Last active
October 3, 2023 09:02
-
-
Save LeoAndo/75f79d7e5fc2a0fd9dcc9547ed06e571 to your computer and use it in GitHub Desktop.
[Android] 画面のライフサイクルに対応するサウンドクラス
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package xxx; // TODO 環境に合わせて変更してください | |
import android.content.Context; | |
import android.media.AudioAttributes; | |
import android.media.SoundPool; | |
import android.util.Log; | |
import androidx.annotation.NonNull; | |
import androidx.lifecycle.DefaultLifecycleObserver; | |
import androidx.lifecycle.LifecycleOwner; | |
/** | |
* 画面のライフサイクルに対応するサウンドクラス. | |
*/ | |
public class MainSoundPlayer implements DefaultLifecycleObserver { | |
private static final String LOG_TAG = MainSoundPlayer.class.getSimpleName(); | |
private static final float LEFT_VOLUME_VALUE = 1.0f; // left volume value (range = 0.0 to 1.0) | |
private static final float RIGHT_VOLUME_VALUE = 1.0f;// right volume value (range = 0.0 to 1.0) | |
private static final int SOUND_LOOP_MODE_NO_LOOP = 0; // loop mode (0 = no loop, -1 = loop forever) | |
private static final float SOUND_PLAY_BACK_RATE = 1.0f; // playback rate (1.0 = normal playback, range 0.5 to 2.0) | |
private static final int PRIORITY_1 = 1; | |
private final SoundPool soundPool; | |
private final int clappingSoundId; | |
private int clappingStreamId; | |
public MainSoundPlayer(Context context) { | |
final AudioAttributes audioAttributes = new AudioAttributes.Builder() | |
.setUsage(AudioAttributes.USAGE_MEDIA) | |
.setContentType(AudioAttributes.CONTENT_TYPE_MUSIC) | |
.build(); | |
final int maxStreams = 1; // TODO ストリーム数 (適宜変更してください) | |
soundPool = new SoundPool.Builder() | |
.setAudioAttributes(audioAttributes) | |
.setMaxStreams(maxStreams) | |
.build(); | |
clappingSoundId = soundPool.load(context, R.raw.clapping, PRIORITY_1); // TODO: R.raw.xxx は自分の環境に合わせてください | |
} | |
// 効果音を再生する | |
public void playClappingSound() { | |
clappingStreamId = soundPool.play( | |
clappingSoundId, LEFT_VOLUME_VALUE, RIGHT_VOLUME_VALUE, | |
PRIORITY_1, SOUND_LOOP_MODE_NO_LOOP, SOUND_PLAY_BACK_RATE); | |
} | |
// 効果音を停止する | |
public void stopClappingSound() { | |
soundPool.stop(clappingStreamId); | |
} | |
// 画面表示時に呼ばれる | |
@Override | |
public void onResume(@NonNull LifecycleOwner owner) { | |
Log.d(LOG_TAG, "call onResume"); | |
if (soundPool == null) return; | |
soundPool.autoResume(); // soundPool.autoPause()したときにアクティブだったすべての音声ファイルを再生する. | |
} | |
// 画面非表示時に呼ばれる | |
@Override | |
public void onPause(@NonNull LifecycleOwner owner) { | |
Log.d(LOG_TAG, "call onPause"); | |
if (soundPool == null) return; | |
soundPool.autoPause(); // 全ての再生中の音声ファイルを停止する. | |
} | |
// 画面終了時に呼ばれる | |
@Override | |
public void onDestroy(@NonNull LifecycleOwner owner) { | |
Log.d(LOG_TAG, "call onDestroy release soundPool: " + soundPool); | |
if (soundPool == null) return; | |
soundPool.release(); // SoundPoolによって使用されているすべてのメモリとネイティブリソースを解放する. | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
使用した効果音データ
https://taira-komori.jpn.org/human01.html
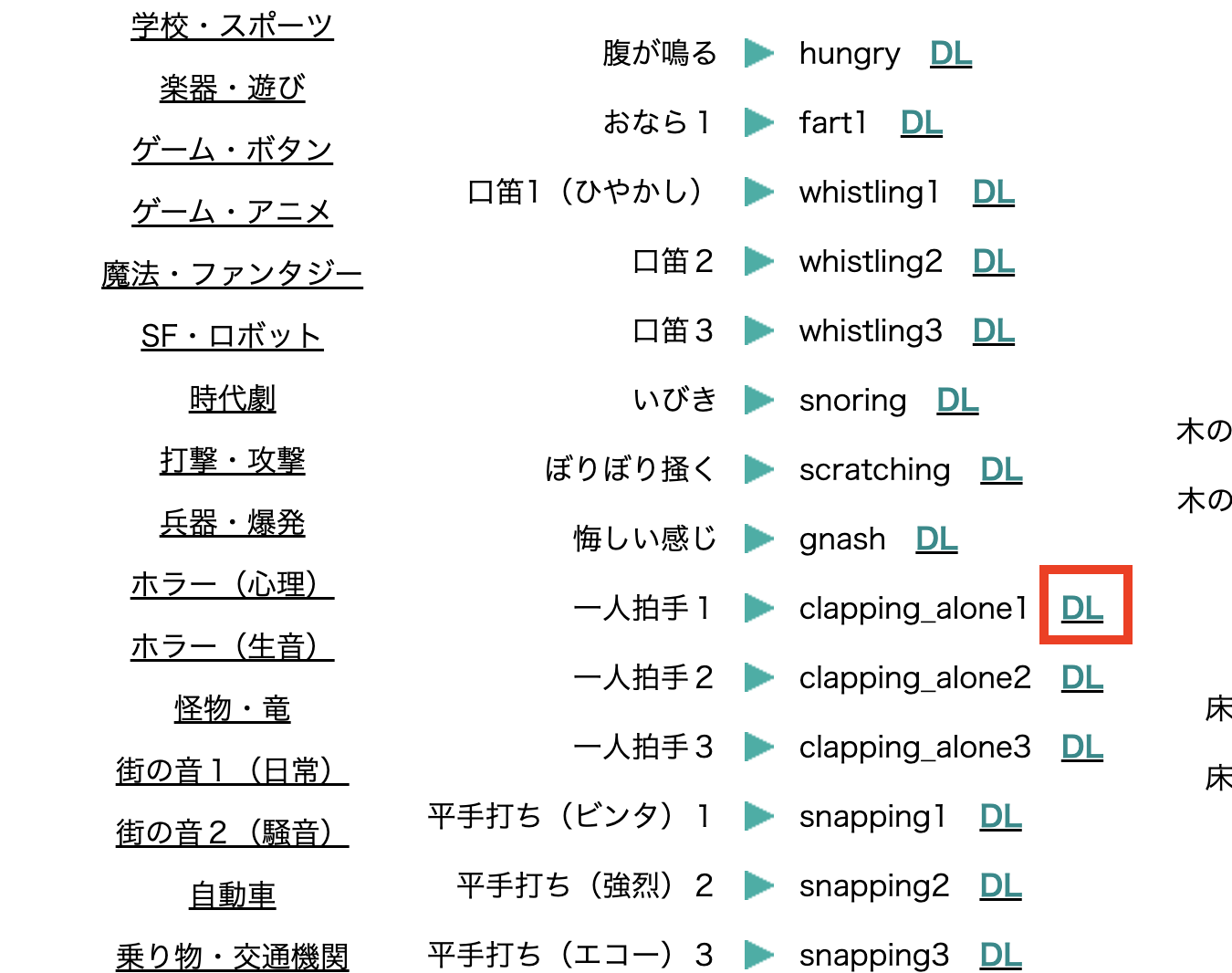
使い方
rawディレクトリをres配下に作成する
MainActivity.java
activity_main.xml
capture API 30 (OS 11)
STARTボタンを押したら効果音が再生される
STOPボタンを押したら効果音が停止される
device-2023-10-03-180056.webm