Last active
May 12, 2024 09:21
-
-
Save Jimbly/9f6c6a0d9414310347f2803902ac7bb7 to your computer and use it in GitHub Desktop.
Steam CD Key Batch query
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* jshint esversion: 6*/ | |
const assert = require('assert'); | |
const async = require('async'); | |
const fs = require('fs'); | |
let request = require('request'); | |
const FileCookieStore = require('tough-cookie-filestore'); | |
if (!fs.existsSync('cookies.json')) { fs.writeFileSync('cookies.json', '{}'); } | |
let j = request.jar(new FileCookieStore('cookies.json')); | |
request = request.defaults({ jar : j }); | |
// Fill cookie string here by grabbing it from a request in Chrome | |
// Should look like 'sessionid=c7...; steamLogin=2...; ...' | |
let cookies_from_browser = 'fill=me; in=please'; // Make sure not to check this in | |
let cookie_url = 'https://partner.steamgames.com'; | |
cookies_from_browser.split('; ').forEach(function (cookie) { | |
j.setCookie(cookie, cookie_url); | |
}); | |
function queryKeys(next) { | |
let keys = fs.readFileSync('keys.csv', 'utf8').split('\n').map(function (s) { | |
return s.trim(); | |
}); | |
let out = []; | |
async.eachSeries(keys, function (key, next) { | |
if (!key) { | |
console.log(''); | |
out.push(''); | |
return void next(); | |
} | |
let retries = 0; | |
function doAttempt() { | |
let url = `https://partner.steamgames.com/querycdkey/cdkey?cdkey=${key}&method=Query`; | |
request({ | |
url: url, | |
headers: { | |
'user-agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/78.0.3904.97 Safari/537.36', | |
}, | |
}, function (err, resp, body) { | |
if (err) { | |
throw err; | |
} | |
let result = body.split('<h2>Activation Details</h2>')[1]; | |
if (!result && !err) { | |
if (retries < 5) { | |
++retries; | |
console.log(`Error, retrying ${retries}...`); | |
return doAttempt(); | |
} | |
fs.writeFileSync('out.html', body); | |
console.log('Error quering CD Key, check out.html to see the response returned from Steamworks,'); | |
console.log(' if it is a login page, your cookies_from_browser string is probably invalid'); | |
process.exit(-1); | |
} | |
assert(result); | |
result = result.split('</table>')[0]; | |
result = result.match(/<td>.*<\/td>/gu); | |
result = result.map(function (line) { | |
return line.replace(/<[^>]*>/gu, ''); | |
}); | |
let line = [key, (result[0] === 'Activated') ? '"' + result[1] + '"' : result[0]].join('\t'); | |
console.log(line); | |
out.push(line); | |
return next(); | |
}); | |
} | |
doAttempt(); | |
}, function (err) { | |
fs.writeFileSync('out.tsv', out.join('\n')); | |
next(err); | |
}); | |
} | |
queryKeys(function (err) { | |
if (err) { | |
throw err; | |
} else { | |
console.log('Done.'); | |
} | |
}); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"dependencies": { | |
"async": "^2.4.1", | |
"request": "^2.81.0", | |
"tough-cookie-filestore": "0.0.1" | |
} | |
} |
Just wanted to say thank you, helping me sort 55k+ steam keys right now :)
@BrandyBuizel Glad to help, and glad to hear it's still working =)
Great work! :)
There is easier way that don't need you to install anything, and it's derived of this gist here. My revision only needs you to edit the JS code and place in into DevTools console directly. No login cookies and things like that. https://gist.github.com/petersvp/270f7d5d7d548448f4897586a0d389c0
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Instructions for use:
index.js
Cookie:
, should start withsessionid=blah
.steamLoginSecure=blah
)let cookies_from_browser = 'fill=me; in=please';
keys.csv
npm install
node index.js
Any problems or questions? Please contact Jimbly on Steam or GitHub.
Find this script useful? Buy my game on Steam.
Trouble finding your cookie string, look here:
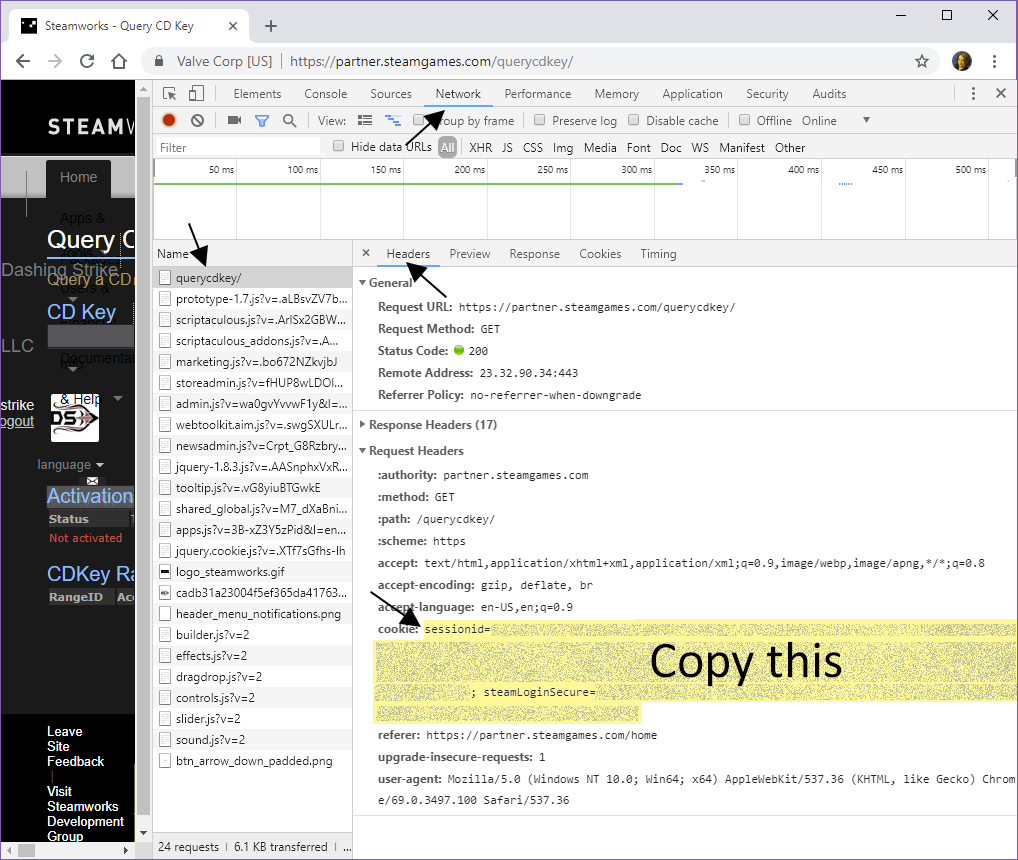