Created
July 25, 2020 23:09
-
-
Save Hubcapp/a63f90d9fe846b20ec50f588197a999f to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import os | |
from os.path import isfile, join | |
import datetime | |
from time import sleep | |
import tkinter | |
from tkinter import messagebox | |
mypath = r"C:\Users\Tyler\Documents\GPUFanSpeed" | |
waitTime = 20 | |
# hide "main window" for message box errors | |
root = tkinter.Tk() | |
root.withdraw() | |
messagebox.showinfo("Service Started", "cool, the service started.") | |
while True: | |
# retrieve all log files | |
onlyfiles = [f for f in os.listdir(mypath) if isfile(join(mypath, f))] | |
# remove old log files to save space | |
latestFile = onlyfiles[-1:][0] | |
for filename in onlyfiles: | |
if filename != latestFile: | |
print("deleting old file: " + filename) | |
os.remove(mypath + "\\" + filename) | |
# fetch last line of file quickly, this will be the latest fan speeds | |
with open(mypath + "\\" + latestFile, 'rb') as f: | |
f.seek(-2, os.SEEK_END) | |
while f.read(1) != b'\n': | |
f.seek(-2, os.SEEK_CUR) | |
last_line = f.readline().decode().split(",") | |
timeLogged = last_line[0][:-3] | |
gpuFan = last_line[1] | |
psuFan = last_line[2][:-2] | |
print("Time: " + timeLogged) | |
print("GPU Fan Speed: " + gpuFan) | |
print("PSU Fan Speed: " + psuFan) | |
# check how long ago these values were logged, to make sure that iCUE is doing its job populating the file. | |
timeLoggedDateTime = datetime.datetime.strptime(timeLogged, '%d/%m/%Y %H:%M:%S') | |
howOld = (datetime.datetime.now() - timeLoggedDateTime).total_seconds() | |
if howOld > waitTime * 3: | |
messagebox.showerror("iCUE watcher", "iCUE is not logging!") | |
# alert user if their system temperatures are higher than they need to be due to the harmful "zero fan mode" | |
# This leads to an early death of the hardware, and higher profits for hardware manufacturers | |
if gpuFan == "0RPM": | |
messagebox.showerror("GPU Zero RPM Warning", "warning: harmful zero fan mode is running on the GPU!") | |
if psuFan == "0RPM": | |
messagebox.showerror("PSU Zero RPM Warning", "warning: harmful zero fan mode is running on the PSU!") | |
sleep(waitTime) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
You will have to change the directory & set up logging in iCUE.
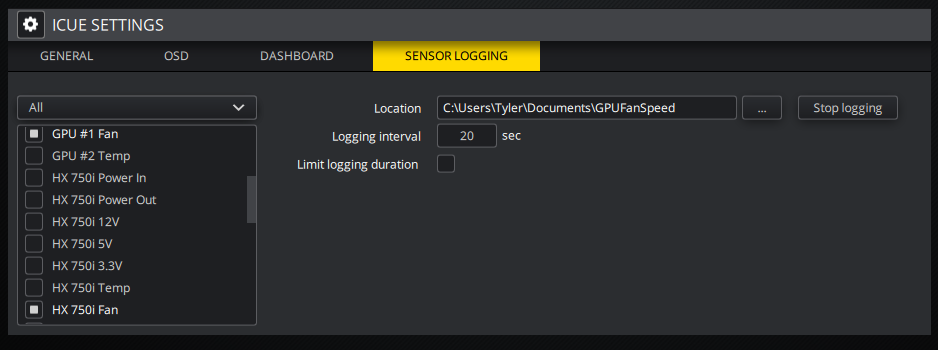