Created
April 12, 2021 17:53
-
-
Save Da9el00/0eb373e7173018dc5903ce6f3c8888fc to your computer and use it in GitHub Desktop.
Play Sound with JavaFX
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package sample; | |
import javafx.fxml.FXML; | |
import javafx.scene.input.MouseEvent; | |
import javafx.scene.media.Media; | |
import javafx.scene.media.MediaPlayer; | |
import java.io.File; | |
public class Controller{ | |
MediaPlayer mediaPlayer; | |
@FXML | |
void play(MouseEvent event) { | |
String fileName = "SurvivetheMontageRKVC.mp3"; | |
playHitSound(fileName); | |
} | |
private void playHitSound(String fileName){ | |
String path = getClass().getResource(fileName).getPath(); | |
Media media = new Media(new File(path).toURI().toString()); | |
mediaPlayer = new MediaPlayer(media); | |
mediaPlayer.setCycleCount(MediaPlayer.INDEFINITE); | |
mediaPlayer.play(); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package sample; | |
import javafx.application.Application; | |
import javafx.fxml.FXMLLoader; | |
import javafx.scene.Parent; | |
import javafx.scene.Scene; | |
import javafx.stage.Stage; | |
public class Main extends Application { | |
@Override | |
public void start(Stage primaryStage) throws Exception{ | |
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml")); | |
primaryStage.setTitle("Hello World"); | |
primaryStage.setScene(new Scene(root)); | |
primaryStage.show(); | |
} | |
public static void main(String[] args) { | |
launch(args); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="UTF-8"?> | |
<?import javafx.scene.control.Button?> | |
<?import javafx.scene.layout.AnchorPane?> | |
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/15.0.1" xmlns:fx="http://javafx.com/fxml/1" fx:controller="sample.Controller"> | |
<children> | |
<Button layoutX="274.0" layoutY="182.0" mnemonicParsing="false" onMouseClicked="#play" text="Button" /> | |
</children> | |
</AnchorPane> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
File structure:
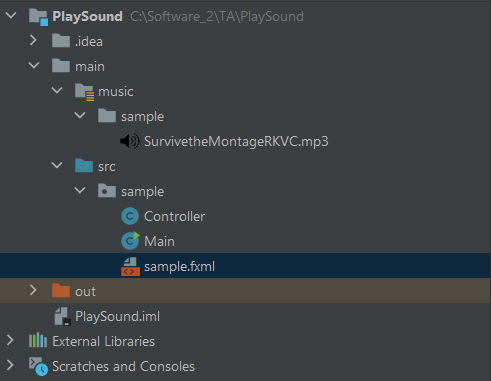