Last active
January 25, 2023 15:52
-
-
Save vincentsarago/57c6213c10e714f2b62f914c2462dc1a to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"cells": [ | |
{ | |
"cell_type": "markdown", | |
"id": "6d77e3f8", | |
"metadata": {}, | |
"source": [ | |
"# TiTiler-PgSTAC Demo\n", | |
"\n", | |
"\n", | |
"For this demo I'm going to use https://github.com/developmentseed/eoAPI\n", | |
"\n", | |
"eoAPI let's you deploy a PgSTAC database + a STAC + a TiTiler-PGSTAC services\n", | |
"\n", | |
"\n", | |
"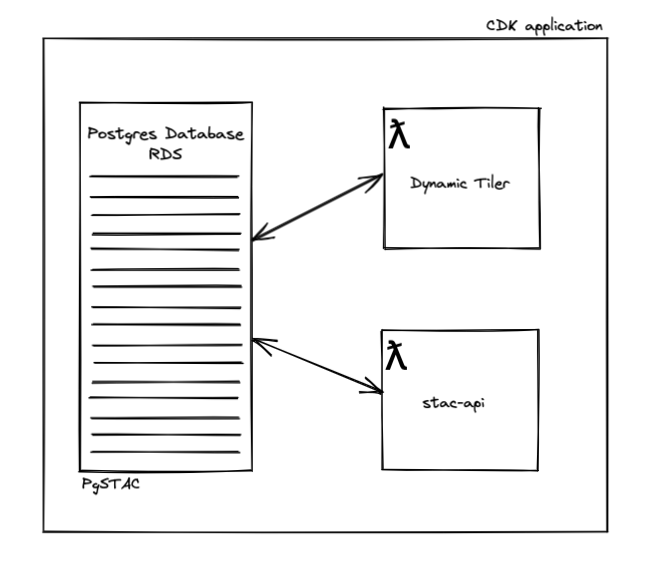" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 14, | |
"id": "763e0780", | |
"metadata": {}, | |
"outputs": [], | |
"source": [ | |
"import json\n", | |
"import httpx\n", | |
"\n", | |
"from folium import Map, TileLayer, GeoJson\n", | |
"\n", | |
"from geojson_pydantic import Feature, Polygon\n", | |
"\n", | |
"\n", | |
"raster_endpoint = \"http://127.0.0.1:8082\"\n", | |
"stac_endpoint = \"http://127.0.0.1:8081\"" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 9, | |
"id": "12163188", | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"{\n", | |
" \"collections\": [\n", | |
" {\n", | |
" \"id\": \"noaa-emergency-response\",\n", | |
" \"links\": [\n", | |
" {\n", | |
" \"rel\": \"items\",\n", | |
" \"type\": \"application/geo+json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/collections/noaa-emergency-response/items\"\n", | |
" },\n", | |
" {\n", | |
" \"rel\": \"parent\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/\"\n", | |
" },\n", | |
" {\n", | |
" \"rel\": \"root\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/\"\n", | |
" },\n", | |
" {\n", | |
" \"rel\": \"self\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/collections/noaa-emergency-response\"\n", | |
" }\n", | |
" ],\n", | |
" \"title\": \"NOAA Emergency Response Imagery\",\n", | |
" \"extent\": {\n", | |
" \"spatial\": {\n", | |
" \"bbox\": [\n", | |
" [\n", | |
" -180,\n", | |
" -90,\n", | |
" 180,\n", | |
" 90\n", | |
" ]\n", | |
" ]\n", | |
" },\n", | |
" \"temporal\": {\n", | |
" \"interval\": [\n", | |
" [\n", | |
" \"2005-01-01T00:00:00Z\",\n", | |
" \"null\"\n", | |
" ]\n", | |
" ]\n", | |
" }\n", | |
" },\n", | |
" \"license\": \"public-domain\",\n", | |
" \"description\": \"NOAA Emergency Response Imagery hosted on AWS Public Dataset.\",\n", | |
" \"stac_version\": \"1.0.0\"\n", | |
" }\n", | |
" ],\n", | |
" \"links\": [\n", | |
" {\n", | |
" \"rel\": \"root\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/\"\n", | |
" },\n", | |
" {\n", | |
" \"rel\": \"parent\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/\"\n", | |
" },\n", | |
" {\n", | |
" \"rel\": \"self\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/collections\"\n", | |
" }\n", | |
" ]\n", | |
"}\n" | |
] | |
} | |
], | |
"source": [ | |
"# Before the demo I've created a collections and some items using \n", | |
"# https://github.com/developmentseed/eoAPI/tree/master/demo/noaa\n", | |
"\n", | |
"r = httpx.get(f\"{stac_endpoint}/collections\").json()\n", | |
"\n", | |
"print(json.dumps(r, indent=4))" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 13, | |
"id": "764d5a94", | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"{\n", | |
" \"limit\": 10,\n", | |
" \"matched\": 163,\n", | |
