-
-
Save ALiwoto/07bc4907be9e60242df3eab97e0c905a to your computer and use it in GitHub Desktop.
class_name MyParticles2D01 | |
extends Particles2D | |
# Declare member variables here. Examples: | |
# var a = 2 | |
# var b = "text" | |
# Called when the node enters the scene tree for the first time. | |
func _ready(): | |
self.emitting = true | |
self.amount = 40 | |
#Time: | |
self.lifetime = 1.5 | |
self.one_shot = false | |
self.preprocess = 0 | |
self.speed_scale = 1 | |
self.explosiveness = 0.07 | |
self.randomness = 0 | |
self.fixed_fps = 0 | |
self.fract_delta = true | |
#Drawing: | |
self.visibility_rect = Rect2(-100, -100, 200, 200) | |
self.local_coords = true | |
self.draw_order = Particles2D.DRAW_ORDER_INDEX | |
#Process Material: | |
self.process_material = self.get_process_material() | |
# Textures: | |
self.texture = load("res://resources/floaty_64.png") | |
# Transform: | |
# self.transform = Transform2D() | |
self.z_index = 0 | |
self.z_as_relative = true | |
# Material: | |
self.material = self.get_material() | |
func get_process_material() -> Material: | |
var my_m := ParticlesMaterial.new() | |
my_m.render_priority = 0 | |
my_m.next_pass = null | |
# Time: | |
my_m.lifetime_randomness = 0 | |
# Trail: | |
my_m.trail_divisor = 1 | |
my_m.trail_size_modifier = null | |
my_m.trail_color_modifier = null | |
# Emission Shape: | |
my_m.emission_shape = ParticlesMaterial.EMISSION_SHAPE_SPHERE | |
my_m.emission_sphere_radius = 12 | |
my_m.emission_ring_axis = Vector3(0, 0, 0) | |
# Flags: | |
my_m.flag_align_y = false | |
my_m.flag_rotate_y = false | |
my_m.flag_disable_z = true | |
# Direction: | |
my_m.direction = Vector3(1, 0, 1) | |
my_m.spread = 180 | |
my_m.flatness = 0 | |
# Gravity | |
my_m.gravity = Vector3(0, -100, 0) | |
# Initial Velocity: | |
my_m.initial_velocity = 50 | |
my_m.initial_velocity_random = 0 | |
# Angular Velocity: | |
my_m.angular_velocity = 100 | |
my_m.angular_velocity_random = 1 | |
my_m.angular_velocity_curve = null | |
# Orbit Velocity: | |
my_m.orbit_velocity = 0 | |
my_m.orbit_velocity_random = 0 | |
my_m.orbit_velocity_curve = null | |
# Linear Accel | |
my_m.linear_accel = 0 | |
my_m.linear_accel_random = 0 | |
my_m.linear_accel_curve = null | |
# Radial Accel: | |
my_m.radial_accel = 0 | |
my_m.radial_accel_random = 0 | |
my_m.radial_accel_curve = null | |
# Tangential Accel: | |
my_m.tangential_accel = 0 | |
my_m.tangential_accel_random = 0 | |
my_m.tangential_accel_curve = null | |
# Damping: | |
my_m.damping = 50 | |
my_m.damping_random = 0 | |
my_m.damping_curve = null | |
# Angle: | |
my_m.angle = 360 | |
my_m.angle_random = 1 | |
my_m.angle_curve = null | |
# Scale: | |
my_m.scale = 1 | |
my_m.scale_random = 0.2 | |
my_m.scale_curve = null | |
# Color: | |
my_m.color = Color.gray | |
# Hue Variation: | |
my_m.hue_variation = 0.01 | |
my_m.hue_variation_random = 1 | |
my_m.hue_variation_curve = null | |
# Animation: | |
my_m.anim_speed = 1 | |
my_m.anim_speed_random = 0 | |
my_m.anim_speed_curve = null | |
my_m.anim_offset = 0 | |
my_m.anim_offset_random = 0 | |
my_m.anim_offset_curve = null | |
return my_m | |
func get_material() -> Material: | |
var my_m := CanvasItemMaterial.new() | |
my_m.blend_mode = CanvasItemMaterial.BLEND_MODE_ADD | |
my_m.light_mode = CanvasItemMaterial.LIGHT_MODE_NORMAL | |
my_m.particles_animation = true | |
my_m.particles_anim_h_frames = 7 | |
my_m.particles_anim_v_frames = 7 | |
my_m.particles_anim_loop = false | |
my_m.render_priority = 0 | |
my_m.next_pass = null | |
return my_m | |
# Called every frame. 'delta' is the elapsed time since the previous frame. | |
#func _process(delta): | |
