The latest beta (3.5) includes separate color settings for light & dark mode. Toggling dark mode automatically switches colors.
Vist iTerm2 homepage or use brew install iterm2-beta
to download the beta. Thanks @stefanwascoding.
- Add
switch_automatic.py
to~/Library/ApplicationSupport/iTerm2/Scripts/AutoLaunch
with:
#!/usr/bin/env python3
import asyncio
import iterm2
async def main(connection):
async with iterm2.VariableMonitor(connection, iterm2.VariableScopes.APP, "effectiveTheme", None) as mon:
while True:
# Block until theme changes
theme = await mon.async_get()
# Themes have space-delimited attributes, one of which will be light or dark.
parts = theme.split(" ")
if "dark" in parts:
preset = await iterm2.ColorPreset.async_get(connection, "Dark Background")
else:
preset = await iterm2.ColorPreset.async_get(connection, "Light Background")
# Update the list of all profiles and iterate over them.
profiles=await iterm2.PartialProfile.async_query(connection)
for partial in profiles:
# Fetch the full profile and then set the color preset in it.
profile = await partial.async_get_full_profile()
await profile.async_set_color_preset(preset)
iterm2.run_forever(main)
Change Dark Background
and Light Background
to color presets you like.
- Enabled
switch_automatic.py
from Scripts menu.
i got this error... why?
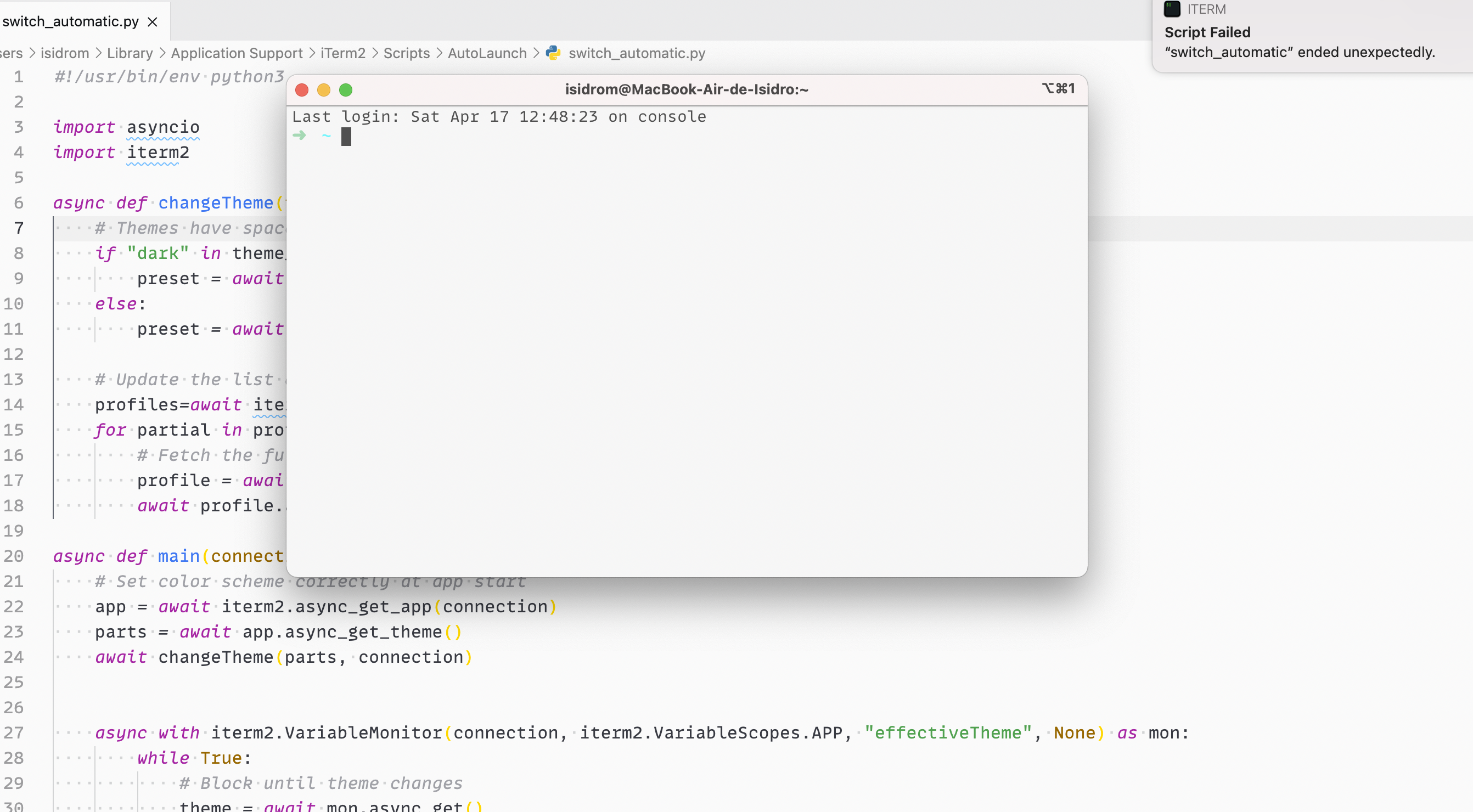
ended unexpectedly