-
-
Save ShawnHymel/327f9fc4cc57594e4f482b868be02e93 to your computer and use it in GitHub Desktop.
/** | |
* Mozilla WebThings BME280 example | |
* Date: August 25, 2019 | |
* Author: Shawn Hymel | |
* | |
* Notes: | |
* - Install ArduinoJSON latest v5 (not v6!) | |
* - Install ESPAsyncTCP | |
*/ | |
#include <Adafruit_Sensor.h> | |
#include <Adafruit_BME280.h> | |
#include <Thing.h> | |
#include <WebThingAdapter.h> | |
const char* ssid = "<YOUR SSID HERE>"; | |
const char* password = "<YOUR WIFI PASSWORD>"; | |
// Hostname used by mDNS | |
const String mDNSHostname = "weathersensor"; | |
// BME280 sensor (I2C) | |
Adafruit_BME280 bme280; | |
// Handle to connection between Thing and Gateway | |
WebThingAdapter* adapter; | |
// @type members: Capabilities supported by your Thing | |
// See schemas: https://iot.mozilla.org/schemas#capabilities | |
const char* sensorTypes[] = {"TemperatureSensor", nullptr}; | |
// Description of your Thing | |
// ThingDevice device(id, title, types) | |
// id: unique identifier for Thing (part of URL: http://<IP>/things/<id>) | |
// description: string that shows up in Gateway for your Thing | |
// types: array of @types | |
ThingDevice sensor("bme280", "BME280 Weather Sensor", sensorTypes); | |
// Define one or more properties supported by your Thing | |
// ThingProperty property(id, description, type, atType) | |
// id: unique identifier for property | |
// description: user-readable description of property | |
// type: NO_STATE, BOOLEAN, NUMBER, or STRING | |
// atType: property @type (https://iot.mozilla.org/schemas#properties) | |
ThingProperty sensorTemp("temperature", "", NUMBER, "TemperatureProperty"); | |
ThingProperty sensorHumd("humidity", "", NUMBER, nullptr); | |
ThingProperty sensorPres("pressure", "", NUMBER, nullptr); | |
void setup() { | |
// Debug info | |
Serial.begin(115200); | |
Serial.print("BME280 Weather Sensor"); | |
// Connect to BME280 sensor | |
if ( !bme280.begin() ) { | |
Serial.println("Could not initialize BME280 sensor"); | |
while(1); | |
} | |
// Connect to WiFi access point | |
Serial.print("Connecting to "); | |
Serial.println(ssid); | |
WiFi.mode(WIFI_STA); | |
WiFi.begin(ssid, password); | |
while ( WiFi.status() != WL_CONNECTED ) { | |
delay(500); | |
Serial.print("."); | |
} | |
Serial.println(); | |
// Show connection details | |
Serial.println("Connected!"); | |
Serial.print("IP address: "); | |
Serial.println(WiFi.localIP()); | |
// Create new WebThings connection handle (default port: 80) | |
adapter = new WebThingAdapter(mDNSHostname, WiFi.localIP()); | |
// Set units for properties | |
sensorTemp.unit = "celsius"; | |
sensorHumd.unit = "%"; | |
sensorPres.unit = "hPa"; | |
// Associate properties with device | |
sensor.addProperty(&sensorTemp); | |
sensor.addProperty(&sensorHumd); | |
sensor.addProperty(&sensorPres); | |
// Associate device with connection | |
adapter->addDevice(&sensor); | |
// Start mDNS and HTTP server | |
adapter->begin(); | |
Serial.println("HTTP server started"); | |
Serial.print("http://"); | |
Serial.print(WiFi.localIP()); | |
Serial.print("/things/"); | |
Serial.print(sensor.id); | |
} | |
void loop() { | |
ThingPropertyValue tpVal; | |
float temp, humd, pres; | |
// Read sensor values | |
temp = bme280.readTemperature(); | |
humd = bme280.readHumidity(); | |
pres = bme280.readPressure() / 100.0F; | |
// Print readings to console | |
#if 0 | |
Serial.print("Temperature: "); | |
Serial.print(temp); | |
Serial.println(" C"); | |
Serial.print("Humidity: "); | |
Serial.print(humd); | |
Serial.println("%"); | |
Serial.print("Pressure: "); | |
Serial.print(pres); | |
Serial.println(" hPa"); | |
Serial.println(); | |
#endif | |
// Update device values | |
tpVal.number = temp; | |
sensorTemp.setValue(tpVal); | |
// UI bug prevents more than one property from being updated | |
/*tpVal.number = humd; | |
sensorHumd.setValue(tpVal); | |
tpVal.number = pres; | |
sensorPres.setValue(tpVal);*/ | |
// Update all properties and events over the connection | |
adapter->update(); | |
// Do nothing for a bit | |
delay(1000); | |
} |
Managed to make it work..
Here is the code.
