Last active
May 16, 2024 01:58
-
-
Save SahbiOuali13/c6faa6e03c79148b76ed3f8c16c17682 to your computer and use it in GitHub Desktop.
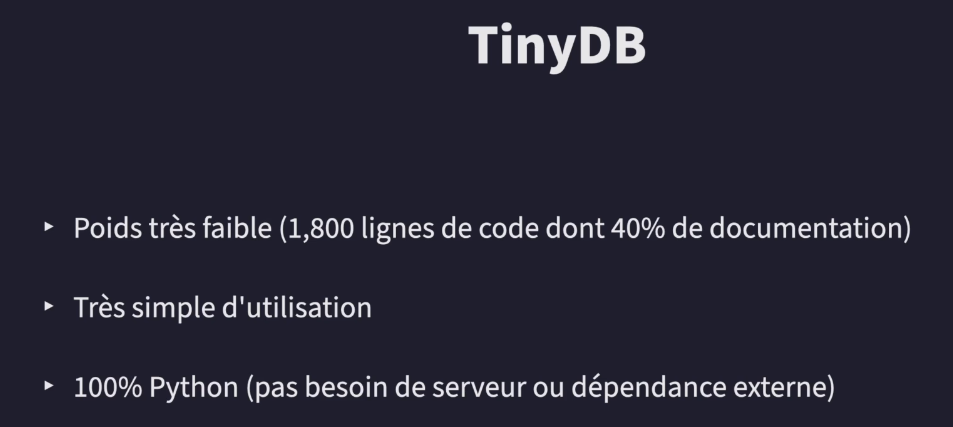
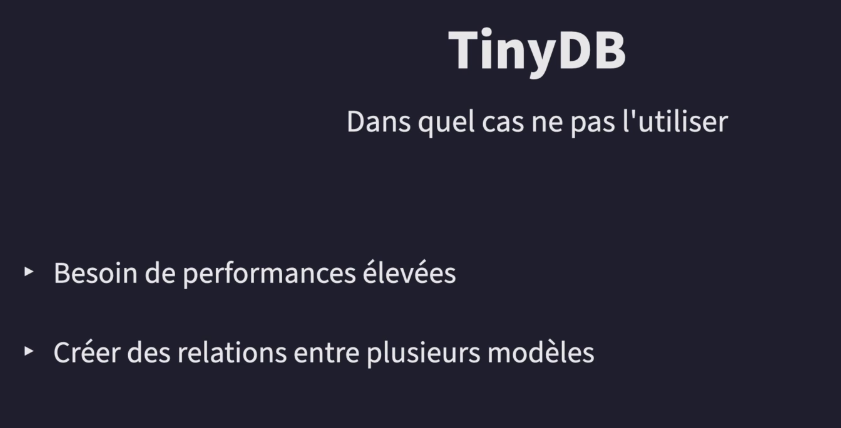 # How to use
- pip install tinydb
# CRUD
- Cr
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from tinydb import TinyDB | |
# Disk storage | |
db = TinyDB('data.json') | |
# Memory storage | |
from tinydb.storages import MemoryStorage, Storage | |
db = TinyDB(storage=MemoryStorage) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from tinydb import TinyDB | |
db = TinyDB('data.json', indent=4) | |
# single insertion | |
db.insert({'name': 'Patrick', | |
'score': 0}) | |
# multiple insertion | |
db.insert_multiple([{'name': 'Paul', 'score': 120}, | |
{'name': 'Julie', 'score': 50}]) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from tinydb import TinyDB, Query, where | |
db = TinyDB("data.json", indent=4) | |
users = db.table("Users") | |
roles = db.table("Roles") | |
users.insert({'name': 'Patrick', "salary": 25000}) | |
users.insert({'name': 'Paul', "salary": 35000}) | |
users.insert({'name': 'Julie', "salary": 45000}) | |
roles.insert_multiple([{'name': 'Pythonista'}, | |
{'name': 'Javaista'}, | |
{'name': 'Javascripta'}]) | |
# Main issue is that there is no possibilities to link the two tables. "Pas de jointure possible !" |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from tinydb import TinyDB, Query, where | |
db = TinyDB("data.json", indent=4) | |
User = Query() | |
patrick = db.search(User.name == 'Paul') | |
print(f"{patrick}") | |
# another way | |
patrick_unique = db.get(User.name == 'Patrick') | |
print(patrick_unique) | |
patrick_where = db.get(where('name') == 'Patrick') | |
print(f"{patrick_where}") | |
# searching for users have a score higher than 0 | |
higher_than_zero = db.search(where('score') > 0) | |
print(higher_than_zero) | |
# number of entries | |
print(len(db)) | |
# Verify if the user Patrick is in the db | |
print(db.contains(User.name == 'Patrick')) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
from tinydb import TinyDB, Query, where | |
db = TinyDB("data.json", indent=4) | |
# change entries | |
db.update({'score': 10}, where('name') == "Paul") | |
# add columns | |
db.update({'roles': ['Junior']}) # default column for all entries | |
db.update({'roles': ['Pythonisa']}, where('name') == 'Julie') | |
# it updates but can insert at the same time UPdate + inSERT | |
db.upsert({'name': 'Samir', 'score': 58, 'roles': ["BG"]}, where('name') == 'Pierre') | |
# remove ebtries | |
db.remove(where('score') == 58) | |
# clear all db | |
db.truncate() |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment