Created
March 10, 2019 11:15
-
-
Save wridhdhi/db962e00522686bf03424236042700b7 to your computer and use it in GitHub Desktop.
Advanced Python and OOP
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"cells": [ | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"# 1.OOP Structure in Python" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"An object has two characteristics:\n", | |
"\n", | |
"- attributes(**variables**)\n", | |
"- behavior(**functions**)\n", | |
"\n", | |
"A class is a blueprint for the object.\n", | |
"\n", | |
"An object (instance) is an **instance** of a class. When class is defined, only the description for the object is defined, no memory allocation done, objects take up memory.\n", | |
"\n", | |
"```python\n", | |
"class NewClass:\n", | |
" '''This is a docstring. I have created a new class'''\n", | |
" pass\n", | |
"\n", | |
"object=NewClass()\n", | |
"```\n", | |
"\n", | |
"- access docstring by `print(MyClass.__doc__)`\n", | |
"- `__init__()` is a **Constructor**\n", | |
"- since Objects are memory allocated objects, they can be deleted usin `del obj` statement.\n", | |
"- Similarly you can delete object attributes `del obj.var1`, class attributes `del __class__.var` and even methods() `del className.method`" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 1, | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"This is a BIRD Class\n", | |
"Blu is a bird\n", | |
"Woo is also a bird\n", | |
"Blu is 10 years old\n", | |
"Woo is 15 years old\n", | |
"Blu sings 'Happy'\n" | |
] | |
} | |
], | |
"source": [ | |
"class Parrot:\n", | |
" '''This is a BIRD Class'''\n", | |
"\n", | |
"\n", | |
" species = \"bird\" # this is a class variable,common to all objects of the class\n", | |
"\n", | |
" def __init__(self, name, age): #this is a initation Constructor\n", | |
" self.name = name\n", | |
" self.age = age\n", | |
" \n", | |
" \n", | |
" def sing(self, song):\n", | |
" return \"{} sings {}\".format(self.name, song)\n", | |
"\n", | |
"# -----------------------------------------------------------------------\n", | |
"\n", | |
"# CREATE TWO NEW OBJECTS OF THE CLASS\n", | |
"blu = Parrot(\"Blu\", 10)\n", | |
"woo = Parrot(\"Woo\", 15)\n", | |
"\n", | |
"print(blu.__doc__)\n", | |
"\n", | |
"# access the class attributes NOTE HERE : \"__class__.species\" same as \"obj.species\"\n", | |
"print(\"Blu is a {}\".format(blu.__class__.species))\n", | |
"print(\"Woo is also a {}\".format(woo.species))\n", | |
"\n", | |
"# access the instance attributes\n", | |
"print(\"{} is {} years old\".format( blu.name, blu.age))\n", | |
"print(\"{} is {} years old\".format( woo.name, woo.age))\n", | |
"\n", | |
"\n", | |
"# call our instance methods\n", | |
"print(blu.sing(\"'Happy'\"))" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"---\n", | |
"| head 1 | head 2 |\n", | |
"|---|---|\n", | |
"|body1|body2|\n", | |
"\n", | |
"## Inheritance\n", | |
"\n", | |
"\n", | |
"Inheritance is a way of creating new class for using details of **MOTHER** class without modifying it. \n", | |
"\n", | |
"The newly formed class is a derived class (or **child class**). Similarly, the MOTHER class is a **base class** (or parent \n", | |
"class).\n", | |
"```python\n", | |
"class BaseClass:\n", | |
" Body of base class\n", | |
"class DerivedClass(BaseClass):\n", | |
" Body of derived class\n", | |
" ```\n", | |
"\n", | |
"- use `super().variable/method()` to access parent variables/methods\n", | |
"- use `ParentClass.variable/method()` to access parent variables/methods\n", | |
"- creating a method() overrides a method() of **same name** in the parent class.\n", | |
"- wo built-in functions `isinstance(obj,Classname)` \n", | |
"- and USE `issubclass(subClass,ParentClass)` are used to check inheritances. \n", | |
"- for huge multilevel inheritances use `classname.mro()` to understand levelling *MRO of a class can be viewed as the `classname.__mro__` attribute or `mro()` method. The former returns a tuple while latter returns a list.* [more](https://www.programiz.com/python-programming/multiple-inheritance)\n", | |
"\n", | |
"\n", | |
"\n", | |
"```python\n", | |
"# parent class\n", | |
"class Bird(object): #<-------- this is of type object\n", | |
" \n", | |
" def __init__(self):\n", | |
" print(\"Bird is ready\")\n", | |
"\n", | |
" def whoisThis(self):\n", | |
" print(\"Bird\")\n", | |
"\n", | |
" def swim(self):\n", | |
" print(\"Swim faster\")\n", | |
"\n", | |
"# child class\n", | |
"class Penguin(Bird):#<-------this is of type parent\n", | |
"\n", | |
" def __init__(self):\n", | |
