Last active
February 3, 2021 04:35
-
-
Save LRENZ/c3c532dfc1a01860eeca8d4bd00a9a9c to your computer and use it in GitHub Desktop.
when copied/pasted code into the console after loading the page, it will allow to display in the console the name and the price of items shown. If the user scrolls the page, the code should automatically push information of the new products shown to the console.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* | |
* Sample website: https://www.leslipfrancais.fr/maillots-de-bain-326# | |
* If an element is visible, which mean its' top and left both greater than 0 | |
* and its' right and bottom should less than the screen | |
* This is how we know whether an element is on the screen | |
*/ | |
function isVisible(elem) { | |
var location = elem.getBoundingClientRect(); | |
return ( | |
location.top >= 0 && | |
location.left >= 0 && | |
location.bottom <= (window.innerHeight || document.documentElement.clientHeight) && | |
location.right <= (window.innerWidth || document.documentElement.clientHeight) | |
); | |
}; | |
/* | |
* Add EventListener to scroll event | |
* Only console the element which is on screen | |
* By the way, I find out that if we trying to use "windows.addEventListener(scroll)" | |
* It won't work, for reason that the website has used the Scroll-linked effects | |
* "windows.addEventListener(scroll)" has listened to other function | |
* By the way, We don't want to console too many times when scrolling | |
* So we add a new array to save the products which have consoled | |
* Copy and paste the code into Console. You will see that the products information will be displayed in the console when scrolling | |
* If we only need product_name or product_price, we can use String.Split("\n") to get more specific the information | |
* If it doesn't work, try to refresh the page and paste the code again. Or try to test in a higher version of the browser(Chrome or Firefox) | |
* more detail in : https://gist.github.com/LRENZ/c3c532dfc1a01860eeca8d4bd00a9a9c | |
* Add new Version to console the product info without the scolling trigger | |
*/ | |
var temp = [] | |
function extract_element(){ | |
var product = document.getElementsByClassName("product-container"); | |
if (product) { | |
for (var prod of product) { | |
if (isVisible(prod)) { | |
if (!temp.includes(prod.innerText)) { | |
console.log(prod.innerText); | |
temp.push(prod.innerText); | |
} | |
} | |
} | |
} | |
} | |
extract_element() | |
document.addEventListener('scroll', function(event) { | |
extract_element() | |
}); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
When you copied and pasted the code into Console, try to Srcoll, You will See the following:
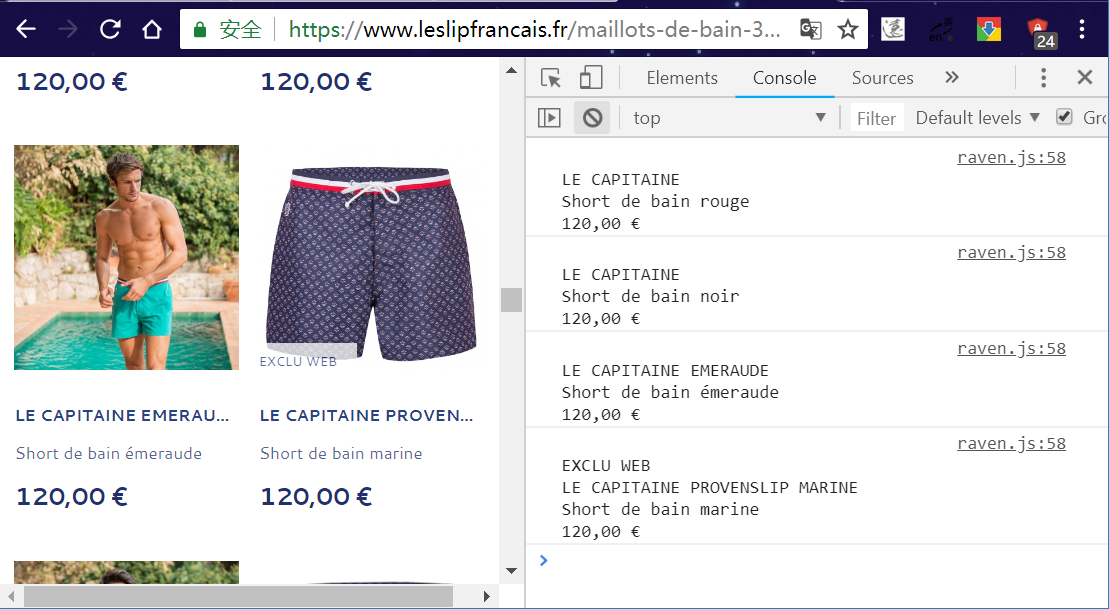
If it doesn't work, try to refresh the page and paste the code again. Or try to test in a higher version of the browser(Chrome or Firefox)