Tonic makes transactions fee free. With tonic, there is no need to buy tokens to use DAO DAO.
To use Tonic, a user signs a message and submits that message to a third party, the executor. The executor then collects messages and, individually or in batches, submits them to Tonic. Tonic verifies each message's signature and creates an account for each user. If a message's signature is valid, Tonic forwards the message to the signer's account, and the account executes it.
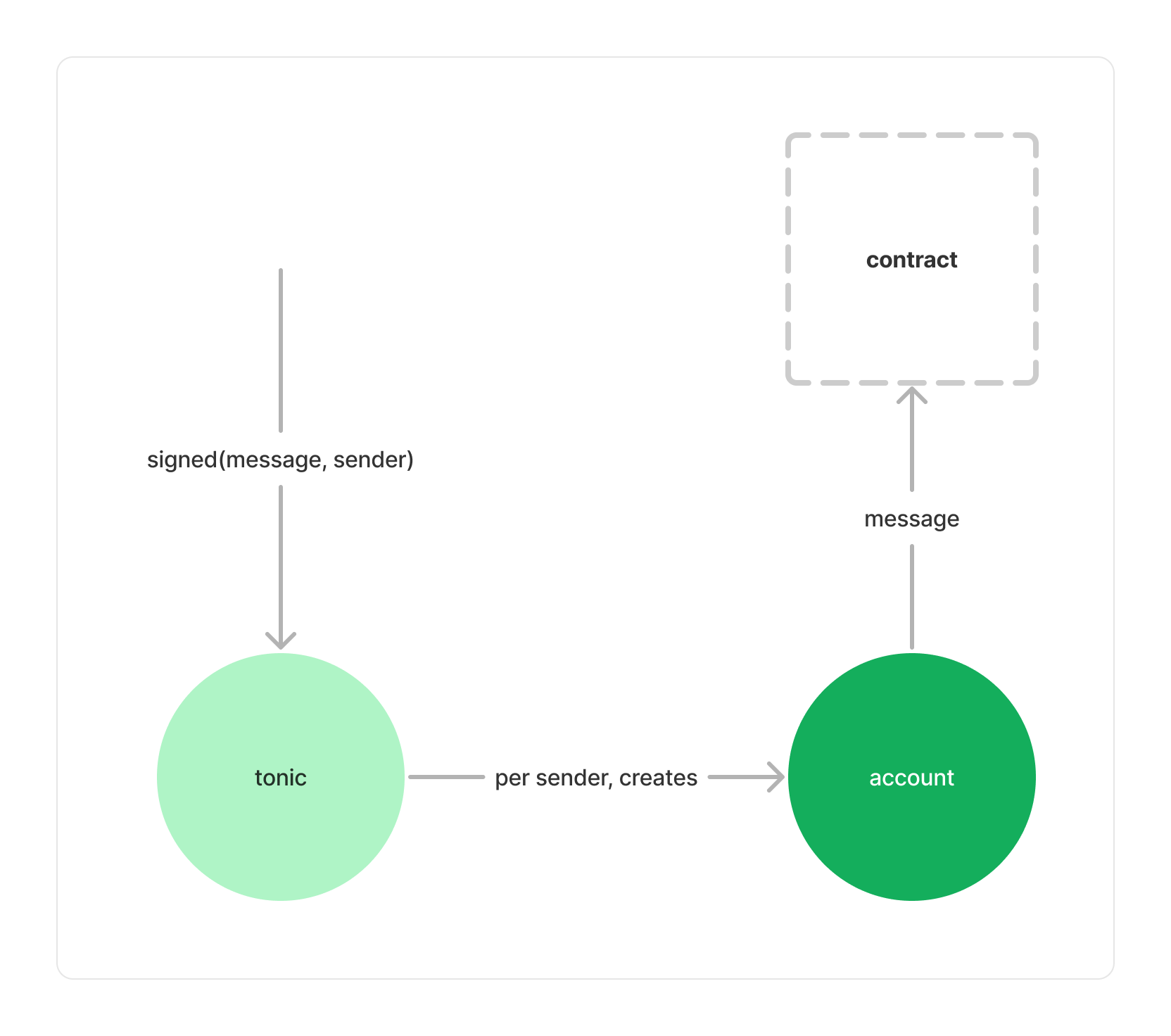
This design means, Tonic's users have two addresses: one derived from their public key, and one account with Tonic. We plan to use Tonic only for accounts created with web3auth, in which case a new account is created per-app anyway. The DAO DAO UI will display a user's tonic account address, instead of their public-key derived one.