...Array(totd)].map(x=>val.slice()); // call the function in the dual algebra.\n if (transpose) for (var i=0; i<totd; i++) for (var j=0; j<totd; j++) { r[i][j] = rval[i][j+1]; } // downcast transpose from dual algebra to Jacobian vector.\n else for (var i=0; i<totd; i++) for (var j=0; j<totd; j++) { r[j][i] = rval[i][j+1]; } // downcast from dual algebra to Jacobian vector.\n return r.length<=2?r[0]:r; // return derivative or jacobian.\n }\n }\n }\n Object.defineProperty(res, 'D', {configurable:true,get(){ if (_D) return _D; _D = makeD(false); return _D }});\n Object.defineProperty(res, 'Dt', {configurable:true,get(){ if (_DT) return _DT; _DT = makeD(true); return _DT }});\n\n // If a function was passed in, translate, call and return its result. Else just return the Algebra.\n if (fu instanceof Function) return res.inline(fu)(); else return res;\n }\n}));\n\n // take a closure on element before the next cell replaces it\n (function(element) {\n (requirejs||require)(['Algebra'], function(Algebra) {\n var opts = {\"p\": 2, \"q\": 0, \"r\": 1, \"mv_length\": \"8\", \"graph\": {\"conformal\": false, \"gl\": false, \"useUnnaturalLineDisplayForPointPairs\": true, \"grid\": true, \"labels\": true}}; // injected from python\n var output = Algebra({p: opts.p, q: opts.q, r: opts.r, baseType: Float64Array}).inline((opts)=>{\n var data = [13696993, [[0, 0, 0, 0, 1, -1, 1, 0], [0, 0, 0, 0, 1, 1, 1, 0], [0, 0, 0, 0, -1, 1, 1, 0]].map(x=>x.length==8?new Element(x):x), 8921736, [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 1.0, 0.0], \"project B onto AC\", 8921736, [0.0, 4.0, 2.0, -2.0, 0.0, 0.0, 0.0, 0.0], \"project AC onto B\", 34884, [0, 0, 2, 2, 0, 0, 0, 0], \"reject AC from B\", 43656, [0, 0, 2, -2, 0, 0, 0, 0], \"AC\", 2245768, [0, 0, 0, 0, 1, -1, 1, 0], \"A\", 2245768, [0, 0, 0, 0, 1, 1, 1, 0], \"B\", 2245768, [0, 0, 0, 0, -1, 1, 1, 0], \"C\"]; // injected from python\n // When we get a file, we load and display.\n var canvas;\n var h=0, p=0;\n // convert arrays of floats back to CGA elements.\n data = data.map(x=>x.length==opts.mv_length?new Element(x):x);\n // add the graph to the page.\n canvas = this.graph(data, opts.graph);\n canvas.options.h = h;\n canvas.options.p = p;\n // make it big.\n canvas.style.width = '100%';\n canvas.style.height = '50vh';\n return canvas;\n })(opts);\n element.append(output);\n var a = document.createElement(\"button\");\n var t = document.createTextNode(\"💾 Save\");\n a.appendChild(t);\n function screenshot(){\n //output.width = 1920; output.height = 1080;\n output.update(output.value);\n output.toBlob(function(blob) {\n var url = URL.createObjectURL(blob);\n window.open(url, '_blank');\n });\n }\n window.addEventListener('resize', function() {\n output.update(output.value);\n });\n a.onclick = screenshot\n var butnelem = element.append(a);\n });\n })(element);\n "},"metadata":{}}]},{"metadata":{"trusted":true},"cell_type":"code","source":"","execution_count":null,"outputs":[]}],"metadata":{"kernelspec":{"name":"python3","display_name":"Python 3","language":"python"},"language_info":{"name":"python","version":"3.7.8","mimetype":"text/x-python","codemirror_mode":{"name":"ipython","version":3},"pygments_lexer":"ipython3","nbconvert_exporter":"python","file_extension":".py"}},"nbformat":4,"nbformat_minor":1} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here is what the visualisation outputs:
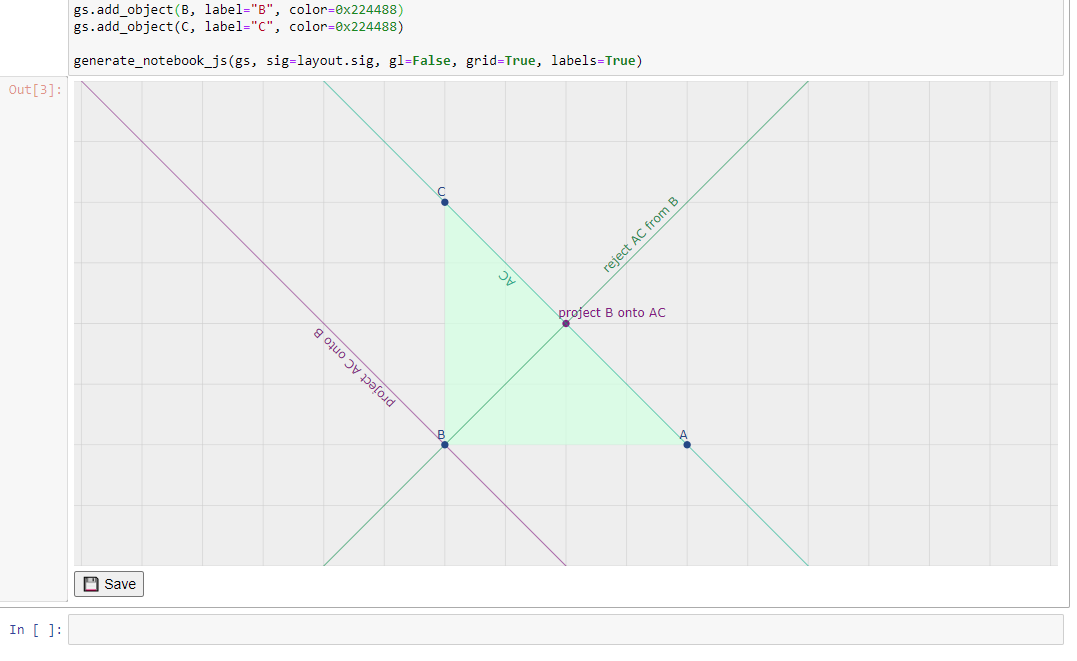