Last active
August 23, 2020 02:37
-
-
Save AllieUbisse/01f1f137a838b3898ddedc000cee1942 to your computer and use it in GitHub Desktop.
Mini script to help start, stop and check the status/public ip address for your aws ec2 via aws-cli
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/bin/bash | |
######################################################################################## | |
# START, STOP or STATUS # | |
# ---------------------------- # | |
# This scrip is intended to help you start, stop or get the IP address of # | |
# Current running EC2. # | |
# This will require you to 1st configure your AWC-CLI, namualy to ensure safety # | |
# # | |
# Please read the code to ensure that It does not cause any security issues # | |
# # | |
# AUTHOR: ALLIE .S UBISSE # | |
# # | |
######################################################################################## | |
# | |
# | |
ACTION=$1 # argument to pass: start, stop, status | |
# Set your EC2 Instance ID here | |
INSTANCE_ID=i-XXXXXXXXXXXXXXXXX | |
if [[ "$ACTION" == "start" ]] | |
then | |
# start instance with matching ID, wait until it response as running then get Puplic IP Address | |
aws ec2 start-instances --instance-ids $INSTANCE_ID | grep -e "PreviousState" -e "CurrentState" -e "Name" | |
aws ec2 wait instance-running --instance-ids $INSTANCE_ID | |
HOSTNAME=$(aws ec2 describe-instances --instance-ids $INSTANCE_ID --query 'Reservations[*].Instances[*].PublicIpAddress' --output text) | |
echo "=======================MINI INFO==========================" | |
echo "EC2 instance running: ID=$INSTANCE_ID Public-IP=$HOSTNAME" | |
elif [[ "$ACTION" == "stop" ]] | |
then | |
aws ec2 stop-instances --instance-ids $INSTANCE_ID | grep -e "PreviousState" -e "CurrentState" -e "Name" | |
aws ec2 wait instance-stopped --instance-ids $INSTANCE_ID | |
echo " EC2 instance with ID $INSTANCE_ID has stopped..." | |
elif [[ "$ACTION" == "status" ]] | |
then | |
IP_ADDRESS=$(aws ec2 describe-instances --instance-ids $INSTANCE_ID --query 'Reservations[*].Instances[*].PublicIpAddress' --output text) | |
STATE=$(aws ec2 describe-instances --instance-ids $INSTANCE_ID --query 'Reservations[*].Instances[*].State[].Name' --output text) | |
echo "=======================Current Taged EC2 Instance==========================" | |
echo "EC2 instance ID: $INSTANCE_ID" | |
echo "State: $STATE" | |
echo "Public-IP: $IP_ADDRESS" | |
else | |
echo "Try to pass start, stop or status as the argument" | |
fi |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
HOW TO:
Recommended To 1st Install the AWS CLI version 2 on Linux
configure aws-cl
Mini script to help start, stop and check the status/public ip address for your aws ec2 via aws-cli
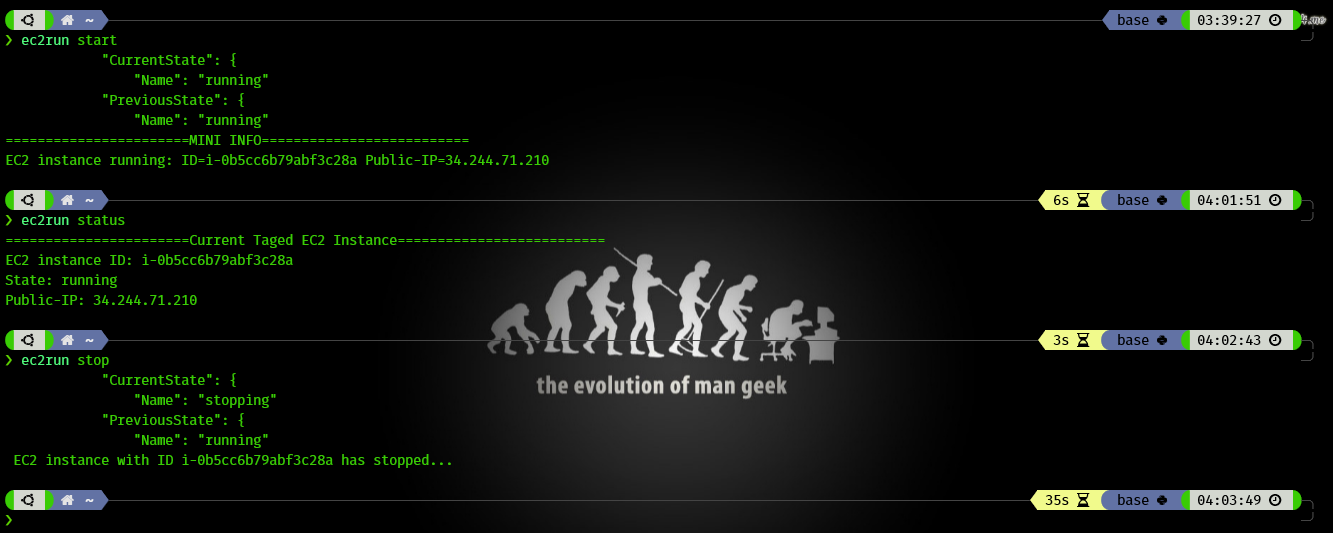
Add the ec2run.sh file to Bash terminal or zsh:
download script file into
~/home/$USER/.scripts
and Edit2.1 download
wget https://gist.githubusercontent.com/AllieUbisse/01f1f137a838b3898ddedc000cee1942\ /raw/f22f9b40ec0ad46be93bfc871b1427f7f3ebbd3c/ec2run.sh # make script executable chmod +x ec2run.sh
2.2 # Add the Instance ID to script, replacing

INSTANCE_ID=
i-XXXXXXXXXXXXXXXXX.Vim hotkeys: press I to insert/edit then when done hit Esc to exit editing mode --> now hit Shift + : then wq + ENTER
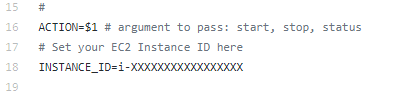
Append alias to
~/.bashrc or/& ~/.zshrc
Lets use the script
4.1 start EC2 instance
4.2 check status / get public IP for EC2 instance
4.3 Stop the EC2 instance