" \"returned\": 10\n", | |
"}\n" | |
] | |
} | |
], | |
"source": [ | |
"r = httpx.get(f\"{stac_endpoint}/collections/noaa-emergency-response/items\").json()\n", | |
"\n", | |
"print(json.dumps(r[\"context\"], indent=4))" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 15, | |
"id": "b606082a", | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/html": [ | |
"<div style=\"width:100%;\"><div style=\"position:relative;width:100%;height:0;padding-bottom:60%;\"><span style=\"color:#565656\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\"<!DOCTYPE html>\n", | |
"<head> \n", | |
" <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\n", | |
" \n", | |
" <script>\n", | |
" L_NO_TOUCH = false;\n", | |
" L_DISABLE_3D = false;\n", | |
" </script>\n", | |
" \n", | |
" <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\n", | |
" <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\n", | |
" <script src="https://cdn.jsdelivr.net/npm/leaflet@1.6.0/dist/leaflet.js"></script>\n", | |
" <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\n", | |
" <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/js/bootstrap.min.js"></script>\n", | |
" <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\n", | |
" <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.6.0/dist/leaflet.css"/>\n", | |
" <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css"/>\n", | |
" <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap-theme.min.css"/>\n", | |
" <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.6.3/css/font-awesome.min.css"/>\n", | |
" <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\n", | |
" <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\n", | |
" \n", | |
" <meta name="viewport" content="width=device-width,\n", | |
" initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\n", | |
" <style>\n", | |
" #map_e221fd1cfd80f38784d275226e4223ca {\n", | |
" position: relative;\n", | |
" width: 100.0%;\n", | |
" height: 100.0%;\n", | |
" left: 0.0%;\n", | |
" top: 0.0%;\n", | |
" }\n", | |
" </style>\n", | |
" \n", | |
"</head>\n", | |
"<body> \n", | |
" \n", | |
" <div class="folium-map" id="map_e221fd1cfd80f38784d275226e4223ca" ></div>\n", | |
" \n", | |
"</body>\n", | |
"<script> \n", | |
" \n", | |
" var map_e221fd1cfd80f38784d275226e4223ca = L.map(\n", | |
" "map_e221fd1cfd80f38784d275226e4223ca",\n", | |
" {\n", | |
" center: [36.162499999999994, -86.225],\n", | |
" crs: L.CRS.EPSG3857,\n", | |
" zoom: 8,\n", | |
" zoomControl: true,\n", | |
" preferCanvas: false,\n", | |
" }\n", | |
" );\n", | |
"\n", | |
" \n", | |
"\n", | |
" \n", | |
" \n", | |
" var tile_layer_ce38462837a103516ab0932339dc2246 = L.tileLayer(\n", | |
" "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\n", | |
" {"attribution": "Data by \\u0026copy; \\u003ca href=\\"http://openstreetmap.org\\"\\u003eOpenStreetMap\\u003c/a\\u003e, under \\u003ca href=\\"http://www.openstreetmap.org/copyright\\"\\u003eODbL\\u003c/a\\u003e.", "detectRetina": false, "maxNativeZoom": 18, "maxZoom": 18, "minZoom": 0, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\n", | |
" ).addTo(map_e221fd1cfd80f38784d275226e4223ca);\n", | |
" \n", | |
" \n", | |
" function geo_json_ee5c3b4ea4cc5f3c5f582aa46941df3d_styler(feature) {\n", | |
