Last active
January 16, 2023 08:08
-
-
Save SureshKumar311/8ab9f4f310861a95c1777e282075521b to your computer and use it in GitHub Desktop.
Flutter Web URL Encryption
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
//This method uses encrypt:^5.0.1 package to encrypt the url | |
//Key and IV should be same for both encryption and decryption | |
//https://pub.dev/packages/encrypt | |
import 'package:encrypt/encrypt.dart' as encrypt; | |
import 'package:flutter/material.dart'; | |
String enCryptURL(String url, {bool isrestoreRoute = false}) { | |
//final Uri uri = Uri.parse(enCryptURL(routeInformation.location ?? "")); | |
//return RouteInformation(location: enCryptURL(location, isrestoreRoute: true)); | |
RegExp base64 = RegExp( | |
r'^(?:[A-Za-z0-9+\/]{4})*(?:[A-Za-z0-9+\/]{2}==|[A-Za-z0-9+\/]{3}=|[A-Za-z0-9+\/]{4})$'); | |
final key = encrypt.Key.fromUtf8('FntDBmHdSpgtVBsLLtcNTQwu'); | |
final iv = encrypt.IV.fromLength(16); | |
final encrypter = | |
encrypt.Encrypter(encrypt.AES(key, mode: encrypt.AESMode.ctr, padding: null)); | |
String screen = url; | |
try { | |
if (isrestoreRoute) { | |
screen = encrypter.encrypt(url, iv: iv).base64; | |
} else { | |
if (base64.hasMatch(url.replaceFirst("/", ""))) { | |
screen = | |
encrypter.decrypt(encrypt.Encrypted.from64(url.replaceFirst("/", "")), iv: iv); | |
} else { | |
screen = url; | |
} | |
} | |
} catch (e) { | |
debugPrintStack(label: "Error on enCryptURL :-> ${e.toString()}"); | |
screen = url; | |
} | |
debugPrintStack( | |
label: "enCrypt url >>>key${key.base64}\n iv=>>${iv.base64} \n Screen==>> $screen"); | |
return screen; | |
} | |
String enCryptURLManual(String url, {bool isrestoreRoute = false}) { | |
// final Uri uri = Uri.parse(enCryptURL(routeInformation.location ?? "")); | |
// RouteInformation(location: enCryptURL(location, isrestoreRoute: true)); | |
Map<String, String> screenKeys = { | |
"portfolioSummary": "CryptportfolioSummary", | |
"dashboard": "dashboardCrypt" | |
}; | |
final encryptedscreens = []; | |
try { | |
final screens = url.split("/"); | |
for (var element in screens) { | |
if (isrestoreRoute) { | |
screenKeys.containsKey(element) | |
? encryptedscreens.add(screenKeys[element]) | |
: encryptedscreens.add(element); | |
} else { | |
screenKeys.containsValue(element) | |
? encryptedscreens | |
.add((screenKeys.keys.firstWhere((key) => screenKeys[key] == element))) | |
: encryptedscreens.add(element); | |
} | |
} | |
return Uri.tryParse(encryptedscreens.join("/")).toString(); | |
} catch (e) { | |
debugPrintStack(label: "Error on enCryptURL :-> ${e.toString()}"); | |
} | |
return encryptedscreens.join("/"); | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Example
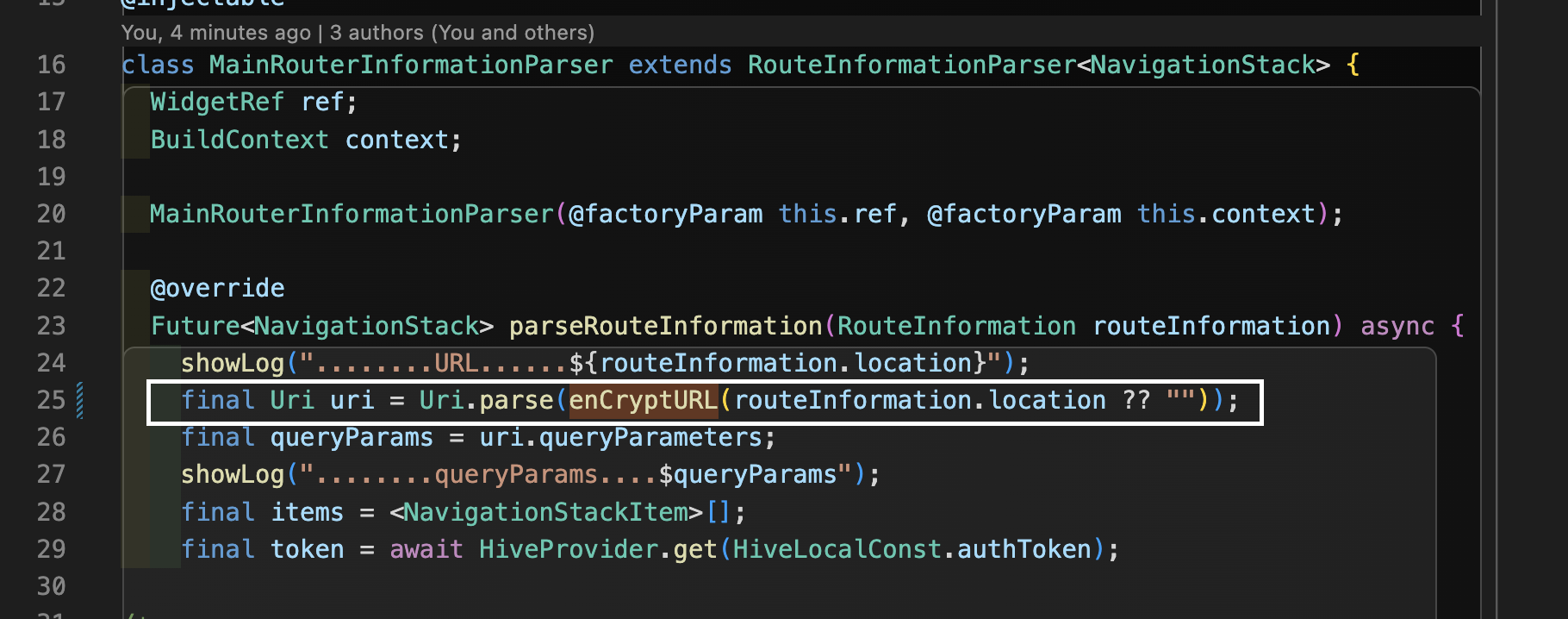