Last active
September 28, 2021 11:52
-
-
Save NKKFu/b5bb706270b9e5a780e1c867c40d7b8a to your computer and use it in GitHub Desktop.
Utilitário para criação de carrosséis na Unity
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Collections; | |
using System.Collections.Generic; | |
using System.Linq; | |
using UnityEngine; | |
using UnityEngine.EventSystems; | |
using UnityEngine.UI; | |
[RequireComponent (typeof (ScrollRect))] | |
public class Carrousel : MonoBehaviour, IBeginDragHandler, IEndDragHandler { | |
[SerializeField] | |
[Range (0.05f, 1f)] | |
private float lerpAmount = 0.1f; | |
private ScrollRect scrollRect { get { return GetComponent<ScrollRect> (); } } | |
private HorizontalLayoutGroup content { get { return scrollRect.content.GetComponent<HorizontalLayoutGroup> (); } } | |
private RectTransform contentRectTransform { get { return scrollRect.content.GetComponent<RectTransform> (); } } | |
private List<RectTransform> contentObjects { get { return content.transform.Cast<RectTransform> ().ToList (); } } | |
private float cardSize { get { return contentObjects[0].rect.width; } } | |
private float cardQuantity { get { return contentObjects.Count; } } | |
public int CurrentCardIndex = 0; | |
private bool isDrag = false; | |
private void OnEnable () { | |
RectTransform viewport = scrollRect.viewport; | |
Debug.Log (viewport.rect.width); | |
int padding = (int) (viewport.rect.width - cardSize) / 2; | |
content.padding.left = content.padding.right = (int) (scrollRect.GetComponent<RectTransform> ().rect.width - cardSize) / 2; | |
} | |
public void OnBeginDrag (PointerEventData e) => isDrag = true; | |
public void OnEndDrag (PointerEventData e) => isDrag = false; | |
public float RoundValue (float current, float multiplier) { | |
float rest = current % multiplier; | |
float minimum = current - rest; | |
float maximum = minimum + multiplier; | |
return rest > multiplier / 2 ? maximum : minimum; | |
} | |
float objetiveValue = -1; | |
public void AddIndexToCarrousel (int index) { | |
CurrentCardIndex = Mathf.Clamp (CurrentCardIndex + index, 0, (int) cardQuantity - 1); | |
objetiveValue = CurrentCardIndex * (1 / (cardQuantity - 1)); | |
} | |
private void Update () { | |
if (!isDrag) { | |
if (objetiveValue != -1) { | |
scrollRect.horizontalScrollbar.value = Mathf.Lerp ( | |
scrollRect.horizontalScrollbar.value, | |
CurrentCardIndex * (1 / (cardQuantity - 1)), | |
0.1f); | |
if (Mathf.Abs (objetiveValue - scrollRect.horizontalScrollbar.value) < 0.01f) | |
objetiveValue = -1; | |
return; | |
} | |
int padding = content.padding.left; | |
float carrouselSize = cardSize * cardQuantity; | |
float cardSizeInScroll = 1f / (cardQuantity - 1); | |
float paddingInScroll = padding / (contentRectTransform.sizeDelta.x); | |
float roundedValue = Mathf.Clamp (RoundValue (scrollRect.horizontalScrollbar.value, cardSizeInScroll), 0f, 1f); | |
scrollRect.horizontalScrollbar.value = Mathf.Lerp (scrollRect.horizontalScrollbar.value, roundedValue, lerpAmount); | |
} | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Use da seguinte maneira:
Código dentro do objeto na seta vermelha
Adicione o componente "Horizontal Layout Group" e "Content Size Fitter", arrange-os desta maneira:
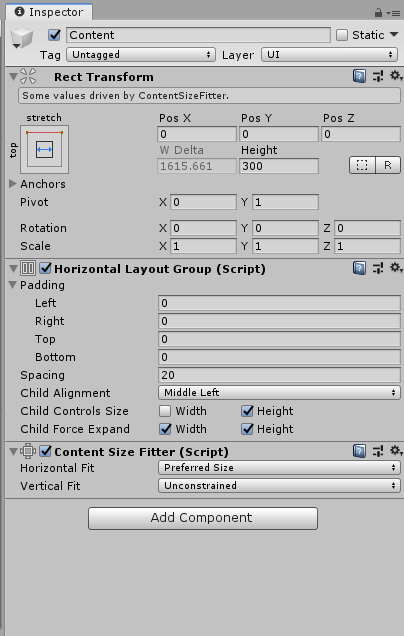
Pronto, tudo feito, aproveite e mexa de acordo com suas preferências.