Created
December 28, 2022 20:53
-
-
Save Fevol/54349fa207e49f92c712bf577013720f to your computer and use it in GitHub Desktop.
Obsidian - Foldable settings section
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import {Plugin} from 'obsidian'; | |
import {SampleSettingTab} from "./settings"; | |
export default class MyPlugin extends Plugin { | |
settings: { [key: string]: any } = {}; | |
// `data` variable contains data that will *not* be saved on app unload, this means that the sections that were closed/opened | |
// will be forgotten once the app restarts. | |
// If you want these folds to be saved across app restarts too, then store the open: {} in settings instead. | |
data: { open: { [key: string]: boolean } ; } | |
= { open: {} }; | |
async onload() { | |
await this.loadSettings(); | |
this.addSettingTab(new SampleSettingTab(this.app, this)); | |
} | |
async loadSettings() { | |
this.settings = Object.assign({}, DEFAULT_SETTINGS, await this.loadData()); | |
} | |
async saveSettings() { | |
await this.saveData(this.settings); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/** Notes: | |
* - Implementation is partially based on the Admonition plugin, accessible here: https://github.com/valentine195/obsidian-admonition/blob/master/src/settings.ts | |
* - Above plugin also includes animations of the handler icons, which look much better than what is offered by default | |
*/ | |
import {App, PluginSettingTab, Setting} from "obsidian"; | |
import MyPlugin from "./main"; | |
export class SampleSettingTab extends PluginSettingTab { | |
plugin: MyPlugin; | |
constructor(app: App, plugin: MyPlugin) { | |
super(app, plugin); | |
this.plugin = plugin; | |
} | |
display(): void { | |
const {containerEl} = this; | |
containerEl.empty(); | |
// Used for demonstrative purposes, you might not want to do it this way | |
const settingsExample = [ | |
{ id: "general", displayName: "General Settings", func: this.generateGeneralSettings }, | |
{ id: "function", displayName: "Functional Settings", func: this.generateFunctionSettings }, | |
] | |
settingsExample.forEach((setting) => { | |
const section = this.createSection(containerEl, setting.id, setting.displayName); | |
setting.func(section); | |
}); | |
} | |
createSection(container: HTMLElement, sectionId: string, displayName: string) { | |
const sectionEl = container.createEl('details', { | |
cls: 'YOURPLUGIN-settings-section', | |
attr: { | |
...(this.plugin.data.open[sectionId] ? {open: true} : {}) | |
} | |
}); | |
// On toggle, hide or show the section | |
sectionEl.ontoggle = () => { this.plugin.data.open[sectionId] = sectionEl.open; }; | |
const summaryEl = sectionEl.createEl('summary', { cls: 'YOURPLUGIN-settings-section-header', text: displayName }); | |
return sectionEl; | |
} | |
generateGeneralSettings(section: HTMLElement) { | |
new Setting(section) | |
.setName('Setting 1') | |
.setDesc('Description 1') | |
.addDropdown((dropdown) => { | |
dropdown | |
.addOption('option 1', 'value 1') | |
.addOption('option 2', 'value 2') | |
.setValue('option 1') | |
}); | |
} | |
generateFunctionSettings(section: HTMLElement) { | |
new Setting(section) | |
.setName('Setting 2') | |
.setDesc('Description 2') | |
.addText((text) => { | |
text | |
.setPlaceholder('placeholder') | |
.setValue('value') | |
}); | |
} | |
} | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
How it looks:
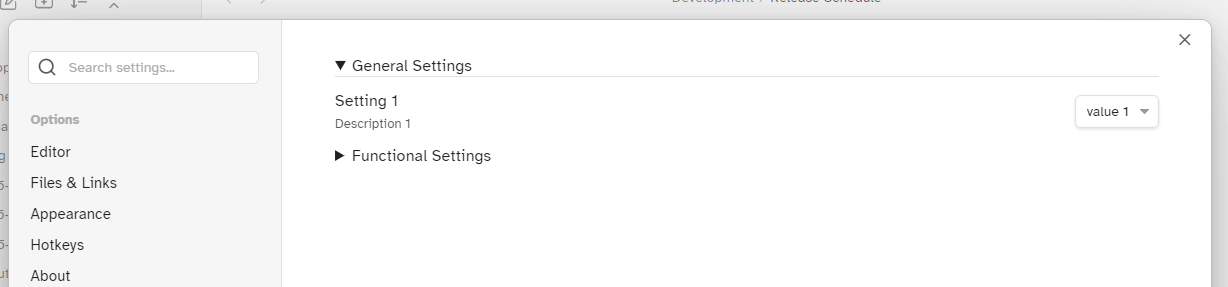