Created
February 15, 2021 22:09
-
-
Save Da9el00/a050bb270f23a9f8cb2eaa8ef360da95 to your computer and use it in GitHub Desktop.
JavaFX with Scene Builder: creating a datePicker where you can pick exactly 2 dates.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package sample; | |
import javafx.collections.FXCollections; | |
import javafx.collections.ObservableList; | |
import javafx.fxml.FXML; | |
import javafx.fxml.Initializable; | |
import javafx.scene.control.DateCell; | |
import javafx.scene.control.DatePicker; | |
import javafx.scene.input.MouseEvent; | |
import javafx.util.Callback; | |
import java.net.URL; | |
import java.time.LocalDate; | |
import java.util.ResourceBundle; | |
public class Controller implements Initializable { | |
ObservableList<LocalDate> selectedDates = FXCollections.observableArrayList(); | |
@FXML | |
private DatePicker datePicker; | |
@FXML | |
void getDate(MouseEvent event) { | |
for (LocalDate date : selectedDates) { | |
System.out.println(date); | |
} | |
} | |
@Override | |
public void initialize(URL url, ResourceBundle resourceBundle) { | |
datePicker.setOnAction(event -> { | |
if (selectedDates.size() > 1){ | |
selectedDates.clear(); | |
} | |
selectedDates.add(datePicker.getValue());} | |
); | |
datePicker.setDayCellFactory(new Callback<DatePicker, DateCell>() { | |
@Override | |
public DateCell call(DatePicker param) { | |
return new DateCell() { | |
@Override | |
public void updateItem(LocalDate item, boolean empty) { | |
super.updateItem(item, empty); | |
boolean alreadySelected = selectedDates.contains(item); | |
setDisable(alreadySelected); | |
setStyle(alreadySelected ? "-fx-background-color: #C06C84;" : ""); | |
} | |
}; | |
} | |
}); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package sample; | |
import javafx.application.Application; | |
import javafx.fxml.FXMLLoader; | |
import javafx.scene.Parent; | |
import javafx.scene.Scene; | |
import javafx.stage.Stage; | |
public class Main extends Application { | |
@Override | |
public void start(Stage primaryStage) throws Exception{ | |
Parent root = FXMLLoader.load(getClass().getResource("sample.fxml")); | |
primaryStage.setTitle("Hello World"); | |
primaryStage.setScene(new Scene(root)); | |
primaryStage.show(); | |
} | |
public static void main(String[] args) { | |
launch(args); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?xml version="1.0" encoding="UTF-8"?> | |
<?import javafx.scene.control.Button?> | |
<?import javafx.scene.control.DatePicker?> | |
<?import javafx.scene.layout.AnchorPane?> | |
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/15.0.1" xmlns:fx="http://javafx.com/fxml/1" fx:controller="sample.Controller"> | |
<children> | |
<Button layoutX="88.0" layoutY="168.0" mnemonicParsing="false" onMouseClicked="#getDate" prefHeight="65.0" prefWidth="122.0" text="getDates" /> | |
<DatePicker fx:id="datePicker" layoutX="306.0" layoutY="188.0" /> | |
</children> | |
</AnchorPane> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
You can need to reopen the datePicker to pick a second date and then when you pick the 3rd date it reset to just having 1 day picked.
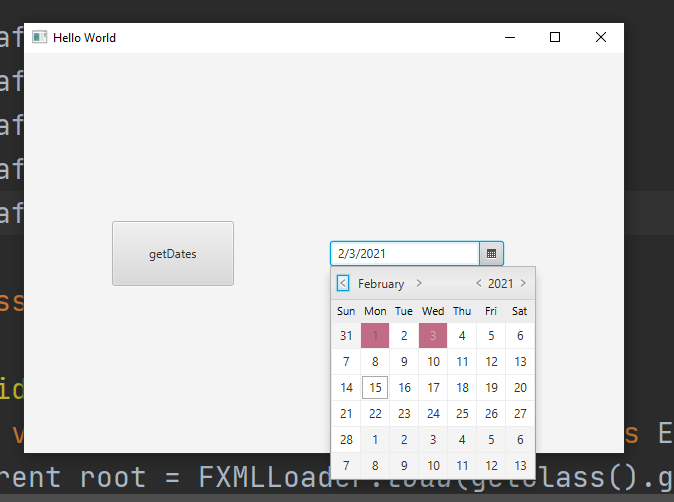