Created
October 30, 2022 00:06
-
-
Save Thespikedballofdoom/122c7cd62200042f9e662662f31b9544 to your computer and use it in GitHub Desktop.
Spikes edit of yt patch collection
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// ==UserScript== | |
// @name YouTube Patch Collection | |
// @version 2.0.0 | |
// | |
// @description Allows for changing of yt.config_ values -- visit my members at yt.config_.EXPERIMENT_FLAGS in the console | |
// | |
// @author Aubrey Pankow (aubyomori@gmail.com) | |
// @author Taniko Yamamoto (kirasicecreamm@gmail.com) | |
// @author Thespikedballofdoom (biden@whitehouse.gov) | |
// @downloadURL https://raw.githubusercontent.com/aubymori/YouTubePatchCollection/main/YouTubePatchCollection.user.js | |
// @license Unlicense | |
// @match *://*.youtube.com/* | |
// @icon https://www.youtube.com/favicon.ico | |
// @run-at document-start | |
// @grant none | |
// ==/UserScript== | |
// @updateURL https://raw.githubusercontent.com/aubymori/YouTubePatchCollection/main/YouTubePatchCollection.user.js | |
// Attributes to remove from <html> | |
const ATTRS = [ | |
"system-icons", | |
"typography", | |
"typography-spacing" | |
]; | |
// Regular config keys. | |
const CONFIGS = { | |
BUTTON_REWORK: true | |
} | |
// Experiment flags. | |
const EXPFLAGS = { | |
kevlar_system_icons: false, | |
kevlar_watch_metadata_refresh: false, | |
enable_sasde_for_html: true, | |
enable_inline_preview_controls: true, | |
il_use_view_model_logging_context: true, | |
live_chat_collapse_merch_banner: true, | |
enable_player_param_truncation_before_navigation_on_web: true, | |
enable_sasde_for_html: false, | |
web_amsterdam_playlists: false, | |
web_animated_like: false, | |
//web_animated_like_lazy_load: false, // | |
//web_button_rework: false, //biggens thumbs icon if disabled, fucks dislikes if enabled. | |
//web_darker_dark_theme: false, | |
web_guide_ui_refresh: false, | |
//web_modern_buttons: false, //Fucks dislike button if enabled | |
web_modern_ads: false, | |
web_modern_chips: false, | |
web_modern_dialogs: false, | |
web_modern_playlists: false, | |
web_modern_subscribe: false, | |
web_rounded_containers: false, | |
web_rounded_thumbnails: false, | |
web_searchbar_style: "default", | |
//web_segmented_like_dislike_button: false, | |
web_sheets_ui_refresh: false, | |
kevlar_flexible_menu: false, | |
kevlar_live_report_menu_item: true, | |
kevlar_modern_sd: false, | |
kevlar_updated_logo_icons: false, | |
kevlar_watch_cinematics: false, | |
kevlar_watch_hide_comments_teaser: false, | |
kevlar_watch_metadata_refresh: false, | |
kevlar_watch_metadata_refresh_attached_subscribe: false, | |
kevlar_watch_metadata_refresh_clickable_description: false, | |
kevlar_watch_metadata_refresh_compact_view_count: false, | |
kevlar_watch_metadata_refresh_description_info_dedicated_line: false, | |
kevlar_watch_metadata_refresh_description_inline_expander: false, //??? | |
kevlar_watch_metadata_refresh_description_lines: 3, | |
kevlar_watch_metadata_refresh_description_primary_color: false, | |
kevlar_watch_metadata_refresh_full_width_description: false, | |
kevlar_watch_metadata_refresh_relative_date: false, | |
kevlar_watch_modern_metapanel: false, | |
kevlar_watch_modern_panels: false | |
} | |
// Player flags | |
// !!! USE STRINGS FOR VALUES !!! | |
// For example: "true" instead of true | |
const PLYRFLAGS = { | |
web_player_move_autonav_toggle: "false" | |
} | |
class YTP { | |
static observer = new MutationObserver(this.onNewScript); | |
static _config = {}; | |
static isObject(item) { | |
return (item && typeof item === "object" && !Array.isArray(item)); | |
} | |
static mergeDeep(target, ...sources) { | |
if (!sources.length) return target; | |
const source = sources.shift(); | |
if (this.isObject(target) && this.isObject(source)) { | |
for (const key in source) { | |
if (this.isObject(source[key])) { | |
if (!target[key]) Object.assign(target, { [key]: {} }); | |
this.mergeDeep(target[key], source[key]); | |
} else { | |
Object.assign(target, { [key]: source[key] }); | |
} | |
} | |
} | |
return this.mergeDeep(target, ...sources); | |
} | |
static onNewScript(mutations) { | |
for (var mut of mutations) { | |
for (var node of mut.addedNodes) { | |
YTP.bruteforce(); | |
} | |
} | |
} | |
static start() { | |
this.observer.observe(document, {childList: true, subtree: true}); | |
} | |
static stop() { | |
this.observer.disconnect(); | |
} | |
static bruteforce() { | |
if (!window.yt) return; | |
if (!window.yt.config_) return; | |
this.mergeDeep(window.yt.config_, this._config); | |
} | |
static setCfg(name, value) { | |
this._config[name] = value; | |
} | |
static setCfgMulti(configs) { | |
this.mergeDeep(this._config, configs); | |
} | |
static setExp(name, value) { | |
if (!("EXPERIMENT_FLAGS" in this._config)) this._config.EXPERIMENT_FLAGS = {}; | |
this._config.EXPERIMENT_FLAGS[name] = value; | |
} | |
static setExpMulti(exps) { | |
if (!("EXPERIMENT_FLAGS" in this._config)) this._config.EXPERIMENT_FLAGS = {}; | |
this.mergeDeep(this._config.EXPERIMENT_FLAGS, exps); | |
} | |
static decodePlyrFlags(flags) { | |
var obj = {}, | |
dflags = flags.split("&"); | |
for (var i = 0; i < dflags.length; i++) { | |
var dflag = dflags[i].split("="); | |
obj[dflag[0]] = dflag[1]; | |
} | |
return obj; | |
} | |
static encodePlyrFlags(flags) { | |
var keys = Object.keys(flags), | |
response = ""; | |
for (var i = 0; i < keys.length; i++) { | |
if (i > 0) { | |
response += "&"; | |
} | |
response += keys[i] + "=" + flags[keys[i]]; | |
} | |
return response; | |
} | |
static setPlyrFlags(flags) { | |
if (!window.yt) return; | |
if (!window.yt.config_) return; | |
if (!window.yt.config_.WEB_PLAYER_CONTEXT_CONFIGS) return; | |
var conCfgs = window.yt.config_.WEB_PLAYER_CONTEXT_CONFIGS; | |
if (!("WEB_PLAYER_CONTEXT_CONFIGS" in this._config)) this._config.WEB_PLAYER_CONTEXT_CONFIGS = {}; | |
for (var cfg in conCfgs) { | |
var dflags = this.decodePlyrFlags(conCfgs[cfg].serializedExperimentFlags); | |
this.mergeDeep(dflags, flags); | |
this._config.WEB_PLAYER_CONTEXT_CONFIGS[cfg] = { | |
serializedExperimentFlags: this.encodePlyrFlags(dflags) | |
} | |
} | |
} | |
} | |
window.addEventListener("yt-page-data-updated", function tmp() { | |
YTP.stop(); | |
for (i = 0; i < ATTRS.length; i++) { | |
document.getElementsByTagName("html")[0].removeAttribute(ATTRS[i]); | |
} | |
window.removeEventListener("yt-page-date-updated", tmp); | |
}); | |
YTP.start(); | |
YTP.setCfgMulti(CONFIGS); | |
YTP.setExpMulti(EXPFLAGS); | |
YTP.setPlyrFlags(PLYRFLAGS); |
I have no idea. I gave up because firemonkey was pissing me off so much and just use violentmonkey now. I suspect tampermonkey is closed source just because it can handle stupid shit like this for no reason to dominate the userscript 'market'.
Either way, this script is just an edit of https://raw.githubusercontent.com/aubymori/YouTubePatchCollection/main/YouTubePatchCollection.user.js so I'd ask her
Stopped using tampermonkey for the same reason a couple of years ago, violentmonkey works flawlessly.
I've heard Violentmonkey has had security/privacy issues in the past, which is why I wanted to go with FireMonkey.
That said, I may swap to ViolentMonkey for now, and submit this to the Compatability Issues section for FireMonkey.
Thanks anyway!
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hey, thank you so much for this! It worked great on TamperMonkey but I just switched to FireMonkey and there's a lot of issues suddenly, mostly just "best practice" nags admittedly:
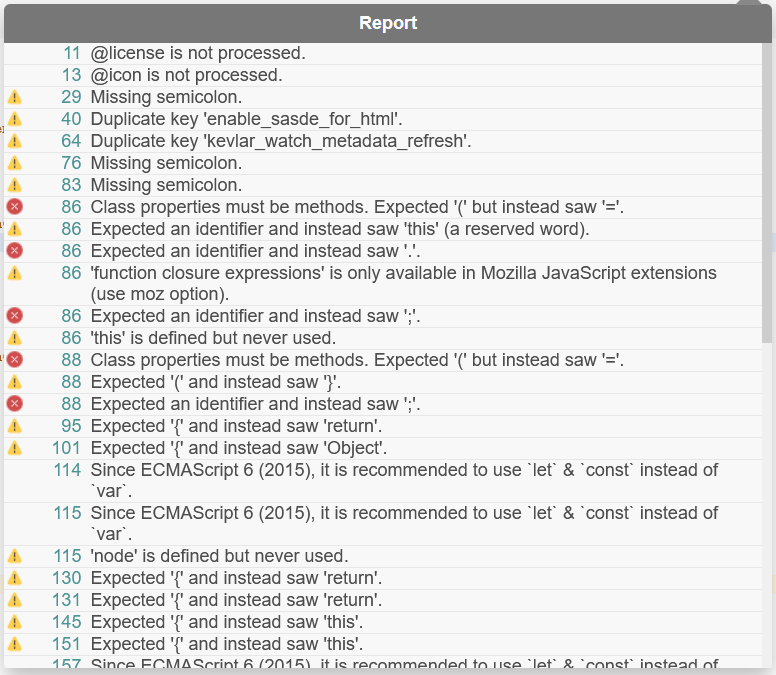
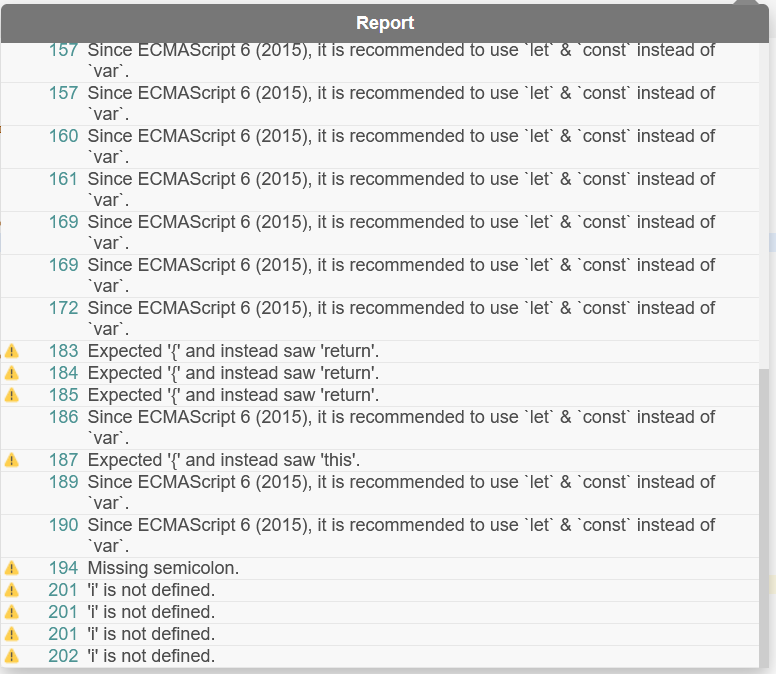
I could fix pretty much all of these really easily, only took a couple minutes of going through and updating things... but...
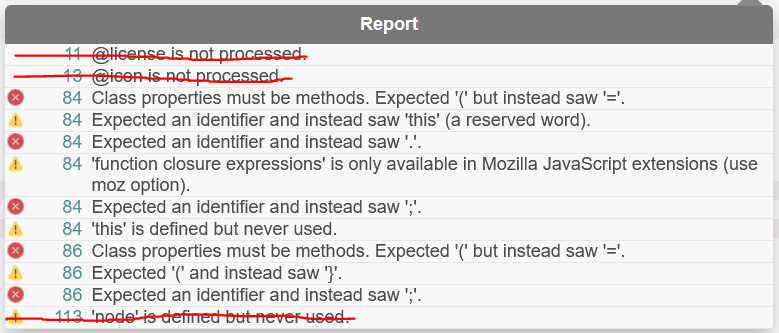
there are some major issues, presumably what's stopping it from loading, and I'm not sure what to do to fix them.
Any idea what I could do to get around the issues with class properties? Not sure why it's only letting methods work, my searches came up with something about classes not being fully implemented in Javascript, but if that's the case I don't get why it works in TamperMonkey.