Created
October 15, 2020 16:22
-
-
Save MarchCorbin/5007f08f8aaa0abfba475bb411fad6b5 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# Problem | |
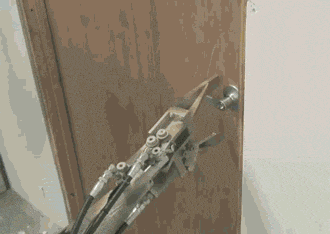 | |
You are working with a computer simulation of a mobile robot. The robot moves on an plane, and its movements are described by a command string consisting of one or more | |
of the following letters: | |
* `G` instructs the robot to move forward one step | |
* `L` instructs the robot to turn left | |
* `R` instructs the robot to turn right | |
The robot CANNOT go backwards - poor robot. After running all of the movement commands, you want to know if the robot returns to its original starting location. | |
For instance, the command `GRGRGRG` would make the robot return to its original starting location. | |
# Instructions | |
1. Copy this markdown and paste in your own, privte gist | |
2. In your private gist, fill out the questions below | |
4. Submit by the due time as instructed in Zoom | |
Do not publish your code on a public repl.it or repo or other public means. | |
## Rewrite the question in your own words: | |
Create a boolean that determines whether the robot is in its starting location given a string of directions for the robot | |
## What assumptions will you make about this problem if you cannot ask any more clarifying questions? What are your reasons for making those assumptions? | |
We will be mapping out a grid and we will be keeping track of the robots orientation (built like a tank). | |
## What are your initial thoughts about this problem? (high level design, 2-3 sentences) | |
Probably be keeping track of location and orientation to help us get to an answer. | |
## How would you identify the elements of this problem? | |
- [ ] Searching of Data | |
- [ ] Sorting of Data | |
- [ ] Pattern Recognition | |
- [x] Build/Navigate a Grid | |
- [ ] Math | |
- [ ] Language API knowledge | |
- [ ] Optimization | |
## Which data structure(s) do you think you'll use? What pros/cons do you see with that choice? | |
Array of coordiates ex: [(0,0), (0,1), (1,0)] | |
Current orientation of the robot will be calculated using an array of strings ['N','E','S','W'] | |
current Orientation: ArrayofStrings[2] === 'Facing south' | |
## Write out a few lines of initial pseudocode: (mid-level design, NOT REAL CODE) | |
Grid out coordinates on a grid. Create conditional logic that sets the direction of the robot based on receiving either an L or an R command. Each coordinate will work off of the previous set of coordinates. | |
if the robot moves forward with a 'G' command and is facing north (1,1) we will subtract 1 from the second integer in the coordiantes (1,0) if the robot is facing south and receives a G command (1,1) will add one to the first integer to (2,1) | |
After all the moving logic is set write a final conditional that checks to see if the final set of given coordinates is equal to the starting position (1,1) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment