Created
May 22, 2021 10:53
-
-
Save LuisThiamNye/c92e6dca8319b2e88d119806dfd4b861 to your computer and use it in GitHub Desktop.
Roam/Render component for showing the days until a date.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
(ns com.luisthiamnye.roam-render.days-until | |
(:require | |
[roam.datascript :as d] | |
[clojure.string])) | |
(defn inherited-uids [uid] | |
(d/q '[:find [?uids ...] | |
:in $ ?uid | |
:where | |
[?b :block/uid ?uid] | |
(or-join [?b ?uids] | |
(and [?b :block/refs ?brefs] | |
[?brefs :block/uid ?uids]) | |
(and [?b :block/parents ?p] | |
(or-join [?p ?uids] | |
(and [?p :block/refs ?prefs] | |
[?prefs :block/uid ?uids]) | |
[?p :block/uid ?uids])))] | |
uid)) | |
(defn get-date [uid] | |
(-> (inherited-uids uid) | |
(->>(into [] | |
(comp | |
(map (fn [x] | |
(let [[_ m d y] (re-find #"(\d\d)-(\d\d)-(\d\d\d\d)" x)] | |
[y m d]))) | |
(filter (fn [x] | |
(some some? x)))))) | |
first | |
(as-> x (js/Date. (clojure.string/join \- x))))) | |
(defn days-between [d1 d2] | |
(.round js/Math (/ (- d2 d1) 1000 60 60 24))) | |
(defn _ [{:keys [block-uid]}] | |
(let [days-until (days-between (js/Date. (.toDateString (js/Date.))) | |
(get-date block-uid))] | |
[:span {:style {:background "gold" | |
:color "rgb(111,78,55)" | |
:padding "2px 5px" | |
:border-radius "3px" | |
:box-shadow "1px 1px 3px rgba(0 0 0 / 0.1)"}} | |
[:b | |
(when (pos? days-until) "In ") | |
[:span {:style {:color "rgb(64,40,22)"}} | |
(if (zero? days-until) | |
"Today" | |
(.abs js/Math days-until))] | |
(when-not (zero? days-until) " Days") | |
(when (neg? days-until) | |
" ago")]])) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Used like any other roam/render component:
{{[[roam/render]]: ((block-ref-of-clojure-codeblock))}}
The component searches for the closest [[page link]] to a daily notes page throughout the block ancestry. If there are no page links, it can also use the date corresponding to the daily note page it is within.
Examples demonstrating how it works:
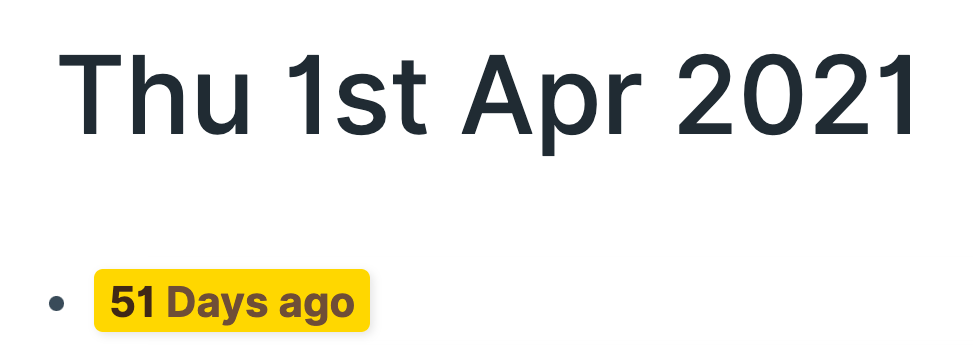
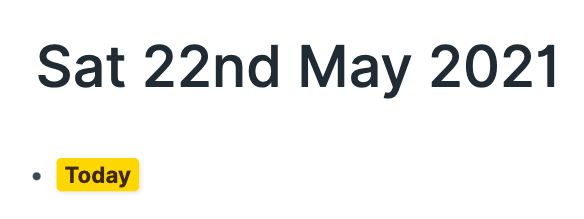
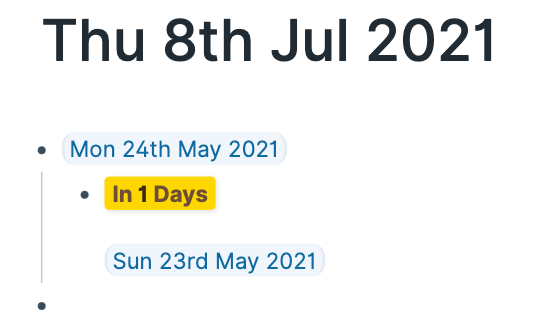
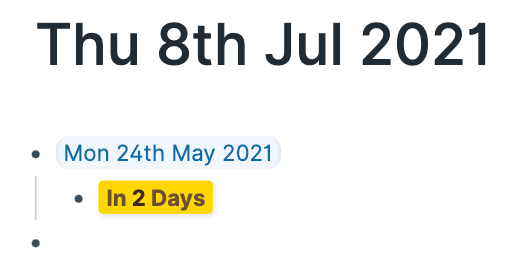
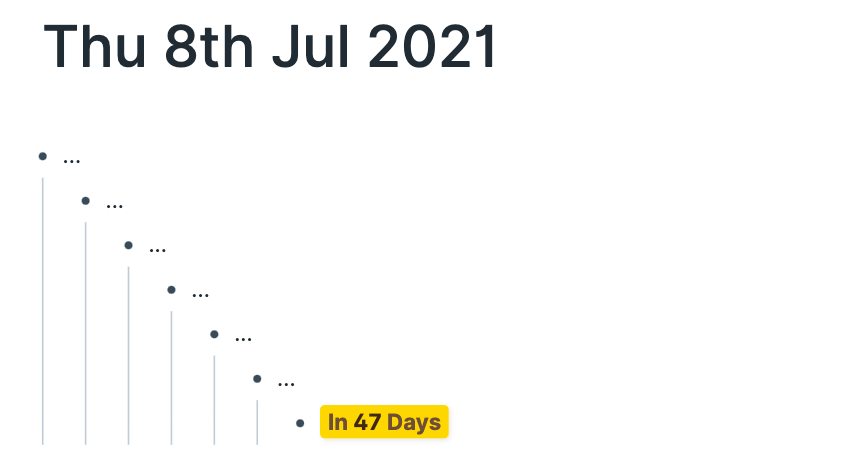
You can easily customise the colours by changing a few parts of the code.
Some ideas for improvement:
Note: for custom page formats I use https://github.com/thesved/ViktoRoam