Last active
October 16, 2023 04:55
-
-
Save Kif11/cdd4a68a2133aa42a582 to your computer and use it in GitHub Desktop.
PyQt scaffold for creating dockable Maya window
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from PySide import QtCore | |
from PySide import QtGui | |
from maya.app.general.mayaMixin import MayaQWidgetDockableMixin | |
class MainWindow(MayaQWidgetDockableMixin, QtGui.QMainWindow): | |
def __init__(self, parent=None): | |
super(MainWindow, self).__init__(parent=parent)\ | |
# Main widget | |
main_widget = QtGui.QWidget() | |
main_layout = QtGui.QVBoxLayout() | |
# Create UI widgets | |
self.test_btn = QtGui.QPushButton('Test') | |
# Attach widgets to the main layout | |
main_layout.addWidget(self.test_btn) | |
# Set main layout | |
main_widget.setLayout(main_layout) | |
self.setCentralWidget(main_widget) | |
# Connect buttons signals | |
self.test_btn.clicked.connect(self.on_test_btn_click) | |
def on_test_btn_click(self): | |
print 'Test button was clicked' | |
def main(): | |
w = MainWindow() | |
w.show(dockable=True, floating=False, area='left') |
thanks, can you please show an altered version.
a- loading UI via Qloader .ui file and set it as window or central widget. huge thanks in advance.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
updated version, tested in maya 2022
also added a window title, which enables you to see what the window is about when dragging it in a side dockable bar in maya
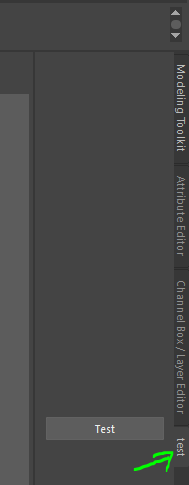