Last active
June 14, 2022 17:51
-
-
Save SergProduction/c826a3f740117442c48cf8290822536b to your computer and use it in GitHub Desktop.
date react hook convertation string to Date object
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import React, { useState } from 'react' | |
const Nothing = Symbol() | |
const normDoubleSymbol = (strOrNumber: string | number) => strOrNumber.toString().length === 1 | |
? `0${strOrNumber}` | |
: strOrNumber.toString() | |
export const parseDateToString = (dateMiliseconds: number) => { | |
const d = new Date(dateMiliseconds) | |
const dateStr = `${normDoubleSymbol(d.getDate())}/${normDoubleSymbol(d.getMonth() + 1)}/${d.getFullYear()}` | |
const timeStr = `${normDoubleSymbol(d.getHours())}:${normDoubleSymbol(d.getMinutes())}` | |
return { dateStr, timeStr } | |
} | |
const validDate = (str: string): typeof Nothing | number[] => { | |
const res = str | |
.split(/ |\//) | |
.map(d => parseInt(d, 10)) | |
const isEveryValueNumber = res.every(d => Number.isNaN(d) === false) | |
if ( | |
res.length !== 3 | |
|| isEveryValueNumber === false | |
|| res[0] < 0 | |
|| res[0] > 31 | |
|| res[1] < 0 | |
|| res[1] > 12 | |
|| res[2] < 1970 | |
) { | |
return Nothing | |
} | |
const [d, m, y] = res | |
return [y, m - 1, d] | |
} | |
const validTime = (str: string): typeof Nothing | number[] => { | |
const res = str | |
.split(/ |:/) | |
.map(d => parseInt(d, 10)) | |
const isEveryValueNumber = res.every(d => Number.isNaN(d) === false) | |
if ( | |
res.length !== 2 | |
|| isEveryValueNumber === false | |
|| res[0] < 0 | |
|| res[0] > 59 | |
|| res[1] < 0 | |
|| res[1] > 59 | |
) { | |
return Nothing | |
} | |
return res | |
} | |
type DateTypes | |
= 'fromDate' | |
| 'fromTime' | |
| 'toDate' | |
| 'toTime' | |
type DateErrors = { [key in DateTypes]: boolean } | |
type Props = { | |
initFormsDate: { [key in DateTypes]: string }, | |
} | |
const defaultProps: Props = { | |
initFormsDate: { | |
fromDate: '', | |
fromTime: '', | |
toDate: '', | |
toTime: '' | |
} | |
} | |
export const useDate = ({ | |
initFormsDate = defaultProps.initFormsDate | |
}: Props) => { | |
const [values, setValues] = useState(initFormsDate) | |
const [errors, setErrors] = useState({ | |
fromDate: false, | |
fromTime: false, | |
toDate: false, | |
toTime: false | |
}) | |
const onChange = (type: DateTypes) => (e: React.SyntheticEvent<HTMLInputElement>) => { | |
const value = e.currentTarget.value | |
setValues({ | |
...values, | |
[type]: value, | |
}) | |
setErrors({ | |
...errors, | |
[type]: false | |
}) | |
} | |
const getDate = (): void | { from: Date, to: Date } => { | |
const fromDate = validDate(values.fromDate) | |
const fromTime = validTime(values.fromTime) | |
const toDate = validDate(values.toDate) | |
const toTime = validTime(values.toTime) | |
if ([fromDate, fromTime, toDate, toTime].some(f => f === Nothing)) { | |
const timeParts = { fromDate, fromTime, toDate, toTime } | |
const errorsTimeParts = Object.entries(timeParts).reduce((acc, [key, value]) => ({ | |
...acc, | |
[key]: value === Nothing | |
}), {} as DateErrors) | |
setErrors(errorsTimeParts) | |
return | |
} | |
const from = new Date(...fromDate, ...fromTime) | |
const to = new Date(...toDate, ...toTime) | |
return { from, to } | |
} | |
const clearDate = () => { | |
setValues({ | |
fromDate: '', | |
fromTime: '', | |
toDate: '', | |
toTime: '' | |
}) | |
setErrors({ | |
fromDate: false, | |
fromTime: false, | |
toDate: false, | |
toTime: false | |
}) | |
} | |
return { | |
values, | |
errors, | |
onChange, | |
clearDate, | |
getDate | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
using example

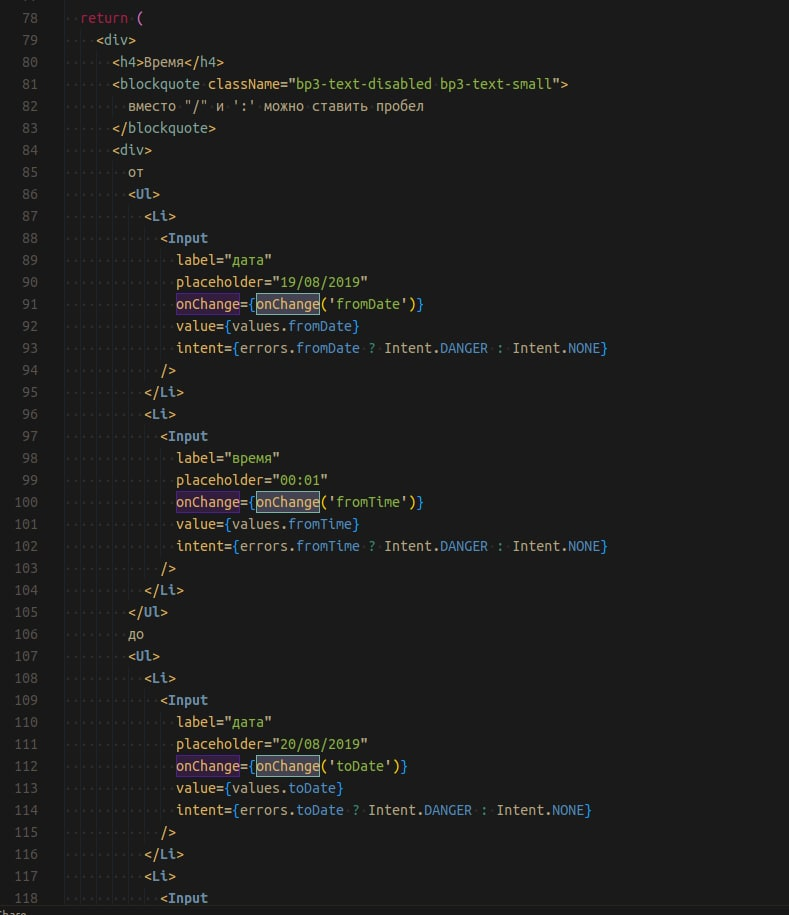