Last active
January 14, 2020 12:49
-
-
Save CYHSM/f98b49fc244e786fb39dd843e400c0cb to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import numpy as np | |
import matplotlib.pyplot as plt | |
import tensorflow as tf | |
from tensorflow.keras.layers import Dense, Activation, Input | |
from tensorflow.keras import Model | |
from tensorflow.keras.optimizers import SGD | |
# Implement activation function | |
def dCaAP_activation(x): | |
return 4*tf.maximum(0.0, tf.cast(sigmoid_derivative(x), 'float32')) * tf.cast((x > 0), 'float32') | |
def sigmoid_derivative(x): | |
return tf.sigmoid(x) * (1 - tf.sigmoid(x)) | |
# Create model | |
def create_model(random_init): | |
input_layer = Input(shape=(2,)) | |
if random_init: | |
out = Dense(units=1)(input_layer) | |
else: | |
out = Dense(units=1, kernel_initializer=tf.keras.initializers.Constant(1))(input_layer) | |
out = Activation(dCaAP_activation)(out) | |
model = Model(inputs=input_layer, outputs=out) | |
model.compile(SGD(lr=0.1), loss='binary_crossentropy', metrics=['accuracy']) | |
return model | |
# Plot dCaAP activation function | |
xplot = np.linspace(-10, 10, 100) | |
yplot = dCaAP_activation(xplot).numpy() | |
plt.plot(xplot, yplot) | |
plt.show() | |
# Create input output for XOR problem | |
X = np.array([[0,0],[0,1],[1,0],[1,1]]) | |
y = np.array([[0],[1],[1],[0]]) | |
# Run model for weights initialized to 1, solves XOR roughly 5/10 times | |
for ii in range(0, 10): | |
model = create_model(random_init=False) | |
model_weights = model.get_weights() | |
print('------------------------------------------------------------------') | |
print('Running init {}, w_1 = {:.3f}, w_2 = {:.3f}, b = {:.3f}'.format(ii, model_weights[0][0,0], model_weights[0][1,0], model_weights[1][0])) | |
model.fit(X, y, epochs=1000, batch_size=1, verbose=0) | |
new_model_weights = model.get_weights() | |
model_prediction = model.predict(X) | |
print('Trained weights, w_1 = {:.3f}, w_2 = {:.3f}, b = {:.3f}'.format(new_model_weights[0][0,0], new_model_weights[0][1,0], new_model_weights[1][0])) | |
print('Model Predictions: [{:.3f},{:.3f},{:.3f},{:.3f}] ---> XOR Problem Solved: {}'.format(model_prediction[0,0], model_prediction[1,0], | |
model_prediction[2,0], model_prediction[3,0], all(y==(model_prediction > 0.5)))) # np.set_printoptions(precision=3) | |
# Run model for random inits, solves XOR roughly 1/10 times | |
for ii in range(0, 10): | |
model = create_model(random_init=True) | |
model_weights = model.get_weights() | |
print('------------------------------------------------------------------') | |
print('Running init {}, w_1 = {:.3f}, w_2 = {:.3f}, b = {:.3f}'.format(ii, model_weights[0][0,0], model_weights[0][1,0], model_weights[1][0])) | |
model.fit(X, y, epochs=1000, batch_size=1, verbose=0) | |
new_model_weights = model.get_weights() | |
model_prediction = model.predict(X) | |
print('Trained weights, w_1 = {:.3f}, w_2 = {:.3f}, b = {:.3f}'.format(new_model_weights[0][0,0], new_model_weights[0][1,0], new_model_weights[1][0])) | |
print('Model Predictions: [{:.3f},{:.3f},{:.3f},{:.3f}] ---> XOR Problem Solved: {}'.format(model_prediction[0,0], model_prediction[1,0], | |
model_prediction[2,0], model_prediction[3,0], all(y==(model_prediction > 0.5)))) # np.set_printoptions(precision=3) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Colab:

Activation Function:
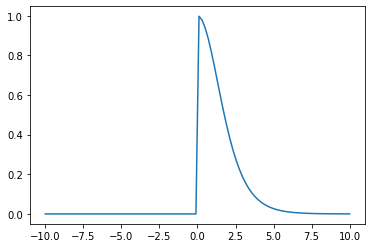
Example Output: