Last active
January 22, 2021 12:08
-
-
Save Patabugen/4e92de8a404bcc322b5c78402176a186 to your computer and use it in GitHub Desktop.
A little script to clear all your history at https://myactivity.google.com/myactivity
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
/** | |
* A little script to clear all your history at https://myactivity.google.com/myactivity | |
* | |
* Load Developer Console (usually F12), paste this into the Console and hit Enter (or Run). | |
* | |
* Doesn't use any cool magic, just simulates clicking on all the delete buttons. | |
**/ | |
var ClearGoogleMyAccount = | |
{ | |
numTimesRun: 0, | |
numTimesToRun: 3, | |
init: function() | |
{ | |
ClearGoogleMyAccount.loadjQuery(); | |
// Give jQuery time to load, then get started | |
setTimeout(function(){ | |
ClearGoogleMyAccount.queueClickDeleteButton(); | |
}, 1000) | |
}, | |
loadjQuery: function () | |
{ | |
// Load jQuerry, because it was quicker for me to script | |
var script = document.createElement("script"); | |
script.src = "//ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js"; | |
document.head.appendChild(script); | |
}, | |
/** | |
* Clicks on all the Delete buttons which can be found - this causes a confirmation | |
* popup to appear | |
*/ | |
clickDeleteButton: function () { | |
console.log("Clicking Delete Buttons"); | |
jQuery('.swipe-div .fp-date-block-date-holder button').trigger('click'); | |
}, | |
/** | |
* Click on all the confirmation popups which can be found - this does the actual deleting | |
* | |
**/ | |
clickDeleteConfirmationButtons: function () { | |
console.log("Clicking Confirmation Buttons"); | |
jQuery('.fp-delete-confirmation-actions [aria-label=Delete]').trigger('click'); | |
}, | |
/** | |
* So that we can keep deleting until everything is gone, we use a function which | |
* can call itself. | |
**/ | |
queueClickDeleteButton: function () | |
{ | |
// Open up some dialog boxes | |
ClearGoogleMyAccount.clickDeleteButton(); | |
console.log("Queueing clickDeleteConfirmationButtons in 1.5 seconds") | |
setTimeout(function(){ | |
// Let the dialogs open, then click all the Confirmation buttons | |
ClearGoogleMyAccount.clickDeleteConfirmationButtons() | |
}, 2000) | |
// See how many items we have left to delete | |
var itemsRemaining = jQuery('.swipe-div .fp-date-block-date-holder button').length | |
console.log(itemsRemaining + " Items Remaining"); | |
setTimeout(function(){ | |
if (itemsRemaining > 0) { | |
// If we have some items left, start deleting them | |
ClearGoogleMyAccount.queueClickDeleteButton(); | |
} else { | |
// To be sure we get everything, we run the script a few times. This accounts for Ajax loading/slow pages. | |
ClearGoogleMyAccount.numTimesRun++; | |
if (ClearGoogleMyAccount.numTimesRun < ClearGoogleMyAccount.numTimesToRun) { | |
console.log("Running Clear My Google Account count: " + ClearGoogleMyAccount.numTimesRun); | |
ClearGoogleMyAccount.queueClickDeleteButton(); | |
} else { | |
console.log("Done!"); | |
} | |
} | |
}, 5000) | |
} | |
} | |
ClearGoogleMyAccount.init(); |
Hey Chupro,
Thanks for reporting the error - I've not used Google for a couple of years so I'm afraid I've not maintained this script and it's likely they've changed their UI since then.
I won't be updating this scrpit any more, but if you're able to you're welcome to fork it and fix the bugs.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Don`t work ((**
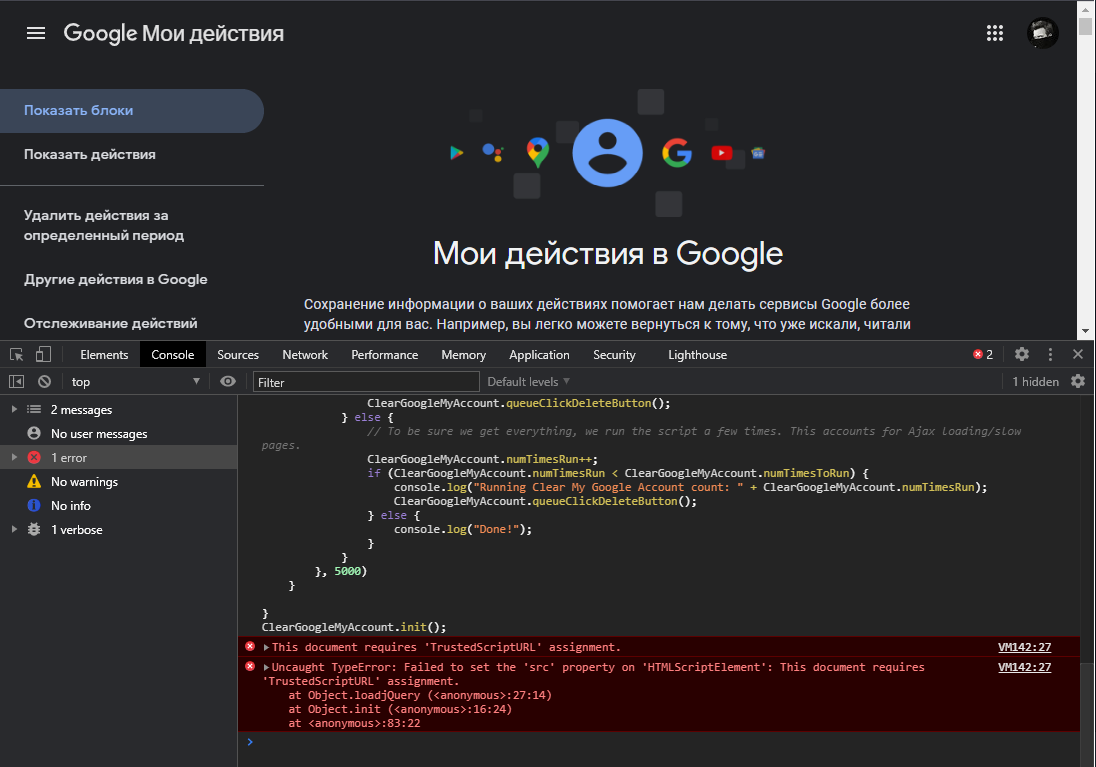