Last active
January 21, 2024 17:50
-
-
Save Fizzyhex/1c818a3a669d428934bdb8cd8d9ca70c to your computer and use it in GitHub Desktop.
⭐ Star Rating UI (Fusion v0.3 pre-release)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
-- Note that the components in this script should ideally be split up into different scripts; which I didn't do here. | |
local ReplicatedStorage = game:GetService("ReplicatedStorage") | |
local Fusion = require(ReplicatedStorage.Packages.Fusion) | |
local Computed = Fusion.Computed | |
local New = Fusion.New | |
local Children = Fusion.Children | |
local ForValues = Fusion.ForValues | |
local function Star(props) | |
return New "ImageLabel" { | |
Name = "Star", | |
Image = "rbxassetid://14717297422", | |
Parent = props.Parent, | |
ImageColor3 = Color3.new(0, 0, 0), | |
BackgroundTransparency = 1, | |
Size = UDim2.fromOffset(50, 50), | |
[Children] = { | |
New "Frame" { | |
Size = Computed(function(use) | |
local x = use(props.Fill) or 1 | |
return UDim2.fromScale(x, 1) | |
end), | |
ClipsDescendants = true, | |
BackgroundTransparency = 1, | |
[Children] = { | |
New "ImageLabel" { | |
Image = "rbxassetid://14717297422", | |
Size = UDim2.fromOffset(50, 50), | |
ImageColor3 = Color3.new(1, 1, 0), | |
BackgroundTransparency = 1, | |
} | |
} | |
} | |
} | |
} | |
end | |
-- This decides how many stars to create and how filled in they should be. | |
local function ComputeStarFills(rating) | |
return Computed(function(use) | |
local remaining = use(rating) | |
local fills = {} | |
while remaining > 0 do | |
local starFill = math.min(1, remaining) | |
remaining -= starFill | |
table.insert(fills, starFill) | |
end | |
return fills | |
end) | |
end | |
local function Rating(props) | |
return New "Frame" { | |
Parent = props.Parent, | |
BackgroundTransparency = 1, | |
Name = "Rating", | |
AutomaticSize = Enum.AutomaticSize.XY, | |
[Children] = { | |
New "UIListLayout" { | |
FillDirection = Enum.FillDirection.Horizontal, | |
Padding = UDim.new(0, 8) | |
}, | |
ForValues(ComputeStarFills(props.Rating), function(_, fill) | |
return Star { Fill = fill } | |
end, Fusion.cleanup) | |
} | |
} | |
end | |
return Rating |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
local ReplicatedStorage = game:GetService("ReplicatedStorage") | |
local Rating = require(script.Parent.Rating) | |
local Fusion = require(ReplicatedStorage.Packages.Fusion) | |
local Value = Fusion.Value | |
return function(target) | |
local ui = Rating { | |
Parent = target, | |
Rating = Value(5) | |
} | |
return function() | |
ui:Destroy() | |
end | |
end |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Demo:
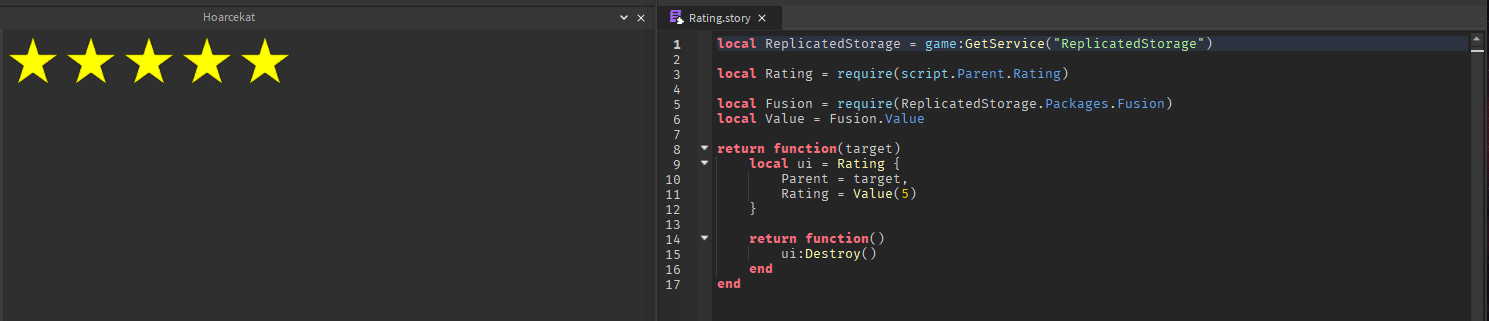