" # call super() function\n", | |
" super().__init__()\n", | |
" print(\"Penguin is ready\")\n", | |
"\n", | |
" def whoisThis(self): #This over rides the Parent class function. \n", | |
" print(\"Penguin\")\n", | |
"\n", | |
" def run(self):\n", | |
" print(\"Run faster\")\n", | |
" \n", | |
"``` \n", | |
"### Multiple Inheritance\n", | |
"```python\n", | |
"class Base1:\n", | |
" pass\n", | |
"\n", | |
"class Base2:\n", | |
" pass\n", | |
"\n", | |
"class MultiDerived(Base1, Base2):\n", | |
" pass\n", | |
"\n", | |
"```\n", | |
"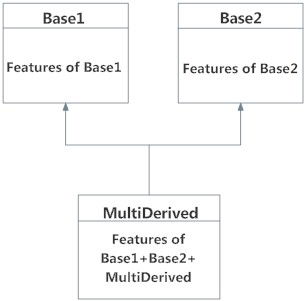\n", | |
"### Multilevel Inheritance\n", | |
"\n", | |
"```python\n", | |
"class Base:\n", | |
" pass\n", | |
"\n", | |
"class Derived1(Base):\n", | |
" pass\n", | |
"\n", | |
"class Derived2(Derived1):\n", | |
" pass\n", | |
" ```\n", | |
"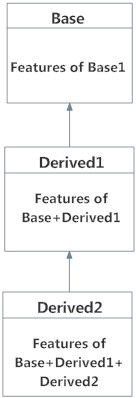" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"## Encapsulation\n", | |
"Using OOP in Python, we can restrict access to methods and variables. \n", | |
"\n", | |
"This prevent data from **direct modification** which is called **encapsulation**. \n", | |
"\n", | |
"- we denote private attribute using underscore as prefix i.e single “ _ “ or double “__“.\n", | |
"- `__maxprice` as private attributes.\n", | |
"- Can only be changes `setter()` functions" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 2, | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"Selling Price: 900\n", | |
"Selling Price: 900\n", | |
"Selling Price: 1000\n" | |
] | |
} | |
], | |
"source": [ | |
"class Computer:\n", | |
"\n", | |
" def __init__(self):\n", | |
" self.__maxprice = 900 #PRIVATE\n", | |
"\n", | |
" def sell(self):\n", | |
" print(\"Selling Price: {}\".format(self.__maxprice))\n", | |
"\n", | |
" def setMaxPrice(self, price):\n", | |
" self.__maxprice = price\n", | |
"\n", | |
"c = Computer()\n", | |
"c.sell()\n", | |
"\n", | |
"\n", | |
"c.__maxprice = 1000 #THIS WONT/Can't CHANGE since its private\n", | |
"c.sell()\n", | |
"\n", | |
"\n", | |
"c.setMaxPrice(1000) #SETTER CAN CHANGE ONLY\n", | |
"c.sell()" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"## Polymorphism\n", | |
"Polymorphism is an ability (in OOP) to use common interface for multiple form (data types)." | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 3, | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"Parrot can fly\n", | |
"Penguin can't fly\n" | |
] | |
} | |
], | |
"source": [ | |
"\n", | |
"class Parrot:\n", | |
"\n", | |
" def fly(self):\n", | |
" print(\"Parrot can fly\")\n", | |
" \n", | |
" def swim(self):\n", | |
" print(\"Parrot can't swim\")\n", | |
"\n", | |
"class Penguin:\n", | |
"\n", | |
" def fly(self):\n", | |
" print(\"Penguin can't fly\")\n", | |
" \n", | |
" def swim(self):\n", | |
" print(\"Penguin can swim\")\n", | |
"#------------------------------------------------------------------------\n", | |
"# common interface\n", | |
"def flying_test(bird):\n", | |
" bird.fly()\n", | |
"#------------------------------------------------------------------------\n", | |
"#instantiate objects\n", | |
"blu = Parrot()\n", | |
"peggy = Penguin()\n", | |
"\n", | |
"# passing the object\n", | |
"flying_test(blu)\n", | |
"flying_test(peggy)" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"##### Deleting Objects\n", | |
"When we do `obj = className(1,3)`, a new instance object is created in memory and the name c1 binds with it.\n", | |
"\n", | |
"On the command del c1, this binding is removed and the name c1 is deleted from the corresponding namespace. The object however continues to exist in memory and if **no other name is bound to it, it is later automatically destroyed.**" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"### OperatorOverloading\n", | |
"\n", | |
"- rewrite the special functions `def ___mul___(self):` to see what is done when two objects are **multiplied** or whatever.\n", | |
"- **Remmember to return `ClassName(variables)`** i.e return an object of type `ClassName(parameters)` <- this is an object\n", | |
"\n", | |
"```python\n", | |
"class Point:\n", | |
" def __init__(self, x = 0, y = 0):\n", | |
" self.x = x\n", | |
" self.y = y\n", | |
" \n", | |
" def __str__(self):\n", | |
" return \"({0},{1})\".format(self.x,self.y)\n", | |
" \n", | |
" def __add__(self,other):\n", | |
" x = self.x + other.x\n", | |
" y = self.y + other.y\n", | |
" return Point(x,y)\n", | |
" ```" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"---\n", | |
"\n", | |
"\n", | |
"# 2.Extra Tricks\n", | |
"\n", | |
"\n", | |
"1. `sides = [i for i in range(no_of_sides)]` #to fill an array in one line\n", | |
"\n", | |
"2. `sides = [float(input(\"Enter side \"+str(i+1)+\" : \")) for i in range(self.n)]` #to take inputs in one line\n", | |
"\n", | |
"3. Using `enumerate(list,startIndex)` --this loops over the items in a list with the index example below <a id='another_cell'>Here</a> A new built-in function, enumerate(), will make certain loops a bit clearer. enumerate(thing), where thing is either an iterator or a sequence, returns a iterator that will return `(0, thing[0]), (1, thing[1]), (2, thing[2])`, and so forth.\n", | |
"\n", | |
"4. **Multiple iteration** in **FOR loops**\n", | |
"using<br> `for a,b in dict.items()` <br> `for x,y in enumerate(list)` <br>\n", | |
"\n", | |
"\n" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 4, | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"data": { | |
"text/plain": [ | |
"[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19]" | |
] | |
}, | |
"execution_count": 4, | |
"metadata": {}, | |
"output_type": "execute_result" | |
} | |
], | |
"source": [ | |
"\n", | |
"sides = [i for i in range(20)]\n", | |
"sides" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"[Another Cell](#another_cell)" | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": 5, | |
"metadata": {}, | |
"outputs": [ | |
{ | |
"name": "stdout", | |
"output_type": "stream", | |
"text": [ | |
"1 apple\n", | |
"2 banana\n", | |
"3 grapes\n", | |
"4 pear\n" | |
] | |
} | |
], | |
"source": [ | |
"#USe of Enumerate\n", | |
"\n", | |
"my_list = ['apple', 'banana', 'grapes', 'pear']\n", | |
"for c, value in enumerate(my_list, 1): #SEE the multiple iteration\n", | |
" print(c, value)" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"#### \\*args and \\**kwargs\n", | |
"\n", | |
"The syntax is the * and **. The names *args and **kwargs are only by convention but there's no hard requirement to use them.\n", | |
"1. Use *args when you're **not sure how many arguments** might be passed to your function, i.e. it allows you pass an arbitrary number of arguments to your function. For example:\n", | |
"\n", | |
" ```python\n", | |
" >>> def print_everything(*args):\n", | |
" for count, thing in enumerate(args):\n", | |
" ... print( '{0}. {1}'.format(count, thing))\n", | |
" ...\n", | |
" >>> print_everything('apple', 'banana', 'cabbage')```\n", | |
" ```\n", | |
" 0. apple\n", | |
" 1. banana\n", | |
" 2. cabbage\n", | |
" ```\n", | |
"\n", | |
"2. Similarly, **kwargs allows you to handle named arguments that you have not defined in advance:**\n", | |
" ```python\n", | |
" >>> def table_things(**kwargs):\n", | |
" ... for name, value in kwargs.items():\n", | |
" ... print( '{0} = {1}'.format(name, value))\n", | |
" ...\n", | |
" >>> table_things(apple = 'fruit', cabbage = 'vegetable')\n", | |
" cabbage = vegetable\n", | |
" apple = fruit\n", | |
" ```\n", | |
"\n", | |
"3. You can use these along with **named arguments** too. The explicit arguments get values first and then everything else is passed to *args and **kwargs. The named arguments come first in the list.**`\n", | |
" def table_things(titlestring, **kwargs)`\n", | |
"\n", | |
"4. You can also use both in the same function definition but `*args` must occur before `**kwargs`.\n", | |
"\n", | |
"5. You can also use the * and ** syntax when calling a function. For example:\n", | |
" ```python\n", | |
" >>> def print_three_things(a, b, c):\n", | |
" ... print( 'a = {0}, b = {1}, c = {2}'.format(a,b,c))\n", | |
" ...\n", | |
" >>> mylist = ['aardvark', 'baboon', 'cat']\n", | |
" >>> print_three_things(*mylist)# <-------------- during call\n", | |
" a = aardvark, b = baboon, c = cat\n", | |
" ```\n", | |
"\n", | |
" As you can see in this case it takes the list (or tuple) of items and unpacks it. By this it matches them to the arguments in the function. \n", | |
"6. Of course, you could have a * both in the function definition and in the function call." | |
] | |
}, | |
{ | |
"cell_type": "code", | |
"execution_count": null, | |
"metadata": {}, | |
"outputs": [], | |
"source": [] | |
} | |
], | |
"metadata": { | |
"kernelspec": { | |
"display_name": "Python 3", | |
"language": "python", | |
"name": "python3" | |
}, | |
"language_info": { | |
"codemirror_mode": { | |
"name": "ipython", | |
"version": 3 | |
}, | |
"file_extension": ".py", | |
"mimetype": "text/x-python", | |
"name": "python", | |
"nbconvert_exporter": "python", | |
"pygments_lexer": "ipython3", | |
"version": "3.6.4" | |
} | |
}, | |
"nbformat": 4, | |
"nbformat_minor": 2 | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment