Last active
June 12, 2021 18:23
-
-
Save Munawwar/6ac51e33e901d89750ee61319d064aa5 to your computer and use it in GitHub Desktop.
Create an artistic svg placeholder for an image (node.js)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!-- the blur filter makes it look more like a placeholder than a final image --> | |
<img src="./output.svg" style="width: 1200px; filter: blur(5px)" /> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import Jimp from 'jimp' | |
import { Bitmap, ImageRunner, ShapeTypes, SvgExporter } from 'geometrizejs' | |
import fs from 'fs' | |
const hex = decimalNumberAsString => parseInt(decimalNumberAsString).toString(16).padStart(2, '0'); | |
(async () => { | |
const start = Date.now(); | |
// load png/jpeg/gif,bmp/tiff image from url, file path or Buffer using jimp: | |
// get parrots.png from https://raw.githubusercontent.com/cancerberoSgx/geometrizejs/master/geometrizejs/test/assets/parrots.png | |
const image = await (await Jimp.read('parrots.png')).scaleToFit(100, 100); // resize makes geometrizejs faster | |
console.log('starting..'); | |
const bitmap = Bitmap.createFromByteArray(image.bitmap.width, | |
image.bitmap.height, image.bitmap.data) | |
const runner = new ImageRunner(bitmap) | |
const options = { | |
shapeTypes: [ShapeTypes.TRIANGLE], | |
candidateShapesPerStep: 50, | |
shapeMutationsPerStep: 100, // this has implication on speed and quality | |
alpha: 128 | |
} | |
const iterations = 200 // lower than 200 polygons makes terrible quality. more polygons makes larger svgs | |
const svgData = [] | |
for (let i = 0; i < iterations; i++) { | |
svgData.push(SvgExporter.exportShapes(runner.step(options))) | |
} | |
const svg = SvgExporter.getSvgPrelude() + | |
SvgExporter.getSvgNodeOpen(bitmap.width, bitmap.height).slice(0, -2) + | |
' fill-opacity="0.5">' + | |
svgData | |
.join('') | |
// steps to make the svg output smaller | |
.replace(/ fill-opacity="[^"]*"\/>/g, '/>') | |
.replace(/rgb\((\d+),(\d+),(\d+)\)/g, (m,r,g,b) => `#${hex(r)}${hex(g)}${hex(b)}`) + | |
SvgExporter.getSvgNodeClose() | |
fs.writeFileSync('output.svg', svg) | |
console.log('time take=', Date.now() - start) | |
// takes around 1.5 sec on my machine | |
// output is around 10 KB svg. brotli compressed to ~2.6 KB / gzip ~3.1 KB | |
})() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Input:
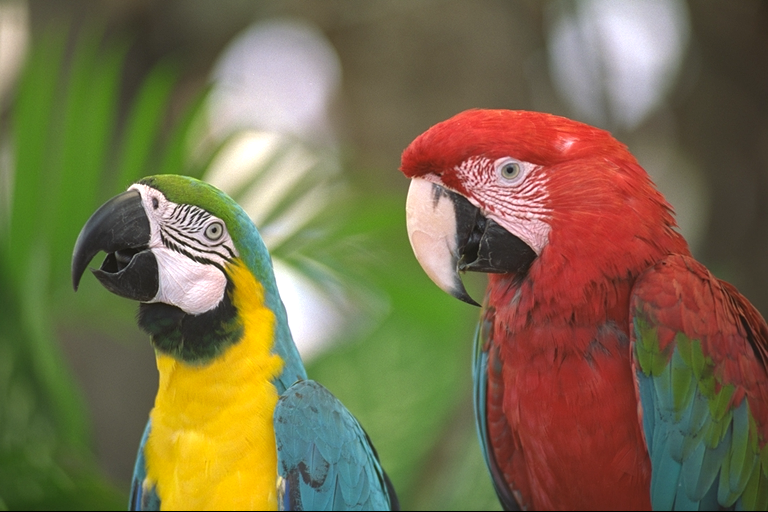
Output (screenshot):
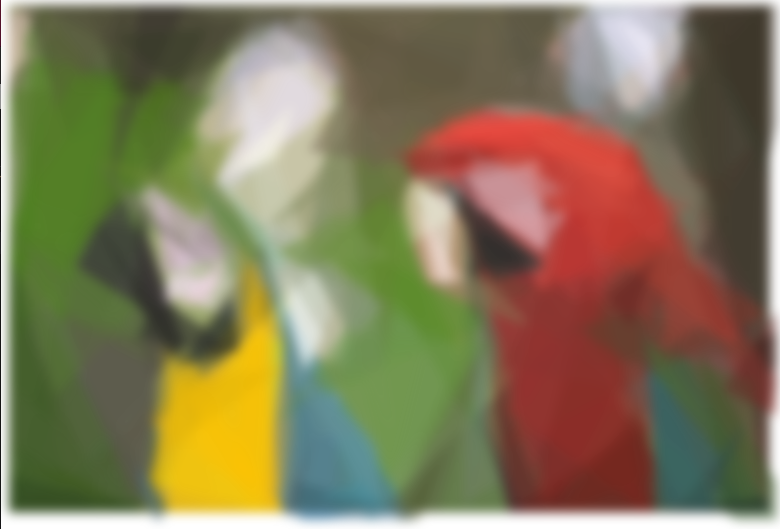
Size is going to be mostly constant as it depends on the number of polygons generated (which is also constant here)