" switch(feature.id) {\n", | |
" default:\n", | |
" return {"dashArray": "1", "fillOpacity": 0, "opacity": 1, "weight": 1};\n", | |
" }\n", | |
" }\n", | |
"\n", | |
" function geo_json_ee5c3b4ea4cc5f3c5f582aa46941df3d_onEachFeature(feature, layer) {\n", | |
" layer.on({\n", | |
" });\n", | |
" };\n", | |
" var geo_json_ee5c3b4ea4cc5f3c5f582aa46941df3d = L.geoJson(null, {\n", | |
" onEachFeature: geo_json_ee5c3b4ea4cc5f3c5f582aa46941df3d_onEachFeature,\n", | |
" \n", | |
" style: geo_json_ee5c3b4ea4cc5f3c5f582aa46941df3d_styler,\n", | |
" });\n", | |
"\n", | |
" function geo_json_ee5c3b4ea4cc5f3c5f582aa46941df3d_add (data) {\n", | |
" geo_json_ee5c3b4ea4cc5f3c5f582aa46941df3d\n", | |
" .addData(data)\n", | |
" .addTo(map_e221fd1cfd80f38784d275226e4223ca);\n", | |
" }\n", | |
" geo_json_ee5c3b4ea4cc5f3c5f582aa46941df3d_add({"features": [{"geometry": {"coordinates": [[[-87.0251, 36.0999], [-85.4249, 36.0999], [-85.4249, 36.2251], [-87.0251, 36.2251], [-87.0251, 36.0999]]], "type": "Polygon"}, "id": "0", "type": "Feature"}], "type": "FeatureCollection"});\n", | |
"\n", | |
" \n", | |
"</script>\" style=\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>" | |
], | |
"text/plain": [ | |
"<folium.folium.Map at 0x107c61910>" | |
] | |
}, | |
"execution_count": 15, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"# bounds of the noaa-eri-nashville2020.json items\n", | |
"bounds = (-87.0251, 36.0999, -85.4249, 36.2251)\n", | |
"\n", | |
"poly = Polygon.from_bounds(*bounds)\n", | |
"geojson = Feature(geometry=poly).dict(exclude_none=True)\n", | |
"\n", | |
"m = Map(\n", | |
" tiles=\"OpenStreetMap\",\n", | |
" location=((bounds[1] + bounds[3]) / 2,(bounds[0] + bounds[2]) / 2),\n", | |
" zoom_start=8\n", | |
")\n", | |
"\n", | |
"geo_json = GeoJson(\n", | |
" data=geojson,\n", | |
" style_function=lambda x: {\n", | |
" 'opacity': 1, 'dashArray': '1', 'fillOpacity': 0, 'weight': 1\n", | |
" },\n", | |
")\n", | |
"geo_json.add_to(m)\n", | |
"m" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 18, | |
"id": "214b5060", | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"{\n", | |
" \"id\": \"20200307aC0862400w361330\",\n", | |
" \"bbox\": [\n", | |
" -86.4001,\n", | |
" 36.1999,\n", | |
" -86.3749,\n", | |
" 36.2251\n", | |
" ],\n", | |
" \"type\": \"Feature\",\n", | |
" \"links\": [\n", | |
" {\n", | |
" \"rel\": \"collection\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/collections/noaa-emergency-response\"\n", | |
" },\n", | |
" {\n", | |
" \"rel\": \"parent\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/collections/noaa-emergency-response\"\n", | |
" },\n", | |
" {\n", | |
" \"rel\": \"root\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/\"\n", | |
" },\n", | |
" {\n", | |
" \"rel\": \"self\",\n", | |
" \"type\": \"application/geo+json\",\n", | |
" \"href\": \"http://127.0.0.1:8081/collections/noaa-emergency-response/items/20200307aC0862400w361330\"\n", | |
" }\n", | |
" ],\n", | |
" \"assets\": {\n", | |
" \"cog\": {\n", | |
" \"href\": \"https://noaa-eri-pds.s3.us-east-1.amazonaws.com/2020_Nashville_Tornado/20200307a_RGB/20200307aC0862400w361330n.tif\",\n", | |
" \"type\": \"image/tiff; application=geotiff; profile=cloud-optimized\"\n", | |
" }\n", | |
" },\n", | |
" \"geometry\": {\n", | |
" \"type\": \"Polygon\",\n", | |
" \"coordinates\": [\n", | |
" [\n", | |
" [\n", | |
" -86.4001,\n", | |
" 36.2251\n", | |
" ],\n", | |
" [\n", | |
" -86.4001,\n", | |
" 36.1999\n", | |
" ],\n", | |
" [\n", | |
" -86.3749,\n", | |
" 36.1999\n", | |
" ],\n", | |
" [\n", | |
" -86.3749,\n", | |
" 36.2251\n", | |
" ],\n", | |
" [\n", | |
" -86.4001,\n", | |
" 36.2251\n", | |
" ]\n", | |
" ]\n", | |
" ]\n", | |
" },\n", | |
" \"collection\": \"noaa-emergency-response\",\n", | |
" \"properties\": {\n", | |
" \"event\": \"Nashville Tornado\",\n", | |
" \"datetime\": \"2020-03-11T00:00:01Z\"\n", | |
" },\n", | |
" \"stac_version\": \"1.0.0\",\n", | |
" \"stac_extensions\": []\n", | |
"}\n" | |
] | |
} | |
], | |
"source": [ | |
"r = httpx.get(f\"{stac_endpoint}/collections/noaa-emergency-response/items\").json()\n", | |
"item = r[\"features\"][0]\n", | |
"\n", | |
"print(json.dumps(item, indent=4))" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 22, | |
"id": "ded4afbf", | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"{\n", | |
" \"cog\": {\n", | |
" \"bounds\": [\n", | |
" -86.4001,\n", | |
" 36.1999,\n", | |
" -86.3749,\n", | |
" 36.2251\n", | |
" ],\n", | |
" \"minzoom\": 13,\n", | |
" \"maxzoom\": 20,\n", | |
" \"band_metadata\": [\n", | |
" [\n", | |
" \"b1\",\n", | |
" {}\n", | |
" ],\n", | |
" [\n", | |
" \"b2\",\n", | |
" {}\n", | |
" ],\n", | |
" [\n", | |
" \"b3\",\n", | |
" {}\n", | |
" ]\n", | |
" ],\n", | |
" \"band_descriptions\": [\n", | |
" [\n", | |
" \"b1\",\n", | |
" \"\"\n", | |
" ],\n", | |
" [\n", | |
" \"b2\",\n", | |
" \"\"\n", | |
" ],\n", | |
" [\n", | |
" \"b3\",\n", | |
" \"\"\n", | |
" ]\n", | |
" ],\n", | |
" \"dtype\": \"uint8\",\n", | |
" \"nodata_type\": \"Mask\",\n", | |
" \"colorinterp\": [\n", | |
" \"red\",\n", | |
" \"green\",\n", | |
" \"blue\"\n", | |
" ],\n", | |
" \"height\": 18681,\n", | |
" \"driver\": \"GTiff\",\n", | |
" \"overviews\": [\n", | |
" 2,\n", | |
" 4,\n", | |
" 8,\n", | |
" 16,\n", | |
" 32,\n", | |
" 64,\n", | |
" 128\n", | |
" ],\n", | |
" \"width\": 18681,\n", | |
" \"count\": 3\n", | |
" }\n", | |
"}\n" | |
] | |
} | |
], | |
"source": [ | |
"info = httpx.get(\n", | |
" f\"{raster_endpoint}/collections/{item['collection']}/items/{item['id']}/info\",\n", | |
" params={\n", | |
" \"assets\": \"cog\",\n", | |
" }\n", | |
").json()\n", | |
"\n", | |
"print(json.dumps(info, indent=4))" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 24, | |
"id": "b689edff", | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"{'tilejson': '2.2.0', 'version': '1.0.0', 'scheme': 'xyz', 'tiles': ['http://127.0.0.1:8082/collections/noaa-emergency-response/items/20200307aC0862400w361330/tiles/WebMercatorQuad/{z}/{x}/{y}@1x?assets=cog'], 'minzoom': 13, 'maxzoom': 20, 'bounds': [-86.4001, 36.1999, -86.3749, 36.2251], 'center': [-86.38749999999999, 36.2125, 13]}\n" | |
] | |
}, | |
{ | |
"data": { | |
"text/html": [ | |
"<div style=\"width:100%;\"><div style=\"position:relative;width:100%;height:0;padding-bottom:60%;\"><span style=\"color:#565656\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\"<!DOCTYPE html>\n", | |
"<head> \n", | |
" <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\n", | |
" \n", | |
" <script>\n", | |
" L_NO_TOUCH = false;\n", | |
" L_DISABLE_3D = false;\n", | |
" </script>\n", | |
" \n", | |
" <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\n", | |
" <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\n", | |
" <script src="https://cdn.jsdelivr.net/npm/leaflet@1.6.0/dist/leaflet.js"></script>\n", | |
" <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\n", | |
" <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/js/bootstrap.min.js"></script>\n", | |
" <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\n", | |
" <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.6.0/dist/leaflet.css"/>\n", | |
" <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css"/>\n", | |
" <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap-theme.min.css"/>\n", | |
" <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.6.3/css/font-awesome.min.css"/>\n", | |
" <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\n", | |
" <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\n", | |
" \n", | |
" <meta name="viewport" content="width=device-width,\n", | |
" initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\n", | |
" <style>\n", | |
" #map_574ca01b64b36acae301581ac7bc8de5 {\n", | |
" position: relative;\n", | |
" width: 100.0%;\n", | |
" height: 100.0%;\n", | |
" left: 0.0%;\n", | |
" top: 0.0%;\n", | |
" }\n", | |
" </style>\n", | |
" \n", | |
"</head>\n", | |
"<body> \n", | |
" \n", | |
" <div class="folium-map" id="map_574ca01b64b36acae301581ac7bc8de5" ></div>\n", | |
" \n", | |
"</body>\n", | |
"<script> \n", | |
" \n", | |
" var map_574ca01b64b36acae301581ac7bc8de5 = L.map(\n", | |
" "map_574ca01b64b36acae301581ac7bc8de5",\n", | |
" {\n", | |
" center: [36.162499999999994, -86.225],\n", | |
" crs: L.CRS.EPSG3857,\n", | |
" zoom: 13,\n", | |
" zoomControl: true,\n", | |
" preferCanvas: false,\n", | |
" }\n", | |
" );\n", | |
"\n", | |
" \n", | |
"\n", | |
" \n", | |
" \n", | |
" var tile_layer_c9d7fde930c51c0b2a1f5de1436971b9 = L.tileLayer(\n", | |
" "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\n", | |
" {"attribution": "Data by \\u0026copy; \\u003ca href=\\"http://openstreetmap.org\\"\\u003eOpenStreetMap\\u003c/a\\u003e, under \\u003ca href=\\"http://www.openstreetmap.org/copyright\\"\\u003eODbL\\u003c/a\\u003e.", "detectRetina": false, "maxNativeZoom": 18, "maxZoom": 18, "minZoom": 0, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\n", | |
" ).addTo(map_574ca01b64b36acae301581ac7bc8de5);\n", | |
" \n", | |
" \n", | |
" var tile_layer_fb96cf84907dbd5ad062e4c418d6a1f0 = L.tileLayer(\n", | |
" "http://127.0.0.1:8082/collections/noaa-emergency-response/items/20200307aC0862400w361330/tiles/WebMercatorQuad/{z}/{x}/{y}@1x?assets=cog",\n", | |
" {"attribution": "Maxar", "detectRetina": false, "maxNativeZoom": 18, "maxZoom": 18, "minZoom": 0, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\n", | |
" ).addTo(map_574ca01b64b36acae301581ac7bc8de5);\n", | |
" \n", | |
"</script>\" style=\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>" | |
], | |
"text/plain": [ | |
"<folium.folium.Map at 0x11f4708b0>" | |
] | |
}, | |
"execution_count": 24, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"info = httpx.get(\n", | |
" f\"{raster_endpoint}/collections/{item['collection']}/items/{item['id']}/info\",\n", | |
" params={\n", | |
" \"assets\": \"cog\",\n", | |
" }\n", | |
").json()[\"cog\"]\n", | |
"\n", | |
"r = httpx.get(\n", | |
" f\"{raster_endpoint}/collections/{item['collection']}/items/{item['id']}/tilejson.json\",\n", | |
" params = (\n", | |
" (\"assets\", \"cog\"),\n", | |
" (\"minzoom\", info[\"minzoom\"]),\n", | |
" (\"maxzoom\", info[\"maxzoom\"]),\n", | |
" )\n", | |
").json()\n", | |
"print(r)\n", | |
"\n", | |
"m = Map(\n", | |
" location=((bounds[1] + bounds[3]) / 2,(bounds[0] + bounds[2]) / 2),\n", | |
" zoom_start=r[\"minzoom\"]\n", | |
")\n", | |
"\n", | |
"aod_layer = TileLayer(\n", | |
" tiles=r[\"tiles\"][0],\n", | |
" opacity=1,\n", | |
" attr=\"Maxar\"\n", | |
")\n", | |
"aod_layer.add_to(m)\n", | |
"m" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 25, | |
"id": "ee00d9e0", | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"{\n", | |
" \"searchid\": \"6d436413d0eed760acc2f6bd16ca77a5\",\n", | |
" \"links\": [\n", | |
" {\n", | |
" \"rel\": \"metadata\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8082/mosaic/6d436413d0eed760acc2f6bd16ca77a5/info\"\n", | |
" },\n", | |
" {\n", | |
" \"rel\": \"tilejson\",\n", | |
" \"type\": \"application/json\",\n", | |
" \"href\": \"http://127.0.0.1:8082/mosaic/6d436413d0eed760acc2f6bd16ca77a5/tilejson.json\"\n", | |
" }\n", | |
" ]\n", | |
"}\n" | |
] | |
} | |
], | |
"source": [ | |
"bounds = (-87.0251, 36.0999, -85.4249, 36.2251)\n", | |
"\n", | |
"# STAC API /search request\n", | |
"search_request = {\n", | |
" # Filter collection\n", | |
" \"collections\": [\"noaa-emergency-response\"],\n", | |
" # limit bounds of the known items (note: the bbox will also be used in the tilejson response)\n", | |
" \"bbox\": bounds,\n", | |
" \"filter-lang\": \"cql-json\",\n", | |
"}\n", | |
"\n", | |
"r = httpx.post(\n", | |
" f\"{raster_endpoint}/mosaic/register\", json=search_request,\n", | |
").json()\n", | |
"\n", | |
"searchid = response[\"searchid\"]\n", | |
"\n", | |
"print(json.dumps(r, indent=4))" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 29, | |
"id": "eb61ab7b", | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"{\n", | |
" \"tilejson\": \"2.2.0\",\n", | |
" \"name\": \"6d436413d0eed760acc2f6bd16ca77a5\",\n", | |
" \"version\": \"1.0.0\",\n", | |
" \"scheme\": \"xyz\",\n", | |
" \"tiles\": [\n", | |
" \"http://127.0.0.1:8082/mosaic/6d436413d0eed760acc2f6bd16ca77a5/tiles/WebMercatorQuad/{z}/{x}/{y}@1x?assets=cog\"\n", | |
" ],\n", | |
" \"minzoom\": 13,\n", | |
" \"maxzoom\": 20,\n", | |
" \"bounds\": [\n", | |
" -87.0251,\n", | |
" 36.0999,\n", | |
" -85.4249,\n", | |
" 36.2251\n", | |
" ],\n", | |
" \"center\": [\n", | |
" -86.225,\n", | |
" 36.162499999999994,\n", | |
" 13\n", | |
" ]\n", | |
"}\n" | |
] | |
} | |
], | |
"source": [ | |
"r = httpx.get(\n", | |
" f\"{raster_endpoint}/mosaic/{searchid}/tilejson.json?assets=cog\",\n", | |
" params={\n", | |
" \"minzoom\": 13,\n", | |
" \"maxzoom\": 20,\n", | |
" }\n", | |
").json()\n", | |
"print(json.dumps(r, indent=4))" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 31, | |
"id": "c49c2e83", | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/html": [ | |
