Created
December 7, 2017 06:26
-
-
Save 345161974/0b0d32bf3f55e5ba0c34c45efcbf78c5 to your computer and use it in GitHub Desktop.
Vue.js 2.x ToDoList
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!DOCTYPE html> | |
<html lang="en"> | |
<head> | |
<meta charset="UTF-8"> | |
<title>vue.js todo list</title> | |
<script src="https://unpkg.com/vue"></script> | |
<style type="text/css"> | |
#box { width: 350px; margin: 30px auto; background: #eee; padding: 30px 50px; } | |
li { list-style-type: none; } | |
ul { padding-left: 0; } | |
.add { width: 200px; height: 20px; margin-right: 10px; } | |
.span1 {color: skyblue;} | |
.finish {color: #ccc; text-decoration: line-through;} | |
</style> | |
</head> | |
<body> | |
<div id="box"> | |
<h1>任务列表</h1> | |
<p>任务总数: {{ arr.length}} ;还有: {{ choose() }} 未完成; 【<span class="span1" @click='finish()'>清除完成的任务</span>】</p> | |
<ul> | |
<li v-for="(item,index) in arr" v-bind:class='{finish: item.bol}'> | |
<input type="checkbox" v-model='item.bol'></input> | |
<span v-text='item.des' v-show='!item.edit' @click='edit(index)'></span> | |
<input type="text" v-model='item.des' v-show='item.edit' v-on:keyup.13='item.edit = !item.edit' @blur='item.edit = false'> | |
</li> | |
</ul> | |
<input type="text" class="add" v-model='msg' v-on:keyup.13="add"></input><button @click='add()'>添加</button> | |
</div> | |
</body> | |
<script> | |
var app = new Vue({ | |
el: '#box', | |
data: { | |
arr:[ | |
// 数据结构: | |
// des: 任务描述 | |
// bol: 任务完成标志 | |
// edit: 任务编辑标志 | |
{ des:'设计', bol:false, edit:false } | |
], | |
msg: '' | |
}, | |
methods:{ | |
// 添加 | |
add: function() { | |
if (this.msg == '') { | |
return; | |
} | |
this.arr.push({des:this.msg, bol:false, edit: false}); | |
this.msg = '' | |
}, | |
// 返回未完成的数量 | |
choose: function() { | |
var num = 0; // 未完成的数量 | |
this.arr.forEach(function (item){ | |
if (!item.bol) { | |
num += 1; | |
} | |
}); | |
return num; | |
}, | |
// 返回未完成的任务 | |
finish: function() { | |
var temp = this.arr; | |
this.arr = [] | |
for (var i = 0; i < temp.length; i++) { | |
if (!temp[i].bol) { | |
this.arr.push(temp[i]); | |
} | |
} | |
}, | |
// 编辑 | |
edit: function(i) { | |
if (this.arr[i].bol) { | |
return; | |
} | |
this.arr[i].edit = true; | |
} | |
} | |
}); | |
</script> | |
</html> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
演示示例:
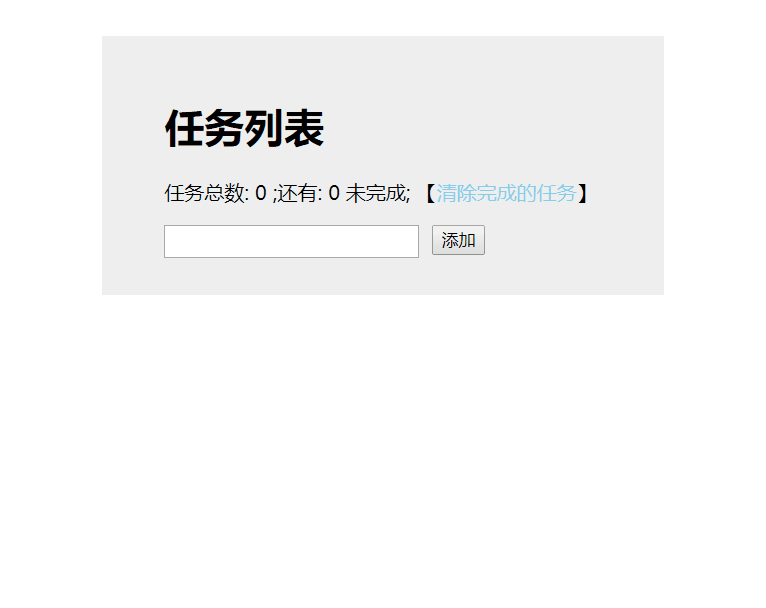