Created
April 7, 2020 15:32
-
-
Save GregoireHebert/bd99e073818fd88180f5e9d3845e38dd to your computer and use it in GitHub Desktop.
API-Platform calculated fields
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
namespace App\Entity; | |
use ApiPlatform\Core\Annotation\ApiResource; | |
use Doctrine\ORM\Mapping as ORM; | |
use Symfony\Component\Serializer\Annotation\Groups; | |
use Symfony\Component\Validator\Constraints as Assert; | |
/** | |
* @ApiResource( | |
* collectionOperations={ | |
* "get" = {"normalization_context" = {"groups" = "Greeting:Collection:Get"}}, | |
* } | |
* ) | |
* @ORM\Entity | |
*/ | |
class Greeting | |
{ | |
/** | |
* @var int The entity Id | |
* | |
* @ORM\Id | |
* @ORM\GeneratedValue | |
* @ORM\Column(type="integer") | |
* @Groups("Greeting:Collection:Get") | |
*/ | |
private $id; | |
private $a = 1; | |
private $b = 2; | |
/** | |
* @var string A nice person | |
* | |
* @ORM\Column | |
* @Groups("Greeting:Collection:Get") | |
*/ | |
public $name = ''; | |
public function getId(): int | |
{ | |
return $this->id; | |
} | |
/** | |
* @return int sum | |
* @Groups("Greeting:Collection:Get") <- MAGIC IS HERE, You can set a group on a method. | |
*/ | |
public function getSum() | |
{ | |
return $this->a+$this->b; | |
} | |
} |
in YAML
# api/config/api_platform/resources.yaml
App\Entity\Greeting:
attributes:
normalization_context:
groups: ['greeting:collection:get']
# api/config/serialization/Greeting.yaml
App\Entity\Greeting:
attributes:
id:
groups: ['greeting:collection:get']
name:
groups: ['greeting:collection:get']
sum:
groups: ['greeting:collection:get']
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
You'll get as result, the calculated field.
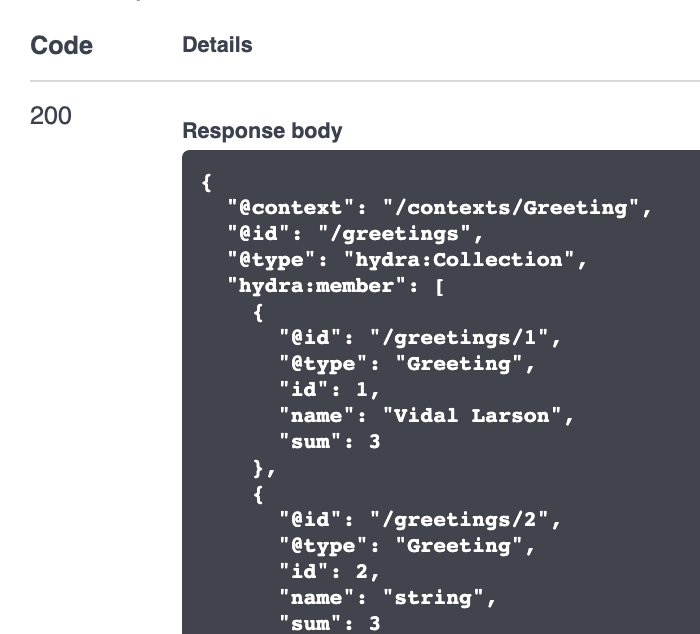