Last active
April 21, 2022 21:49
-
-
Save MarwanShehata/e52de8f6f4149025c43f0e291d3cf57e to your computer and use it in GitHub Desktop.
From 7 - Raising and Handling Events.mp4, Code With Mosh - Mastering React
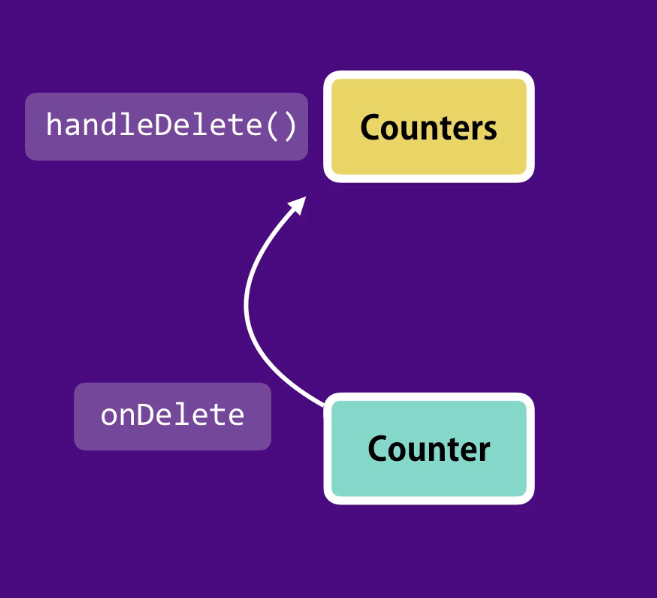
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* Child Component */ | |
import React, { Component } from "react"; | |
class Counter extends Component { | |
render() { | |
const { onDelete } = this.props; | |
return ( | |
<button | |
onClick={() => onDelete(counter.id)} | |
className="btn btn-danger btn-sm m-2" | |
> | |
X | |
</button> | |
); | |
} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/* Parent Component */ | |
import React, { Component } from "react"; | |
import Counter from "./Counter"; | |
class Counters extends Component { | |
state = { | |
counters: [ | |
{ id: 1, value: 0 }, | |
{ id: 2, value: 1 }, | |
{ id: 3, value: 4 }, | |
{ id: 4, value: 0 }, | |
{ id: 5, value: 0 }, | |
], | |
}; | |
handleDelete = (id) => { | |
this.setState({ | |
counters: this.state.counters.filter((el) => el.id !== id), | |
}); | |
}; | |
render() { | |
const { onDelete } = this.props; | |
return <Counter onDelete={this.handleDelete} />; | |
} | |
} | |
export default Counters; |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment