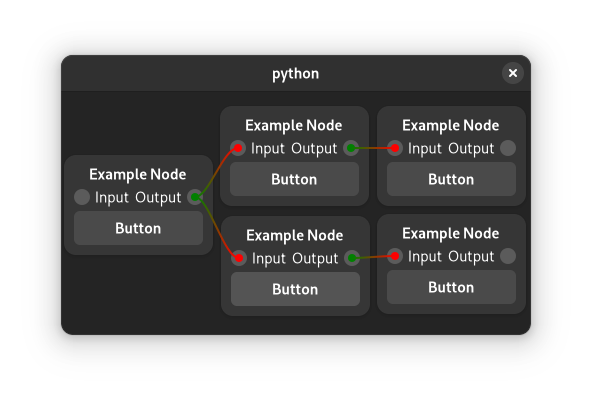
import gi
gi.require_version('Gtk', '4.0')
gi.require_version('Flow', '1.0')
from gi.repository import Gtk
from gi.repository import Gdk
from gi.repository import Flow
def on_activate(app):
win = Gtk.ApplicationWindow(application=app)
nodeview = Flow.NodeView.new()
menu_content = Gtk.Box.new(Gtk.Orientation.VERTICAL, 0)
btn = Gtk.Button(label="Add node")
btn.connect('clicked', lambda _: add_node(nodeview))
menu_content.append(btn)
nodeview.set_menu_content(menu_content)
win.set_child(nodeview)
win.present()
def add_node(nodeview):
node = Flow.Node.new()
node.set_label_name("Example Node", True)
sink = Flow.Sink.with_type(float)
sink.set_name("Input")
sink_color = Gdk.RGBA()
sink_color.parse("red")
sink.set_color(sink_color)
source = Flow.Source.with_type(float)
source.set_name("Output")
source_color = Gdk.RGBA()
source_color.parse("green")
source.set_color(source_color)
node.add_sink(sink)
node.add_source(source)
node.set_content(Gtk.Button(label="Button"))
nodeview.add(node)
app = Gtk.Application(application_id='com.example.GtkApplication')
app.connect('activate', on_activate)
app.run(None)