#include <Adafruit_Sensor.h>
#include <Adafruit_BMP280.h>
#include <Thing.h>
#include <WebThingAdapter.h>
const char* ssid = "Dialup-Net";
const char* password = "";
// Hostname used by mDNS
const String mDNSHostname = "weathersensor";
// BME280 sensor (I2C)
Adafruit_BMP280 bmp280;
// Handle to connection between Thing and Gateway
WebThingAdapter* adapter;
// @type members: Capabilities supported by your Thing
// See schemas: https://iot.mozilla.org/schemas#capabilities
const char* sensorTypes[] = {"TemperatureSensor", nullptr};
ThingDevice sensor("bmp280", "BMP280 Weather Sensor", sensorTypes);
ThingProperty sensorTemp("temperature", "", NUMBER, "TemperatureProperty");
ThingProperty sensorHumd("humidity", "", NUMBER, nullptr);
ThingProperty sensorPres("pressure", "", NUMBER, nullptr);
void setup() {
// Debug info
Serial.begin(115200);
Serial.print("BMP280 Weather Sensor");
// Connect to BME280 sensor
if ( !bmp280.begin(0x76) ) {
Serial.println("Could not initialize BME280 sensor");
while(1);
}
// Connect to WiFi access point
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
while ( WiFi.status() != WL_CONNECTED ) {
delay(500);
Serial.print(".");
}
Serial.println();
// Show connection details
Serial.println("Connected!");
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
// Create new WebThings connection handle (default port: 80)
adapter = new WebThingAdapter(mDNSHostname, WiFi.localIP());
// Set units for properties
sensorTemp.unit = "celsius";
sensorHumd.unit = "%";
sensorPres.unit = "hPa";
// Associate properties with device
sensor.addProperty(&sensorTemp);
sensor.addProperty(&sensorHumd);
sensor.addProperty(&sensorPres);
// Associate device with connection
adapter->addDevice(&sensor);
// Start mDNS and HTTP server
adapter->begin();
Serial.println("HTTP server started");
Serial.print("http://");
Serial.print(WiFi.localIP());
Serial.print("/things/");
Serial.print(sensor.id);
}
void loop() {
Serial.print(F("Temperature = "));
Serial.print(bmp280.readTemperature());
Serial.println(" *C");
Serial.print(F("Pressure = "));
Serial.print(bmp280.readPressure()/100); //displaying the Pressure in hPa, you can change the unit
Serial.println(" hPa");
Serial.print(F("Approx altitude = "));
Serial.print(bmp280.readAltitude(1019.66)); //The "1019.66" is the pressure(hPa) at sea level in day in your region
Serial.println(" m"); //If you don't know it, modify it until you get your current altitude
Serial.println();
delay(2000);
ThingPropertyValue tpVal;
float temp, humd, pres;
// Read sensor values
temp = bmp280.readTemperature();
pres = bmp280.readPressure() / 100.0F;
// Print readings to console
#if 0
Serial.print("Temperature: ");
Serial.print(temp);
Serial.println(" C");
Serial.print("Pressure: ");
Serial.print(pres);
Serial.println(" hPa");
Serial.println();
#endif
// Update device values
tpVal.number = temp;
sensorTemp.setValue(tpVal);
// UI bug prevents more than one property from being updated
/tpVal.number = humd;
sensorHumd.setValue(tpVal);
tpVal.number = pres;
sensorPres.setValue(tpVal);/
// Update all properties and events over the connection
adapter->update();
// Do nothing for a bit
delay(1000);
}
Awesome! Thanks for sharing the code!
Awesome! Thanks for sharing the code!
I`m not programmer :) I just testet unitl i made it work,maybe with some more exp will manage to check and fix if there are some pb with this type of sensor! Best regards and good work you made for us:)
Is there any chance that you can make it to work with BMP280 ?
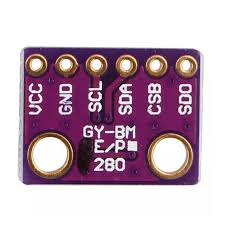
I fount a code that works verry well.
14:02:56.496 -> Temperature = 21.20 *C
14:02:56.496 -> Pressure = 1020.87 hPa
14:02:56.529 -> Approx altitude = 91.37 m
14:02:56.562 ->
the code:
/* This code is to use with Adafruit BMP280 (Metric)
*/
#include <Adafruit_BMP280.h>
Adafruit_BMP280 bmp; // I2C Interface
void setup() {
Serial.begin(9600);
Serial.println(F("BMP280 test"));
if (!bmp.begin()) {
Serial.println(F("Could not find a valid BMP280 sensor, check wiring!"));
while (1);
}
/* Default settings from datasheet. /
bmp.setSampling(Adafruit_BMP280::MODE_NORMAL, / Operating Mode. /
Adafruit_BMP280::SAMPLING_X2, / Temp. oversampling /
Adafruit_BMP280::SAMPLING_X16, / Pressure oversampling /
Adafruit_BMP280::FILTER_X16, / Filtering. /
Adafruit_BMP280::STANDBY_MS_500); / Standby time. */
}
void loop() {
Serial.print(F("Temperature = "));
Serial.print(bmp.readTemperature());
Serial.println(" *C");
}
But i tried to integrate your code for Webthings in this code but i dont get any data.