(4) "Deal 4 damage to an enemy and 1 damage to all other enemies."
- Create a new Conditional SO to filter targets that are not the previous one
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using TcgEngine.AI;
namespace TcgEngine
{
[CreateAssetMenu(fileName = "condition", menuName = "TcgEngine/Condition/ConditionNotLastTarget", order = 10)]
public class ConditionNotLastTarget : ConditionData
{
public override bool IsTargetConditionMet(Game data, AbilityData ability, Card caster, Card target)
{
return target.uid != data.last_selected_target;
}
public override bool IsTargetConditionMet(Game data, AbilityData ability, Card caster, Player target)
{
return target.player_id.ToString() != data.last_selected_target;
}
}
}
-
Create a new conditional using the previous SO
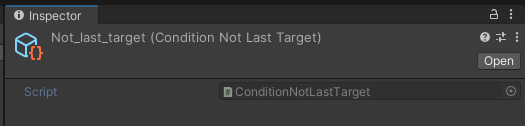
-
Create a new Ability "spell_damage1_all" that deals 1 damage, conditioned to "is_not_empty", "is_enemy" and "not_last_target"
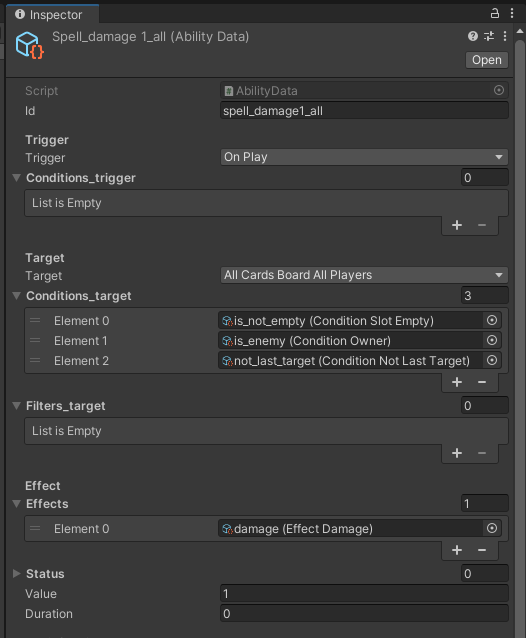
-
Create a new Ability "spell_swap" that deals 4 damage and have "spell_damage1_all" as chain ability
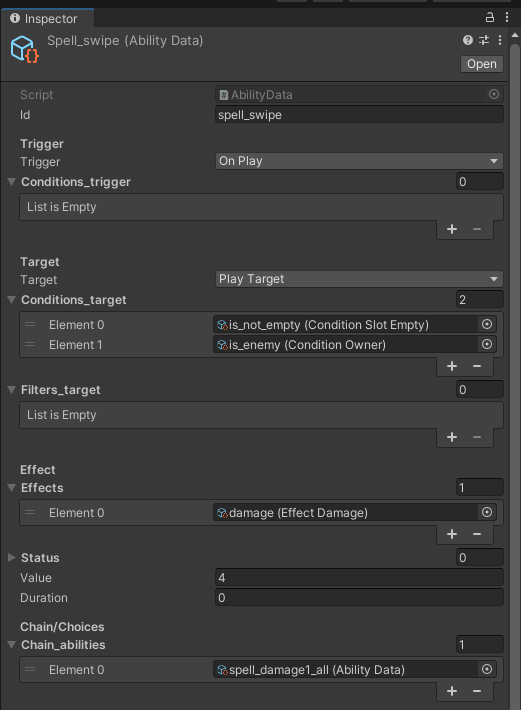
-
Create a new Card "swap"
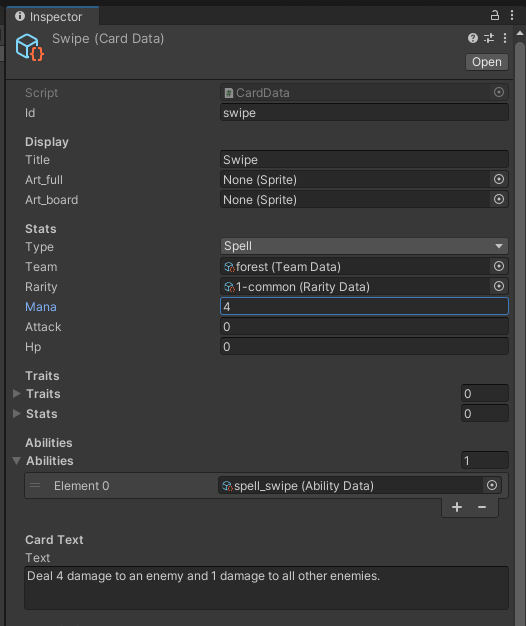
Extra
In order to make swipe work as in Hearthstone we will need to add a property to store the last selected target that could be a player or a card.
- Add a new Enum value to
AbilityTarget
(AbilityData.cs)
AllCardsBoardAllPlayers = 9,
- In AbilityData.cs we also need to change some conditions, and add AreTagetConditionsMet to be able to use conditions in AllPlayers and AllCardsBoardAllPlayers options
// 286 Line (GetCardTargets)
if (target == AbilityTarget.AllCardsBoard || target == AbilityTarget.SelectTarget || target == AbilityTarget.AllCardsBoardAllPlayers)
// 395 Line (GetPlayerTargets)
else if (target == AbilityTarget.AllPlayers || target == AbilityTarget.AllCardsBoardAllPlayers)
{
foreach (Player player in data.players)
{
if (AreTargetConditionsMet(data, caster, player))
targets.Add(player);
}
}
- In Game.cs we need to add the new variable to store last_selected_target
public string last_selected_target;
// 492 (Clone)
dest.last_selected_target = source.last_selected_target;
- In GameLogic.cs we will add the ability to store the last_selected_target and update some conditions to include the new Enum
// 313 Line (ClearTurnData)
game_data.last_selected_target = null;
// 1015 Line (ResolveCardAbilityPlayTarget)
if (iability.CanTarget(game_data, caster, tplayer)) {
game_data.last_selected_target = tplayer.player_id.ToString();
ResolveEffectTarget(iability, caster, tplayer);
}
// 1021 Line (ResolveCardAbilityPlayTarget)
if (iability.CanTarget(game_data, caster, slot_card)) {
game_data.last_selected_target = slot_card.uid;
ResolveEffectTarget(iability, caster, slot_card);
}
// 1257 Line (UpdateOngoingAbilities)
if (ability.target == AbilityTarget.AllPlayers || ability.target == AbilityTarget.PlayerOpponent || ability.target == AbilityTarget.AllCardsBoardAllPlayers)
// 1261 Line
if (ability.target == AbilityTarget.AllPlayers || ability.target == AbilityTarget.AllCardsBoardAllPlayers || tp != player.player_id)
// 1272 Line
if (ability.target == AbilityTarget.AllCardsAllPiles || ability.target == AbilityTarget.AllCardsHand || ability.target == AbilityTarget.AllCardsBoard || ability.target == AbilityTarget.AllCardsBoardAllPlayers)
// 1293 Line
if (ability.target == AbilityTarget.AllCardsAllPiles || ability.target == AbilityTarget.AllCardsBoard || ability.target == AbilityTarget.AllCardsBoardAllPlayers)