Created
October 16, 2022 16:37
-
-
Save 84adam/f25e30df7ffb9937c1ced9f0d9133d72 to your computer and use it in GitHub Desktop.
Simple Go Website
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// a simple Go-based website | |
// based on: https://www.digitalocean.com/community/tutorials/how-to-make-an-http-server-in-go | |
// with 100 bytes of CSS from: https://gist.github.com/JoeyBurzynski/617fb6201335779f8424ad9528b72c41 | |
package main | |
import ( | |
"errors" | |
"fmt" | |
"io" | |
"net/http" | |
"os" | |
) | |
func getRoot(w http.ResponseWriter, r *http.Request) { | |
fmt.Printf("got '/' request: \n--- %s ---\n", r) | |
homeString := "<html><head><style>" + | |
"html {max-width:70ch;padding:3em 1em;margin:auto;line-height:1.75;font-size:1.25em;}" + | |
"</style></head><body>" + | |
"<h1>Simple Go Website</h1>" + | |
"<h2>Welcome!</h2>" + | |
"<a href='/hello'>Hello</a>" + | |
"</body></html>" | |
io.WriteString(w, homeString) | |
} | |
func getHello(w http.ResponseWriter, r *http.Request) { | |
fmt.Printf("got '/hello' request: \n--- %s ---\n", r) | |
helloString := "<html><head><style>" + | |
"html {max-width:70ch;padding:3em 1em;margin:auto;line-height:1.75;font-size:1.25em;}" + | |
"</style></head><body>" + | |
"<h1>Simple Go Website</h1>" + | |
"<h2>Hello, HTTP!</h2>" + | |
"<a href='/'>Home</a>" + | |
"</body></html>" | |
io.WriteString(w, helloString) | |
} | |
func main() { | |
http.HandleFunc("/", getRoot) | |
http.HandleFunc("/hello", getHello) | |
err := http.ListenAndServe(":3333", nil) | |
if errors.Is(err, http.ErrServerClosed) { | |
fmt.Printf("server closed\n") | |
} else if err != nil { | |
fmt.Printf("error starting server: %s\n", err) | |
os.Exit(1) | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
example of requests printed to console:
home:
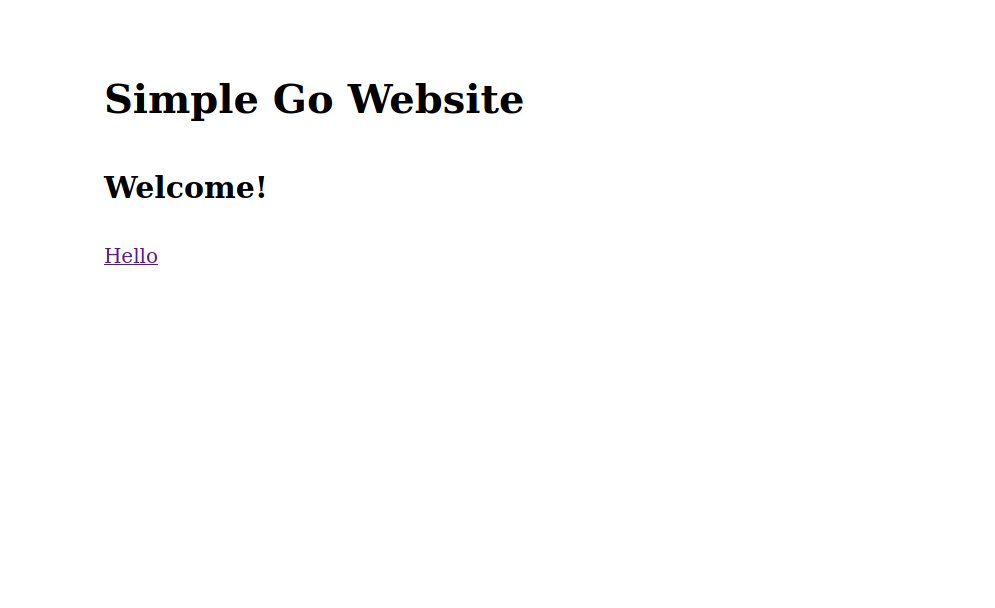
hello:
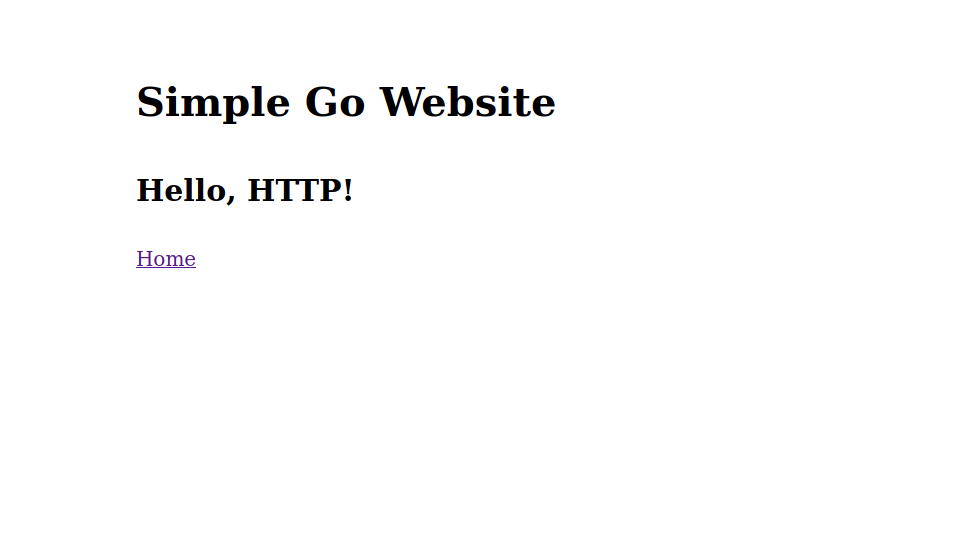