-
-
Save FredrikOseberg/c1e8ec83ade6e89ca84882e33caf599c to your computer and use it in GitHub Desktop.
// MessageParser starter code | |
class MessageParser { | |
constructor(actionProvider, state) { | |
this.actionProvider = actionProvider; | |
this.state = state; | |
} | |
parse(message) { | |
console.log(message) | |
} | |
} | |
export default MessageParser; | |
// ActionProvider starter code | |
class ActionProvider { | |
constructor( | |
createChatBotMessage, | |
setStateFunc, | |
createClientMessage, | |
stateRef, | |
createCustomMessage, | |
...rest | |
) { | |
this.createChatBotMessage = createChatBotMessage; | |
this.setState = setStateFunc; | |
this.createClientMessage = createClientMessage; | |
this.stateRef = stateRef; | |
this.createCustomMessage = createCustomMessage; | |
} | |
} | |
export default ActionProvider; | |
// Config starter code | |
import { createChatBotMessage } from "react-chatbot-kit"; | |
const config = { | |
initialMessages: [createChatBotMessage(`Hello world`)] | |
} | |
export default config |
The package has been updated to version 2.0. You now need to import an external css sheet. Check the updated documentation for instructions. man. 25. okt. 2021 kl. 06:28 skrev urvinprototeam @.***
…
: @.**** commented on this gist. ------------------------------ Hello Fredrik, css in chatbot does not work for me. [image: Screen Shot 2021-10-25 at 10 55 16] https://user-images.githubusercontent.com/70262539/138634609-d2e31ce0-b117-4e6e-b488-d152db27a085.png — You are receiving this because you authored the thread. Reply to this email directly, view it on GitHub https://gist.github.com/c1e8ec83ade6e89ca84882e33caf599c#gistcomment-3938257, or unsubscribe https://github.com/notifications/unsubscribe-auth/AD2WIPRKVHXEUDOP2VNVJYTUITME7ANCNFSM4OZODMXA .
Thanks a lot Fredrik. It is work now.
Hi, is there a way I get the whole css file?
Hi, I try to change the configuration in the 'react-chatbot-kit/build/main.css' but the chatbot is not updated.
For example, I try to change the width of .react-chatbot-kit-chat-container from default 275px to 500px, but there is nothing change in the chatbot at all.
Hi Fredrik, I am creating the chatbot with your guidelines. I just want to know how can we make it like it's dialogue box can be open or closed by clicking the widget like "messenger". Can you help me out?
hi reply please
how to add this in a dialogue box
To anyone who is trying to use this with a platform that uses server-side rendering (Next.js etc), they may have better luck creating this as a dynamic import component and disabling SSR:
import dynamic from 'next/dynamic'
const ChatbotComponentWithNoSSR = dynamic(
() => import('../Components/Chatbot'), // Path to your Chatbot component
{ ssr: false }
)
https://nextjs.org/docs/advanced-features/dynamic-import#with-no-ssr
Also for those having issues with styles, check the docs for v2 with external stylesheet import:
import Chatbot from 'react-chatbot-kit';
import 'react-chatbot-kit/build/main.css';
https://fredrikoseberg.github.io/react-chatbot-kit-docs/docs/
Great project by the way!
Hello! I've been following your tutorial and it's worked great. I'm just having one problem I hope you can help me out with. Currently the chatbot is displaying correctly on my browser, but when I'm receiving an error that is causing the bot to not appear on my mobile version in Expo Go. Any ideas as to why it's not working? Thank you.
I'm having this issue of sending empty user messages when clicking on the send button. Have any suggestion on how I can avoid this @FredrikOseberg
Hello @FredrikOseberg, I have a question of how you can access the messages when trying to use localStorage? The documentation didn't bring it out clearly for me. Can you please help me?
Thanks
Hii @FredrikOseberg, Is there any way to use dynamic Backend data into your chatbot ?
how can I resolved these
how can I resolved these
I see the same
Hi Fredrik, I am creating the chatbot with your guidelines. I just want to know how can we make it like it's dialogue box can be open or closed by clicking the widget like "messenger". Can you help me out?
Hey were you able to do the same?
The package has been updated to version 2.0. You now need to import an external css sheet. Check the updated documentation for instructions. man. 25. okt. 2021 kl. 06:28 skrev urvinprototeam @.***
…
: @.**** commented on this gist. ------------------------------ Hello Fredrik, css in chatbot does not work for me. [image: Screen Shot 2021-10-25 at 10 55 16] https://user-images.githubusercontent.com/70262539/138634609-d2e31ce0-b117-4e6e-b488-d152db27a085.png — You are receiving this because you authored the thread. Reply to this email directly, view it on GitHub https://gist.github.com/c1e8ec83ade6e89ca84882e33caf599c#gistcomment-3938257, or unsubscribe https://github.com/notifications/unsubscribe-auth/AD2WIPRKVHXEUDOP2VNVJYTUITME7ANCNFSM4OZODMXA .
Hi @FredrikOseberg I am getting same error can you please let me know how can add css to this bot
Hii I getting error like this Cannot read properties of undefined (reading 'createChatBotMessage')
Hi, @FredrikOseberg , Does this library works with react-native? Because I am not able to get resources on it.
Hi, @FredrikOseberg , Does this library works with react-native? Because I am not able to get resources on it.
yes even I am trying to do the same, any support for react native?
Hi, @FredrikOseberg , Does this library works with react-native? Because I am not able to get resources on it.
yes even I am trying to do the same, any support for react native?
Hi @devansh-nigam , it does not work for react-native.
Hello @FredrikOseberg, I have a question of how you can access the messages when trying to use localStorage? The documentation didn't bring it out clearly for me. Can you please help me? Thanks
Hi.....!!! Did you able to solve it.........????
config.js file
import React from "react";
import { createChatBotMessage } from "react-chatbot-kit";
import Options from "../Options/Options"
const config = {
botName: "LearningBot",
initialMessages: [
createChatBotMessage("Hello, Welcome to student info system!", {
widget: "options",
})
],
widgets: [
{
widgetName: "options",
widgetFunc: (props) => <Options {...props} />,
},
{
widgetName: "gotIt",
widgetFunc: (props) => <Options {...props} />,
}
]
};
export default config;
ActionProvider.js file
class ActionProvider {
constructor(createChatBotMessage, setStateFunc) {
this.createChatBotMessage = createChatBotMessage;
this.setState = setStateFunc;
}
greet() {
const greetingMessage = this.createChatBotMessage("Hi, friend.")
this.updateChatbotState(greetingMessage)
}
handleGotIt() {
const message = this.createChatBotMessage("Great! I'm glad I was able to help.",{
widget: "gotIt",
})
this.updateChatbotState(message)
}
updateChatbotState(message) {
this.setState(prevState => ({
...prevState, messages: [...prevState.messages, message]
}))
}
}
export default ActionProvider;
Options.js file
import React from "react";
import "./Options.css";
const Options = (props) => {
const options = [
{
text: "Got It!",
handler: props.actionProvider.handleGotIt,
id: 1,
},
{
text: "Exit",
handler: () => {},
id: 2,
},
{
text: "Go Back",
handler: () => {},
id: 3,
},
];
const buttonsMarkup = options.map((option) => (
{option.text}
));
return
};
export default Options;
MessageParesr.js file
class MessageParser {
constructor(actionProvider, state) {
this.actionProvider = actionProvider;
this.state = state;
}
parse(message) {
const lowerCaseMessage = message.toLowerCase();
if (lowerCaseMessage.includes("hello")) {
this.actionProvider.greet();
}
if (lowerCaseMessage.includes("got it")) {
this.actionProvider.handleGotIt();
}
}
}
export default MessageParser;
App.js File
import React from 'react';
import {Chatbot} from "react-chatbot-kit"
import 'react-chatbot-kit/build/main.css';
import './App.css';
import config from './Chatbot/config';
import ActionProvider from './Chatbot/ActionProvider';
import MessageParser from './Chatbot/MessageParser';
function App() {
return (
);
}
export default App;
I am getting an error on clicking the got it button
Cannot read properties of undefined (reading 'createChatBotMessage')
TypeError: Cannot read properties of undefined (reading 'createChatBotMessage')
at handleGotIt (http://localhost:3000/main.aeced91d0d525144c6c2.hot-update.js:27:26)
at HTMLUnknownElement.callCallback (http://localhost:3000/static/js/bundle.js:11944:18)
at Object.invokeGuardedCallbackDev (http://localhost:3000/static/js/bundle.js:11988:20)
at invokeGuardedCallback (http://localhost:3000/static/js/bundle.js:12045:35)
at invokeGuardedCallbackAndCatchFirstError (http://localhost:3000/static/js/bundle.js:12059:29)
at executeDispatch (http://localhost:3000/static/js/bundle.js:16203:7)
at processDispatchQueueItemsInOrder (http://localhost:3000/static/js/bundle.js:16229:11)
at processDispatchQueue (http://localhost:3000/static/js/bundle.js:16240:9)
at dispatchEventsForPlugins (http://localhost:3000/static/js/bundle.js:16249:7)
at http://localhost:3000/static/js/bundle.js:16409:16
Please help me with the code as soon as possible
The package has been updated to version 2.0. You now need to import an external css sheet. Check the updated documentation for instructions. man. 25. okt. 2021 kl. 06:28 skrev urvinprototeam @.***
…
: @.**** commented on this gist. ------------------------------ Hello Fredrik, css in chatbot does not work for me. [image: Screen Shot 2021-10-25 at 10 55 16] https://user-images.githubusercontent.com/70262539/138634609-d2e31ce0-b117-4e6e-b488-d152db27a085.png — You are receiving this because you authored the thread. Reply to this email directly, view it on GitHub https://gist.github.com/c1e8ec83ade6e89ca84882e33caf599c#gistcomment-3938257, or unsubscribe https://github.com/notifications/unsubscribe-auth/AD2WIPRKVHXEUDOP2VNVJYTUITME7ANCNFSM4OZODMXA .Hi @FredrikOseberg I am getting same error can you please let me know how can add css to this bot
PREFER DOC: https://fredrikoseberg.github.io/react-chatbot-kit-docs/docs/
import 'react-chatbot-kit/build/main.css';
@pinki-mandal Thanks for providing Style
@ManishaSwain8 thanks for the reference doc !
Hi @FredrikOseberg - great work with the chatbot component. Have a question: can the chatbot component pass data back to its parent component, or raise an event when a new user message is added to the component?
Hey @FredrikOseberg - great package. I am facing one issue, I created a widget to show the links in each chatbot response but whenever I am generating a new response the previous all the widget responses in the UI are getting updated with the new links.
How can I resolve this
Hello Fredrik, css in chatbot does not work for me.
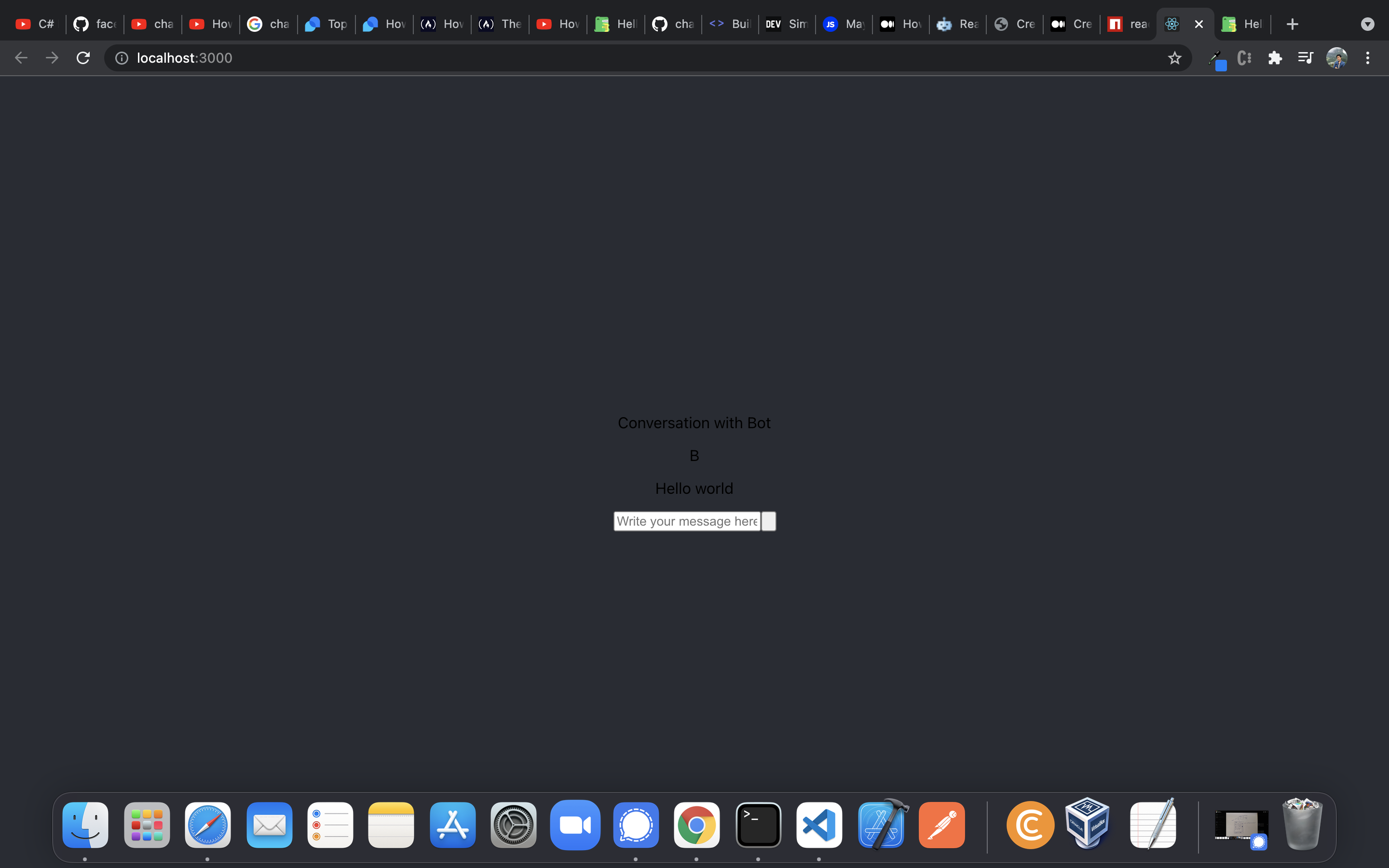