Created
December 10, 2022 05:36
-
-
Save Equlnox/1265416a46db671454644f9ae0cd0bc4 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# inspired from https://www.instagram.com/reel/Cl4WkH9OPXc/?igshid=YmMyMTA2M2Y%3D | |
from matplotlib import pyplot as plt | |
import numpy as np | |
import random | |
from math import sqrt | |
def plot_points(points): | |
x = [p[0] for p in points] | |
y = [p[1] for p in points] | |
margin = 0.1 | |
maxx = max(x) + margin | |
maxy = max(y) + margin | |
minx = min(x) - margin | |
miny = min(y) - margin | |
# plt.rcParams['lines.markersize'] = 0.1 | |
plt.rcParams["figure.figsize"] = [18, 16] | |
plt.rcParams["figure.autolayout"] = True | |
plt.xlim(minx, maxx) | |
plt.ylim(miny, maxy) | |
plt.grid() | |
s = [0.005] * len(x) | |
plt.scatter(x, y, s=s, marker="o") | |
plt.show() | |
def generate_points(triangle_points, first_point, num_to_generate): | |
generated_points = [first_point] | |
for idx in range(num_to_generate): | |
selected_triangle_point = random.choice(triangle_points) | |
last_generated_point = generated_points[-1] | |
new_point = ((selected_triangle_point[0] + last_generated_point[0]) / 2, (selected_triangle_point[1] + last_generated_point[1]) / 2) | |
generated_points.append(new_point) | |
return generated_points | |
t1 = (0, 0) | |
t2 = (1, 0) | |
t3 = (1/2, sqrt(3) / 2) | |
first_point = (0.5 , 0.5) | |
generated_points = generate_points([t1, t2, t3], first_point, 1000000) | |
plot_points([t1, t2, t3] + generated_points) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
This is the image generated from the code above
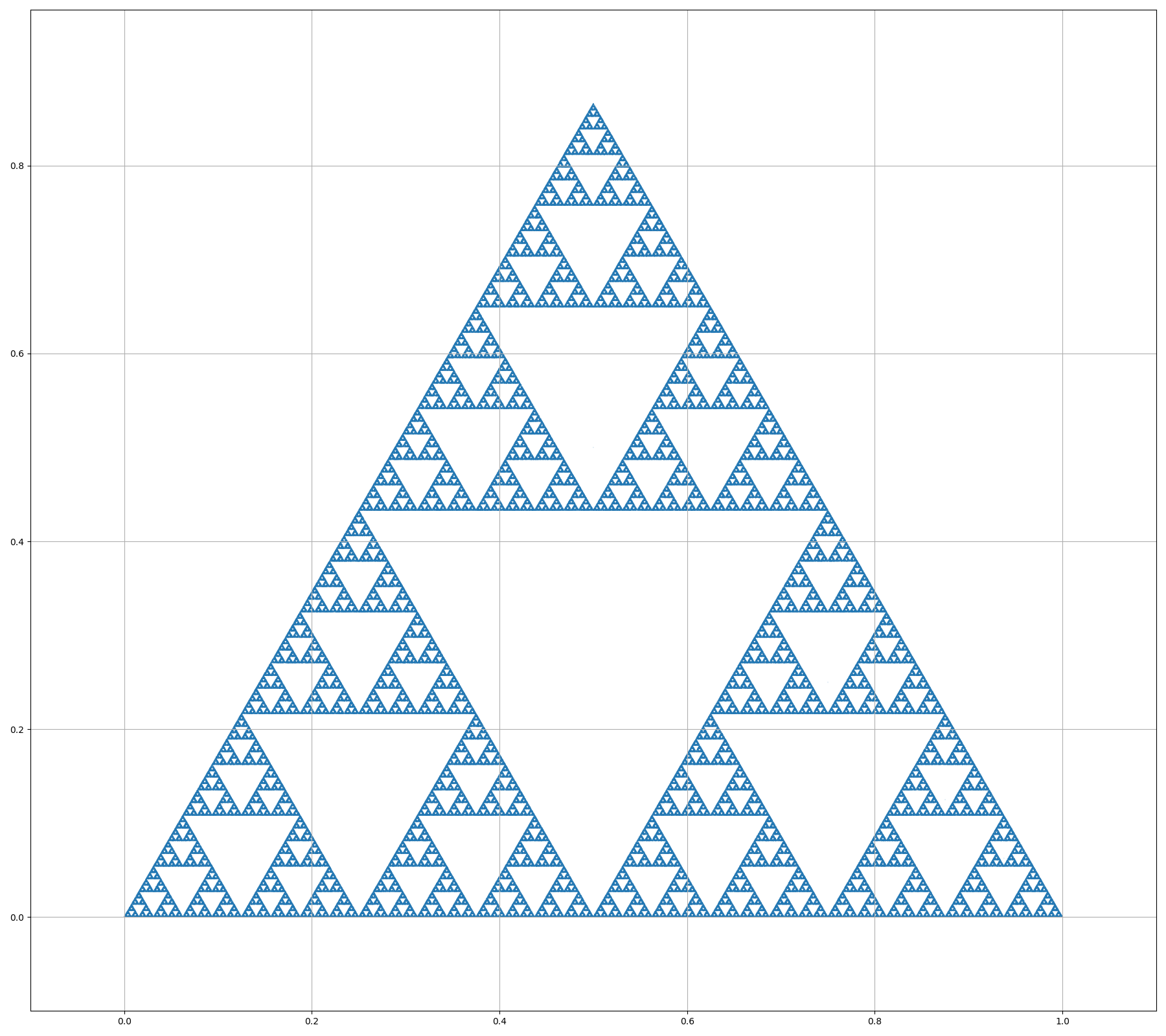