-
-
Save TheMuellenator/83294ef2c2f606f146e51f4c4e547cab to your computer and use it in GitHub Desktop.
//Don't change this code: | |
func calculator() { | |
let a = Int(readLine()!)! //First input | |
let b = Int(readLine()!)! //Second input | |
add(n1: a, n2: b) | |
subtract(n1: a, n2: b) | |
multiply(n1: a, n2: b) | |
divide(n1: a, n2: b) | |
} | |
//Write your code below this line to make the above function calls work. | |
func add(n1: Int, n2: Int) { | |
print(n1 + n2) | |
} | |
func subtract(n1: Int, n2: Int) { | |
print(n1 - n2) | |
} | |
func multiply(n1: Int, n2: Int) { | |
print(n1 * n2) | |
} | |
func divide(n1: Int, n2: Int) { | |
let decimalN1 = Double(n1) | |
let decimalN2 = Double(n2) | |
print(decimalN1 / decimalN2) | |
} | |
//Don't move or change this code: | |
calculator() |
//Don't change this code:
func calculator() {
let a = Int(readLine()!)! //First input
let b = Int(readLine()!)! //Second input
add(n1: a, n2: b)
subtract(n1: a, n2: b)
multiply(n1: a, n2: b)
divide(n1: a, n2: b)
}
//Write your code below this line to make the above function calls work.
func add(n1: Int, n2: Int) {
print(n1 + n2)
}
func subtract(n1: Int, n2: Int) {
print(n1 - n2)
}
func multiply(n1: Int, n2: Int) {
print(n1*n2)
}
func divide(n1: Int, n2: Int) {
if n2 != 0 {
print( Double(n1) / Double(n2))
}
}I'm not understanding how this works. There aren't even any numbers in here. I feel like we are being asked to do things on these challenges that we are not taught. Is there a video of someone explaining this?
Hi! I also don't understand if you found a video that explains this, could you please share? Thank you in advance!
hey guys I've been running this on a playground but how the hello do you run the function ????
I've tried this :
calculator(n1: 2, n2: 3)
but I DONT GET ANYTHING
//Don't change this code:
func calculator (){
let n1 = 3
let n2 = 4
print(n1+n2)
print(n1-n2)
print(n1*n2)
let decimalN1 = Double(n1)
let decimalN2 = Double(n2)
print(decimalN1/decimalN2)
}
//Don't move or change this code:
calculator()
`let a = 3 //First input
let b = 4 //Second input
func add(n1: Int, n2: Int) {
print(n1 + n2)
}
func subtract(n1: Int, n2: Int) {
print(n1 - n2)
}
func multiply(n1: Int, n2: Int) {
print(n1 * n2)
}
func divide(n1: Int, n2: Int) {
print(Double(n1) / Double(n2))
}
`
ok this is a rather confusing challenge, not because it's hard, but because it kinda does not fit to the place where you are in the course.
first off, the solution DOES NOT work in the playground (i don't know why). so you kinda stuck with repl.it.
then, this challenge uses USER INPUT, which wasn't explained in the course before. that is why many people are confused with the absence of any numbers inside of the code.
so here:
let a = Int(readLine()!)! //First input
let b = Int(readLine()!)! //Second input
the function reads the input, which you suppose to provide in console after running the function WITHOUT any arguments, as there are no parameters required.
this part
add(n1: a, n2: b)
subtract(n1: a, n2: b)
multiply(n1: a, n2: b)
divide(n1: a, n2: b)
calls function, which you declare AFTER the function. that does not matter, as you call the function also AFTER you add missing functions.
the functions, which you need to write are as follows:
func add(n1: Int, n2: Int) {
print(n1 + n2)
}
func subtract(n1: Int, n2: Int) {
print(n1 - n2)
}
func multiply(n1: Int, n2: Int) {
print(n1 * n2)
}
func divide(n1: Int, n2: Int) {
let decimalN1 = Double(n1)
let decimalN2 = Double(n2)
print(decimalN1 / decimalN2)
}
you need to convert to double, because otherwise only the first digit will be counted as answer (0.75 -> 0). it is not rounding up, that is why you don't see 1 as you might expect.
and to launch this all you need to add
calculator()
in the end.
//Don't change this code:
func calculator() {
let a = 3 //First input
let b = 4 //Second input
add(n1: a, n2: b)
subtract(n1: a, n2: b)
multiply(n1: a, n2: b)
divide(n1: a, n2: b)
}
//Write your code below this line to make the above function calls work.
func add(n1: Int, n2: Int) {
print(n1 + n2)
}
func subtract(n1: Int, n2: Int) {
print(n1 - n2)
}
func multiply(n1: Int, n2: Int) {
print(n1 * n2)
}
func divide(n1: Int, n2: Int) {
print(Double(n1) / Double(n2))
}
calculator()
//Don't change this code:
func calculator() {
let a = Int(readLine()!)! //First input
let b = Int(readLine()!)! //Second input
add(n1: a, n2: b)
subtract(n1: a, n2: b)
multiply(n1: a, n2: b)
divide(n1: a, n2: b)
}
//Write your code below this line to make the above function calls work.
func sum(n1: Int, n2: Int) {
print(n1 + n2)
}
func subtract(n1: Int, n2: Int) {
print(n1 - n2)
}
func multiply(n1: Int, n2: Int) {
print(n1 * n2)
}
func divide(n1: Int, n2: Int) {
let decN1 = Double(n1)
let decN2 = Double(n2)
print(decN1 / decN2)
}
ok this is a rather confusing challenge, not because it's hard, but because it kinda does not fit to the place where you are in the course.
first off, the solution DOES NOT work in the playground (i don't know why). so you kinda stuck with repl.it.
then, this challenge uses USER INPUT, which wasn't explained in the course before. that is why many people are confused with the absence of any numbers inside of the code.
so here:
let a = Int(readLine()!)! //First input let b = Int(readLine()!)! //Second input
the function reads the input, which you suppose to provide in console after running the function WITHOUT any arguments, as there are no parameters required.
this part
add(n1: a, n2: b) subtract(n1: a, n2: b) multiply(n1: a, n2: b) divide(n1: a, n2: b)
calls function, which you declare AFTER the function. that does not matter, as you call the function also AFTER you add missing functions.
the functions, which you need to write are as follows:
func add(n1: Int, n2: Int) { print(n1 + n2) } func subtract(n1: Int, n2: Int) { print(n1 - n2) } func multiply(n1: Int, n2: Int) { print(n1 * n2) } func divide(n1: Int, n2: Int) { let decimalN1 = Double(n1) let decimalN2 = Double(n2) print(decimalN1 / decimalN2) }
you need to convert to double, because otherwise only the first digit will be counted as answer (0.75 -> 0). it is not rounding up, that is why you don't see 1 as you might expect.
and to launch this all you need to add
calculator()
in the end.
still don't get it. Could you explain in more detail? Appreciated!
func addation() {
print("(a + b)")
}
var a = 3
var b = 4
addation()
func subtraction() {
print("(a - b)")
}
var a = 3
var b = 4
subtraction()
func multiply()
{
var a = 3
var b = 4
print("(a * b)")
}
multiply()
func add(n1: Int, n2: Int) {
print(n1 + n2)
}
func subtract(n1: Int, n2: Int) {
print(n1 - n2)
func multiply(n1: Int, n2: Int) {
print(n1 * n2)
}
func divide(n1: Int, n2: Int) {
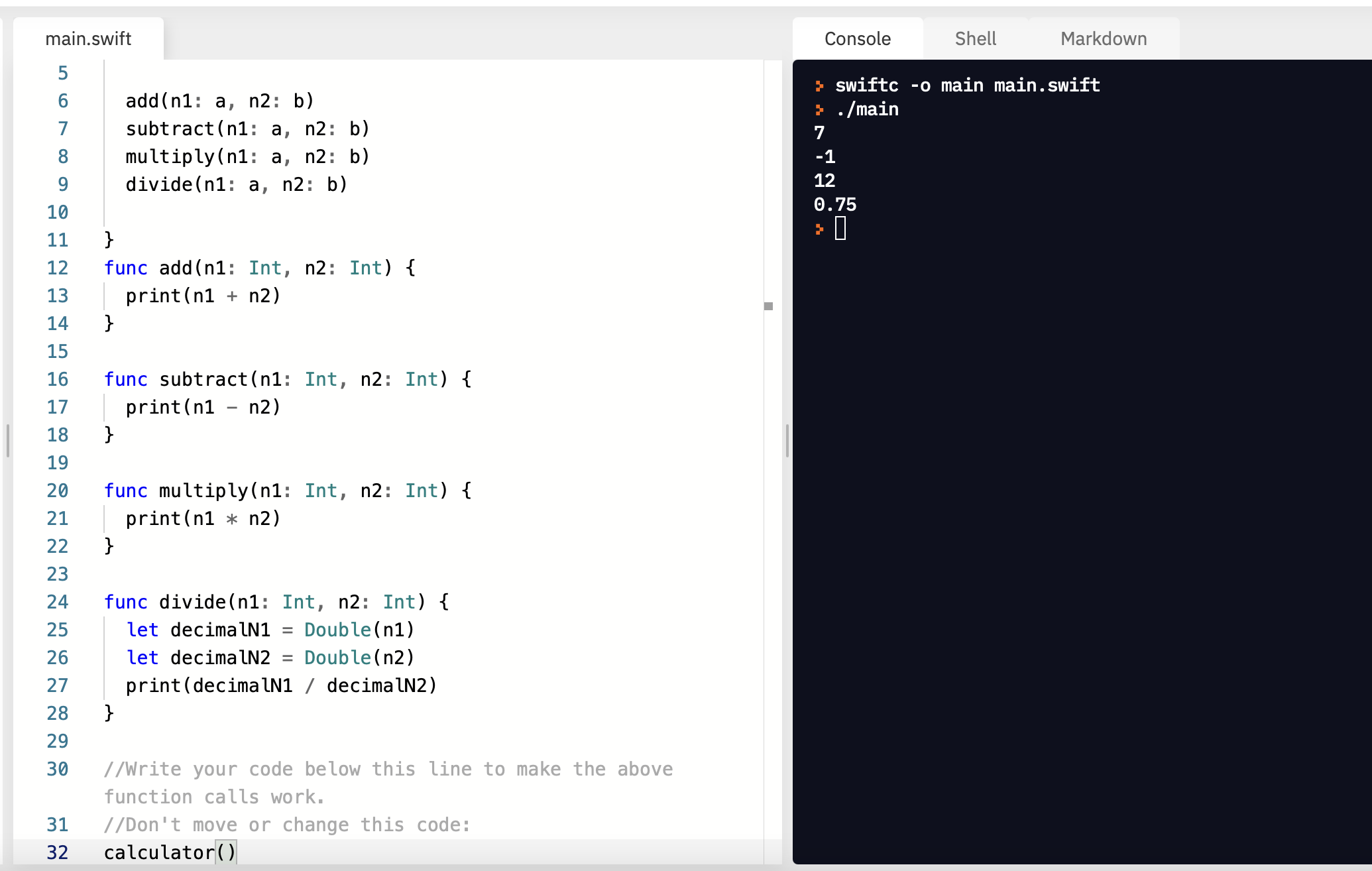
let decimalN1 = Double(n1)
let decimalN2 = Double(n2)
print(decimalN1 / decimalN2)
}
//Don't move or change this code:
calculator()