Last active
September 9, 2021 07:29
-
-
Save Inventsable/6c6df669f6357e17c87820b2a8f2c54a to your computer and use it in GitHub Desktop.
Recursively sort all layers in Illustrator alphabetically
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
var deep = true, // Set to false if you don't want to recursively sort layers within layers too | |
isLowerFirst = true; // Set it to false if you want to place the layers with the first uppercase letter above | |
// Convert ILST collection into standard Array so we can use Array methods | |
function get(type, parent) { | |
if (arguments.length == 1 || !parent) parent = app.activeDocument; | |
var result = []; | |
if (!parent[type]) return result; | |
for (var i = 0; i < parent[type].length; i++) result.push(parent[type][i]); | |
return result; | |
} | |
// Polyfill for Array.forEach | |
Array.prototype.forEach = function (callback) { | |
for (var i = 0; i < this.length; i++) callback(this[i], i, this); | |
}; | |
function sortLayersInside(parent) { | |
function isUpperCase(text) { | |
return /[A-Z]/.test(text.charAt(0)) | |
} | |
function isLowerCase(text) { | |
return /[a-z]/.test(text.charAt(0)) | |
} | |
function hasMixedCase(a, b) { | |
if (a.charAt(0).toLowerCase() !== b.charAt(0).toLowerCase()) return false; | |
return isUpperCase(a) && isLowerCase(b) ? true : isLowerCase(a) && isUpperCase(b); | |
} | |
function handleMixedCase(a, b) { | |
var result = a === b ? 0 : isUpperCase(a) ? 1 : -1; | |
return isLowerFirst ? result : result * -1; | |
} | |
get("layers", parent) | |
.sort(function (a, b) { | |
return hasMixedCase(a.name, b.name) ? | |
handleMixedCase(a.name, b.name) : | |
a.name.toLowerCase().localeCompare(b.name.toLowerCase()) | |
}) | |
.forEach(function (layer) { | |
layer.zOrder(ZOrderMethod.SENDTOBACK); | |
if (layer.layers && layer.layers.length && deep) sortLayersInside(layer); | |
}); | |
} | |
sortLayersInside(); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
I manually look at the result of sorting mixed alphabets with .localeCompare() and .toUpperCase(). I didn't check Chinese characters, because I don't know the correct order =)
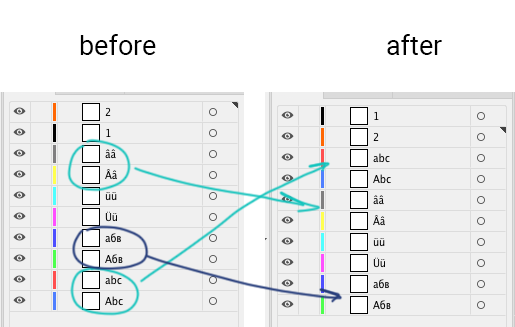