# pass |
class_name MyParticles2D02 | |
extends Particles2D | |
# Declare member variables here. Examples: | |
# var a = 2 | |
# var b = "text" | |
# Called when the node enters the scene tree for the first time. | |
func _ready(): | |
self.emitting = true | |
self.amount = 20 | |
#Time: | |
self.lifetime = 1 | |
self.one_shot = false | |
self.preprocess = 0 | |
self.speed_scale = 1 | |
self.explosiveness = 0.0 | |
self.randomness = 0 | |
self.fixed_fps = 0 | |
self.fract_delta = true | |
#Drawing: | |
self.visibility_rect = Rect2(-100, -100, 200, 200) | |
self.local_coords = true | |
self.draw_order = Particles2D.DRAW_ORDER_INDEX | |
#Process Material: | |
self.process_material = self.get_process_material() | |
# Textures: | |
self.texture = load("res://resources/offset_glow.png") | |
# Transform: | |
# self.transform = Transform2D() | |
self.z_index = 0 | |
self.z_as_relative = true | |
# Material: | |
self.material = self.get_material() | |
func get_process_material() -> Material: | |
var my_m := ParticlesMaterial.new() | |
my_m.render_priority = 0 | |
my_m.next_pass = null | |
# Time: | |
my_m.lifetime_randomness = 0 | |
# Trail: | |
my_m.trail_divisor = 1 | |
my_m.trail_size_modifier = null | |
my_m.trail_color_modifier = null | |
# Emission Shape: | |
my_m.emission_shape = ParticlesMaterial.EMISSION_SHAPE_SPHERE | |
my_m.emission_sphere_radius = 5 | |
my_m.emission_ring_axis = Vector3(0, 0, 1) | |
# Flags: | |
my_m.flag_align_y = false | |
my_m.flag_rotate_y = false | |
my_m.flag_disable_z = true | |
# Direction: | |
my_m.direction = Vector3(1, 0, 0) | |
my_m.spread = 45 | |
my_m.flatness = 0 | |
# Gravity | |
my_m.gravity = Vector3(0, -150, 0) | |
# Initial Velocity: | |
my_m.initial_velocity = 0 | |
my_m.initial_velocity_random = 0 | |
# Angular Velocity: | |
my_m.angular_velocity = 1 | |
my_m.angular_velocity_random = 1 | |
my_m.angular_velocity_curve = null | |
# Orbit Velocity: | |
my_m.orbit_velocity = 0 | |
my_m.orbit_velocity_random = 0 | |
my_m.orbit_velocity_curve = null | |
# Linear Accel | |
my_m.linear_accel = 0 | |
my_m.linear_accel_random = 0 | |
my_m.linear_accel_curve = null | |
# Radial Accel: | |
my_m.radial_accel = 0 | |
my_m.radial_accel_random = 0 | |
my_m.radial_accel_curve = null | |
# Tangential Accel: | |
my_m.tangential_accel = 0 | |
my_m.tangential_accel_random = 0 | |
my_m.tangential_accel_curve = null | |
# Damping: | |
my_m.damping = 0 | |
my_m.damping_random = 0 | |
my_m.damping_curve = null | |
# Angle: | |
my_m.angle = 360 | |
my_m.angle_random = 1 | |
my_m.angle_curve = null | |
# Scale: | |
my_m.scale = 0.5 | |
my_m.scale_random = 0.3 | |
my_m.scale_curve = null | |
# Color: | |
my_m.color = Color.gray | |
# Hue Variation: | |
my_m.hue_variation = 0.0 | |
my_m.hue_variation_random = 0 | |
my_m.hue_variation_curve = null | |
# Animation: | |
my_m.anim_speed = 0 | |
my_m.anim_speed_random = 0 | |
my_m.anim_speed_curve = null | |
my_m.anim_offset = 0 | |
my_m.anim_offset_random = 0 | |
my_m.anim_offset_curve = null | |
return my_m | |
func get_material() -> Material: | |
var my_m := CanvasItemMaterial.new() | |
my_m.blend_mode = CanvasItemMaterial.BLEND_MODE_ADD | |
my_m.light_mode = CanvasItemMaterial.LIGHT_MODE_NORMAL | |
my_m.particles_animation = false | |
# my_m.particles_anim_h_frames = 7 | |
# my_m.particles_anim_v_frames = 7 | |
# my_m.particles_anim_loop = false | |
my_m.render_priority = 0 | |
my_m.next_pass = null | |
return my_m | |
# Called every frame. 'delta' is the elapsed time since the previous frame. | |
#func _process(delta): | |
# pass |
class_name MyParticles2D03 | |
extends Particles2D | |
# Declare member variables here. Examples: | |
# var a = 2 | |
# var b = "text" | |
# Called when the node enters the scene tree for the first time. | |
func _ready(): | |
self.emitting = true | |
self.amount = 6 | |
#Time: | |
self.lifetime = 1 | |
self.one_shot = false | |
self.preprocess = 0 | |
self.speed_scale = 1 | |
self.explosiveness = 0.0 | |
self.randomness = 0 | |
self.fixed_fps = 0 | |
self.fract_delta = true | |
#Drawing: | |
self.visibility_rect = Rect2(-100, -100, 200, 200) | |
self.local_coords = true | |
self.draw_order = Particles2D.DRAW_ORDER_INDEX | |
#Process Material: | |
self.process_material = self.get_process_material() | |
# Textures: | |
self.texture = load("res://resources/random_selection.png") | |
# Transform: | |
self.transform = Transform2D(0.0, Vector2(-4, -4)) | |
self.z_index = 0 | |
self.z_as_relative = true | |
# Material: | |
self.material = self.get_material() | |
func get_process_material() -> Material: | |
var my_m := ParticlesMaterial.new() | |
my_m.render_priority = 0 | |
my_m.next_pass = null | |
# Time: | |
my_m.lifetime_randomness = 0 | |
# Trail: | |
my_m.trail_divisor = 1 | |
my_m.trail_size_modifier = null | |
my_m.trail_color_modifier = null | |
# Emission Shape: | |
my_m.emission_shape = ParticlesMaterial.EMISSION_SHAPE_POINT | |
my_m.emission_ring_axis = Vector3(0, 0, 1) | |
# Flags: | |
my_m.flag_align_y = false | |
my_m.flag_rotate_y = false | |
my_m.flag_disable_z = true | |
# Direction: | |
my_m.direction = Vector3(1, 0, 0) | |
my_m.spread = 45 | |
my_m.flatness = 0 | |
# Gravity | |
my_m.gravity = Vector3(0, 0, 0) | |
# Initial Velocity: | |
my_m.initial_velocity = 0 | |
my_m.initial_velocity_random = 0 | |
# Angular Velocity: | |
my_m.angular_velocity = 0 | |
my_m.angular_velocity_random = 0 | |
my_m.angular_velocity_curve = null | |
# Orbit Velocity: | |
my_m.orbit_velocity = 0 | |
my_m.orbit_velocity_random = 0 | |
my_m.orbit_velocity_curve = null | |
# Linear Accel | |
my_m.linear_accel = 0 | |
my_m.linear_accel_random = 0 | |
my_m.linear_accel_curve = null | |
# Radial Accel: | |
my_m.radial_accel = 0 | |
my_m.radial_accel_random = 0 | |
my_m.radial_accel_curve = null | |
# Tangential Accel: | |
my_m.tangential_accel = 0 | |
my_m.tangential_accel_random = 0 | |
my_m.tangential_accel_curve = null | |
# Damping: | |
my_m.damping = 0 | |
my_m.damping_random = 0 | |
my_m.damping_curve = null | |
# Angle: | |
my_m.angle = 360 | |
my_m.angle_random = 1 | |
my_m.angle_curve = null | |
# Scale: | |
my_m.scale = 1 | |
my_m.scale_random = 0.3 | |
my_m.scale_curve = null | |
# Color: | |
my_m.color = Color.gray | |
# Hue Variation: | |
my_m.hue_variation = 0.2 | |
my_m.hue_variation_random = 1 | |
my_m.hue_variation_curve = null | |
# Animation: | |
my_m.anim_speed = 0 | |
my_m.anim_speed_random = 0 | |
my_m.anim_speed_curve = null | |
my_m.anim_offset = 1 | |
my_m.anim_offset_random = 1 | |
my_m.anim_offset_curve = null | |
return my_m | |
func get_material() -> Material: | |
var my_m := CanvasItemMaterial.new() | |
my_m.blend_mode = CanvasItemMaterial.BLEND_MODE_ADD | |
my_m.light_mode = CanvasItemMaterial.LIGHT_MODE_NORMAL | |
my_m.particles_animation = true | |
my_m.particles_anim_h_frames = 3 | |
my_m.particles_anim_v_frames = 1 | |
# my_m.particles_anim_loop = false | |
my_m.render_priority = 0 | |
my_m.next_pass = null | |
return my_m | |
# Called every frame. 'delta' is the elapsed time since the previous frame. | |
#func _process(delta): | |
# pass |
class_name MyParticles2D04 | |
extends Particles2D | |
# Declare member variables here. Examples: | |
# var a = 2 | |
# var b = "text" | |
# Called when the node enters the scene tree for the first time. | |
func _ready(): | |
self.emitting = true | |
self.amount = 4 | |
#Time: | |
self.lifetime = 1 | |
self.one_shot = false | |
self.preprocess = 0 | |
self.speed_scale = 1 | |
self.explosiveness = 0.0 | |
self.randomness = 0 | |
self.fixed_fps = 0 | |
self.fract_delta = true | |
#Drawing: | |
self.visibility_rect = Rect2(-100, -100, 200, 200) | |
self.local_coords = true | |
self.draw_order = Particles2D.DRAW_ORDER_INDEX | |
#Process Material: | |
self.process_material = self.get_process_material() | |
# Textures: | |
self.texture = load("res://resources/effect_4.png") | |
# Transform: | |
self.transform = Transform2D(0.0, Vector2(-7, -6)) | |
self.z_index = 0 | |
self.z_as_relative = true | |
# Material: | |
self.material = self.get_material() | |
func get_process_material() -> Material: | |
var my_m := ParticlesMaterial.new() | |
my_m.render_priority = 0 | |
my_m.next_pass = null | |
# Time: | |
my_m.lifetime_randomness = 0 | |
# Trail: | |
my_m.trail_divisor = 1 | |
my_m.trail_size_modifier = null | |
my_m.trail_color_modifier = null | |
# Emission Shape: | |
my_m.emission_shape = ParticlesMaterial.EMISSION_SHAPE_POINT | |
my_m.emission_ring_axis = Vector3(0, 0, 1) | |
# Flags: | |
my_m.flag_align_y = false | |
my_m.flag_rotate_y = false | |
my_m.flag_disable_z = true | |
# Direction: | |
my_m.direction = Vector3(1, 0, 0) | |
my_m.spread = 45 | |
my_m.flatness = 0 | |
# Gravity | |
my_m.gravity = Vector3(0, 0, 0) | |
# Initial Velocity: | |
my_m.initial_velocity = 0 | |
my_m.initial_velocity_random = 0 | |
# Angular Velocity: | |
my_m.angular_velocity = -100 | |
my_m.angular_velocity_random = 0 | |
my_m.angular_velocity_curve = null | |
# Orbit Velocity: | |
my_m.orbit_velocity = 0 | |
my_m.orbit_velocity_random = 0 | |
my_m.orbit_velocity_curve = null | |
# Linear Accel | |
my_m.linear_accel = 0 | |
my_m.linear_accel_random = 0 | |
my_m.linear_accel_curve = null | |
# Radial Accel: | |
my_m.radial_accel = 0 | |
my_m.radial_accel_random = 0 | |
my_m.radial_accel_curve = null | |
# Tangential Accel: | |
my_m.tangential_accel = 0 | |
my_m.tangential_accel_random = 0 | |
my_m.tangential_accel_curve = null | |
# Damping: | |
my_m.damping = 0 | |
my_m.damping_random = 0 | |
my_m.damping_curve = null | |
# Angle: | |
my_m.angle = 360 | |
my_m.angle_random = 1 | |
my_m.angle_curve = null | |
# Scale: | |
my_m.scale = 1 | |
my_m.scale_random = 0.0 | |
my_m.scale_curve = null | |
# Color: | |
my_m.color = Color.gray | |
# Hue Variation: | |
my_m.hue_variation = 0.0 | |
my_m.hue_variation_random = 0 | |
my_m.hue_variation_curve = null | |
# Animation: | |
my_m.anim_speed = 0 | |
my_m.anim_speed_random = 0 | |
my_m.anim_speed_curve = null | |
my_m.anim_offset = 0 | |
my_m.anim_offset_random = 0 | |
my_m.anim_offset_curve = null | |
return my_m | |
func get_material() -> Material: | |
var my_m := CanvasItemMaterial.new() | |
my_m.blend_mode = CanvasItemMaterial.BLEND_MODE_ADD | |
my_m.light_mode = CanvasItemMaterial.LIGHT_MODE_NORMAL | |
my_m.particles_animation = false | |
# my_m.particles_anim_h_frames = 3 | |
# my_m.particles_anim_v_frames = 1 | |
# my_m.particles_anim_loop = false | |
my_m.render_priority = 0 | |
my_m.next_pass = null | |
return my_m | |
# Called every frame. 'delta' is the elapsed time since the previous frame. | |
#func _process(delta): | |
# pass |
class_name MyParticles2D05 | |
extends Particles2D | |
# Declare member variables here. Examples: | |
# var a = 2 | |
# var b = "text" | |
# Called when the node enters the scene tree for the first time. | |
func _ready(): | |
self.emitting = true | |
self.amount = 5 | |
#Time: | |
self.lifetime = 1 | |
self.one_shot = false | |
self.preprocess = 0 | |
self.speed_scale = 1 | |
self.explosiveness = 0.08 | |
self.randomness = 0 | |
self.fixed_fps = 0 | |
self.fract_delta = true | |
#Drawing: | |
self.visibility_rect = Rect2(-100, -100, 200, 200) | |
self.local_coords = true | |
self.draw_order = Particles2D.DRAW_ORDER_INDEX | |
#Process Material: | |
self.process_material = self.get_process_material() | |
# Textures: | |
self.texture = load("res://resources/spotlight_8.png") | |
# Transform: | |
self.transform = Transform2D(0.0, Vector2(-11, -6)) | |
self.z_index = 0 | |
self.z_as_relative = true | |
# Material: | |
self.material = self.get_material() | |
func get_process_material() -> Material: | |
var my_m := ParticlesMaterial.new() | |
my_m.render_priority = 0 | |
my_m.next_pass = null | |
# Time: | |
my_m.lifetime_randomness = 0 | |
# Trail: | |
my_m.trail_divisor = 1 | |
my_m.trail_size_modifier = null | |
my_m.trail_color_modifier = null | |
# Emission Shape: | |
my_m.emission_shape = ParticlesMaterial.EMISSION_SHAPE_BOX | |
my_m.emission_box_extents = Vector3(20, 30, 1) | |
my_m.emission_ring_axis = Vector3(0, 0, 1) | |
# Flags: | |
my_m.flag_align_y = false | |
my_m.flag_rotate_y = false | |
my_m.flag_disable_z = true | |
# Direction: | |
my_m.direction = Vector3(1, 0, 0) | |
my_m.spread = 45 | |
my_m.flatness = 0 | |
# Gravity | |
my_m.gravity = Vector3(0, 0, 0) | |
# Initial Velocity: | |
my_m.initial_velocity = 0 | |
my_m.initial_velocity_random = 0 | |
# Angular Velocity: | |
my_m.angular_velocity = 0 | |
my_m.angular_velocity_random = 0 | |
my_m.angular_velocity_curve = null | |
# Orbit Velocity: | |
my_m.orbit_velocity = 0 | |
my_m.orbit_velocity_random = 0 | |
my_m.orbit_velocity_curve = null | |
# Linear Accel | |
my_m.linear_accel = 0 | |
my_m.linear_accel_random = 0 | |
my_m.linear_accel_curve = null | |
# Radial Accel: | |
my_m.radial_accel = 0 | |
my_m.radial_accel_random = 0 | |
my_m.radial_accel_curve = null | |
# Tangential Accel: | |
my_m.tangential_accel = 0 | |
my_m.tangential_accel_random = 0 | |
my_m.tangential_accel_curve = null | |
# Damping: | |
my_m.damping = 0 | |
my_m.damping_random = 0 | |
my_m.damping_curve = null | |
# Angle: | |
my_m.angle = 0 | |
my_m.angle_random = 0 | |
my_m.angle_curve = null | |
# Scale: | |
my_m.scale = 0.3 | |
my_m.scale_random = 0.3 | |
my_m.scale_curve = load("res://new_curvetexture.curvetex") | |
# Color: | |
my_m.color = Color.gray | |
# Hue Variation: | |
my_m.hue_variation = 0.0 | |
my_m.hue_variation_random = 0 | |
my_m.hue_variation_curve = null | |
# Animation: | |
my_m.anim_speed = 0 | |
my_m.anim_speed_random = 0 | |
my_m.anim_speed_curve = null | |
my_m.anim_offset = 0 | |
my_m.anim_offset_random = 0 | |
my_m.anim_offset_curve = null | |
return my_m | |
func get_material() -> Material: | |
var my_m := CanvasItemMaterial.new() | |
my_m.blend_mode = CanvasItemMaterial.BLEND_MODE_ADD | |
my_m.light_mode = CanvasItemMaterial.LIGHT_MODE_NORMAL | |
my_m.particles_animation = false | |
# my_m.particles_anim_h_frames = 3 | |
# my_m.particles_anim_v_frames = 1 | |
# my_m.particles_anim_loop = false | |
my_m.render_priority = 0 | |
my_m.next_pass = null | |
return my_m | |
# Called every frame. 'delta' is the elapsed time since the previous frame. | |
#func _process(delta): | |
# pass |
● float orbit_velocity
set_param(value) setter
get_param() getter
Orbital velocity applied to each particle. Makes the particles circle around origin. Specified in number of full rotations around origin per second.