"<div style=\"width:100%;\"><div style=\"position:relative;width:100%;height:0;padding-bottom:60%;\"><span style=\"color:#565656\">Make this Notebook Trusted to load map: File -> Trust Notebook</span><iframe srcdoc=\"<!DOCTYPE html>\n", | |
"<head> \n", | |
" <meta http-equiv="content-type" content="text/html; charset=UTF-8" />\n", | |
" \n", | |
" <script>\n", | |
" L_NO_TOUCH = false;\n", | |
" L_DISABLE_3D = false;\n", | |
" </script>\n", | |
" \n", | |
" <style>html, body {width: 100%;height: 100%;margin: 0;padding: 0;}</style>\n", | |
" <style>#map {position:absolute;top:0;bottom:0;right:0;left:0;}</style>\n", | |
" <script src="https://cdn.jsdelivr.net/npm/leaflet@1.6.0/dist/leaflet.js"></script>\n", | |
" <script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>\n", | |
" <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/js/bootstrap.min.js"></script>\n", | |
" <script src="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.js"></script>\n", | |
" <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/leaflet@1.6.0/dist/leaflet.css"/>\n", | |
" <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css"/>\n", | |
" <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap-theme.min.css"/>\n", | |
" <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.6.3/css/font-awesome.min.css"/>\n", | |
" <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/Leaflet.awesome-markers/2.0.2/leaflet.awesome-markers.css"/>\n", | |
" <link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/python-visualization/folium/folium/templates/leaflet.awesome.rotate.min.css"/>\n", | |
" \n", | |
" <meta name="viewport" content="width=device-width,\n", | |
" initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />\n", | |
" <style>\n", | |
" #map_1b608c413681ddc3cf6b27bbc4d133b4 {\n", | |
" position: relative;\n", | |
" width: 100.0%;\n", | |
" height: 100.0%;\n", | |
" left: 0.0%;\n", | |
" top: 0.0%;\n", | |
" }\n", | |
" </style>\n", | |
" \n", | |
"</head>\n", | |
"<body> \n", | |
" \n", | |
" <div class="folium-map" id="map_1b608c413681ddc3cf6b27bbc4d133b4" ></div>\n", | |
" \n", | |
"</body>\n", | |
"<script> \n", | |
" \n", | |
" var map_1b608c413681ddc3cf6b27bbc4d133b4 = L.map(\n", | |
" "map_1b608c413681ddc3cf6b27bbc4d133b4",\n", | |
" {\n", | |
" center: [36.162499999999994, -86.225],\n", | |
" crs: L.CRS.EPSG3857,\n", | |
" zoom: 13,\n", | |
" zoomControl: true,\n", | |
" preferCanvas: false,\n", | |
" }\n", | |
" );\n", | |
"\n", | |
" \n", | |
"\n", | |
" \n", | |
" \n", | |
" var tile_layer_716c60a5f8ddac8b595eb29b5783e6ba = L.tileLayer(\n", | |
" "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png",\n", | |
" {"attribution": "Data by \\u0026copy; \\u003ca href=\\"http://openstreetmap.org\\"\\u003eOpenStreetMap\\u003c/a\\u003e, under \\u003ca href=\\"http://www.openstreetmap.org/copyright\\"\\u003eODbL\\u003c/a\\u003e.", "detectRetina": false, "maxNativeZoom": 18, "maxZoom": 18, "minZoom": 0, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\n", | |
" ).addTo(map_1b608c413681ddc3cf6b27bbc4d133b4);\n", | |
" \n", | |
" \n", | |
" function geo_json_aff5d91052367ef1efff12bc7baaf0b4_styler(feature) {\n", | |
" switch(feature.id) {\n", | |
" default:\n", | |
" return {"dashArray": "1", "fillOpacity": 0, "opacity": 1, "weight": 1};\n", | |
" }\n", | |
" }\n", | |
"\n", | |
" function geo_json_aff5d91052367ef1efff12bc7baaf0b4_onEachFeature(feature, layer) {\n", | |
" layer.on({\n", | |
" });\n", | |
" };\n", | |
" var geo_json_aff5d91052367ef1efff12bc7baaf0b4 = L.geoJson(null, {\n", | |
" onEachFeature: geo_json_aff5d91052367ef1efff12bc7baaf0b4_onEachFeature,\n", | |
" \n", | |
" style: geo_json_aff5d91052367ef1efff12bc7baaf0b4_styler,\n", | |
" });\n", | |
"\n", | |
" function geo_json_aff5d91052367ef1efff12bc7baaf0b4_add (data) {\n", | |
" geo_json_aff5d91052367ef1efff12bc7baaf0b4\n", | |
" .addData(data)\n", | |
" .addTo(map_1b608c413681ddc3cf6b27bbc4d133b4);\n", | |
" }\n", | |
" geo_json_aff5d91052367ef1efff12bc7baaf0b4_add({"features": [{"geometry": {"coordinates": [[[-87.0251, 36.0999], [-85.4249, 36.0999], [-85.4249, 36.2251], [-87.0251, 36.2251], [-87.0251, 36.0999]]], "type": "Polygon"}, "id": "0", "type": "Feature"}], "type": "FeatureCollection"});\n", | |
"\n", | |
" \n", | |
" \n", | |
" var tile_layer_274ddc35a0b13ba07786a80846009426 = L.tileLayer(\n", | |
" "http://127.0.0.1:8082/mosaic/6d436413d0eed760acc2f6bd16ca77a5/tiles/WebMercatorQuad/{z}/{x}/{y}@1x?assets=cog",\n", | |
" {"attribution": "esri-noaa", "detectRetina": false, "maxNativeZoom": 18, "maxZoom": 18, "minZoom": 0, "noWrap": false, "opacity": 1, "subdomains": "abc", "tms": false}\n", | |
" ).addTo(map_1b608c413681ddc3cf6b27bbc4d133b4);\n", | |
" \n", | |
"</script>\" style=\"position:absolute;width:100%;height:100%;left:0;top:0;border:none !important;\" allowfullscreen webkitallowfullscreen mozallowfullscreen></iframe></div></div>" | |
], | |
"text/plain": [ | |
"<folium.folium.Map at 0x11f38c5e0>" | |
] | |
}, | |
"execution_count": 31, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"bounds = r[\"bounds\"]\n", | |
"\n", | |
"m = Map(\n", | |
" location=(r[\"center\"][1], r[\"center\"][0]),\n", | |
" zoom_start=r[\"minzoom\"]\n", | |
")\n", | |
"\n", | |
"poly = Polygon.from_bounds(*bounds)\n", | |
"geojson = Feature(geometry=poly).dict(exclude_none=True)\n", | |
"\n", | |
"m = Map(\n", | |
" tiles=\"OpenStreetMap\",\n", | |
" location=((bounds[1] + bounds[3]) / 2,(bounds[0] + bounds[2]) / 2),\n", | |
" zoom_start=13\n", | |
")\n", | |
"\n", | |
"geo_json = GeoJson(\n", | |
" data=geojson,\n", | |
" style_function=lambda x: {\n", | |
" 'opacity': 1, 'dashArray': '1', 'fillOpacity': 0, 'weight': 1\n", | |
" },\n", | |
")\n", | |
"geo_json.add_to(m)\n", | |
"m\n", | |
"\n", | |
"aod_layer = TileLayer(\n", | |
" tiles=r[\"tiles\"][0],\n", | |
" opacity=1,\n", | |
" attr=\"esri-noaa\"\n", | |
")\n", | |
"aod_layer.add_to(m)\n", | |
"m" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"id": "439505f7", | |
"metadata": {}, | |
"outputs": [], | |
"source": [] | |
} | |
], | |
"metadata": { | |
"kernelspec": { | |
"display_name": "Python 3 (ipykernel)", | |
"language": "python", | |
"name": "python3" | |
}, | |
"language_info": { | |
"codemirror_mode": { | |
"name": "ipython", | |
"version": 3 | |
}, | |
"file_extension": ".py", | |
"mimetype": "text/x-python", | |
"name": "python", | |
"nbconvert_exporter": "python", | |
"pygments_lexer": "ipython3", | |
"version": "3.9.13" | |
} | |
}, | |
"nbformat": 4, | |
"nbformat_minor": 5 | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment