Last active
October 6, 2017 12:56
-
-
Save ItsaMeTuni/f418b78f498cff8acb8f9234debfb613 to your computer and use it in GitHub Desktop.
This is a simple class used to bind delegates to a button and invoke that delegate when the button is pressed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* | |
Then attach this class to that object. | |
How this code works: | |
Create a button by going into Edit > Project Settings > Input and add a button. | |
You bind a delegate to that button. | |
Then when this class's update function is called it will check if the button is pressed. | |
If it is pressed then the delegate assigned to the button will be invoked. | |
*/ | |
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
public delegate void BtnPressed(); | |
/** | |
This class invokes delegates for buttons when they are fully pressed. | |
Example to bind a function (recommended to do this at the start function of your class): | |
\code | |
InputHandler.AttachFuncToAxis("FooButton", Foo); //Note that you don't need a reference to an InputReference instance since this method is static. | |
public void Foo() | |
{ | |
//Do your stuff when the button is pressed | |
} | |
\endcode | |
*/ | |
public class InputHandler : MonoBehaviour { | |
/**Dictionary that stores delegates assigned to buttons.*/ | |
public Dictionary<string, BtnPressed> delegateList = new Dictionary<string, BtnPressed>(); | |
// Update is called once per frame | |
void Update () | |
{ | |
//Create array of the keys from the delegateList dictionary | |
string[] delegateListKeys = new string[delegateList.Count]; | |
delegateList.Keys.CopyTo (delegateListKeys, 0); | |
//Iterate over that array | |
foreach (string key in delegateListKeys) | |
{ | |
//If the axis has a value of 1 and its state is false | |
if (Input.GetButtonDown (key)) | |
{ | |
//Invoke delegate | |
delegateList[key] (); | |
} | |
} | |
} | |
/** | |
Assign a function to a button. | |
\param button the button name. | |
\param del the delegate. | |
*/ | |
public static void AttachFuncToBtn(string button, BtnPressed del) | |
{ | |
GameObject.FindObjectOfType<InputHandler> ().delegateList.Add (button, del); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here's an axis I created that I use to toggle my game's inventory GUI.
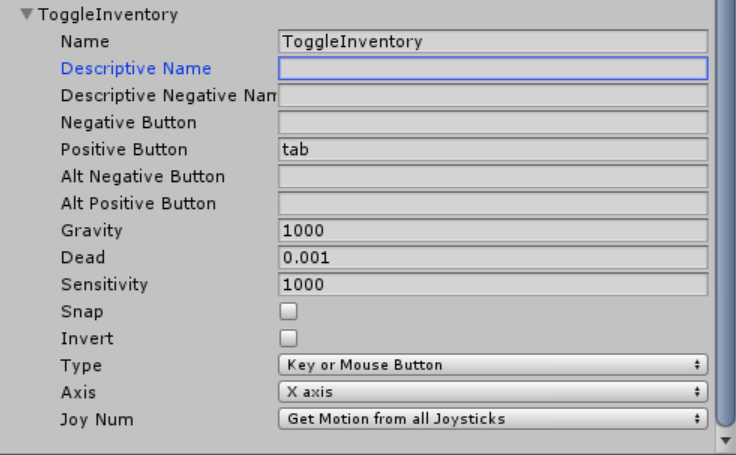