Last active
November 28, 2021 07:07
-
-
Save FlandreDaisuki/77bc5d65fe8d6bf5a92de6544b085c03 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/bin/bash | |
set -e | |
if [ -z "$1" ]; then | |
echo "Usage: $0 <path to video which play on chrome>" | |
exit 1 | |
fi | |
FILEPATH="$1" | |
BASENAME=$(basename "$FILEPATH") | |
NO_EXT_BASENAME="${BASENAME%.*}" | |
# take a screenshot | |
# ref: https://trac.ffmpeg.org/wiki/Seeking | |
SCREENSHOT_PATH=$(mktemp -u -t XXXXXX --suffix .jpg) | |
ffmpeg -ss 00:00:05 -i "$FILEPATH" -frames:v 1 -q:v 5 "${SCREENSHOT_PATH}" | |
# get gameplay area | |
# ref: https://imagemagick.org/script/connected-components.php | |
# the output will be like: | |
# 20342: 960x640+470+165 963.3,484.1 589712 gray(0) | |
# which means: | |
# id: bounding-box centroid area mean-color | |
TMP_STDERR=$(mktemp -u -t XXXXXX --suffix .stderr) | |
THRESHOLD=600000 | |
THRESHOLD_STEP=10000 | |
function get-segmentation-objects() { | |
convert "${SCREENSHOT_PATH}" \ | |
-type bilevel \ | |
-define connected-components:area-threshold="$1" \ | |
-define connected-components:verbose=true \ | |
-connected-components 8 \ | |
/dev/null 2>"${TMP_STDERR}" | |
} | |
function get-gameplay-area() { | |
get-segmentation-objects "$1" | jq --raw-input 'match("960x640\\S+")' | |
} | |
echo "threshold: $THRESHOLD" | |
CONNECTED_COMPONENT_RESULT=$(get-gameplay-area "${THRESHOLD}") | |
while [ -z "$CONNECTED_COMPONENT_RESULT" ] && [ -z "$(cat "$TMP_STDERR")" ] && [ "${THRESHOLD}" -gt 0 ]; do | |
THRESHOLD=$((THRESHOLD - THRESHOLD_STEP)) | |
echo "threshold: $THRESHOLD" | |
CONNECTED_COMPONENT_RESULT=$(get-gameplay-area "${THRESHOLD}") | |
done | |
if [ -z "$CONNECTED_COMPONENT_RESULT" ]; then | |
echo "Failed to get gameplay area" | |
exit 1 | |
fi | |
# the CROP_POSITION will thansform above result to be: "470:165" | |
CROP_POSITION=$( | |
echo "${CONNECTED_COMPONENT_RESULT}" | \ | |
jq --raw-input --raw-output --slurp \ | |
'[match("960x640[+](\\d+)[+](\\d+)").captures[].string] | join(":")' | |
) | |
# do crop video | |
ffmpeg -i "$BASENAME" -vf crop="960:640:${CROP_POSITION}" -c:a copy "$NO_EXT_BASENAME"-crop.mp4 | |
# clean up | |
rm "${SCREENSHOT_PATH}" "${TMP_STDERR}" |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Screenshot example:
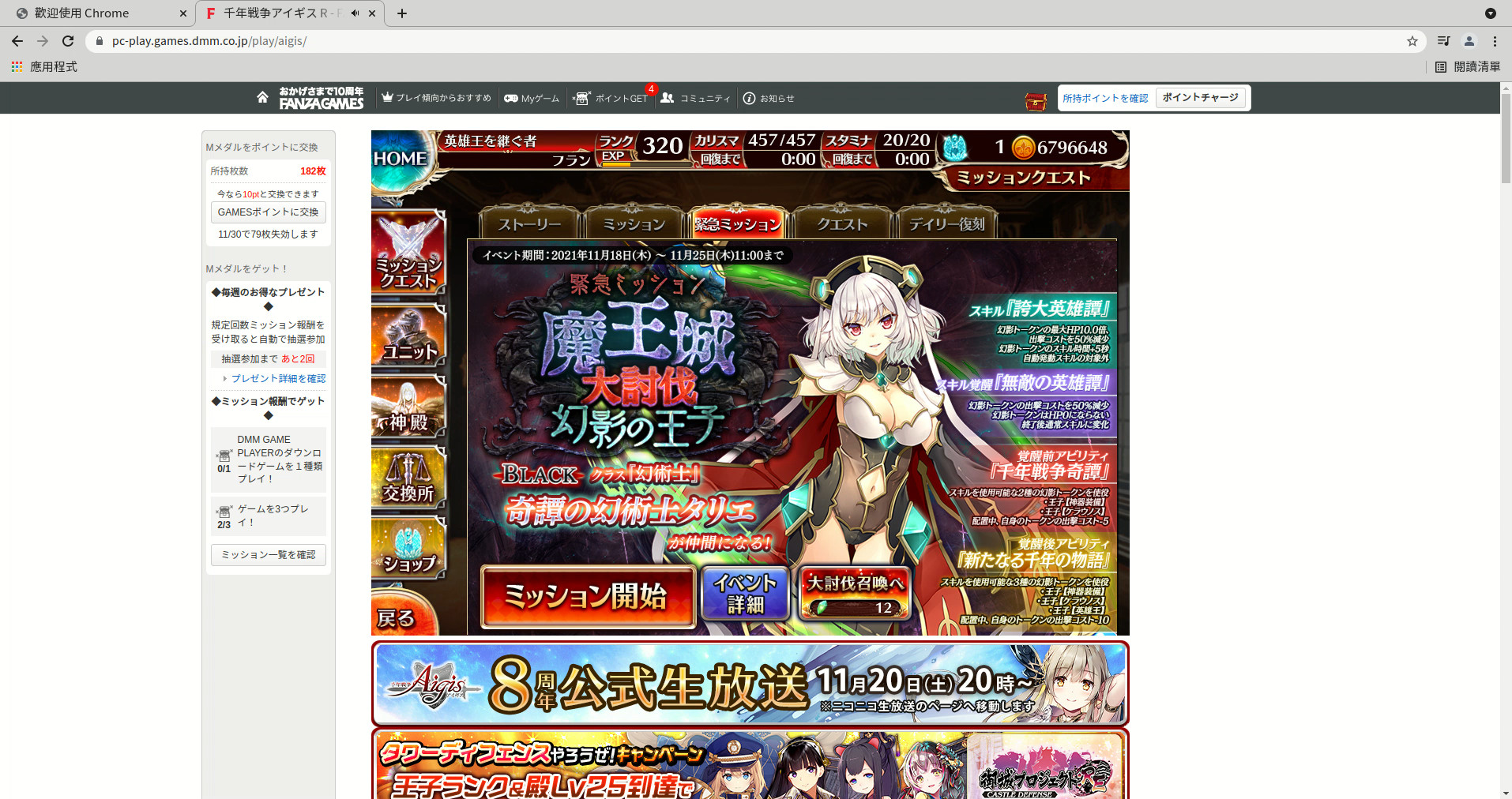