Only available when flag_disable_z is true.
the more you set this to its max value (1000), particles will circle around to the right side (with higher speed), and the more you set this to its min value (-1000), the particles will circle around to the left side (left side as compared to the center of the region), (with higher speed).
if we want a normal speed, we better just set it to 0.
● float angular_velocity [default: 0.0]
set_param(value) setter
get_param() getter
Initial angular velocity applied to each particle. Sets the speed of rotation of the particle.
Only applied when flag_disable_z or flag_rotate_y are true or the SpatialMaterial being used to draw the particle is using SpatialMaterial.BILLBOARD_PARTICLES.
Min: -720
Max: +720
● float angular_velocity_random [default: 0.0]
set_param_randomness(value) setter
get_param_randomness() getter
Angular velocity randomness ratio.
Min: 0.00
Max: 1.00
● float tangential_accel [default: 0.0]
set_param(value) setter
get_param() getter
Tangential acceleration applied to each particle. Tangential acceleration is perpendicular to the particle's velocity giving the particles a swirling motion.
Min: -100
Max: 100
● float tangential_accel_random [default: 0.0]
set_param_randomness(value) setter
get_param_randomness() getter
Tangential acceleration randomness ratio.
Min: 0.00
Max: 1.00
● float damping [default: 0.0]
set_param(value) setter
get_param() getter
The rate at which particles lose velocity.
Min: 0
Max: 100
● float damping_random [default: 0.0]set_param_randomness(value) setterget_param_randomness() getter
Damping randomness ratio.
Min: 0.00
Max: 1.00
● float angle [default: 0.0]
set_param(value) setter
get_param() getter
Initial rotation applied to each particle, in degrees.
Only applied when flag_disable_z or flag_rotate_y are true or the SpatialMaterial being used to draw the particle is using SpatialMaterial.BILLBOARD_PARTICLES.
Min: -720
Max: 720
● float angle_random [default: 0.0]
set_param_randomness(value) setter
get_param_randomness() getter
Rotation randomness ratio.
Min: 0.00
Max: 1.00
● float scale [default: 1.0]
set_param(value) setter
get_param() getter
Initial scale applied to each particle.
warning: don't increase this property too much, you might not even be able to see any particle in the screen if you increase it too much.
Min: 0.00
Max: 1000.00
● float scale_random [default: 0.0]set_param_randomness(value) setterget_param_randomness() getter
Scale randomness ratio.
Min: 0.00
Max: 1.00
● float hue_variation [default: 0.0]
set_param(value) setter
get_param() getter
Initial hue variation applied to each particle.
Min: -1.00
Max: 1.00
Important if you have set hue_variation
property to a non-zero value
● float hue_variation_random [default: 0.0]
set_param_randomness(value) setter
get_param_randomness() getter
Hue variation randomness ratio.
Min: 0.00
Max: 1.00
● float anim_speed [default: 0.0]
set_param(value) setter
get_param() getter
Particle animation speed.
Min: 0.00
Max: 128.00
● float anim_speed_random [default: 0.0]
set_param_randomness(value) setter
get_param_randomness() getter
Animation speed randomness ratio.
Min: 0.00
Max: 1.00
● float anim_offset [default: 0.0]
set_param(value) setter
get_param() getter
Particle animation offset.
Min: 0.00
Max: 1.00
● float anim_offset_random [default: 0.0]
set_param_randomness(value) setter
get_param_randomness() getter
Animation offset randomness ratio.
Min: 0.00
Max: 1.00
linear_accel
property ofprocess_material
acts like the height. the more you increase it, the higher the particle effects will go.This pic is the highest value (100):
and this pic is for lowest value (-100):
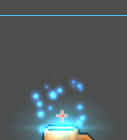
the lower the value, the more they will stay close to the center of their region.
from official docs:
Setting this property to 0 will result in having a normal linear acceleration. (the default situation)