Created
November 19, 2019 16:12
-
-
Save LiLejia/31e5457843deb4e862bbd0206bedef09 to your computer and use it in GitHub Desktop.
Classic slide to unlock animation swift implemntation
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// | |
// ViewController.swift | |
// GradientAnimation | |
// | |
// Created by Henry Lee on 2019/11/19. | |
// Copyright © 2019 Henry Lee. All rights reserved. | |
// | |
import UIKit | |
class ViewController: UIViewController { | |
override func viewDidLoad() { | |
super.viewDidLoad() | |
let height:CGFloat = 30.0 | |
let someView:UIView = UIView(frame: CGRect(x: 0, y: (view.frame.height-height)/2.0, | |
width: view.frame.width, height: height)) | |
let gradientLayer:CAGradientLayer = CAGradientLayer() | |
gradientLayer.colors = [UIColor.black.cgColor,UIColor.white.cgColor,UIColor.black.cgColor] | |
gradientLayer.locations = [0.0,0.5,1.0] | |
gradientLayer.type = .axial | |
gradientLayer.startPoint = CGPoint(x:0.0,y:0.5) | |
gradientLayer.endPoint = CGPoint(x:1.0,y:0.5) | |
gradientLayer.frame = CGRect(x: -someView.bounds.width, y: someView.bounds.minY, | |
width: someView.bounds.width*3, height: someView.bounds.height) | |
someView.layer.addSublayer(gradientLayer) | |
someView.layer.masksToBounds = true | |
let gradientAnimation = CABasicAnimation(keyPath: "locations") | |
gradientAnimation.fromValue = [0.0,0.0,0.25] | |
gradientAnimation.toValue = [0.75,1.0,1.0] | |
gradientAnimation.duration = 3.0 | |
gradientAnimation.repeatCount = Float.infinity | |
gradientLayer.add(gradientAnimation, forKey: nil) | |
let textAttributes: [NSAttributedString.Key: Any] = { | |
let style = NSMutableParagraphStyle() | |
style.alignment = .center | |
return [ | |
NSAttributedString.Key.font: UIFont( | |
name: "HelveticaNeue-Thin", | |
size: 28.0)!, | |
NSAttributedString.Key.paragraphStyle: style | |
] | |
}() | |
let image = UIGraphicsImageRenderer(size: someView.bounds.size) | |
.image { _ in | |
"Slide to unlock".draw(in: someView.bounds, withAttributes: textAttributes) | |
} | |
let maskLayer = CALayer() | |
maskLayer.backgroundColor = UIColor.clear.cgColor | |
maskLayer.frame = someView.bounds.offsetBy(dx: someView.bounds.size.width, dy: 0) | |
maskLayer.contents = image.cgImage | |
gradientLayer.mask = maskLayer | |
self.view.addSubview(someView) | |
} | |
} | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Effect: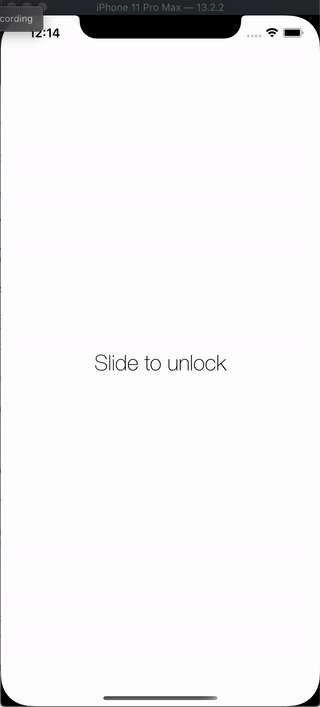